Back-end C++ refers to the use of C++ programming language for server-side development, enabling efficient handling of data and application logic.
Here's a simple example demonstrating a basic C++ program that sets up a server socket:
#include <iostream>
#include <sys/types.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <unistd.h>
int main() {
int server_fd = socket(AF_INET, SOCK_STREAM, 0);
struct sockaddr_in address;
int opt = 1;
int addrlen = sizeof(address);
setsockopt(server_fd, SOL_SOCKET, SO_REUSEADDR, &opt, sizeof(opt));
address.sin_family = AF_INET;
address.sin_addr.s_addr = INADDR_ANY;
address.sin_port = htons(8080);
bind(server_fd, (struct sockaddr *)&address, sizeof(address));
listen(server_fd, 3);
std::cout << "Server is listening on port 8080" << std::endl;
return 0;
}
What is Back End Development?
Back end development refers to the server side of applications—essentially what users don’t see. It is responsible for managing how data is sent and received, as well as executing business logic and database interactions. The back end works in conjunction with the front end, which is the visible interface of applications, to create a cohesive user experience.
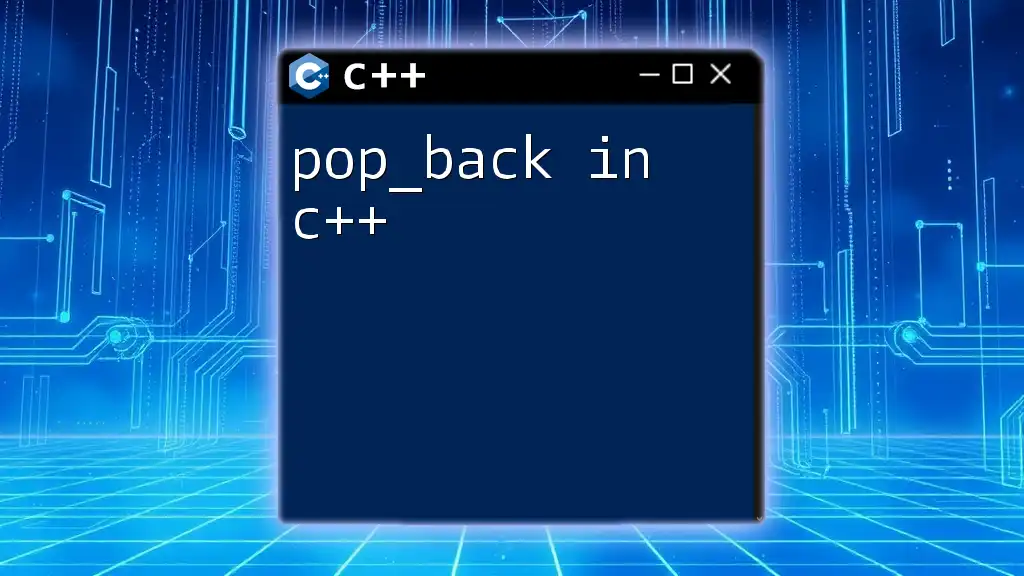
Why Choose C++ for Back End Development?
Choosing C++ for back end development has several compelling reasons. First and foremost, C++ provides exceptional performance and efficiency, making it suitable for applications requiring heavy computation or real-time processing. It also offers fine control over memory management, allowing developers to optimize how memory is allocated and deallocated, leading to improved performance over languages with automatic garbage collection.
Additionally, C++ has a vast ecosystem, powerful libraries, and frameworks that address various development needs, making it a viable option for many back end scenarios.
