The `std::toupper` function in C++ is used to convert a given character to its uppercase equivalent. Here’s how you can use it:
#include <iostream>
#include <cctype> // for std::toupper
int main() {
char lowerChar = 'a';
char upperChar = std::toupper(lowerChar);
std::cout << "Uppercase: " << upperChar << std::endl; // Output: Uppercase: A
return 0;
}
Understanding `toupper`
What is `toupper`?
The `toupper` function is a part of the C++ Standard Library, specifically found in the `<cctype>` header. Its main purpose is to convert a given character to its uppercase equivalent. This function is particularly useful when manipulating string data, such as user input or database entries, allowing for a controlled standardization of text.
Syntax of `toupper`
The function has a simple syntax:
int toupper(int ch);
- Parameter: The function takes a single argument `ch` that represents a character (it can be passed as an `int`).
- Return Value: It returns the uppercase version of the character if applicable. If the character is already uppercase or is not an alphabet letter, it will return the character unchanged.
It's important to note that `toupper` operates based on the ASCII character set, which means it will produce expected results primarily for English letters.

Including Required Libraries
Standard Header File
To use the `toupper` function, you need to include the necessary header file in your program:
#include <cctype>
This inclusion is essential as it contains the declaration for `toupper`, along with a collection of other useful character handling functions. Neglecting to include this header will lead to compilation errors.

Practical Example of Using `toupper`
Simple Character Conversion
Let’s start with a straightforward example where we convert a single lowercase character to uppercase. Here's a simple program demonstrating this:
#include <iostream>
#include <cctype>
int main() {
char lower = 'a';
char upper = toupper(lower);
std::cout << "Original: " << lower << ", Uppercase: " << upper << std::endl;
return 0;
}
Explanation: In this code, we define a character `lower` assigned the value `'a'`, and then use `toupper` to convert it to uppercase, storing the result in `upper`. The program subsequently prints both the original and converted characters. This shows how `toupper` easily converts lowercase to uppercase.
Converting a String Using `toupper`
Iterating Over a String
You can also convert an entire string to uppercase using a loop. Here’s an example that demonstrates this:
#include <iostream>
#include <string>
#include <cctype>
std::string convertToUpper(const std::string& str) {
std::string result;
for(char ch : str) {
result += toupper(ch);
}
return result;
}
int main() {
std::string input = "hello world";
std::string output = convertToUpper(input);
std::cout << "Uppercase: " << output << std::endl;
return 0;
}
Explanation: In this code, we define a function `convertToUpper` which takes a string as input. By iterating over each character in the string, the function applies `toupper` to convert each character to uppercase, appending it to the `result` string. Finally, the main function captures a sample input string and outputs its uppercase version. This illustrates how you can easily transform strings using `toupper`.

Common Use Cases for `toupper`
Data Normalization
`toupper` is instrumental when it comes to data normalization. In many applications, ensuring that all user inputs are consistent, such as converting all text to uppercase, can help eliminate discrepancies during comparisons. For instance, when storing usernames, converting them to uppercase can avoid conflicts caused by case sensitivity.
Ensuring Consistency in Comparisons
Consistency in comparisons is critical, especially in situations where user input or data retrieval from databases is involved. For example, consider the following scenario:
std::string a = "apple";
std::string b = "Apple";
if (toupper(a[0]) == toupper(b[0])) {
// perform some action
}
In this example, we are comparing the first characters of two strings in a case-insensitive manner. By converting both characters to uppercase using `toupper`, you ensure that the comparison function works accurately regardless of the original case of the letters.

Performance Considerations
Efficiency of Using `toupper`
When considering performance, the `toupper` function is quite efficient for converting characters. The computational complexity is typically O(n) for iterating through strings, where n is the length of the string. However, when working with large datasets, you may want to profile your application to ensure that character conversion does not introduce significant delays.

Potential Pitfalls
Non-Alphabet Characters
One must be aware of how `toupper` handles non-alphabet characters. If you pass a number or a special character, the output will remain unchanged. Here’s an illustrative example:
char ch = '1';
std::cout << "Input: " << ch << ", Output: " << toupper(ch) << std::endl; // Output remains '1'
Explanation: In this case, the character `'1'` is not affected by the `toupper` function, demonstrating that the function only affects alphabetic characters.
Locale Considerations
Importance of Locale with `toupper`
Bear in mind that `toupper` operates based on the default "C" locale. In regions where character sets differ (e.g., languages that use special character sets), using `toupper` could yield unexpected results. For more locale-aware conversion, utilize `std::toupper` from the `<locale>` library:
#include <locale>
char toupperLocale(char ch) {
std::locale loc;
return std::toupper(ch, loc);
}
Explanation: This implementation allows for case conversion that respects the specified locale, making it a robust choice for international applications.

Conclusion
The `toupper` function in C++ serves as a critical tool for character manipulation, empowering developers to effectively manage and standardize text data. By understanding and utilizing this simple function, you can enhance the reliability of your applications significantly.

Additional Resources
References for Further Learning
For those who wish to delve deeper into character manipulation in C++, consider exploring the following resources:
- C++ Standard Library documentation
- Online courses focusing on C++ programming
- Books on advanced C++ topics
Community and Support
Joining forums and communities, like Stack Overflow or Reddit’s C++ subreddit, will provide you with additional support as you continue your learning journey. Engaging with others can lead to valuable insights and new techniques.
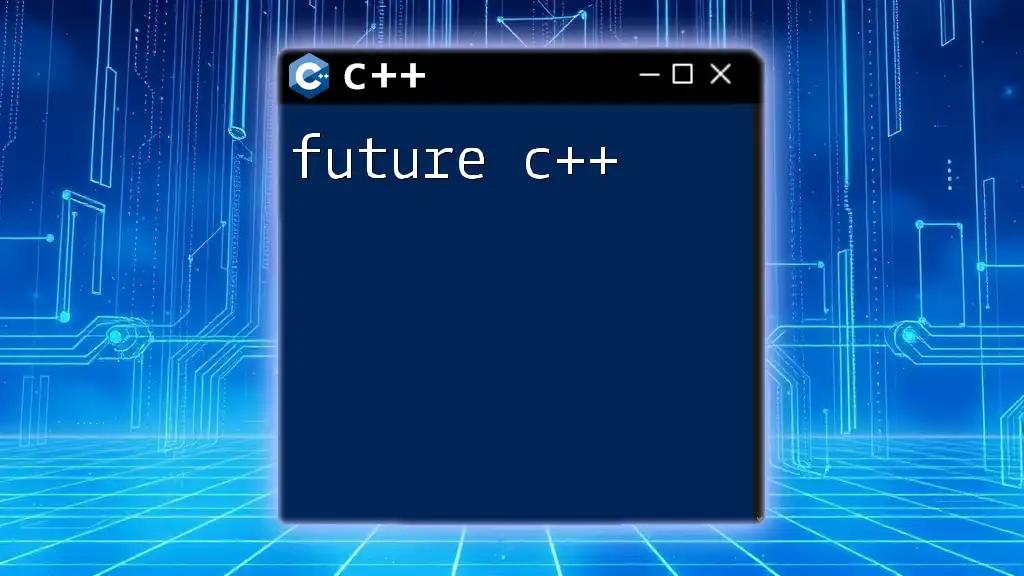
Call to Action
Start integrating `toupper` into your C++ projects to experience firsthand the difference effective character manipulation can make. Share your experiences and challenges with `toupper`, and let the programming community assist you in improving your coding skills!