The `upper_bound` function in C++ is used to find the first position in a sorted range where a given value could be inserted to maintain the order, effectively returning an iterator to the upper bound of that value.
Here's a code snippet demonstrating its usage:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> nums = {1, 2, 4, 4, 5};
int value = 4;
auto it = std::upper_bound(nums.begin(), nums.end(), value);
if (it != nums.end()) {
std::cout << "Upper bound of " << value << " is " << *it << std::endl;
} else {
std::cout << value << " is larger than all elements." << std::endl;
}
return 0;
}
What is `std::upper_bound`?
`std::upper_bound` is a powerful function in C++ that finds the first position in a sorted range where a specified value could be inserted while maintaining the order of the elements. It is part of the Standard Template Library (STL) and plays a crucial role in algorithms that involve searching and sorting.
Using `std::upper_bound` can be incredibly beneficial when dealing with sorted data. It allows developers to perform efficient searches as it leverages the binary search algorithm under the hood, ensuring a quick lookup time.
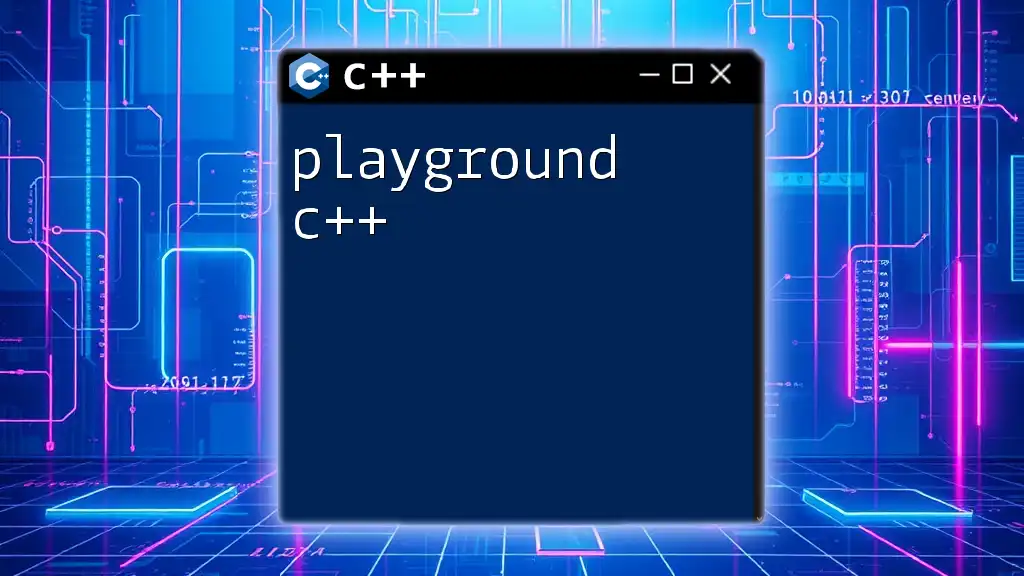
When to use `std::upper_bound`?
There are several scenarios in which `std::upper_bound` proves invaluable:
- Finding insertion points: If you’re managing a sorted dataset and need to know where to place a new element, `std::upper_bound` adeptly provides that information without disrupting the order.
- Efficient searches: In an environment where the performance of searches is crucial, `std::upper_bound` enables quick lookups in logarithmic time, making your code more efficient.
Comparatively, when observing other searching methods like linear searching, which operates in O(n) time complexity, `std::upper_bound` shines with its O(log n) complexity.
