`#pragma once` is a preprocessor directive in C++ that ensures a header file is included only once in a single compilation, preventing duplicate definitions and reducing compilation time.
#pragma once
// Sample header file contents
class Example {
public:
void display();
};
Understanding Include Guards
What are Include Guards?
Include guards are a crucial feature in C++ that helps prevent multiple definitions during the compilation process. When the same header file is included more than once in a compilation unit, it can lead to redefinition errors. Include guards help in resolving this problem by ensuring that the compiler only processes the header file once in a single compilation context.
Traditional Include Guard Syntax
The traditional method of implementing include guards relies on preprocessor directives. Below is an example of how this method is typically structured:
#ifndef MY_HEADER_H
#define MY_HEADER_H
// Declarations and Definitions
#endif // MY_HEADER_H
In this example:
- `#ifndef` checks if `MY_HEADER_H` has not been defined.
- If it's not defined, `#define MY_HEADER_H` is executed, marking that this header file is being processed.
- Finally, the `#endif` directive signals the end of the guard. If the header file is included again, the compiler skips the contents.
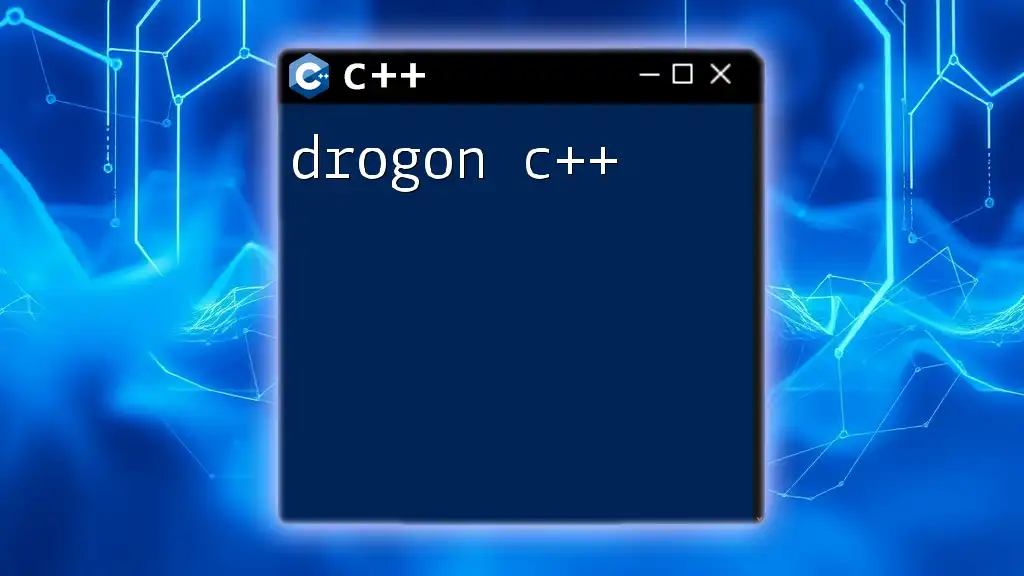
What is `#pragma once`?
Definition
`#pragma once` is a preprocessor directive that simplifies the process of preventing multiple inclusions of the same header file. It serves as an instruction to the compiler to include the header file only once per compilation unit, ensuring optimal performance and code clarity.
Syntax
Using `#pragma once` is straightforward. The syntax is as follows:
#pragma once
// Declarations and Definitions
This single line of code replaces the traditional include guard approach, offering a cleaner and more concise method for managing header files.