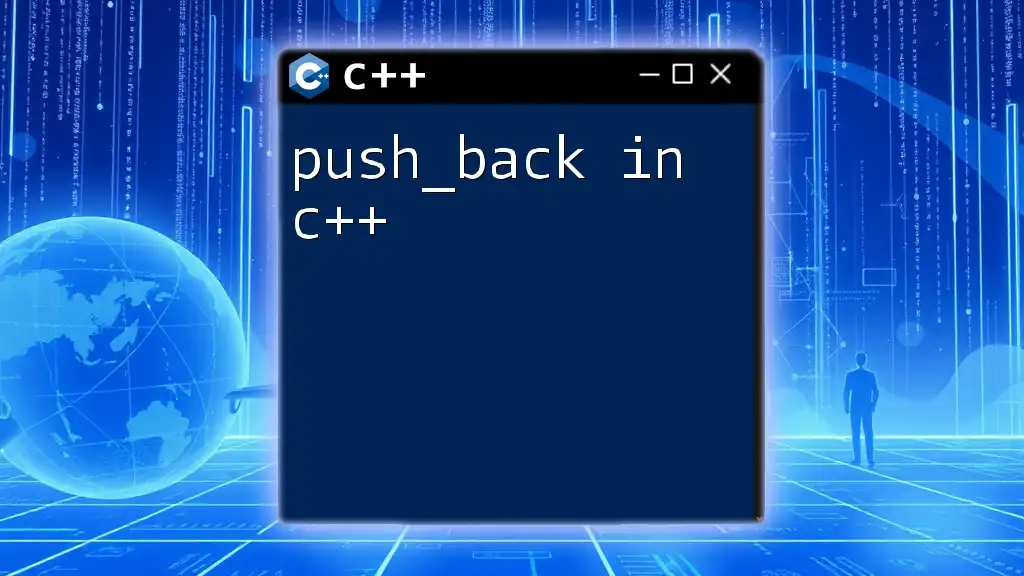
Understanding C++ as a Back End Language
Key Features of C++ that Benefit Back End Development
C++ boasts several key features that enhance its utility in back end development:
-
Object-oriented programming (OOP): The OOP paradigm in C++ helps you design clean, modular, and reusable code, aligning well with modern software engineering principles. It supports encapsulation, inheritance, and polymorphism, facilitating the creation of complex systems efficiently.
-
Low-level memory control: C++ gives developers direct control over system resources. This feature is crucial for performance-critical applications where memory usage must be optimized, such as gaming engines, financial platforms, and real-time simulations.
-
Standard Template Library (STL): C++’s STL provides a collection of well-implemented algorithms and data structures. It enhances productivity by minimizing the effort needed to implement common functionalities, such as sorting, navigating, and managing data.
Comparison with Other Back End Languages
When exploring back end C++ development, it’s valuable to compare it with other languages:
-
C++ vs. Python: While Python is user-friendly and excels in rapid application development, C++ takes the lead in performance and efficiency, making it ideal for resource-intensive applications.
-
C++ vs. Java: Java is platform-independent thanks to the JVM (Java Virtual Machine) but may have slower execution. C++, being compiled directly to machine code, often outperforms Java in execution speed and memory usage.
-
C++ vs. Node.js: Node.js is excellent for I/O-driven applications, while C++ shines in computation and systems-level applications. The choice depends on the specific requirements of the project.
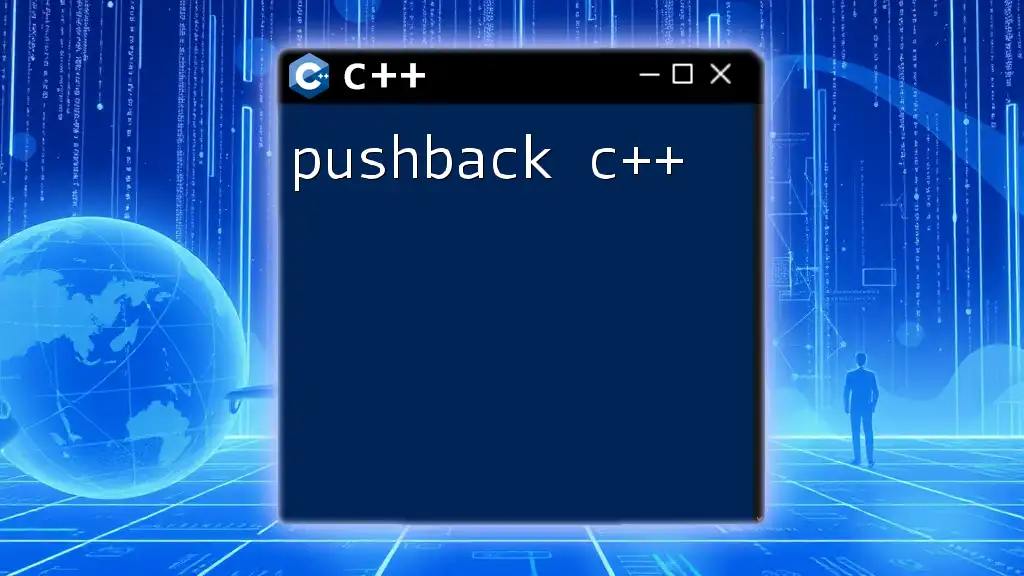
Setting Up Your C++ Back End Development Environment
Tools and IDEs for C++ Development
To develop a back end application in C++, selecting an appropriate Integrated Development Environment (IDE) and tools is critical:
-
Visual Studio: A powerful IDE for Windows that offers debugging tools, code completion, and integrates well with various libraries.
