The `push_back` function in C++ is used to add an element to the end of a vector, dynamically increasing its size.
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers;
numbers.push_back(10); // Adding 10 to the vector
numbers.push_back(20); // Adding 20 to the vector
for(int num : numbers) {
std::cout << num << " "; // Output: 10 20
}
return 0;
}
Understanding Vectors
Defining Vectors
In C++, vectors are part of the Standard Template Library (STL) and provide a way to manage collections of data effectively. A vector is a dynamic array, meaning it can change its size automatically as elements are added or removed. This flexibility sets vectors apart from traditional arrays, which have fixed sizes determined at compile time.
Basic Characteristics of Vectors
Vectors possess several characteristics that make them appealing for storing collections of data:
- Dynamic Sizing: Unlike arrays, vectors can automatically resize when elements are added or removed, allowing for efficient memory usage.
- Storable Data Types: Vectors can store elements of any data type, including primitive types (like `int`, `char`, etc.) and user-defined types (like classes and structures).
- Contiguous Memory Allocation: Vectors are allocated in contiguous memory, which helps in achieving performance benefits when accessing elements, making them more cache-friendly compared to linked lists.
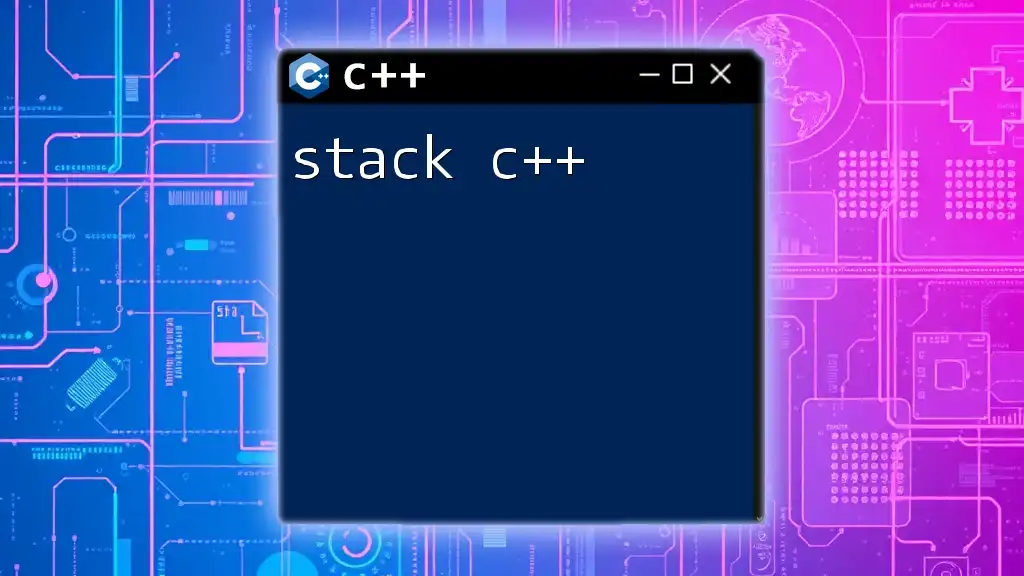
push_back: Definition and Syntax
Understanding push_back
The push_back function is a powerful member function of the vector class in C++. When you use `push_back`, you are appending an element to the end of the vector. This operation is intuitive, as it allows you to build collections easily.
