In C++, a handle is typically a reference to a resource, allowing for efficient management of memory and object lifetimes during runtime.
Here’s a simple example using a smart pointer, which acts as a handle to dynamically allocated memory:
#include <iostream>
#include <memory>
int main() {
std::unique_ptr<int> handle = std::make_unique<int>(42);
std::cout << "Value: " << *handle << std::endl; // Output: Value: 42
return 0;
}
Understanding Handles in C++
What is a Handle?
In C++, a handle is an abstract reference to a resource, utilized primarily to manage the resource's lifecycle without exposing the details of its representation. This allows programmers to work with different kinds of resources, such as files, graphical windows, or custom objects, without having to manage the memory directly. Handles are particularly important in resource-constrained environments, enhancing both safety and efficiency.
Why Use Handles?
There are several compelling reasons to use handles in your C++ code:
- Abstraction: Handles abstract the underlying resource, enabling you to focus on higher-level programming rather than details of resource management.
- Memory Safety: Using handles can help avoid memory corruption, dangling pointers, and memory leaks, making your applications more robust.
- Performance: Handles can provide a method for efficiently managing resource lifetimes while allowing for control over resource management strategies.
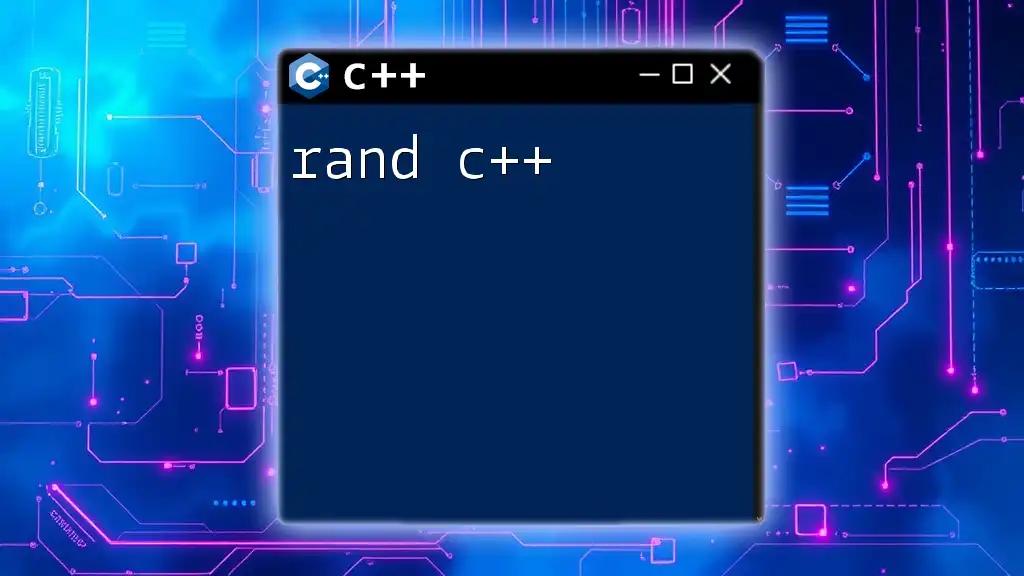
Common Types of Handles in C++
File Handles
Definition and Purpose
A file handle allows you to interact with files in your operating system. When you open a file, you receive a handle, which you then use for various operations such as reading, writing, or closing the file. This encourages a standardized approach to file management.
Example Code Snippet
#include <fstream>
#include <iostream>
int main() {
std::fstream fileHandle;
fileHandle.open("example.txt", std::ios::out);
if (fileHandle.is_open()) {
std::cout << "File opened successfully!" << std::endl;
fileHandle << "Hello, World!";
} else {
std::cout << "Failed to open file." << std::endl;
}
fileHandle.close();
return 0;
}
Error Handling with File Handles
When working with file handles, it is critical to confirm whether a file has been opened successfully. By checking if the file handle is valid, you avoid potential runtime errors. Exception handling can further enhance error management by catching issues that arise during file operations.
Window Handles (WinAPI)
Overview of Windows Handles
In Windows API programming, a window handle (HWND) represents a window or a control. It provides a way to interact with GUI components and is essential for GUI-based applications in C++.