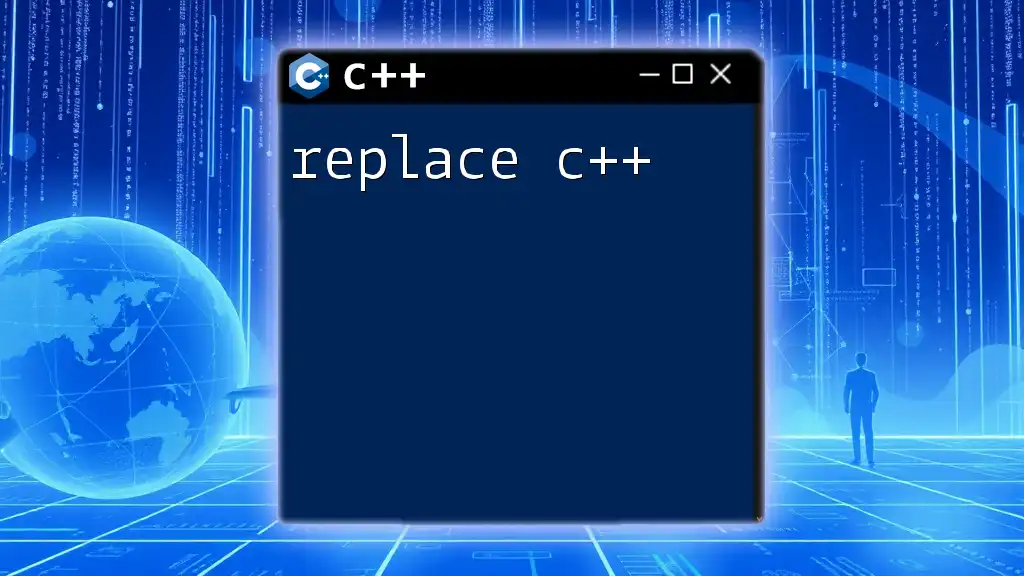
Benefits of Using `#pragma once`
Simplifies Code
One of the most significant advantages of `#pragma once` is how it simplifies your code. With fewer lines of boilerplate code, your header files become cleaner and easier to read. The logic behind inclusion control becomes evident at first glance, allowing you to focus on declarations and definitions without getting bogged down in preprocessor directives.
Performance Benefits
Using `#pragma once` can lead to performance improvements during the compilation process. The compiler is able to manage header files more efficiently, as it only checks for their inclusion state once, rather than processing the conditional guards for every inclusion. This reduction in overhead can aid in speeding up the build times, particularly in larger projects with extensive header files.
Portability
Most modern compilers, including GCC, Clang, and MSVC, support `#pragma once`. This widespread adoption makes it a reliable option for most development environments. Its ease of use, combined with compiler compatibility, greatly enhances the portability of your code across different platforms and tools.
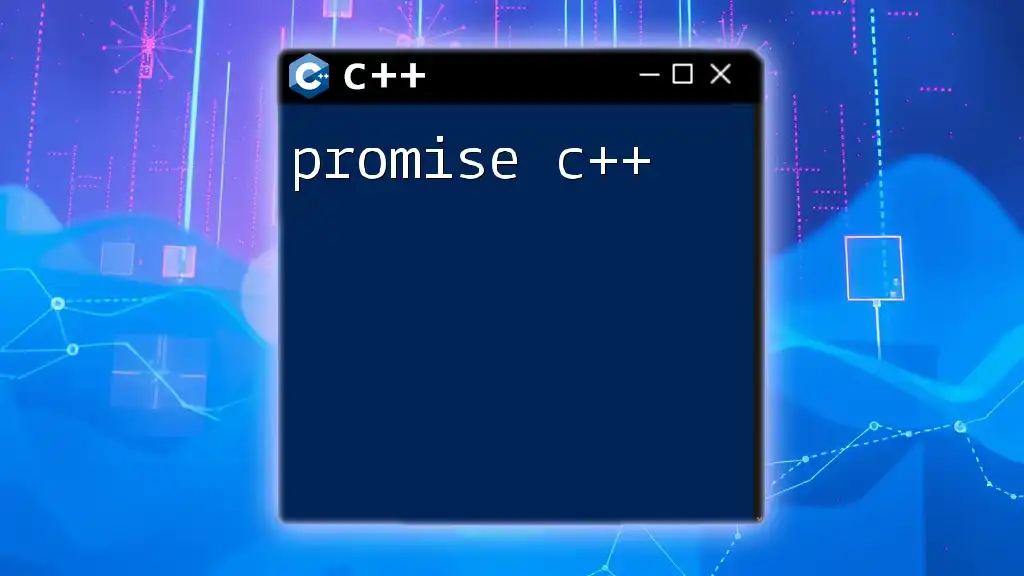
Potential Drawbacks of `#pragma once`
Compiler Dependency
While `#pragma once` is commonly supported, its behavior is not standardized across all compilers. Although major compilers recognize and efficiently handle this directive, some lesser-known or older compilers may not support it, potentially leading to compatibility issues. Developers should be cautious when working in environments that may not fully support modern C++ features.
