OnlineGDB is an online compiler and debugger that allows users to write, execute, and debug C++ code directly in their web browser, making it accessible for quick learning and testing.
Here’s an example code snippet that demonstrates a simple "Hello, World!" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is OnlineGDB?
OnlineGDB is an innovative online integrated development environment (IDE) that allows users to write, compile, and debug code in a variety of programming languages, including C++. This platform excels due to its user-friendly interface and robust functionality, making it suitable for both beginners and experienced developers.
Why Use OnlineGDB for C++?
The advantages of using OnlineGDB for C++ programming are numerous. One of the key reasons to choose this platform is the accessibility it offers. With no installation required, you can start coding in seconds from any device with an internet connection.
Additionally, OnlineGDB features collaborative tools, allowing multiple users to work on the same project in real time. This is particularly beneficial for classroom settings, team projects, or remote coding sessions.
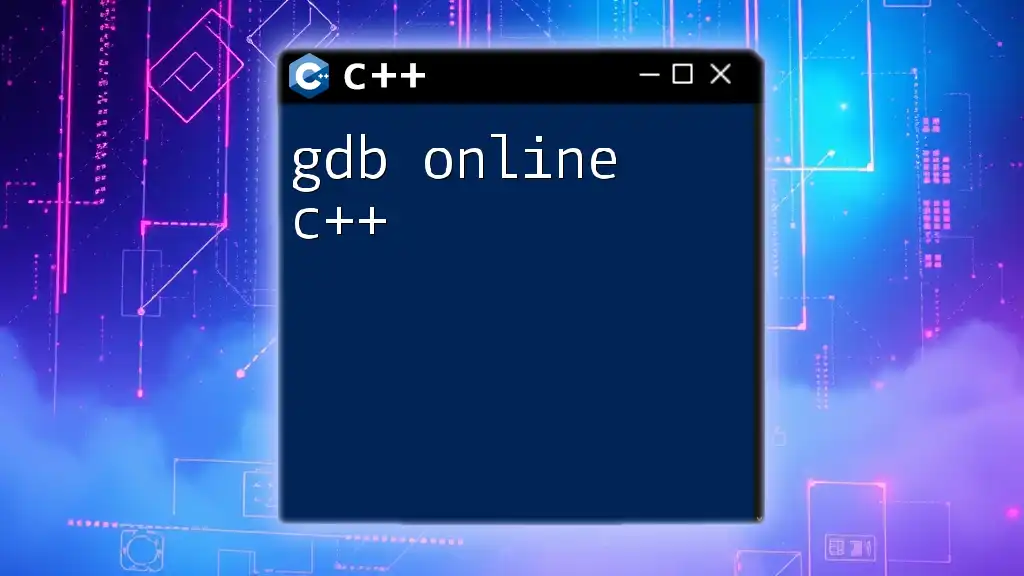
Getting Started with OnlineGDB
Creating Your Account
To begin using OnlineGDB, creating an account is a straightforward process. Simply navigate to the website, click on the "Sign Up" option, and follow the prompts to input your information. While an account isn't strictly necessary to write code, having one enables you to save your projects for future use and easily share them with peers.
Navigating the OnlineGDB Interface
Once you're logged in, you'll find yourself on the dashboard, where you'll see options to create new files, access past projects, and explore sample codes. The coding environment is intuitive, with a menu on the left for navigation, an editor window in the center for writing your C++ code, and output and debugging panels that will display results and errors during execution.