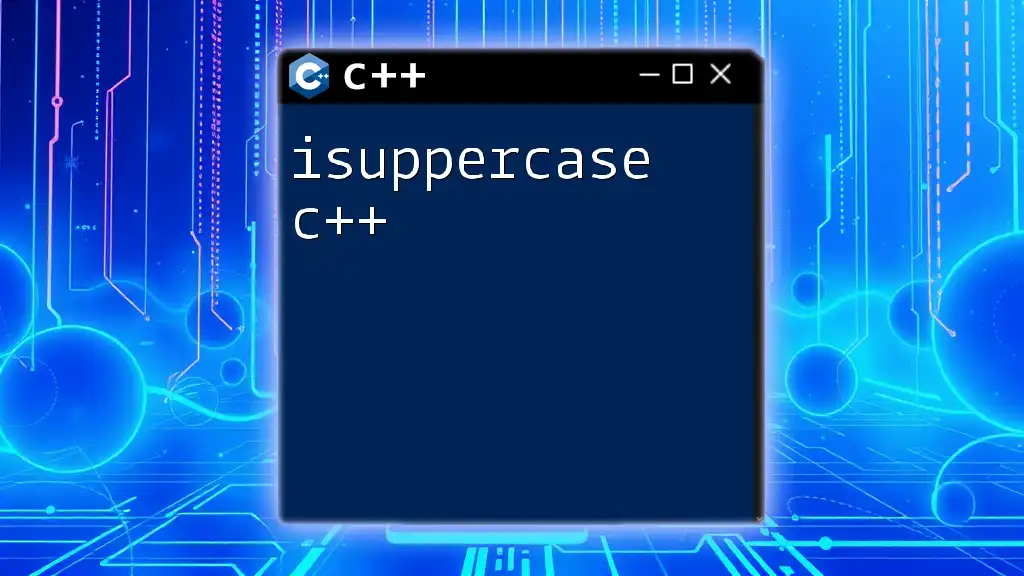
Understanding the Basics of Binary Search
The Concept of Binary Search
Binary search is a classic algorithm used to locate an item in a sorted collection by repeatedly dividing the search interval in half. If the value of the search key is less than the item in the middle of the interval, the search continues in the lower half; otherwise, it proceeds in the upper half. This method significantly reduces the number of comparisons made.
How Binary Search Relates to `upper_bound`
While `std::upper_bound` is an abstraction built on top of binary search, it specifically serves to locate the upper boundary for the specified value. When searching for an element, `upper_bound` moves through the sorted collection by checking which side contains a value that is strictly greater than the target.
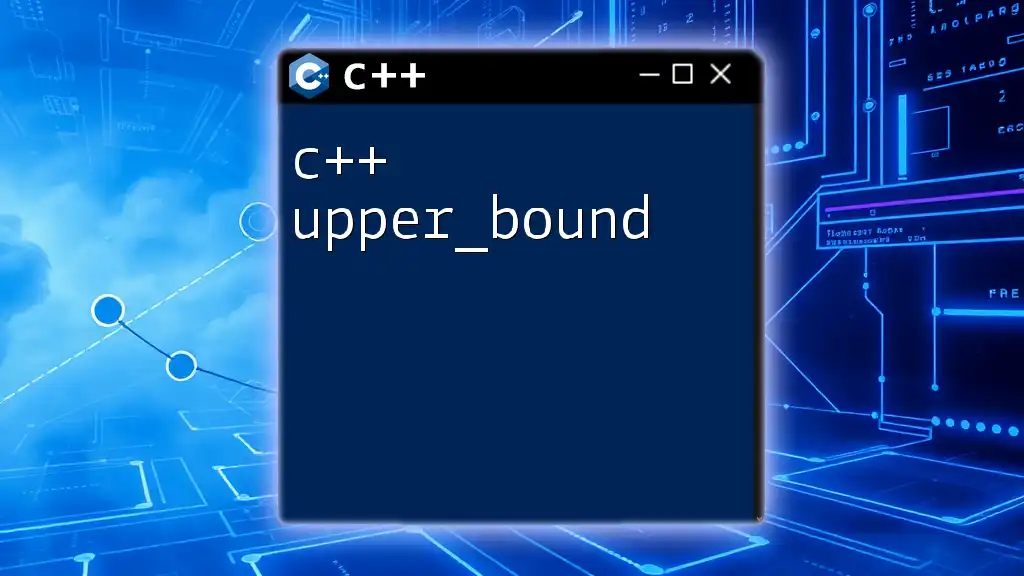
Signature of `std::upper_bound`
Function Signature
The function is defined as follows:
template<class ForwardIt, class T>
ForwardIt upper_bound(ForwardIt first, ForwardIt last, const T& value);
In this signature:
- `ForwardIt`: Indicates that the iterators must meet the requirements of a forward iterator.
- `first` and `last`: Represent the range (beginning and end).
- `value`: The value for which you seek the upper bound.
Template Parameters
`std::upper_bound` is a template function, which means it can accept various data types as long as those types support the operations required (like comparison). The versatility allows it to work with built-in types (like `int`) as well as custom structures with overloaded operators.