Syntax
The basic syntax of the push_back function is as follows:
vectorName.push_back(value);
Here, `vectorName` refers to the vector you wish to modify, while `value` is the element you want to add. For example, if you have a vector named `myVector` and you want to add the integer `10`, you would write:
myVector.push_back(10);
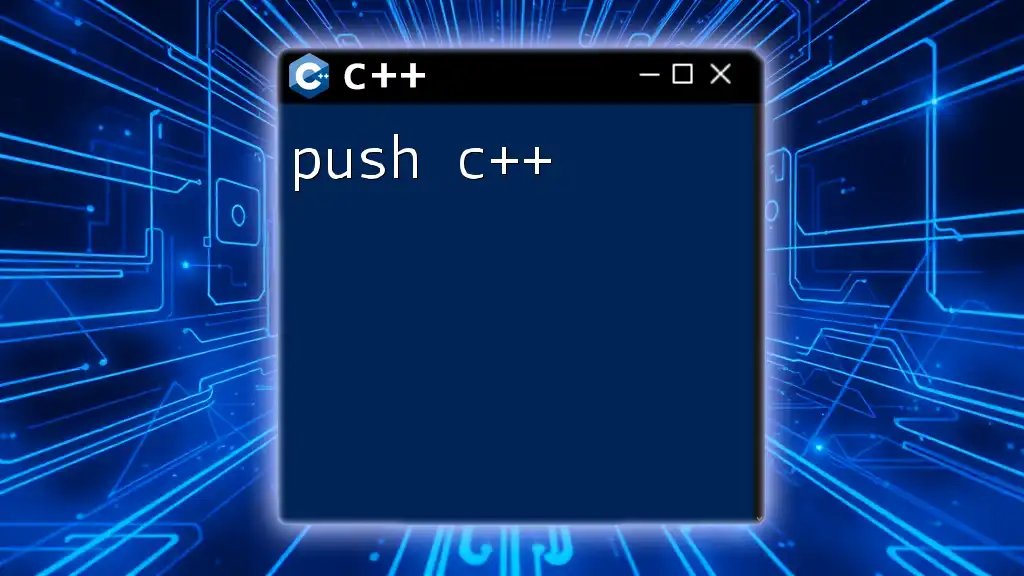
How push_back Works
Memory Management
When using push_back, C++ manages memory for you. Each time you add an item, the vector checks if its current capacity allows for more elements. If additional capacity is needed, a new larger block of memory is allocated, and the existing elements are copied over. This process ensures that the vector remains flexible.
Behavior on Capacity Reaching Limit
When the capacity of a vector is exceeded, `push_back` performs a reallocation operation. Typically, this involves increasing the capacity by about double its current size, which significantly reduces the frequency of allocations and makes the operation more efficient in terms of performance. However, this can lead to increased overhead due to the copying of existing elements to the new memory location.
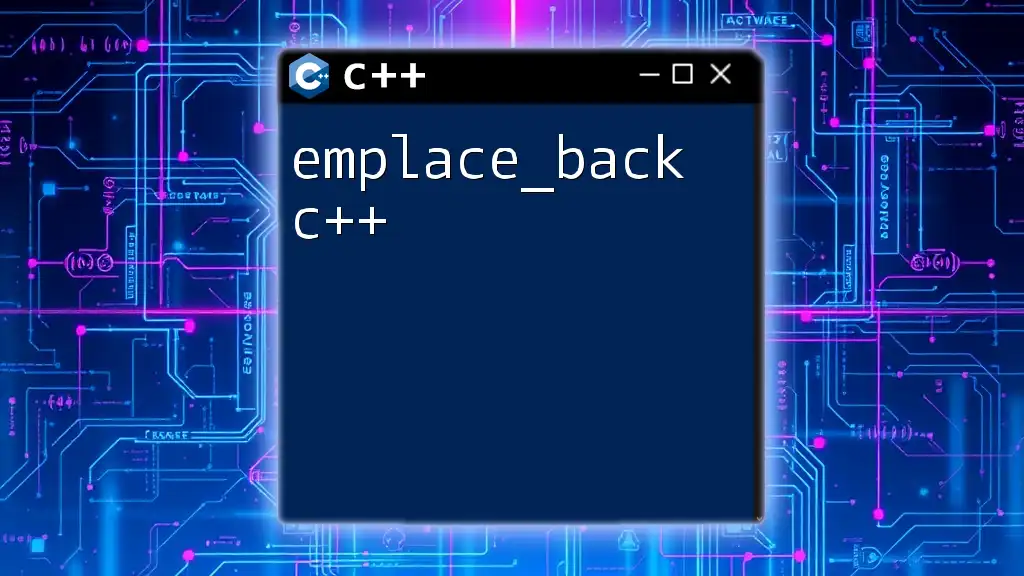
Practical Usage of push_back
Adding Elements to a Vector
Let’s see how push_back works in practice with a straightforward example. Below is a simple C++ program that demonstrates adding integers to a vector.