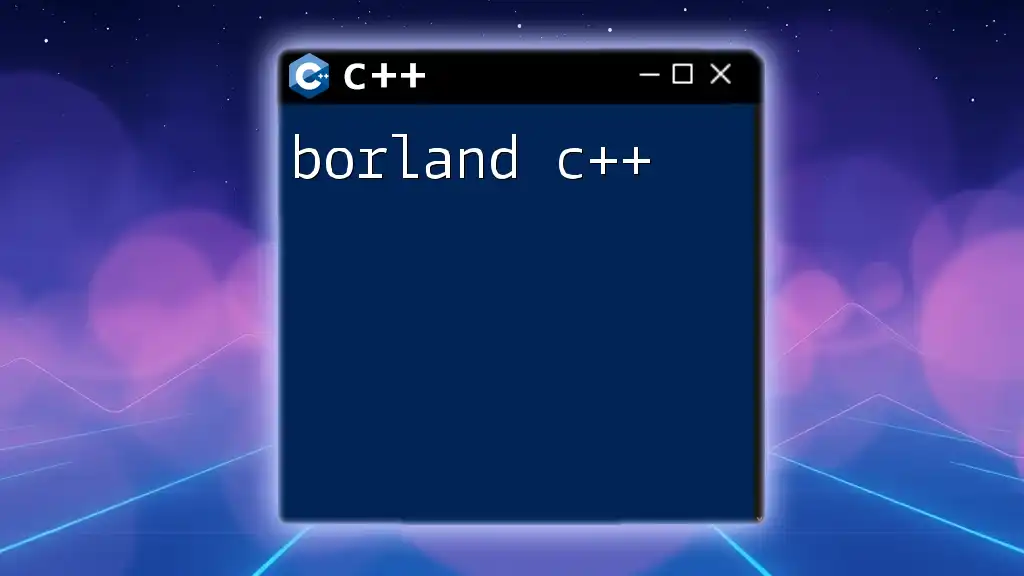
Writing Your First C++ Program on OnlineGDB
Setting Up Your Workspace
To start coding in C++, make sure you select C++ as your chosen programming language in the IDE. You can also select specific compiler versions and set options that dictate how your code is compiled and run.
Basic Syntax and Code Structure
Understanding the basic syntax of C++ is crucial for a smooth coding experience. Here is an example of a very simple C++ program that outputs "Hello, World!" to the console:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this code:
- `#include <iostream>` imports the standard input-output library.
- `using namespace std;` allows us to use names from the standard library without prefixing them with `std::`.
- The `main` function is the entry point of every C++ program.
- `cout` is used to print output to the console, followed by `return 0;` which indicates that the program ended successfully.
Running Your Code
After you have written your code, the next step is to compile and run it. OnlineGDB provides a button for this at the top of the editor. When you click on it, the IDE will compile your code and display any errors in the output panel. If your code is error-free, you will see the output of your program on the screen.
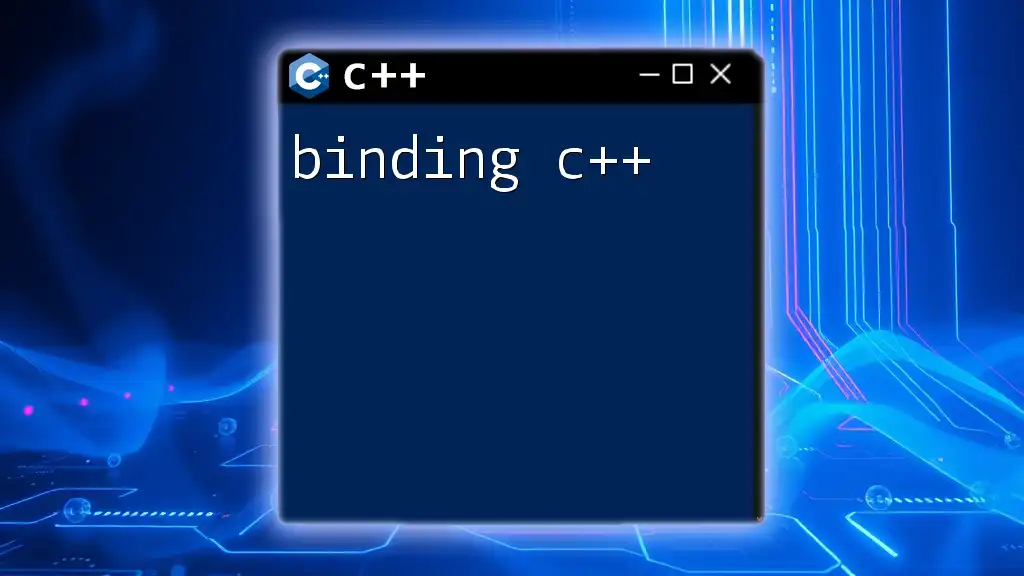
Features of OnlineGDB for C++
Built-in Compiler and Debugger
One of the standout features of OnlineGDB is its integrated compiler and debugger. This built-in compiler supports various C++ standards, which is vital for modern C++ programming.