Example Code Snippet
#include <windows.h>
int main() {
HWND hwnd = FindWindow(NULL, "MyWindow");
if (hwnd) {
std::cout << "Window handle obtained!" << std::endl;
} else {
std::cout << "Failed to obtain window handle." << std::endl;
}
return 0;
}
Use Cases for Window Handles
Window handles are pivotal in many GUI applications, allowing for operations like displaying, hiding, and updating components based on user interactions. Understanding how to manipulate these handles is vital for successful GUI programming.
Handle for Custom Objects
Creating and Using Custom Handles
Custom handles can be defined for your own data types, enhancing flexibility. Below is an example demonstrating how to create and utilize a handle for a custom class.
class MyObject {
public:
MyObject() {}
~MyObject() {}
};
using MyObjectHandle = MyObject*;
void useHandle(MyObjectHandle handle) {
if(handle) {
// Operations using handle
}
}
int main() {
MyObjectHandle handle = new MyObject();
useHandle(handle);
delete handle; // Always clean up
return 0;
}
Smart Pointers as Handles
Smart pointers, namely `std::unique_ptr` and `std::shared_ptr`, are modern alternatives to raw pointers for representing handles. They automatically manage resource lifetimes, thereby reducing the risks associated with manual memory management, such as leaks and dangling pointers.
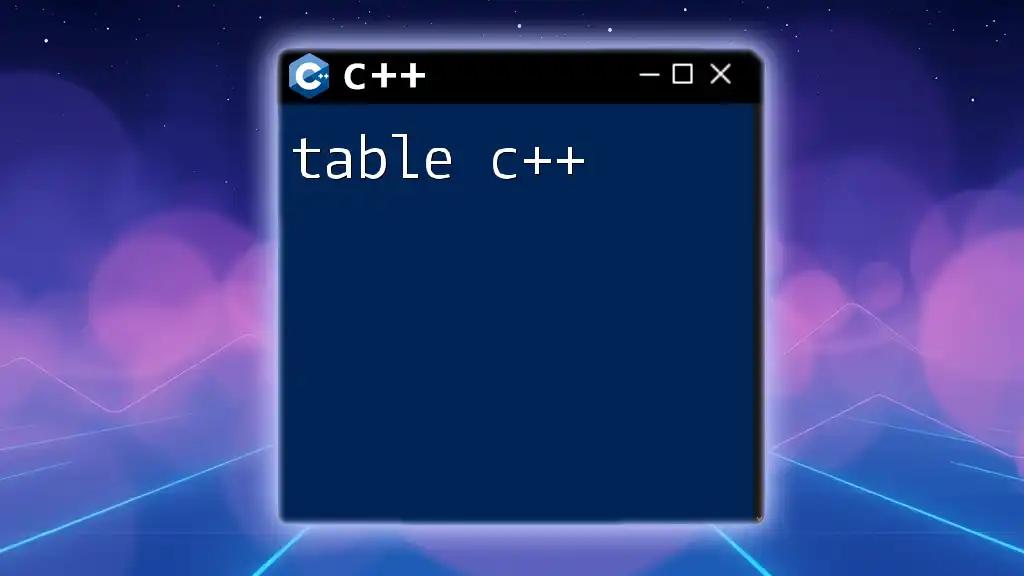
Best Practices for Working with Handles in C++
Memory Management
Effective memory management is crucial when dealing with handles. Improper management can lead to many issues, such as resource leaks or program crashes. Implementing Resource Acquisition Is Initialization (RAII) is a golden rule; allocate resources during object construction and release them through destructors.
Exception Safety
When using handles, be mindful of the potential for exceptions. Envelop your operations in `try-catch` blocks allowing you to gracefully handle exceptions without leaving your resources in a corrupted state. This ensures resource integrity even when unexpected behaviors occur.
Performance Considerations
While handles provide a level of abstraction that eases resource management, there can be performance overheads associated with them. Here, consider the following guidelines:
- Use handles when the overhead is justified: For high-performance applications, evaluate whether the benefits of using handles outweigh potential performance costs.
- Direct memory access: In critical performance paths, consider whether direct memory access is more appropriate than using handles.