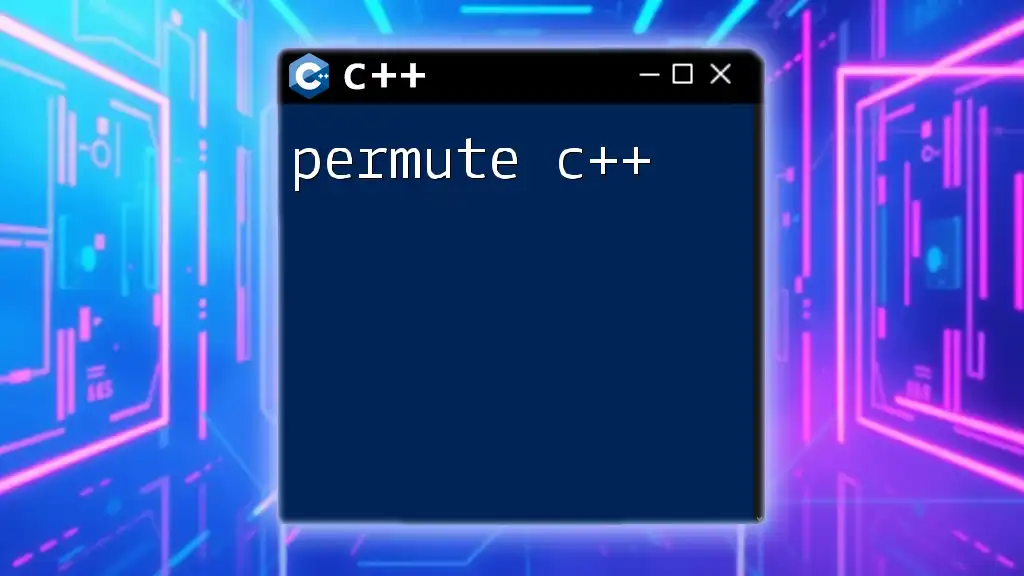
Usage of `std::upper_bound`
Basic Example with Sorted Arrays
To demonstrate the basic functionality of `std::upper_bound`, consider the following example:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
auto it = std::upper_bound(vec.begin(), vec.end(), 3);
std::cout << "Upper bound of 3 is: " << *it << std::endl;
return 0;
}
In this code:
- A vector of integers is created and initialized with sorted values.
- The `std::upper_bound` function is called, searching for the value `3`.
- The resulting iterator points to the first element greater than `3`, which is `4`, demonstrating the efficacy of the function.
Working with Custom Data Types
`std::upper_bound` can be adapted to handle complex structures as well. Here is an example using a custom `Person` structure:
struct Person {
int age;
// compare by age
bool operator<(const Person& other) const {
return age < other.age;
}
};
int main() {
std::vector<Person> people = {{20}, {25}, {30}};
Person target = {25};
auto it = std::upper_bound(people.begin(), people.end(), target);
std::cout << "First person older than 25 is: " << it->age << std::endl;
return 0;
}
In this case:
- We defined a `Person` struct and overloaded the comparison operator to compare ages.
- The `std::upper_bound` function is then applied to a vector of `Person` objects to find the first individual older than the given target.