Debugging on OnlineGDB is intuitive; simply use the debugger tools provided to set breakpoints and inspect variables. For example, if your program isn't behaving as expected, you can pause its execution at a certain line and step through the code to identify where things go awry.
Collaboration Tools
OnlineGDB makes collaboration easier than ever. You can share your code with others by producing a shareable link. This feature is especially valuable for group projects or when seeking help from mentors. Multiple users can open the same code file simultaneously, allowing for real-time discussion and coding.
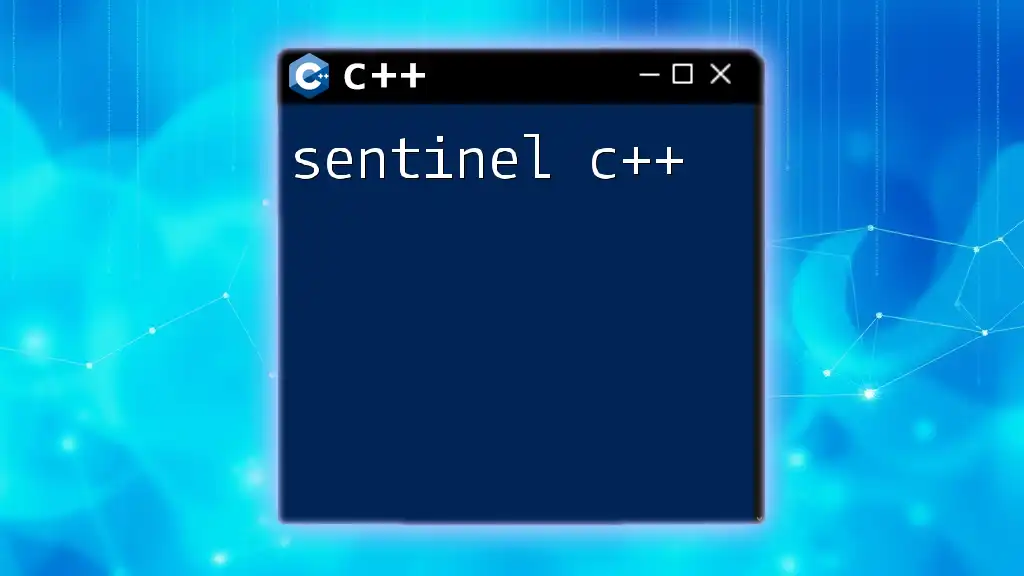
Advanced Features of OnlineGDB
Customizing Your Workspace
Customization is key to enhancing your coding experience. OnlineGDB allows you to set preferences for your coding environment. You can choose a light or dark theme based on your visual comfort and adjust the layout to better suit your workflow.
Using Libraries and Including Headers
Working with libraries in C++ expands your coding capabilities. By including different header files, you can utilize a plethora of functions and data structures. Consider a code example that uses the Standard Template Library (STL):
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int number : numbers) {
std::cout << number << " ";
}
return 0;
}
In this example:
- `#include <vector>` allows you to use the vector container, a part of the STL.
- The for-each loop iterates through the vector and prints each number.
File Handling in OnlineGDB
Understanding file operations is vital for more advanced C++ programming. OnlineGDB supports basic file handling. Here is an example that showcases both writing to and reading from a file:
#include <iostream>
#include <fstream>
int main() {
std::ofstream outFile("example.txt");
outFile << "Writing to a file." << std::endl;
outFile.close();
std::ifstream inFile("example.txt");
std::string line;
while (getline(inFile, line)) {
std::cout << line << std::endl;
}
inFile.close();
return 0;
}
In this code:
- Using `std::ofstream`, we create a file called example.txt and write a line into it.
- The subsequent block uses `std::ifstream` to read from the same file and print out the contents line by line.