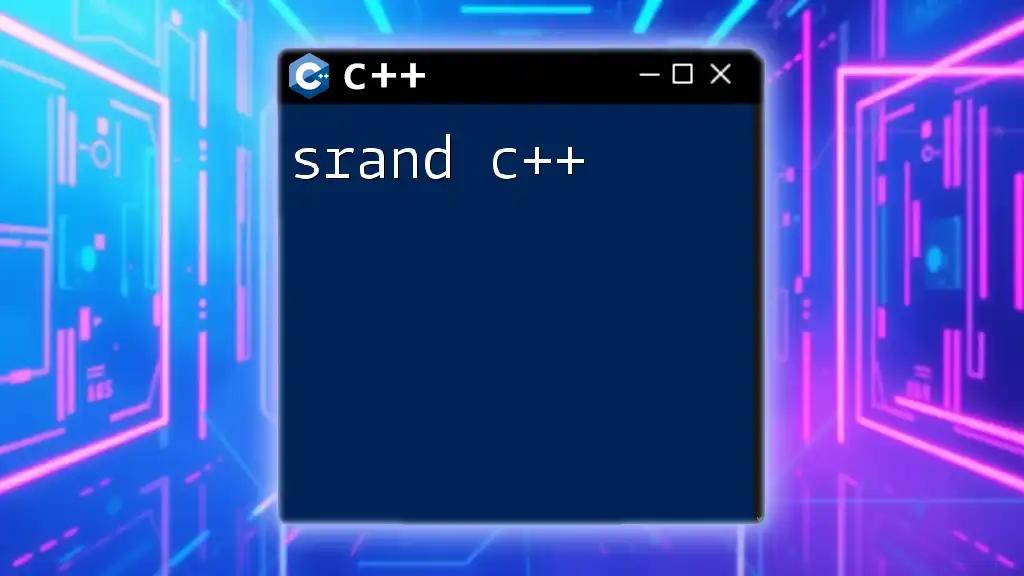
Conclusion
Handling resources effectively is a core skill in C++ programming. Understanding the nature of handles and how they facilitate better resource management can significantly enhance the quality and robustness of your code. By following best practices and leveraging modern techniques such as smart pointers, you can ensure efficient and safe resource management in your applications.
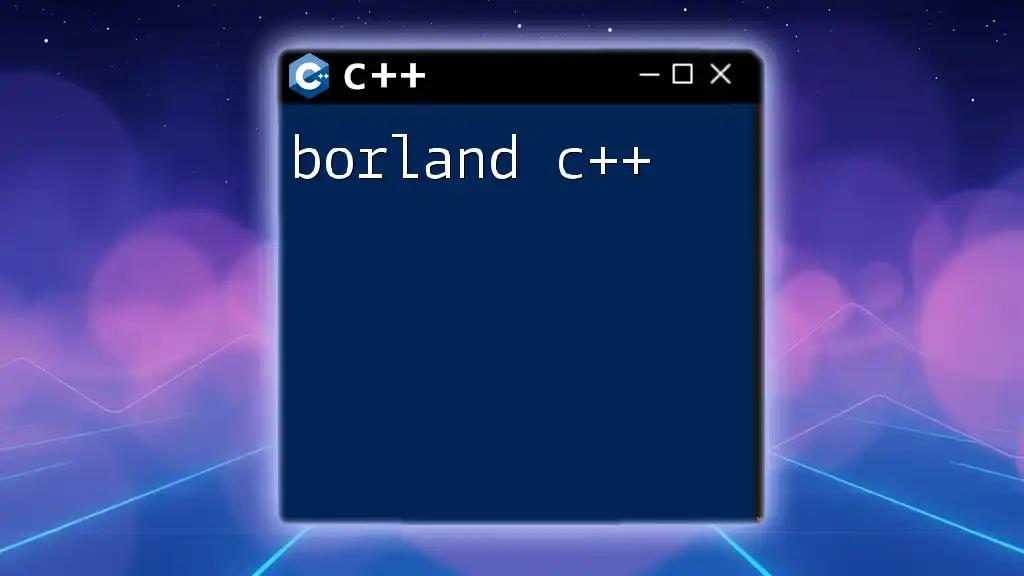
Additional Resources
For further enlightenment on handles in C++, explore the official documentation and consider delving into books or online courses designed to strengthen your understanding of resource management. Engaging in community forums can also provide valuable insights and support as you progress in your programming journey.