GDB (GNU Debugger) is a powerful tool that allows developers to debug their C++ code online, enabling step-by-step execution to identify and fix errors efficiently.
Here’s a simple code snippet demonstrating how to run a C++ program using GDB:
gdb a.out
run
break main
next
print variable_name
In this example, replace `variable_name` with the actual variable you want to inspect during debugging.
What is GDB?
GDB, the GNU Debugger, is a powerful debugging tool for C and C++ programs. It allows developers to see what is happening inside a program while it executes or what a program was doing at the moment it crashed. Debugging is a critical part of development, as it facilitates identifying and fixing bugs that could lead to unwanted behavior, crashes, or vulnerabilities in applications.
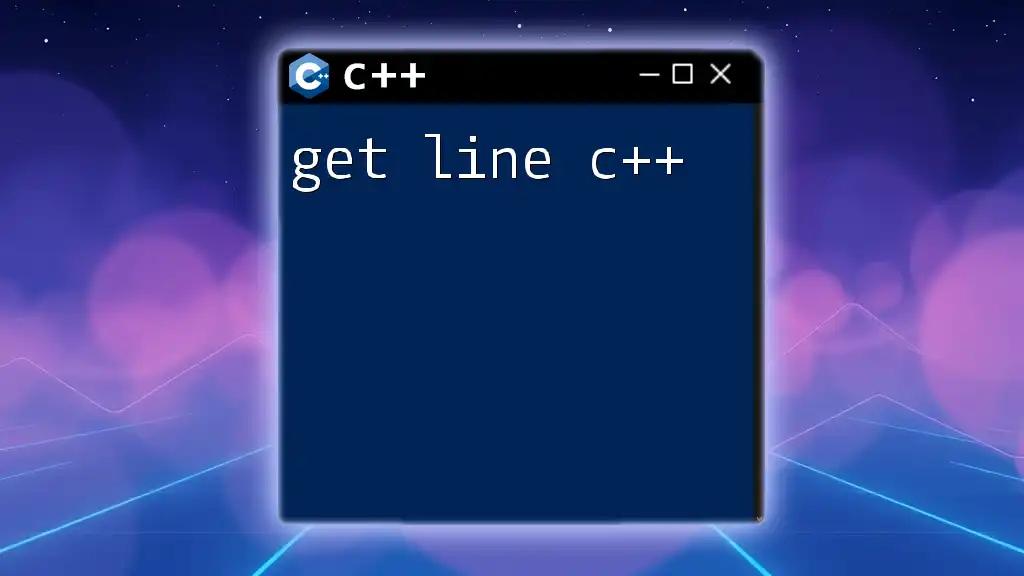
Why Use Online Compilers for GDB?
Online GDB compilers provide a simple and accessible way to use GDB without needing to set up a local development environment. The advantages of using online GDB tools include:
- Accessibility: Easily accessible from any device with an internet connection.
- Ease of Use: They require minimal setup and configuration, perfect for beginners.
- Collaboration: Ideal for sharing code quickly with others for educational purposes or support.