-
CLion: A cross-platform IDE that supports CMake and provides many refactoring features, making it a popular choice among C++ developers.
-
Code::Blocks: A lightweight, open-source IDE that is versatile and easy to use, suitable for developers who lean towards simplicity.
To make development seamless, leverage libraries and frameworks, such as:
-
Boost: A set of portable C++ source libraries that extend functionality and simplify development tasks.
-
POCO: A modern C++ framework that includes classes for networking and HTTP, enabling swift back end development.
-
CPPCMS: A web development framework specifically designed for high-performance C++ applications, making web services easier to develop.
Installing and Configuring Your Environment
Setting up your environment can vary based on your operating system. For Windows, download the Visual Studio installer and select the C++ workload. For macOS, consider using XCode and installing Homebrew to manage packages. Linux users can use the terminal for package installations.
Managing dependencies can be simplified using CMake, a tool that helps manage the build process. You can create a `CMakeLists.txt` file to specify project structure, compiling settings, and linked libraries, facilitating a smoother workflow.
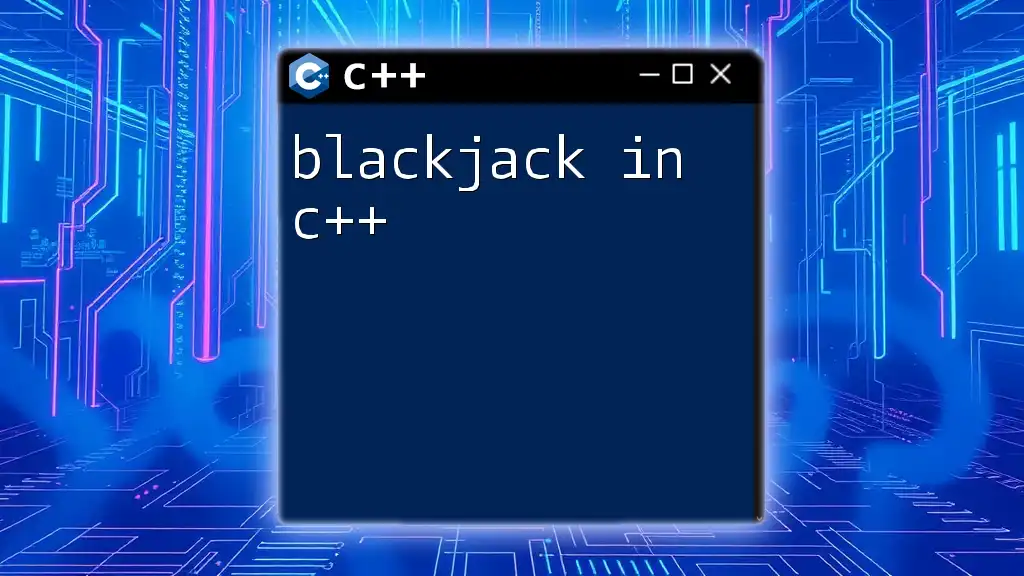
Building a Simple C++ Back End Application
Designing the Architecture
Before coding, decide whether to implement a microservices or monolithic architecture. A microservices-based approach allows for independent deployment and scalability but involves more complex management. On the other hand, a monolithic architecture may simplify development but can become challenging as applications grow.
Developing Your First C++ Back End API
Setting Up a Basic HTTP Server with C++
To create an HTTP server, you can leverage the powerful POCO library. Below is a simple example of setting up a basic server that responds with "Hello, World!":
#include <Poco/Net/HTTPServer.h>
#include <Poco/Net/HTTPServerParams.h>
#include <Poco/Net/HTTPRequestHandler.h>
#include <Poco/Net/HTTPServerRequest.h>
#include <Poco/Net/HTTPServerResponse.h>
class HelloWorldRequestHandler : public Poco::Net::HTTPRequestHandler {
void handleRequest(Poco::Net::HTTPServerRequest& request, Poco::Net::HTTPServerResponse& response) override {
response.setStatus(Poco::Net::HTTPResponse::HTTP_OK);
response.setContentType("text/html");
response.send() << "Hello, World!";
}
};
int main() {
Poco::Net::HTTPServer server(new Poco::Net::HTTPRequestHandlerFactory, Poco::Net::ServerSocket(8080), new Poco::Net::HTTPServerParams);
server.start();
std::cout << "Server running on port 8080..." << std::endl;
getchar(); // Wait for enter to exit
server.stop();
}
Handling Requests and Responses
In creating a RESTful API, familiarize yourself with various HTTP methods like GET, POST, PUT, and DELETE. When handling requests in the handler class, you can differentiate requests based on the method and respond accordingly. For example, a GET request could retrieve data, while a POST request would send data to the server.