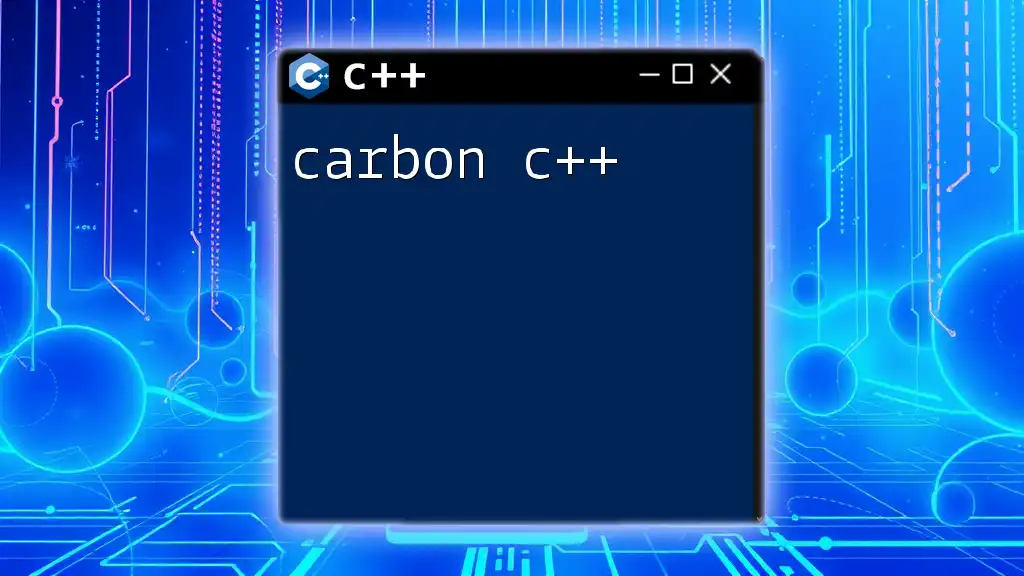
Implementation Details
How `std::upper_bound` Works Internally
Internally, `std::upper_bound` employs a binary search strategy. It keeps dividing the range in half until it finds the position where the specified value would fit beyond all occurrences of equivalent values. Due to this halving strategy, the complexity remains efficient.
Custom Comparison Functions
You can also use custom comparison functions to tweak the behavior of `std::upper_bound`. For instance, consider the following:
auto it = std::upper_bound(vec.begin(), vec.end(), 3, std::greater<int>());
This example inverts the comparison, allowing the search to react differently to the ordering of elements. It will find the first element that is less than or equal to `3`, demonstrating the flexibility of `upper_bound`.
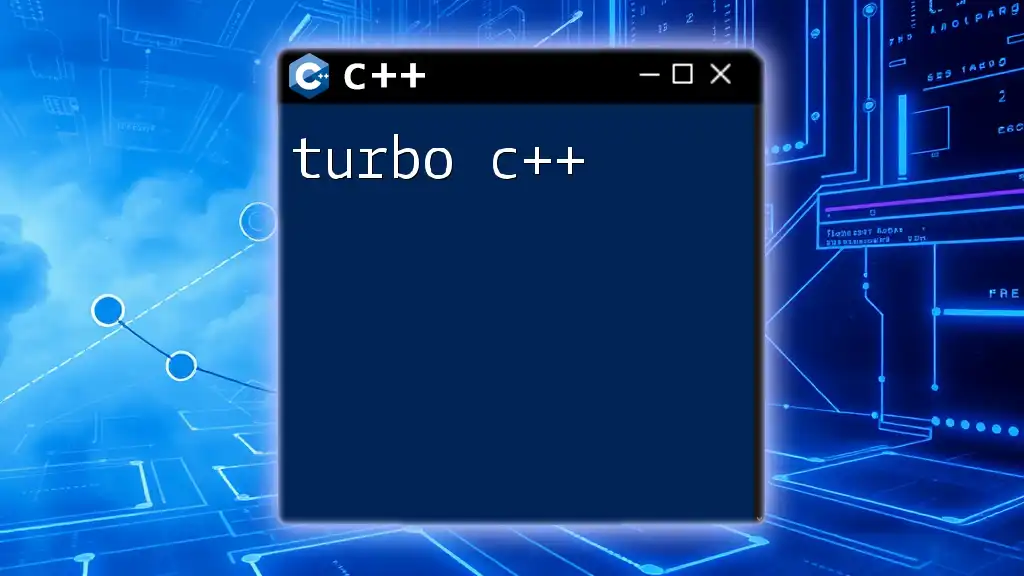
Performance Considerations
Time Complexity Analysis
The time complexity of `std::upper_bound` is O(log n) due to its underlying binary search mechanism. This efficiency makes it a preferred option for searching where performance is a primary concern.
Space Complexity
`std::upper_bound` operates in constant space, which means it does not require additional storage proportional to the input size, making it ideal for environments with limited memory.