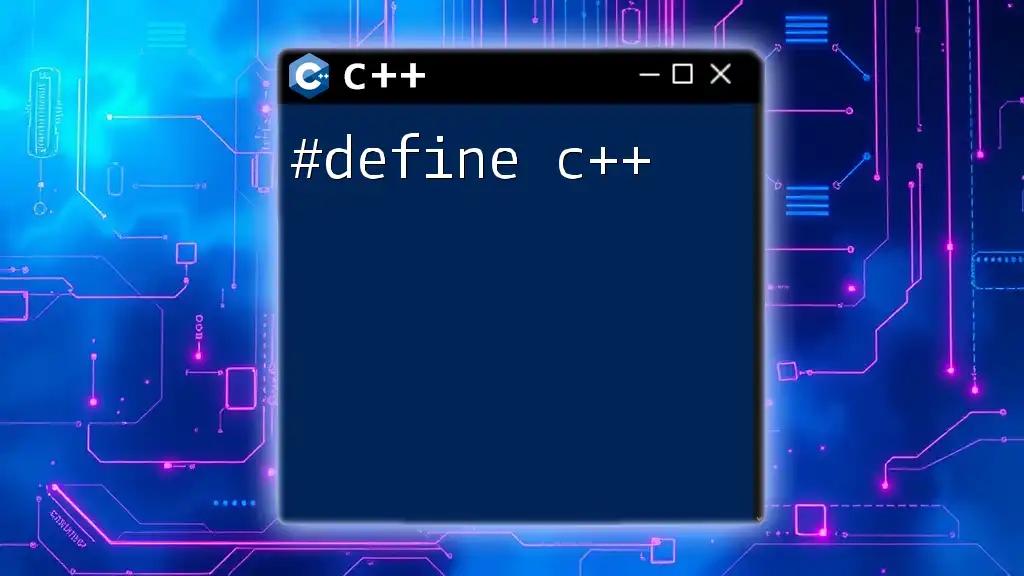
Choosing an Online GDB Compiler
Several options are available for running GDB in an online environment. When choosing an online GDB compiler, look for the following features:
- User-Friendly Interface: An intuitive layout that makes navigating tools and features simple.
- Real-Time Error Reporting: Immediate feedback regarding syntax errors or runtime issues.
- Multi-File Support: Ability to handle larger projects that consist of multiple files.
- Support for Additional Libraries: Access to various libraries and functionalities that can enhance debugging.
Some popular online GDB platforms include Replit, Ideone, and CPP.sh. Each platform comes with its distinct features and user experience, which can significantly affect your debugging journey.
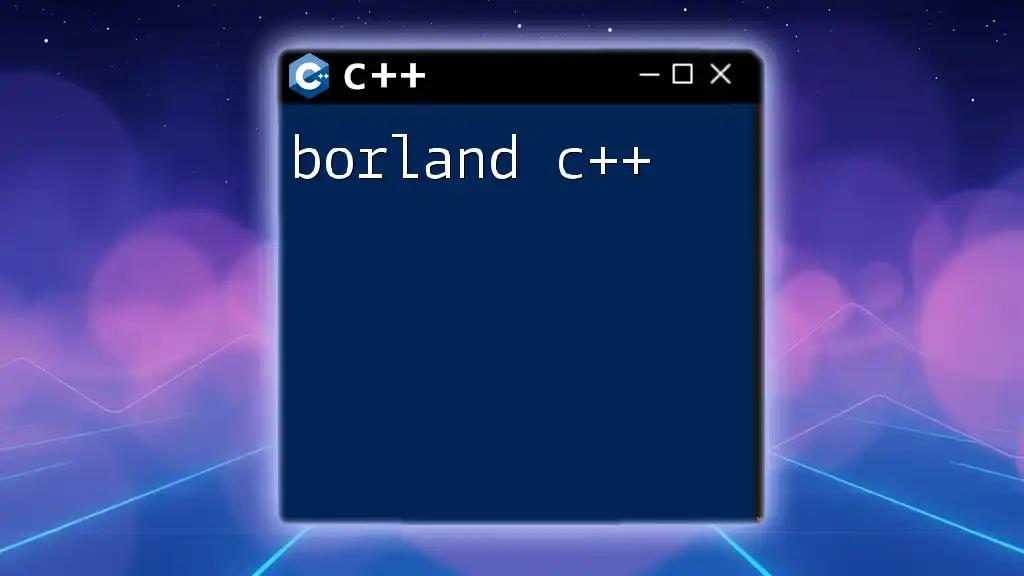
Setting Up Your Environment
To start using GDB online, follow these brief steps:
- Access the Compiler: Navigate to your chosen online GDB platform (e.g., Replit or IDEOne).
- Create an Account: Sign up for an account if necessary or use a guest access mode if available.
- Select C++ as the Programming Language: Choose the language you wish to debug - in this case, C++.

Starting the GDB Session
Once you’ve set up your environment, you can initiate a GDB session by running the command:
gdb program_name
This command opens GDB with the specified program, ready for debugging. Understanding how to navigate this environment is key to effectively using GDB.
