In C++, you can create a simple blackjack game where players can hit or stand, and the program will determine the winner based on the hand values.
Here’s a basic code snippet to get you started:
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
srand(static_cast<unsigned int>(time(0))); // Seed for random number generation
int playerCard = rand() % 11 + 1; // Player's card value (1-11)
int dealerCard = rand() % 11 + 1; // Dealer's card value (1-11)
std::cout << "Player card: " << playerCard << "\n";
std::cout << "Dealer card: " << dealerCard << "\n";
if (playerCard > dealerCard) {
std::cout << "Player wins!\n";
} else if (playerCard < dealerCard) {
std::cout << "Dealer wins!\n";
} else {
std::cout << "It's a tie!\n";
}
return 0;
}
Getting Started with C++
What is C++?
C++ is a powerful, high-performance programming language that has become a cornerstone for developing scalable and efficient software applications. It is an extension of the C programming language, incorporating object-oriented features, which allow developers to structure their codes in a more organized manner. C++ offers a rich set of libraries and functions that enable developers to tackle complex problems with relative ease.
Setting Up Your Development Environment
To begin your journey with Blackjack in C++, you will need to set up your development environment effectively:
- Recommended IDEs: Using an Integrated Development Environment (IDE) can significantly speed up your developmental process. Popular options include Visual Studio, Code::Blocks, and CLion.
- Required Libraries: While C++ has a comprehensive standard library, for this project, you won't need any external libraries beyond what comes standard with C++.

Understanding the Blackjack Game Mechanics
Basic Rules of Blackjack
Before diving into programming the game itself, it’s essential to understand its mechanics:
- The objective of Blackjack is simple: beat the dealer’s hand without exceeding a total of 21.
- The game typically involves one dealer and multiple players. Each player competes against the dealer, not against each other.
Game Flow
The flow of the game is also straightforward:
- Dealing Cards: Each player and the dealer receive two cards at the beginning of the game. Players' cards are usually face up, while the dealer has one card face down (the "hole" card) and one face up.
- Player's Turn vs. Dealer's Turn: Players take turns to make decisions—thus, they can choose to 'hit' (take another card) or 'stand' (keep their current hand). After all players have acted, the dealer reveals their hole card and plays their hand according to set rules.
- Winning Conditions: Players win if their total is closer to 21 than the dealer's total without going over.
Card Values and Aces
In Blackjack, the card values are crucial to understanding how to compute scores:
- Cards 2-10 are worth their face value.
- Jacks, Queens, and Kings are each worth 10.
- Aces can be valued at either 1 or 11, depending on which value is more beneficial to the player.
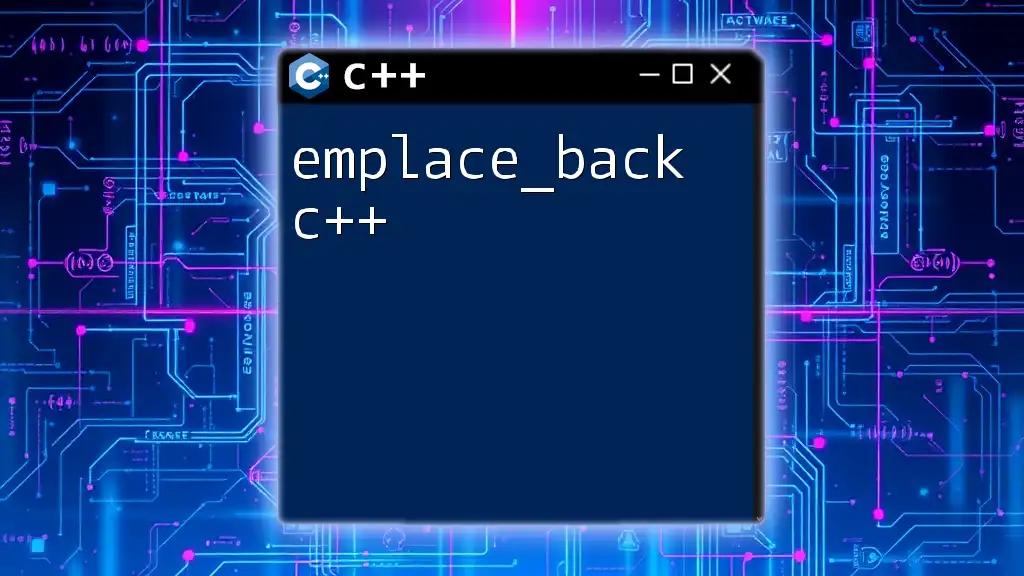
Designing the Game Structure
Class Structure for the Game
An efficient object-oriented design will help structure your Blackjack game program effectively. You will create several classes: `Card`, `Deck`, and `Player`.
