In C++, the `swap` function is used to exchange the values of two variables efficiently.
Here's a code snippet demonstrating the use of `swap`:
#include <iostream>
#include <algorithm> // for std::swap
int main() {
int a = 5, b = 10;
std::swap(a, b);
std::cout << "a: " << a << ", b: " << b << std::endl; // Output: a: 10, b: 5
return 0;
}
Understanding Swapping in C++
What is Swapping?
Swapping refers to the exchange of values between two variables. In programming, this is a fundamental operation often utilized in algorithms that require rearranging elements or changing their order. To illustrate, consider the action of exchanging two items, like switching the positions of two books on a shelf. In both cases, the essential operation remains the same: the items' locations or values are interchanged.
Why Use Swapping?
Swapping is crucial for various reasons. It serves as a basic building block in numerous algorithms, especially sorting algorithms like bubble sort or quicksort. By efficiently swapping values, we can optimize the performance of our algorithms, ultimately leading to faster code execution.
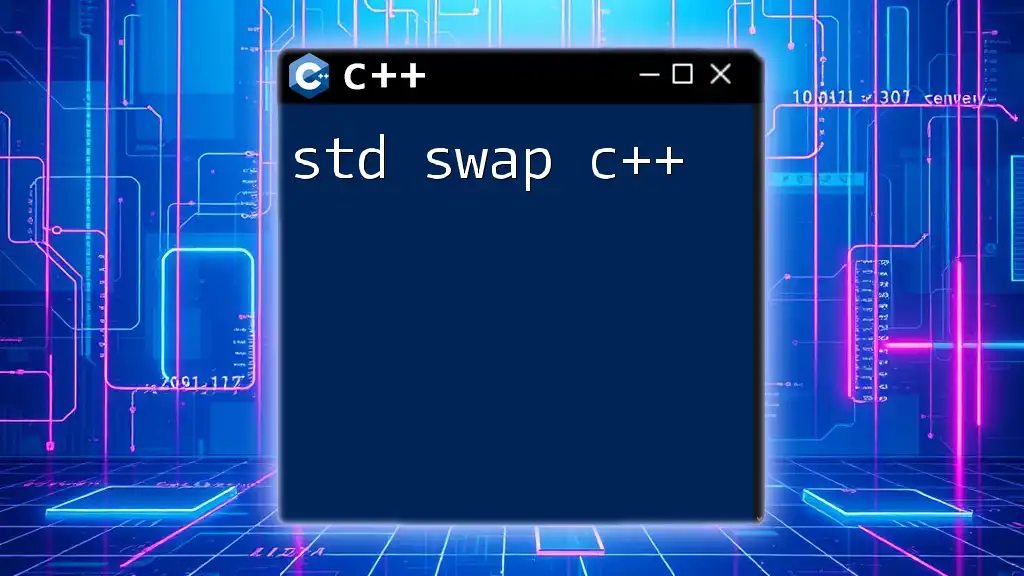
Introduction to `std::swap`
What is `std::swap`?
`std::swap` is a function in C++ provided by the Standard Library to perform the swapping operation. Its signature reads as follows:
template <class T> void swap(T& a, T& b);
This function takes two parameters, a and b, both passed by reference, and swaps their values. It's a generic function, adaptable to any data type, including built-in types, objects, and even user-defined types.
When to Use `std::swap`
You should consider using `std::swap` in situations where you need to exchange values efficiently. This function comes in handy during operations like sorting, rearranging data, or even when implementing algorithms that require exchanging large data structures. Its simplicity and efficiency make it a versatile tool in a programmer's toolkit.
