ReSharper C++ is a powerful extension for Visual Studio that enhances C++ development with features such as code analysis, refactoring, and navigation, enabling developers to write cleaner and more efficient code.
Here’s an example of using ReSharper C++ for refactoring variable names:
int myVariable = 10; // Original variable name
// Press Ctrl + R, R to rename via ReSharper
After renaming, it could become:
int count = 10; // Renamed variable for better clarity
Setting Up ReSharper C++
Installation Process
To start leveraging the powerful features of ReSharper C++, you first need to install it on your system. Ensure that your environment meets the system requirements, including compatible versions of Visual Studio. Follow these steps to install:
- Download the Installer: Visit the JetBrains website and download the ReSharper installer.
- Run the Installer: Follow the prompts in the installer to complete your setup.
- Activate Your License: If you have a valid license, enter the details during the activation process.
After installation, setting up ReSharper C++ to suit your workflow is crucial. You can customize various aspects of the tool, including settings for code styles and formatting, to ensure it aligns with your coding standards and preferences.
Configuring ReSharper C++
To configure ReSharper C++, you can access its options through the main Visual Studio menu. Here’s how to tailor the settings for an optimal experience:
- Code Styles: Navigate to ReSharper > Options > Code Editing > C++ > Code Style. Here, you can set guidelines for formatting, whitespace, and more.
- Integration Settings: Make sure ReSharper integrates seamlessly with Visual Studio's interface. Check for updates regularly to ensure synchronization.
- Custom Shortcuts: Create or modify keyboard shortcuts for various ReSharper functionalities to improve efficiency.
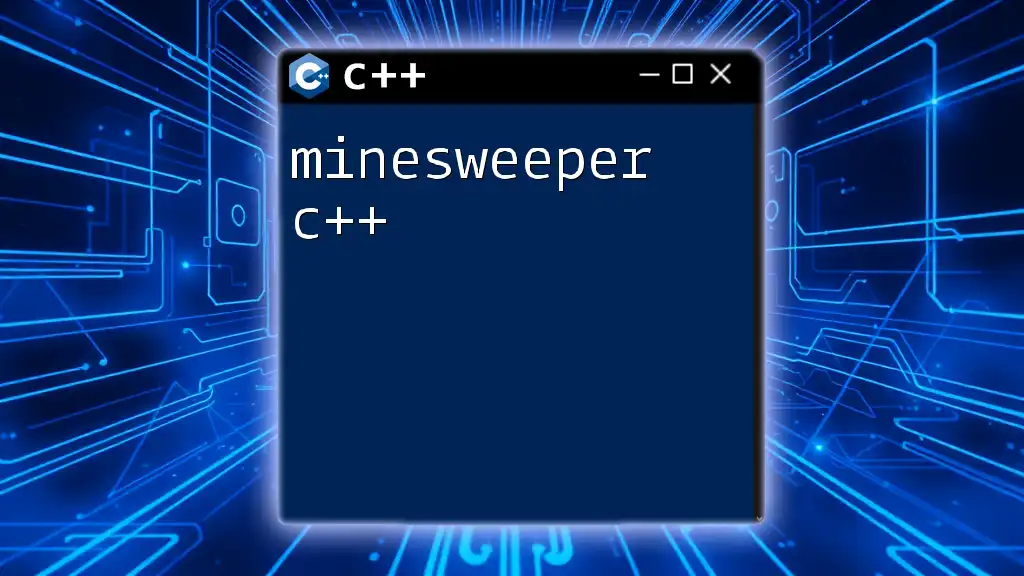
Key Features of ReSharper C++
Code Analysis
Understanding the code analysis capabilities of ReSharper C++ is pivotal for maintaining code quality. ReSharper performs real-time checks on your code, providing immediate feedback on issues ranging from syntax errors to code smells.
Examples of Code Analysis Tools
One of the most significant advantages is the ability to receive quick-fix suggestions. For example, if your loop structure is suboptimal, ReSharper might suggest a more efficient approach:
for(int i = 0; i < arr.size(); ++i) {
// process arr[i]
}
In response, ReSharper offers alternatives like using a range-based for loop, which enhances readability and performance. Furthermore, it can highlight unused variables, ensuring your code remains clean and efficient.
Refactoring Tools
Refactoring is essential for improving the structure of existing code without altering its external behavior. ReSharper C++ offers rich refactoring tools that make this process easier and safer.
Common Refactoring Actions
ReSharper provides a variety of refactoring options:
- Rename: Changes variable, function, or class names while updating all references.
- Change Signature: Modifies function parameters with automated updates throughout your code.
- Extract Function: Allows you to create a new function from a block of code, improving modularity.