Example Code Snippet
class Card {
public:
std::string suit;
std::string value;
int getValue() {
if (value == "Ace") {
return 11; // or 1 based on player choice
} else if (value == "King" || value == "Queen" || value == "Jack") {
return 10;
} else {
return std::stoi(value);
}
}
};
Creating the Game Logic Class
The heart of your Blackjack program will be the `BlackjackGame` class, where the key functions will reside.
Example Code Snippet
class BlackjackGame {
public:
void startGame();
void playerTurn();
void dealerTurn();
};
This class will handle the overall game flow, initiating new rounds and managing player interactions and dealer operations.
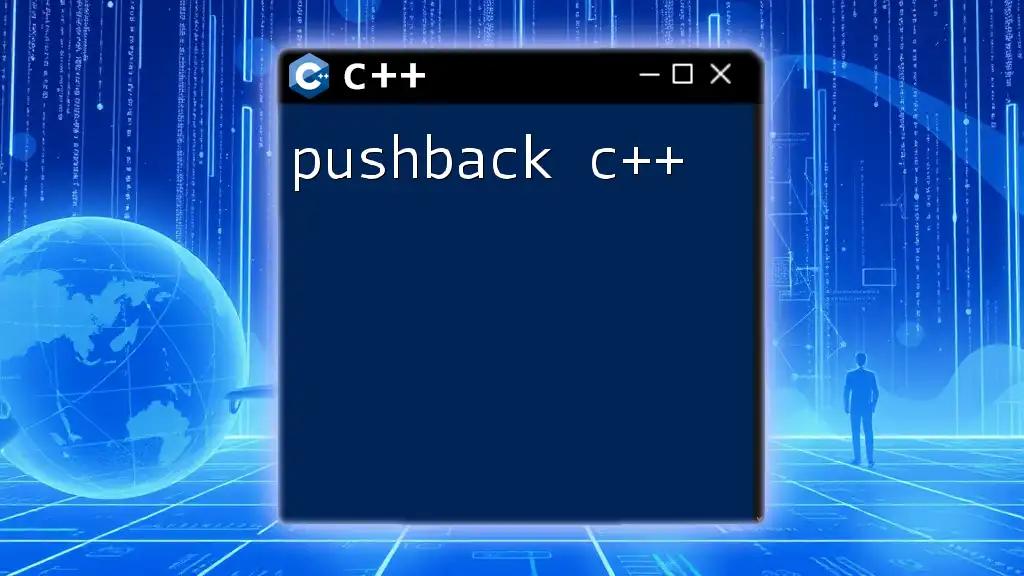
Implementing the Card Deck
Creating a Deck of Cards
A Deck class will facilitate card management by keeping track of card creation, shuffling, and dealing.
Example Code Snippet
class Deck {
public:
std::vector<Card> cards;
void shuffle() {
std::random_shuffle(cards.begin(), cards.end());
}
Card dealCard() {
Card dealtCard = cards.back();
cards.pop_back();
return dealtCard;
}
};
Randomization in C++
For effective shuffling, you can use the `rand()` function in conjuction with `<cstdlib>` and `<algorithm>` headers to randomize your deck.

Player and Dealer Interaction
Player Actions
When players take their turns, they typically have a few options available:
- Hit: Take another card.
- Stand: Keep the current hand.
- Double Down: Double the bet and take one additional card (optional).
Implementing player choices within the game loop will require user inputs that dictate game progression.
Dealer's Turn Logic
The dealer's actions are usually restricted to hitting until reaching a total of 17 or higher. This rigid structure makes it easier to implement, as the dealer's logic can largely be predetermined.

User Interface and Game Display
Creating a Basic Text-based Interface
For this version of Blackjack, a text-based interface will suffice. Display information regarding player hands, dealer actions, and scores clearly in the console.
User Input Handling
Utilizing `cin` for obtaining player decisions will provide a way to interact with your game.