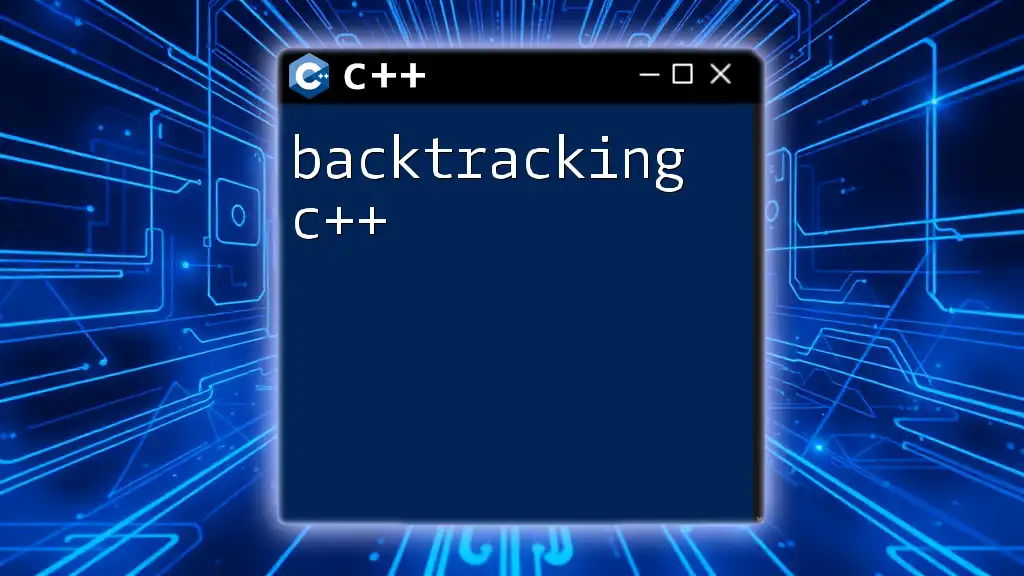
Database Integration in C++
Choosing the Right Database
Selecting a suitable database can significantly affect your application's functionality. SQL databases like SQLite or MySQL work efficiently for structured data, while NoSQL databases such as MongoDB are great for handling unstructured data.
Connecting to a Database with C++
To illustrate connecting to a MySQL database using the MySQL Connector/C++, consider the following example:
#include <mysql_driver.h>
#include <mysql_connection.h>
#include <cppconn/prepared_statement.h>
void connectToDatabase() {
sql::mysql::MySQL_Driver* driver = sql::mysql::get_mysql_driver_instance();
std::unique_ptr<sql::Connection> con(driver->connect("tcp://127.0.0.1:3306", "user", "password"));
con->setSchema("mydatabase");
std::unique_ptr<sql::PreparedStatement> pstmt(con->prepareStatement("SELECT * FROM mytable"));
std::unique_ptr<sql::ResultSet> res(pstmt->executeQuery());
while (res->next()) {
std::cout << "Column 1: " << res->getString(1) << std::endl;
}
}
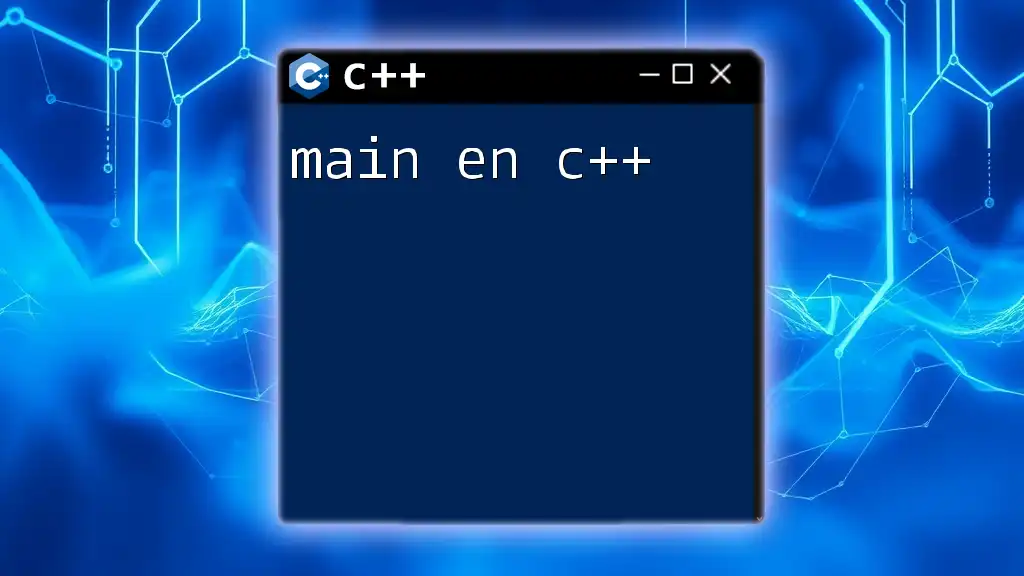
Best Practices for C++ Back End Development
Code Quality and Organization
Writing clean, modular, and maintainable code is crucial in any programming language, particularly in C++. Group related functions and classes together and follow naming conventions for clarity. Utilize commenting to explain complex sections of code to both present and future developers.