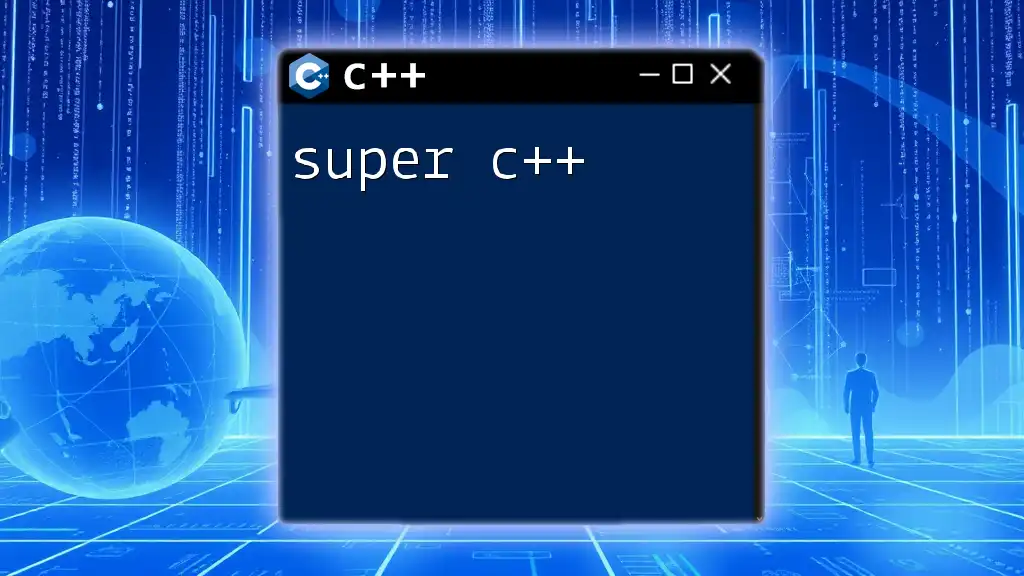
Common Pitfalls
Mistakes to Avoid When Using `std::upper_bound`
One frequent mistake when employing `std::upper_bound` is passing unsorted data, leading to unpredictable results. To ensure reliable outcomes, always pre-sort the collection before applying the function.
When It Might Fail
`std::upper_bound` may fail to produce accurate results if the criteria for comparison are not well-defined or if the elements are not strictly comparable due to data type inconsistencies.
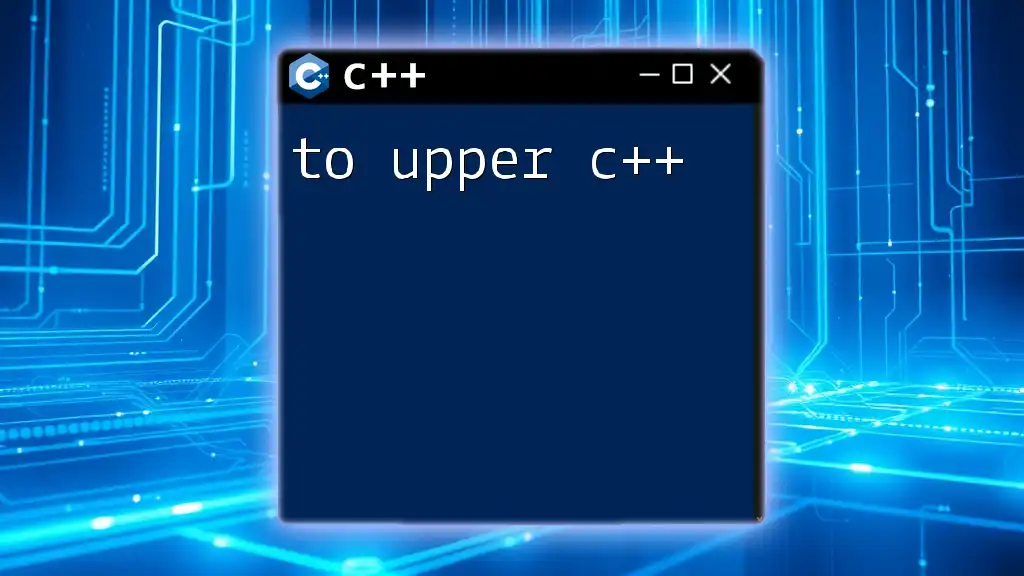
Recap of Key Points
In summary, `std::upper_bound` is a valuable tool in C++ for determining the position in a sorted range for inserting values while maintaining order. Its use cases span simple array searches to complex data structures. By leveraging binary search, it offers quick and efficient lookups, proving its worth in algorithm design.