File System Dependency
Another potential drawback of `#pragma once` involves file systems. In some situations, particularly with network file systems or case-insensitive file systems, `#pragma once` may fail to recognize that the same file is being included multiple times. This issue arises because the directive relies on the file's path and can be misled by variations in case or path structure. Therefore, it's essential to be aware of your development environment and file system when using `#pragma once`.
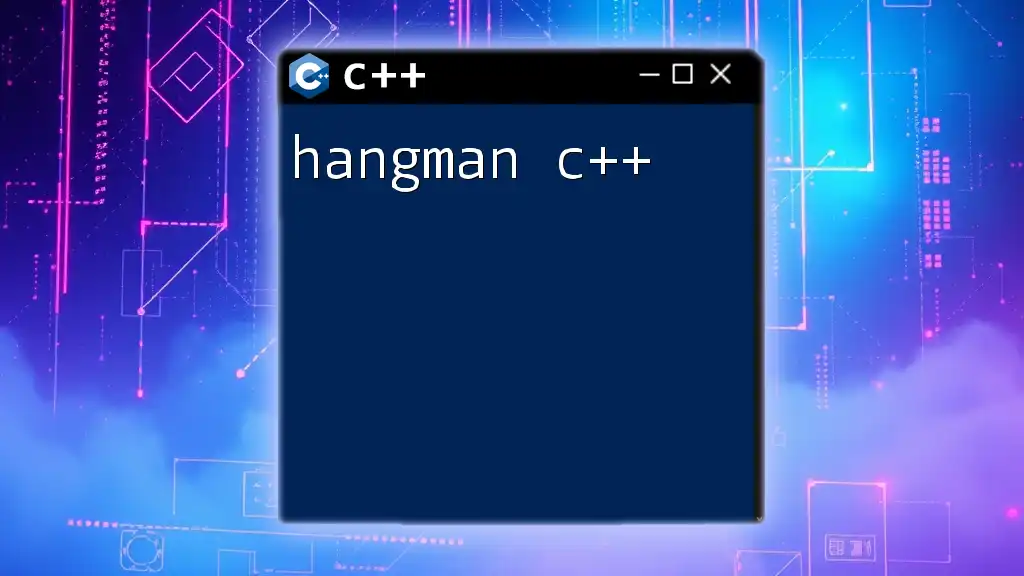
Best Practices for Using `#pragma once`
When to Use
`#pragma once` is best utilized in new projects where code clarity and compile-time performance are priorities. It is particularly advantageous in modern C++ because it aligns with contemporary coding practices. However, in legacy projects or situations where multiple compilers are in use, clarity on whether to employ `#pragma once` versus traditional include guards is necessary.
Combining with Traditional Include Guards
In some cases, employing both `#pragma once` and traditional include guards can provide extra safety, especially when transitioning older codebases. Here's how you might structure a header file using both:
#pragma once
#ifndef MY_HEADER_H
// Declarations and Definitions
#endif // MY_HEADER_H
In this configuration:
- `#pragma once` ensures that the file is included only once, improving performance.
- The traditional include guard serves as a secondary line of defense for compilers that might not fully support `#pragma once`.