- Inline Variable: Replaces references to a variable with its actual value and removes the variable itself.
Refactoring Examples
Consider the following simple function that calculates the square of a number:
int MyFunction(int x) {
return x * x;
}
Using ReSharper, you can easily rename it from `MyFunction` to `CalculateSquare` while automatically updating all its references in your code. The result:
int CalculateSquare(int number) {
return number * number;
}
This demonstrates how ReSharper can streamline refactoring, ensuring consistency and preventing errors.
Navigation and Search
Efficient navigation through code is critical, especially in large projects. ReSharper C++ significantly enhances your ability to jump between files, methods, and definitions seamlessly.
Key Navigation Features
ReSharper allows you to:
- Go to Declaration: Quickly navigate to a function or method's definition.
- Find Usages: Identify all locations where a variable or function has been used across your codebase.
- Navigate to Base/Super Class: Instantly access parent classes in inheritance hierarchies.
By mastering these navigation features, you improve your productivity, saving time that would otherwise be spent searching through your project.
Navigational Shortcuts
Incorporating keyboard shortcuts can vastly improve your workflow. Here are some essential shortcuts:
- `Ctrl` + `T` to open the "Go to Everything" dialog.
- `Shift` + `F12` to find usages.
- `F12` to go to the declaration.
Learning and utilizing these shortcuts will streamline your coding processes.
Code Completion and Suggestions
ReSharper C++ makes coding faster and more accurate through its intelligent code completion features. By understanding the context of your code, it can provide useful suggestions.
Using Code Completion in Practice
Imagine you have a function declaration as follows:
void MyFunction(int x);
When you start typing `MyF`, ReSharper displays options to complete the method name, facilitating faster coding. As you type parameters, it can also suggest type-specific completions, greatly enhancing your productivity and reducing errors.
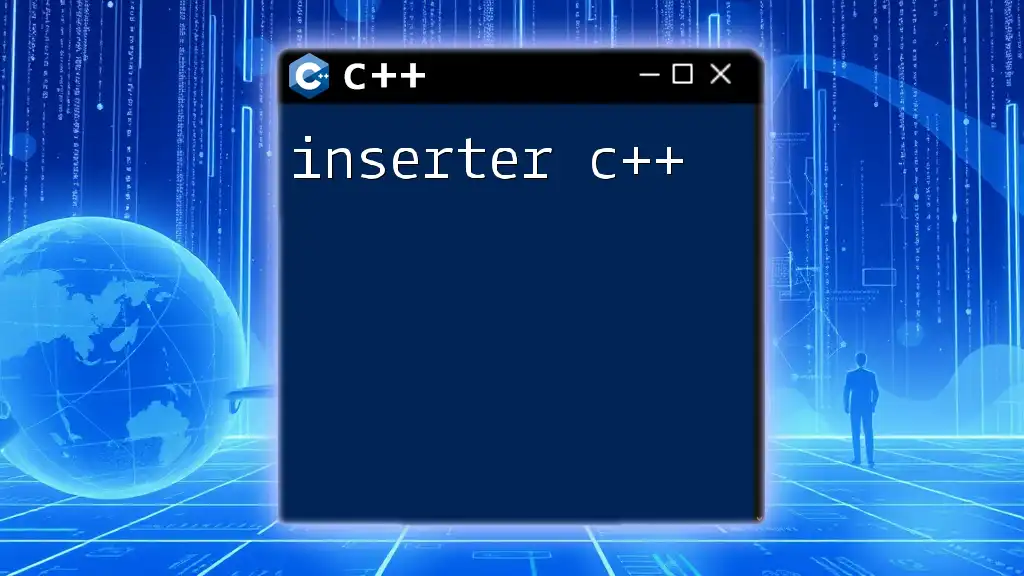
Customizing ReSharper C++
Customizing ReSharper C++ to fit your specific requirements can have a significant impact on your development workflow.
Custom Code Templates
You can create and manage code templates by accessing ReSharper > Templates Explorer. These templates can be incredibly useful for repetitive coding tasks, ensuring consistency and saving time.
Adding External Tools and Plugins
ReSharper integrates seamlessly with various external tools and plugins, enabling a more customizable development environment. Access the Extensions section in the ReSharper options to find additional resources tailored to your use case.