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector;
myVector.push_back(10);
myVector.push_back(20);
myVector.push_back(30);
for(int num : myVector) {
std::cout << num << " ";
}
return 0;
}
In this example, three integers are added to `myVector` using `push_back`, and the elements are then printed to the console. The output would be:
10 20 30
Pushing Different Data Types
Vectors in C++ can store multiple data types. Here’s an example showing how to add strings to a vector:
#include <iostream>
#include <vector>
#include <string>
int main() {
std::vector<std::string> stringVector;
stringVector.push_back("Hello");
stringVector.push_back("World");
for(const auto& str : stringVector) {
std::cout << str << " ";
}
return 0;
}
In this case, the output will be:
Hello World
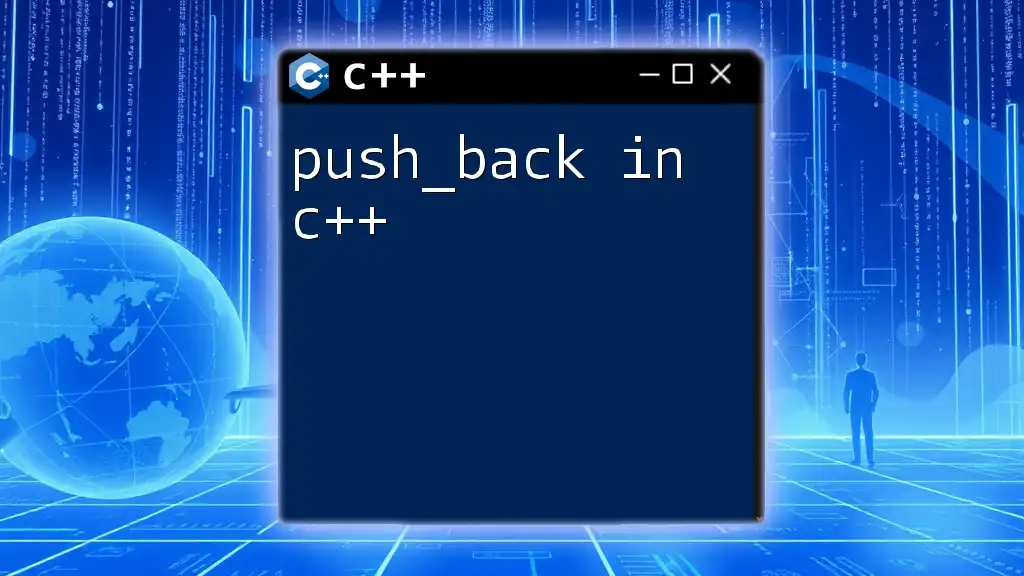
Common Errors and Troubleshooting
Using push_back with Empty Vectors
One common pitfall when using push_back emerges when attempting to push elements into an uninitialized or empty vector. While C++ handles this correctly, it’s essential to ensure that the vector is declared before performing operations on it.
Type Mismatches
Another frequent issue is type mismatches. The data type you are trying to push using push_back must match the vector’s defined type. For example, if you have a vector of type `int`, trying to push a `double` will result in a compilation error. Always verify that the element’s type aligns with the vector's type definition.