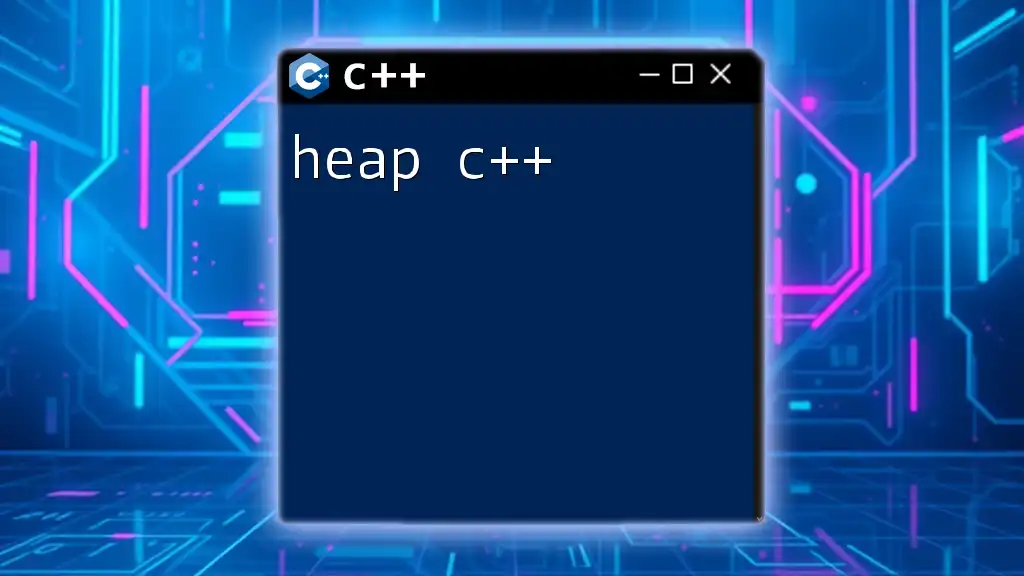
How `std::swap` Works
The Mechanism of Swapping
`std::swap` works by copying the values of the two variables and assigning them to each other. When you invoke `std::swap(a, b)`, the function temporarily stores the value of a in a local variable and then assigns the value of b to a. Finally, it assigns the temporary value to b. Because the parameters are passed by reference, the actual variables' values are modified directly without creating unnecessary copies.
Code Snippet: Basic Usage
Here is a simple example showing the use of `std::swap`:
#include <iostream>
#include <utility> // for std::swap
int main() {
int x = 10, y = 20;
std::swap(x, y);
std::cout << "x: " << x << ", y: " << y; // x: 20, y: 10
return 0;
}
In the example above, after calling `std::swap`, the values of x and y are swapped, demonstrating how easily we can interchange values using `std::swap`.
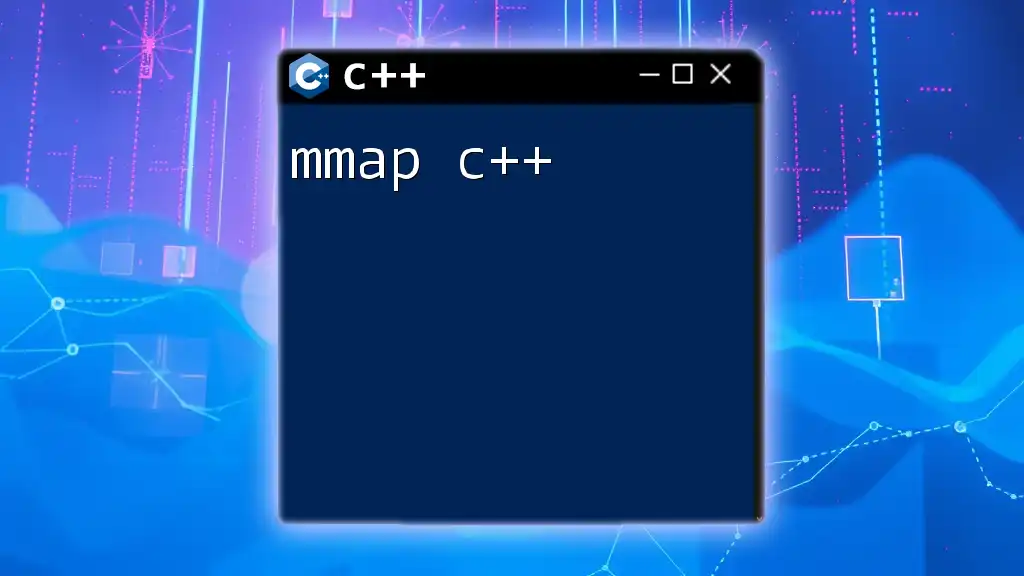
Custom Swap Functions
Writing Your Own Swap Function
While `std::swap` is efficient for most standard uses, you might find it necessary to create a custom swap function, especially for complex types with dynamic resources. When designing a custom swap, ensure it follows the same principles as `std::swap`, focusing on efficient value exchanges.
Code Snippet: Custom Swap Example
Here's an example of how to create a custom swap function for a user-defined class:
struct Point {
int x, y;
void swap(Point& other) {
std::swap(x, other.x);
std::swap(y, other.y);
}
};
In this example, the `Point` structure has its own swap function, which uses `std::swap` internally to exchange the x and y coordinates. This approach ensures that the swapping logic is encapsulated within the class and can handle more complex operations if necessary.