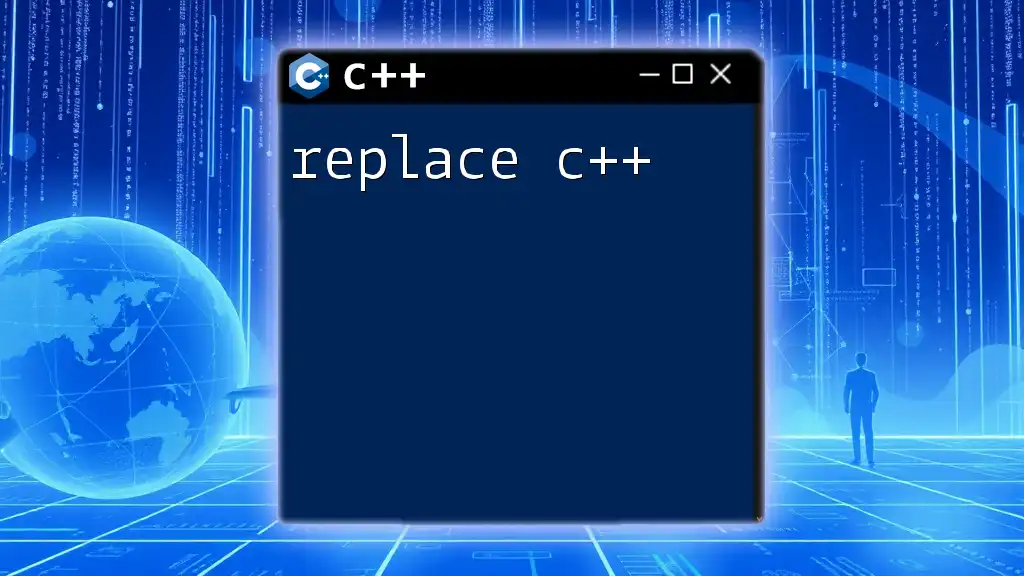
Troubleshooting Common Issues
As with any powerful tool, users may encounter performance issues or conflicts with other plugins. Here are a few tips to troubleshoot effectively:
Performance Tips
For optimal performance, particularly in large codebases, consider the following:
- Regularly update ReSharper to the latest version to benefit from performance optimizations.
- Temporarily disable code analysis for files you know are not a priority to reduce clutter.
Dealing with Conflicts
In some cases, you might face conflicts with other Visual Studio extensions. To address these issues:
- Disable or remove conflicting extensions.
- Check the settings of each plugin to ensure they do not interfere with ReSharper functionalities.
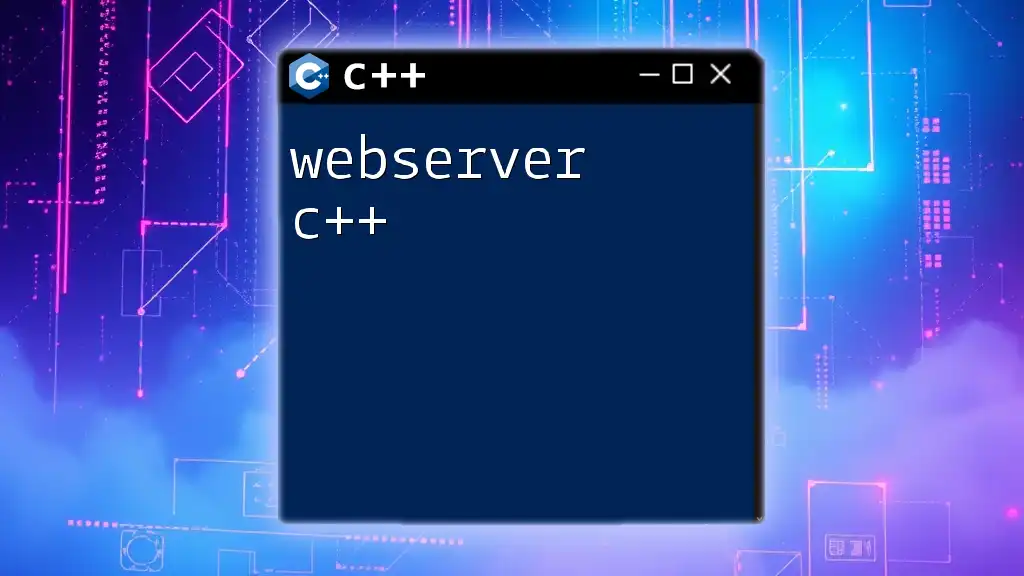
Conclusion
ReSharper C++ emerges as an invaluable tool in the toolkit of modern C++ developers. With its array of features aimed at improving code quality and enhancing productivity, mastering ReSharper can lead to more maintainable and cleaner codebases.
Encourage readers to explore ReSharper, experiment with its features, and integrate it into their daily workflows for a more efficient coding experience. By understanding how to leverage its capabilities effectively, you can elevate your C++ development practices to new heights.