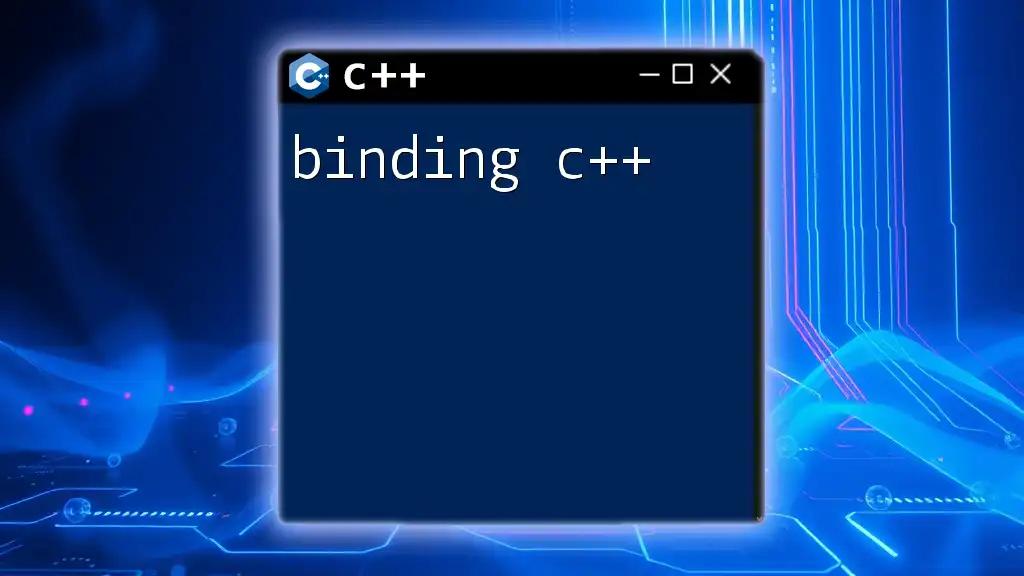
Essential Commands for Beginners
To maximize the usefulness of GDB, you'll need to familiarize yourself with some fundamental commands:
Running a Program
To begin debugging, you first need to run your program. Here’s a simple example:
int main() {
int a = 5;
int b = 0;
return a / b; // This will cause a division by zero error
}
In GDB, use the command `run` to execute your program. When it hits a runtime error, GDB will pause execution, allowing you to investigate the issue.
Setting Breakpoints
Breakpoints are essential for stopping program execution at specific lines of code, enabling you to inspect the state of the program. To set a breakpoint, use the following command:
break line_number
For example, if you want to stop execution at line 3, use:
break 3
Navigating through Code
Once a breakpoint is hit, you can navigate through your code using several commands:
- run: Starts the program.
- step: Executes the current line and moves to the next line, stepping into any called functions.
- next: Executes the current line but skips over function calls.
These commands allow you to control the flow of your program execution closely.
Inspecting Variables
To check the value of variables at a breakpoint, you can utilize the `print` command:
print variable_name
For instance, to inspect the variable `a`, use:
print a
This command allows you to see the state of your variables and understand the flow of your program better.
Examining Call Stacks
Understanding the call stack is crucial when dealing with complex programs. The command `backtrace` provides a snapshot of function calls leading to the current point in the program. This allows you to see how the program reached its current state, aiding in tracing back errors and unexpected behaviors.