Using Custom Swap with `std::swap`
You can further enhance your custom swaps by utilizing `std::swap` within them to benefit from the generic nature of the function, ensuring that even if the class logic changes, the swapping remains efficient and effective.
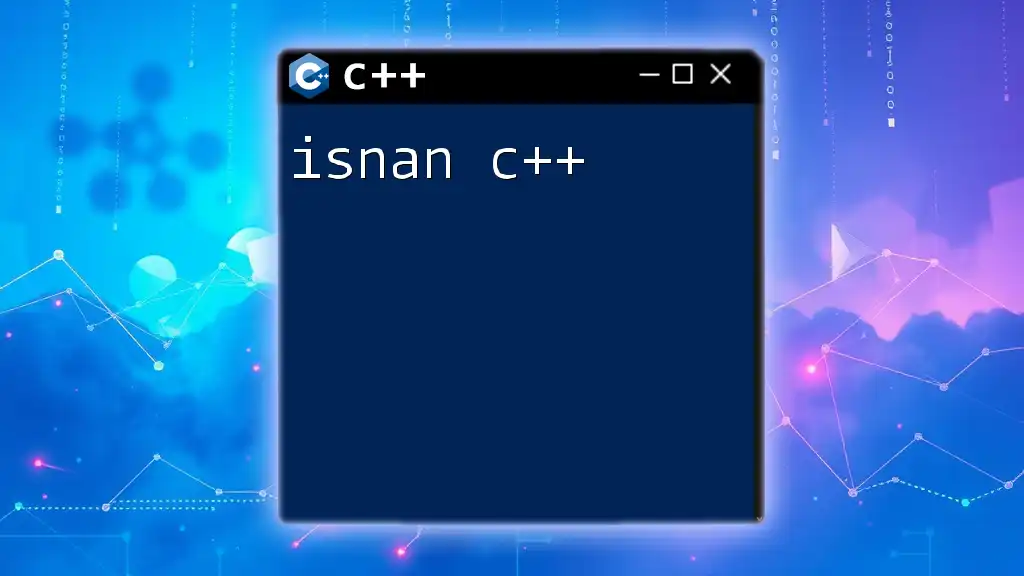
Performance Considerations
Complexity of `std::swap`
The time complexity of `std::swap` is generally constant (O(1)), making it a highly efficient operation when used correctly. However, in certain scenarios—particularly with large objects or types that manage dynamic resources—the performance could be impacted. Always analyze whether swapping might involve a deep copy, which can slow down performance.
Move Semantics and `std::swap`
C++11 introduced move semantics, which greatly enhance the efficiency of operations involving temporary objects. The concept allows resources to be transferred rather than copied. This is particularly beneficial when used in conjunction with `std::swap`, as the swapping of large structures can be executed through moves rather than copies.
Code Snippet: Using Move Semantics
The following example demonstrates how to leverage move semantics in a class with `std::swap`:
#include <iostream>
#include <utility>
#include <vector>
class Widget {
public:
Widget() {}
Widget(Widget&& other) noexcept {
// Transfer ownership of resources
}
// Implement move assignment operator
};
int main() {
Widget a, b;
std::swap(a, b); // Efficient swap using move semantics
return 0;
}
In this snippet, `std::swap(a, b)` can now use move semantics, making the operation more efficient when dealing with resources managed by the `Widget` class.