Error Handling and Debugging Techniques
Error handling is essential to ensure your application runs smoothly. In C++, use exceptions to handle errors gracefully. Familiarize yourself with debugging tools like gdb or integrated debuggers in IDEs to step through your code and identify issues.
Performance Optimization
Performance I is a significant advantage of C++. By profiling your application, you can identify bottlenecks and optimize algorithms for better execution speed. Employ techniques like avoiding memory leaks through careful management of allocated resources.
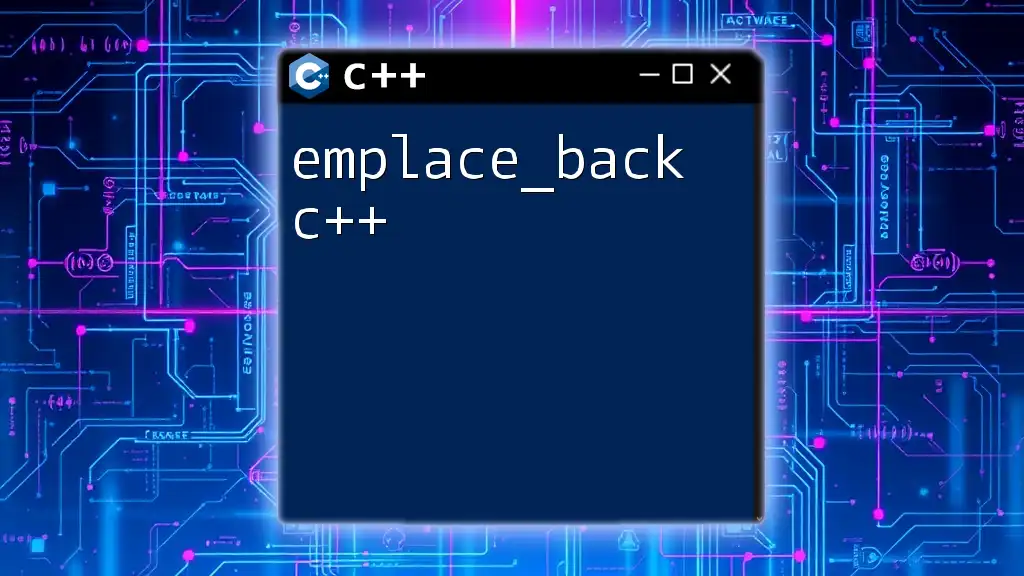
Use Cases and Real-World Applications of C++ in Back End Development
Example Industries Leveraging C++
C++ is utilized in various industries for back end development. For instance, in gaming, C++ serves as the backbone for real-time game servers, handling complex game logic efficiently. In finance, developers rely on C++ for creating high-frequency trading systems where milliseconds can make a difference. The systems programming field also utilizes C++ for developing operating systems and embedded systems due to its performance and efficient resource usage.
Case Study: C++ in Action
Consider a leading real-time online gaming platform that leveraged C++ to build its server infrastructure. The critical aspect of their application was minimizing latency while processing numerous player actions simultaneously. The final implementation resulted in a system that could handle thousands of requests per second, showcasing C++’s speed and reliability.