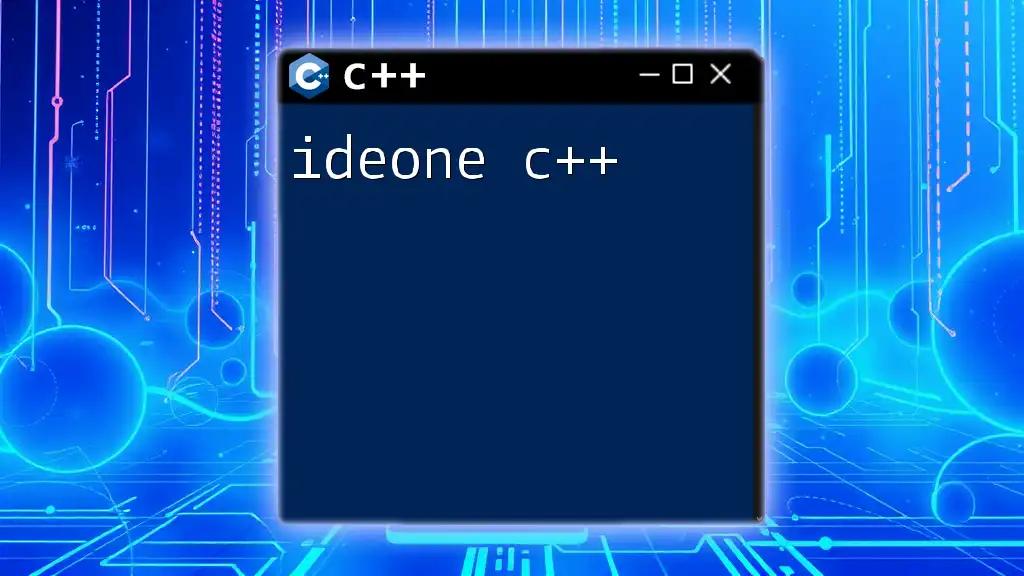
Advanced GDB Features
As you become more proficient with GDB, you may want to explore some advanced capabilities.
Conditional Breakpoints
These breakpoints stop execution only if a specified condition is met. This is useful when debugging loops or recursive functions. The syntax for setting a conditional breakpoint is:
break line_number if condition
For example:
break 10 if a == 5
This will only trigger the breakpoint at line 10 if `a` equals 5.
Watchpoints
Watchpoints monitor specific variables and stop execution when their values change. If you suspect a variable's value is being modified unexpectedly, use a watchpoint:
watch variable_name
This feature allows you to track down issues related to variable changes effectively.
Core Dumps and their Debugging
A core dump is a file that records the memory of a running program at a specific point, usually upon crashing. To handle core dumps, you can use GDB like so:
gdb program_name core
This command enables you to analyze the state of your program at the time of the crash, providing invaluable insight into debugging.
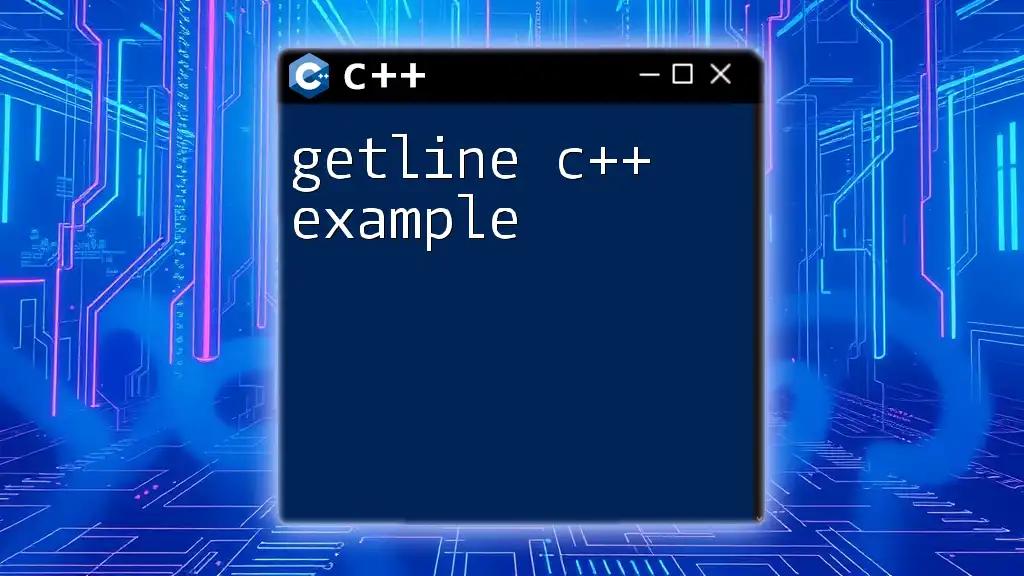
Debugging Common C++ Errors with GDB
Segmentation Faults
A segmentation fault occurs when a program attempts to access memory that it's not allowed to. You can use GDB to trace back the source of the segmentation fault by using the `backtrace` command when the error occurs. This lets you see the stack of function calls leading up to the fault, making it easier to identify the culprit.