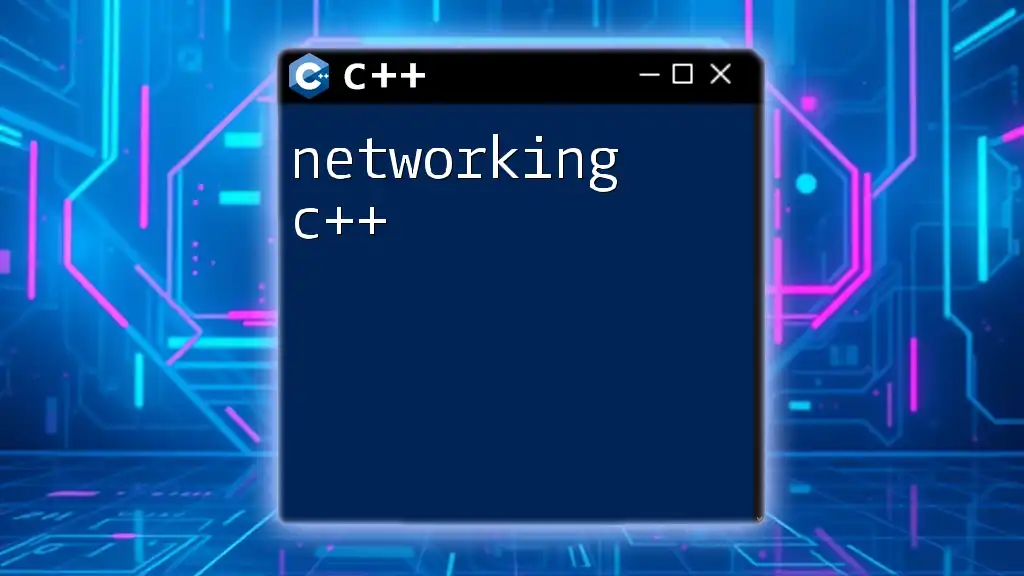
Tips and Best Practices for Using OnlineGDB
Efficient Coding Techniques
To maximize your productivity, practice writing clean and organized code. Always include comments to clarify your intentions. This will make your code easier to read and maintain - both for yourself and others who may review it.
Troubleshooting Common Issues
As you embark on your C++ programming journey, you may encounter common pitfalls. One common error is forgetting to include necessary headers; always ensure your libraries are properly imported. Compile errors often stem from syntax mistakes, so take the time to read any error messages generated by OnlineGDB carefully.
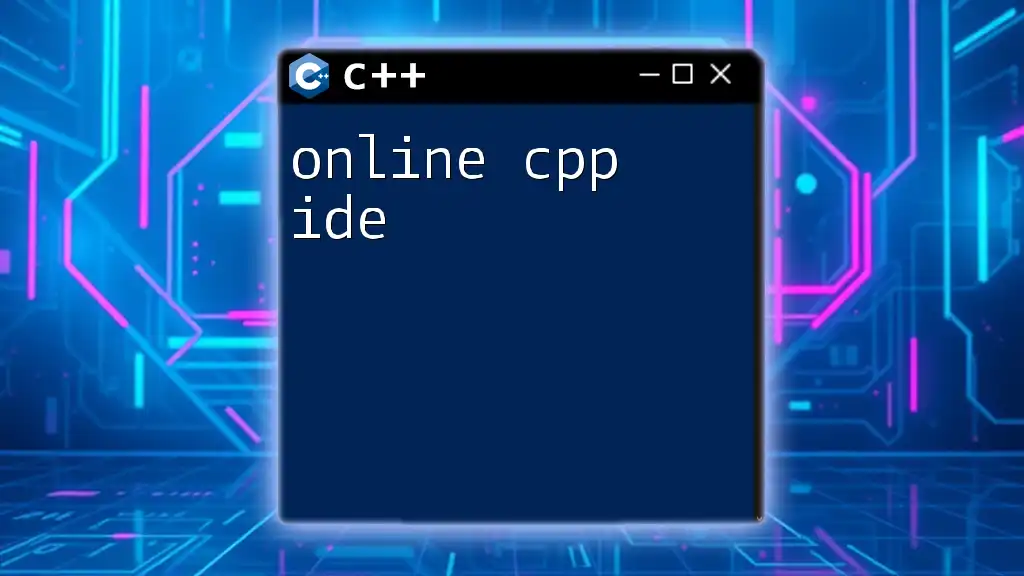
The Future of Online C++ Programming
In conclusion, OnlineGDB offers a powerful platform for anyone interested in learning or advancing their C++ programming skills. The ease of use, accessibility, and collaborative features all make it an invaluable tool for coders of all levels. By embracing OnlineGDB, you are positioning yourself to take full advantage of modern coding practices.
Additional Resources
For further learning, explore various tutorials, forums, and resources dedicated to C++. Engaging with online communities can provide insights, support, and additional learning materials to enhance your coding journey.