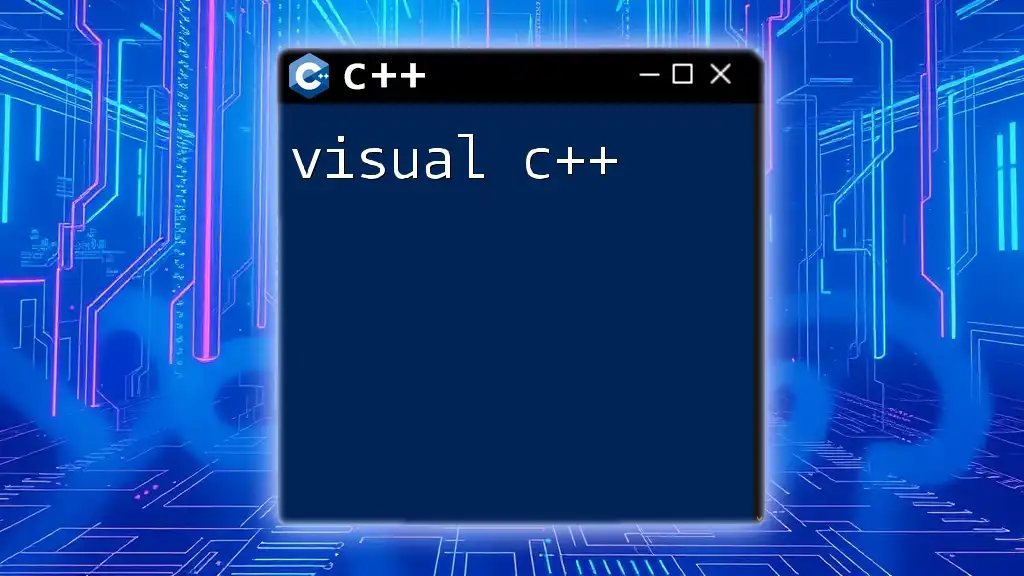
Real-World Applications of `std::swap`
Swapping in Data Structures
In many STL (Standard Template Library) containers, such as vectors or lists, `std::swap` plays a pivotal role in optimizing performance by allowing elements to be exchanged quickly and efficiently. For instance, when resizing a vector, `std::swap` helps minimize the number of copies made when adjusting internal structures.
Code Snippet: Swapping in Sort Algorithms
Swapping is often encountered in sorting algorithms. Here’s how you might implement a bubble sort that utilizes `std::swap`:
#include <algorithm>
#include <vector>
void bubbleSort(std::vector<int>& arr) {
int n = arr.size();
for (int i = 0; i < n - 1; ++i) {
for (int j = 0; j < n - i - 1; ++j) {
if (arr[j] > arr[j + 1]) {
std::swap(arr[j], arr[j + 1]);
}
}
}
}
This example demonstrates how `std::swap` facilitates the process of swapping elements during the sorting routine, ultimately leading to a correctly ordered array.
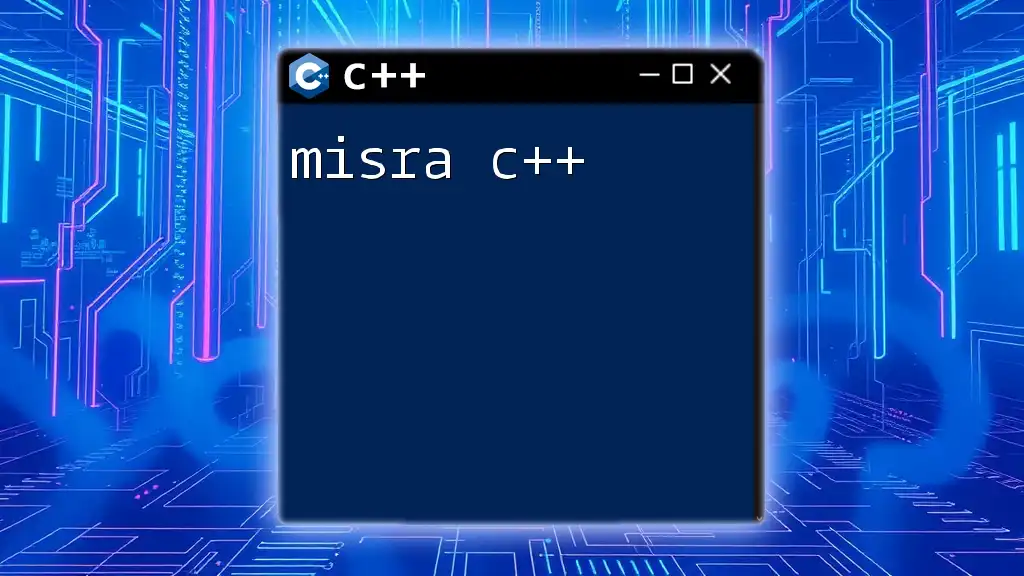
Common Mistakes and Troubleshooting
Common Pitfalls when Using `std::swap`
A frequent mistake programmers make when using `std::swap` is misunderstanding how references work. If you mistakenly pass objects by value instead of by reference, the original objects remain unchanged. Always ensure parameters are passed correctly to avoid such issues.
Debugging Swapping Issues
Occasionally, you might encounter unexpected behavior when using `std::swap`. To troubleshoot, consider the following steps:
- Ensure you're passing variables by reference.
- Check the data types involved for compatibility.
- Review the custom swap implementation for logical errors.