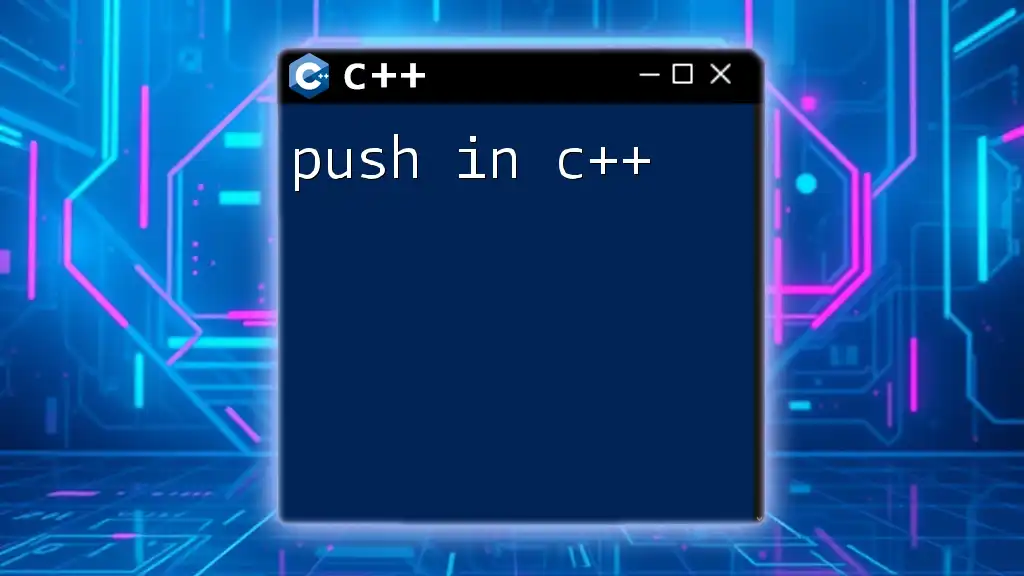
Performance Considerations
Efficiency of push_back
The push_back function generally has an average time complexity of O(1), meaning it can append an element in constant time. However, keep in mind that in the worst case, due to resizing, the complexity can be O(n). Understanding this can help in optimizing your application's performance.
Alternative Methods
C++ provides alternative methods for inserting elements, such as insert() and emplace_back(). Here’s a brief comparison:
- insert() allows you to add elements at specified positions, but it can be more costly regarding performance, especially within large vectors.
- emplace_back() constructs elements in place, which can enhance performance by avoiding unnecessary copies.
Choosing the right method depends on the specific needs of your application, such as requirements for insertion positions or element types.
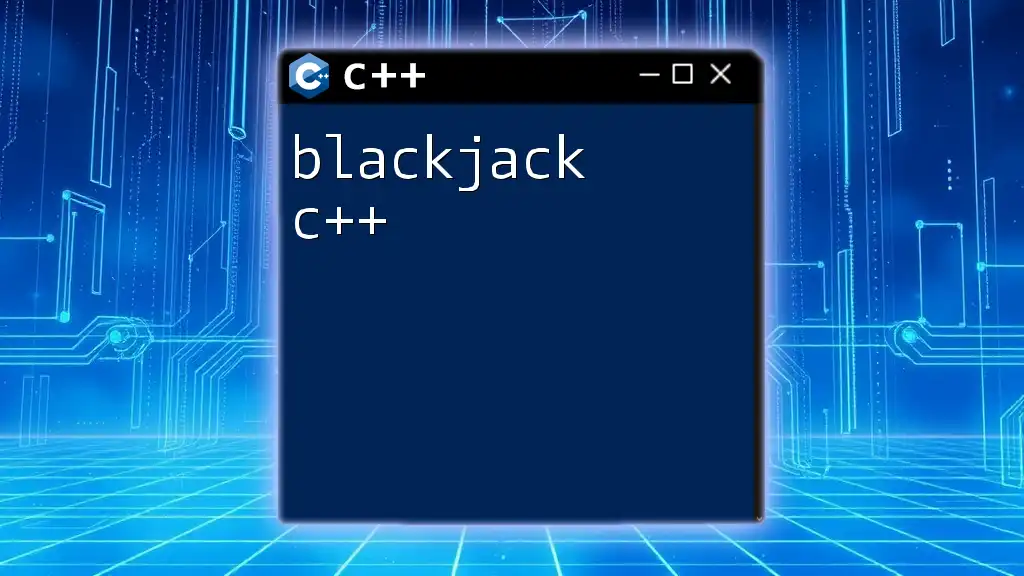
Conclusion
In summary, push_back is a valuable tool for managing dynamic arrays in C++. It allows developers to efficiently build and manipulate collections of data as needed. As you practice implementing `push_back`, you’ll gain more familiarity with C++ vectors and how to use them effectively.