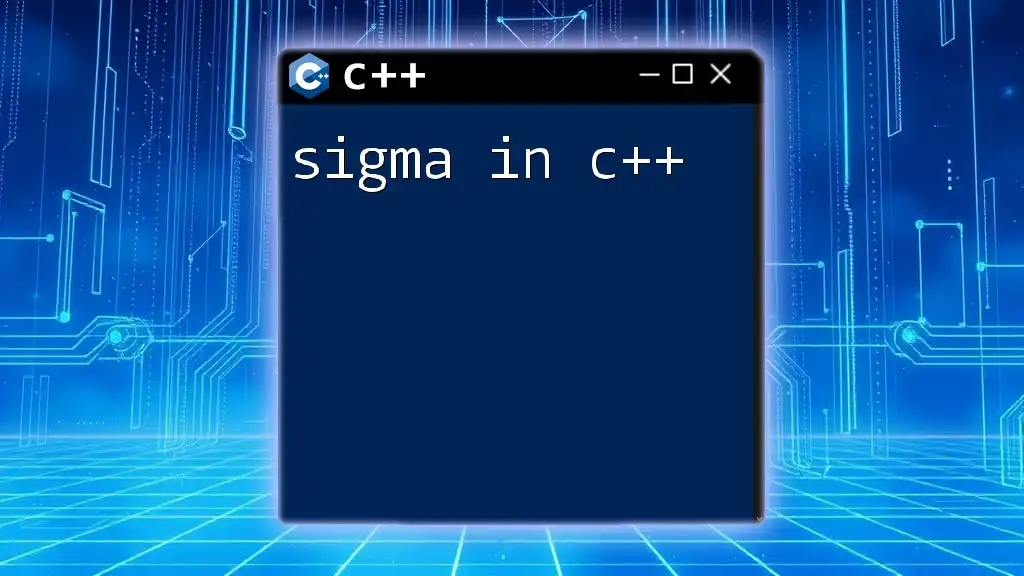
Conclusion
In summary, `#pragma once` serves as a valuable tool for managing header files in C++. It simplifies code maintenance, improves compile-time performance, and enjoys widespread compiler support. While there are considerations for compatibility with specific compilers and file systems, its benefits often outweigh the drawbacks. Developers are encouraged to adopt `#pragma once` in new projects, leveraging its efficiency to write cleaner, more manageable code.
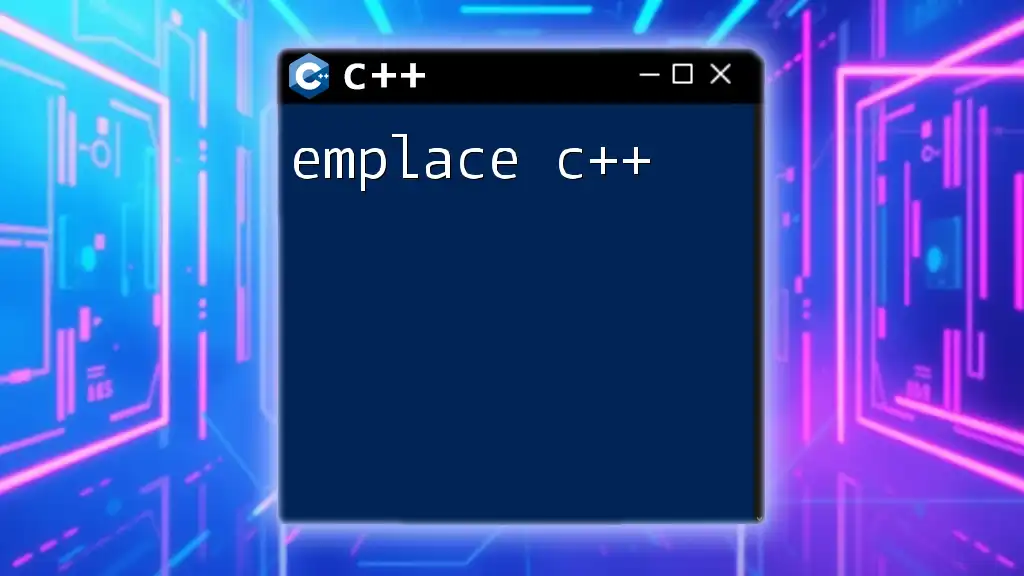
Frequently Asked Questions
Is `#pragma once` standard in C++?
While `#pragma once` is not part of the officially standardized C++ language, it enjoys strong support among modern compilers. Many developers find it effective for managing header files, making its use widespread in contemporary C++ coding practices.
Can I mix `#pragma once` with traditional include guards?
Yes, you can effectively combine both methods. Using `#pragma once` along with traditional include guards serves as a safety net, ensuring compatibility across different compilers and file systems while taking advantage of the benefits offered by both methods.
What should I do if my compiler does not support `#pragma once`?
If you find yourself using a compiler that lacks support for `#pragma once`, it is advisable to stick with traditional include guards. This ensures that your headers are protected from multiple definitions, maintaining cross-compiler compatibility.