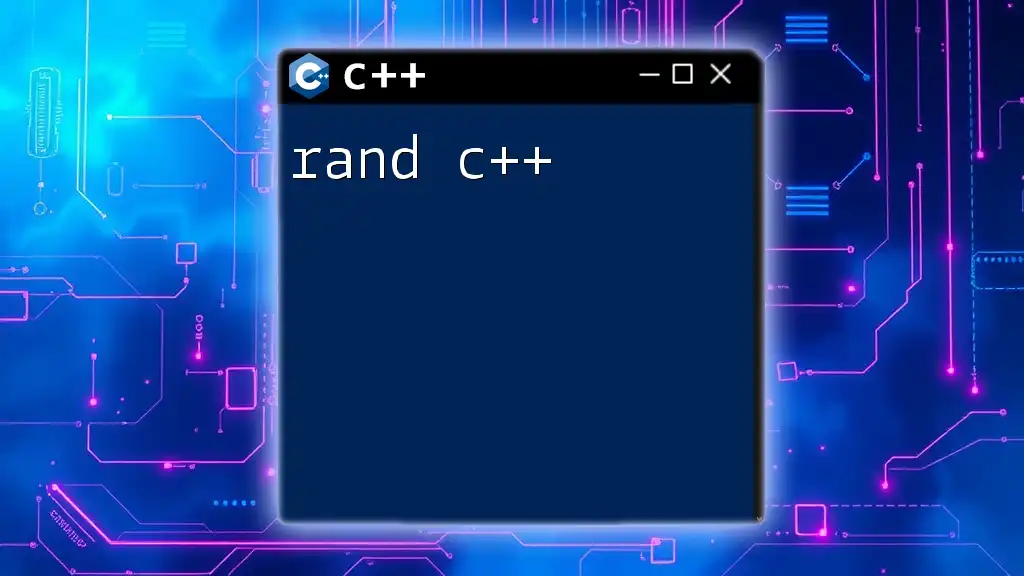
Conclusion
Future of C++ in Back End Development
The future of C++ in back end development appears promising. With emerging trends in high-performance computing, IoT devices, and game development, C++ remains a robust choice. Continuous improvements in libraries and frameworks will also enhance its capabilities, ensuring it remains relevant.
Call to Action
If you're considering diving into back end development, C++ is an excellent language to master. Familiarize yourself with its features and advantages, and explore various resources to elevate your skills. Combine theory with practice, building simple applications to solidify your learning.
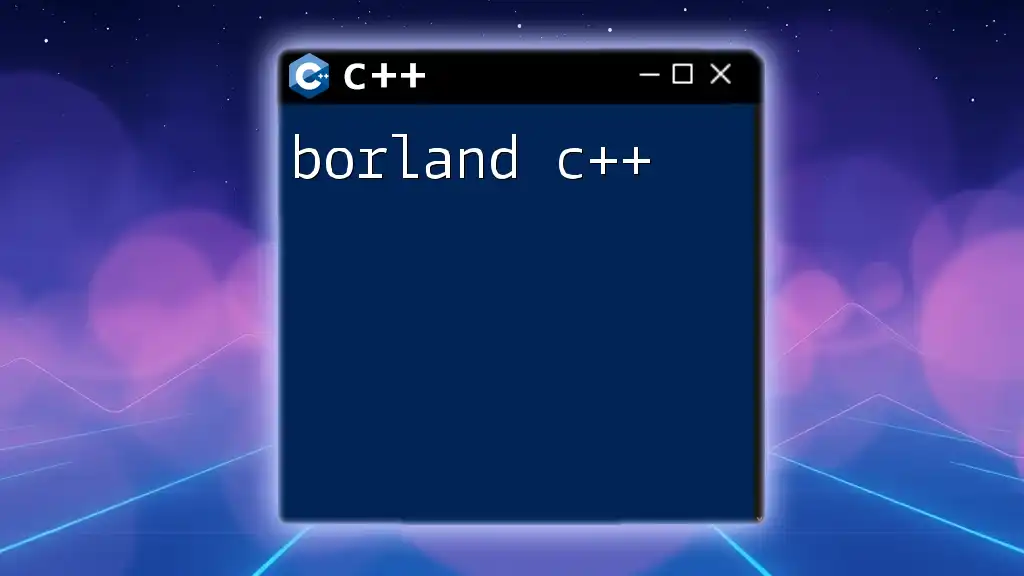
Additional Resources
For those eager to learn more about C++, various resources can aid in your journey. Consider exploring renowned books such as "The C++ Programming Language" by Bjarne Stroustrup or various online courses that provide structured learning paths. Engage with online communities, forums, and contribute to open-source projects to gain practical experience and network with other developers.