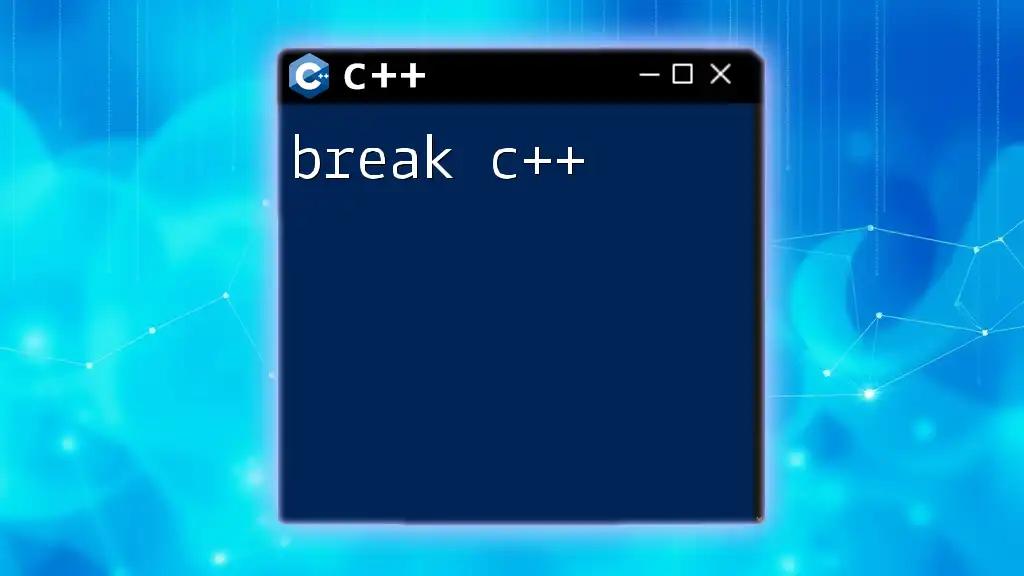
Running the Game
Game Loop Structure
A game loop is crucial for maintaining the game's flow and allowing players to decide whether to continue playing. Below is a basic structure of how your game loop will look:
Example Game Loop Code Snippet
while (true) {
blackjackGame.startGame();
// Additional game logic concerning player actions and dealer turns
std::cout << "Play Again? (Y/N): ";
char choice;
std::cin >> choice;
if (choice != 'Y' && choice != 'y') {
break;
}
}

Enhancements and Features
Adding Betting Mechanics
To make your Blackjack game even more engaging, consider adding betting mechanics. Implementing a system to keep track of player balances and allowing them to place wagers can elevate the game's complexity and enjoyment.
Advanced Features to Consider
Once you're comfortable with the basic implementation, expand the game's capabilities:
- Implement multiple players where each has separate hands.
- Introduce splitting hands when a player has two cards of the same value.
- Create additional game modes such as tournaments for enhanced interaction.
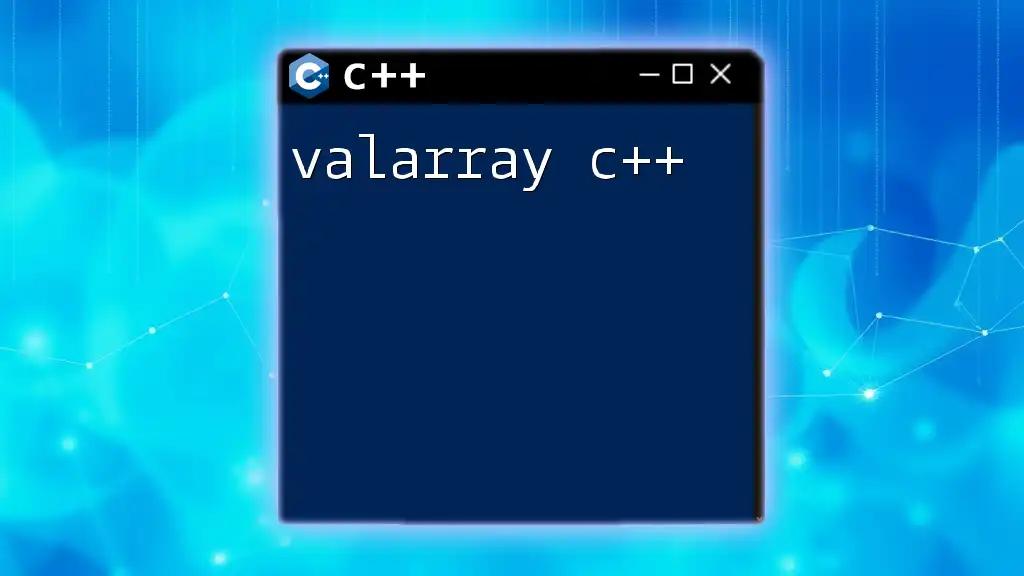
Conclusion
Creating a Blackjack game in C++ provides an excellent opportunity to deepen your programming skills while developing a fun and interactive project. Through this experience, you will have learned about critical C++ concepts such as classes, game flow, and user interactions. For further development, consider diving into more complex features, enriching your knowledge, and enhancing your programming acumen. Make use of additional resources available in books and online communities to continue your journey in C++.