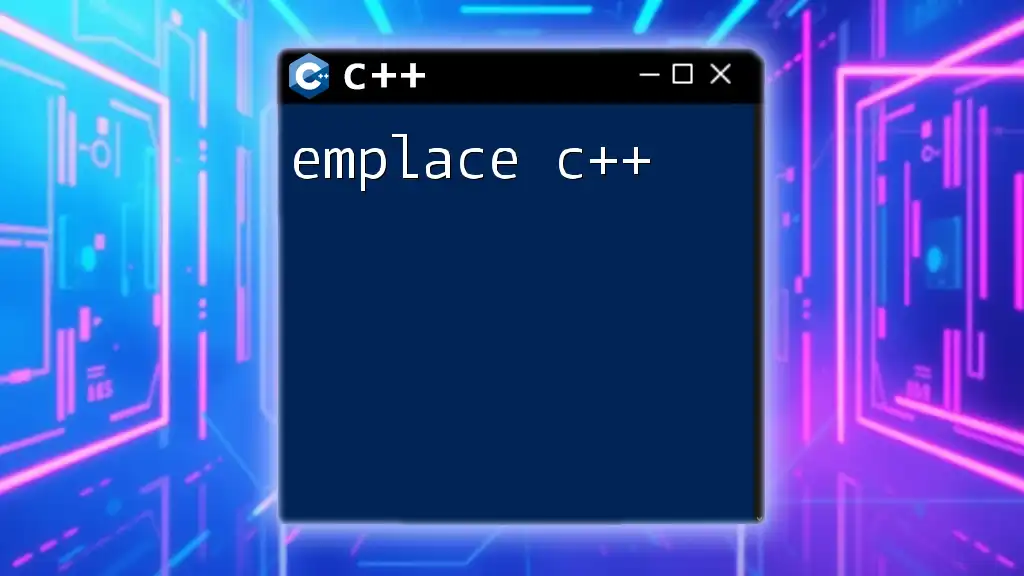
Additional Resources
For further learning about vectors in C++, consider exploring the official C++ documentation, online tutorials, and coding practice platforms to deepen your understanding of the concepts discussed in this guide.
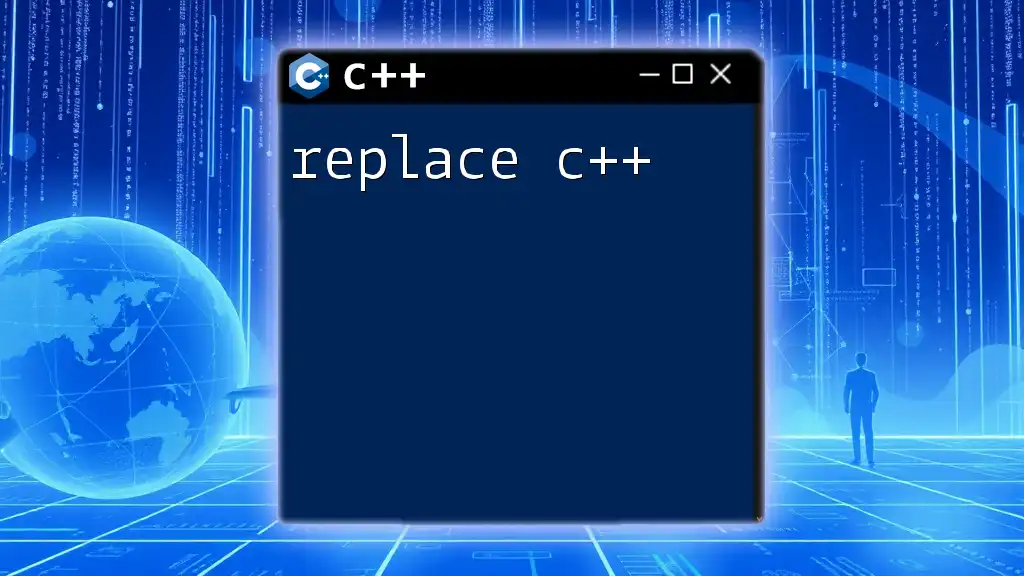
Call to Action
Feel free to share your experiences and questions regarding push_back and vector usage in C++. Engaging with peers can enhance your learning process. Also, check out our company's services designed to help you master C++ commands quickly and effectively.