Memory Leaks
Memory leaks occur when memory allocated during execution is not properly released. GDB can help identify such leaks by tracking memory allocation and deallocation in your program. Look for parts where allocated memory is not freed and ensure proper use of `delete` or `delete[]` in C++.

Tips and Tricks for Efficient Debugging
GDB Shortcuts
Familiarize yourself with useful shortcuts to enhance your debugging efficiency. For example, using `Ctrl + C` to interrupt a running program can save time during long processes.
Best Practices in Debugging with GDB
Consistently document your findings when debugging. This habit can help you keep track of issues and solutions, serving as a valuable resource for future projects.
Common Pitfalls in GDB Usage
Avoid relying solely on GDB for finding bugs; combine it with thorough testing and code reviews. Remember that a good debugging strategy incorporates multiple approaches to identify and fix issues effectively.

Recap of GDB and Online Debugging Benefits
In this guide, we’ve explored the vast capabilities of gdb online c++. Understanding GDB and its functionalities empowers developers to debug their C++ applications efficiently. GDB not only aids in fixing current issues but also helps develop better coding habits.

Encouragement to Practice
Don't hesitate to dive into GDB and start debugging your C++ code today. Practice makes perfect, and with GDB's comprehensive tools at your disposal, you can tackle whatever challenges come your way!
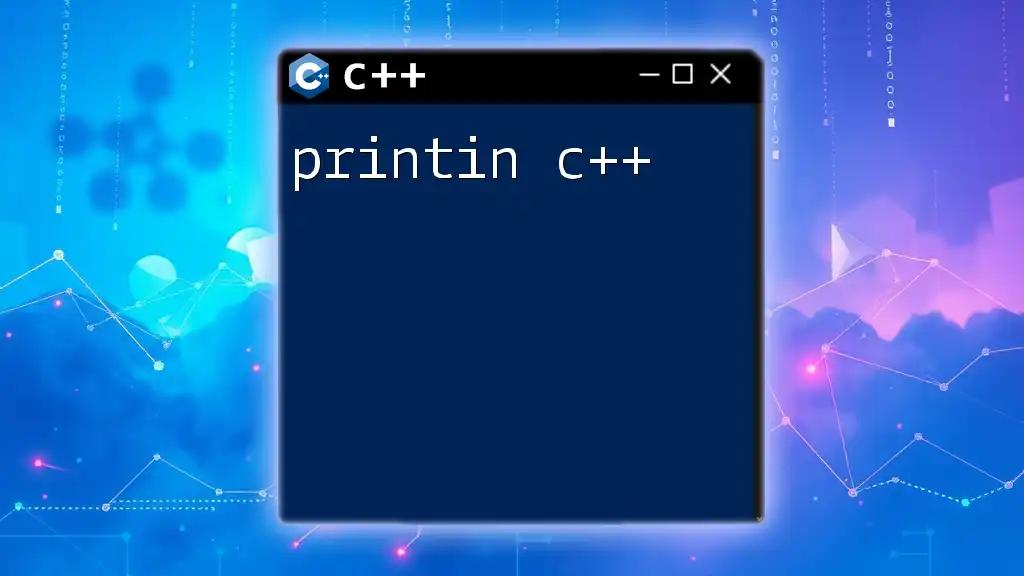
Additional Resources
Further your knowledge by exploring the official GDB documentation, recommended books, and online courses focused on C++ debugging. Joining community forums can also provide further insights and support as you continue your learning journey.