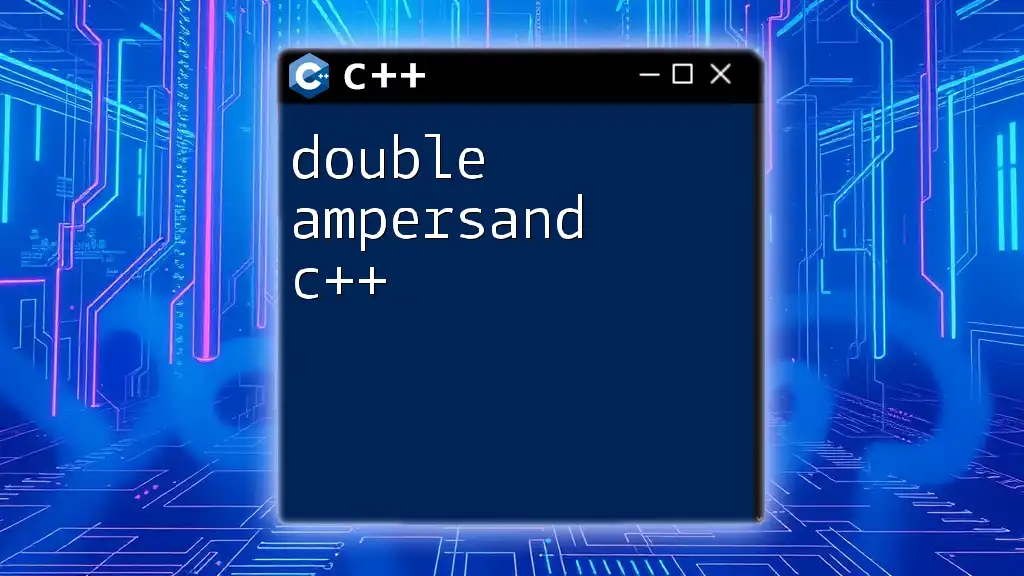
Encouragement to Practice
Embrace the challenge of using `std::upper_bound` in various contexts and with different data types. Understanding its core functionalities will empower you to write optimized and effective C++ code.
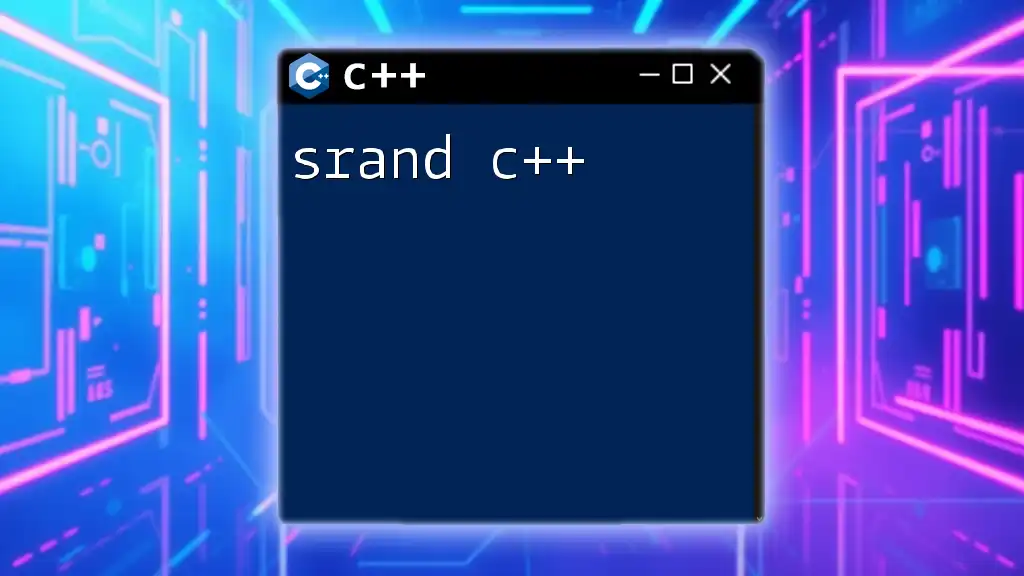
Additional Resources
For further reading, consider checking out C++ STL documentation and various online programming challenges that focus on searching algorithms. Practice problems often provide practical implementations that can solidify your understanding of `std::upper_bound` and its usage in real-world applications.