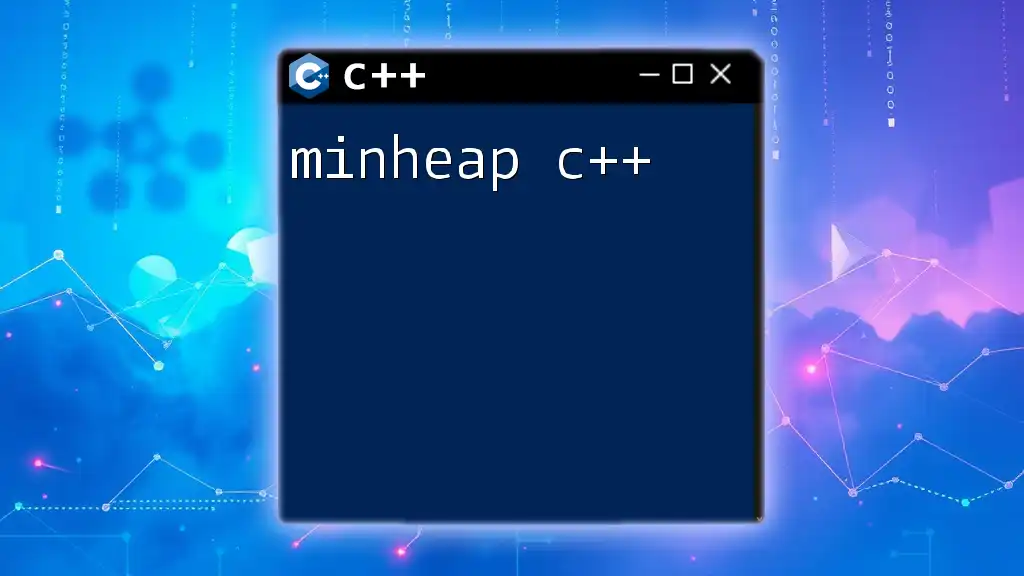
Conclusion
In conclusion, `std::swap` is a powerful tool in C++ that simplifies the swapping process, enhancing the performance and readability of code. By understanding its mechanics, performance implications, and real-world applications, developers can utilize this function effectively across their programming tasks. Embrace `swap c++` in your projects to streamline your operations and optimize your algorithms. For further learning, explore advanced topics or attempt exercises that will reinforce your understanding of this fundamental C++ feature.
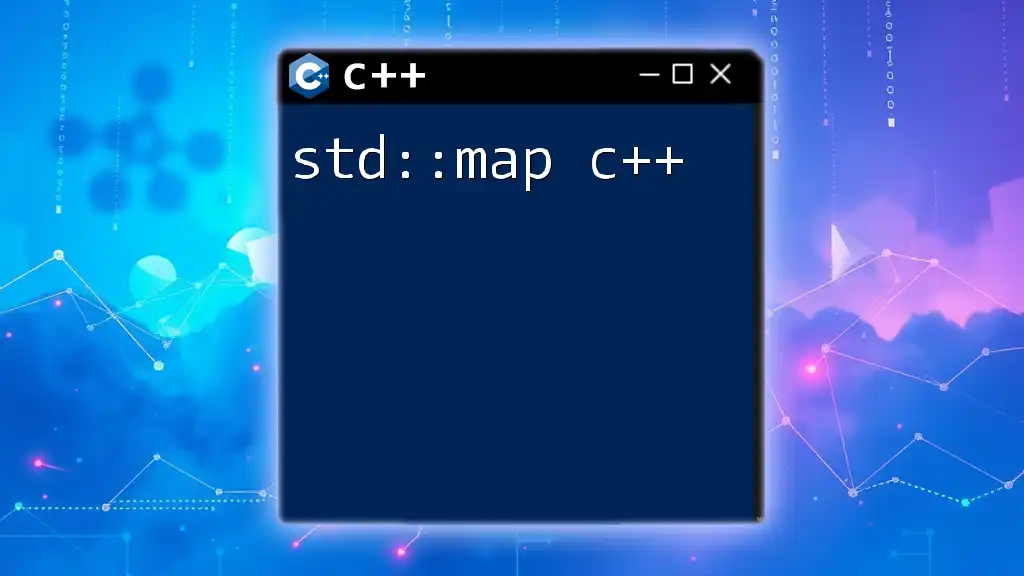
Additional Resources
For those looking to deepen their understanding of swapping and other C++ functionalities, consider exploring the official C++ documentation, online tutorials, or relevant exercises that allow for practical application of the concepts discussed in this guide.