The `std::swap` function in C++ efficiently exchanges the values of two variables by utilizing a simple templated function.
Here's a code snippet demonstrating its usage:
#include <iostream>
#include <algorithm> // for std::swap
int main() {
int a = 5, b = 10;
std::swap(a, b);
std::cout << "a: " << a << ", b: " << b << std::endl; // Output: a: 10, b: 5
return 0;
}
What is std::swap?
`std::swap` is a powerful utility function in C++ that facilitates the exchange of values between two variables. It is defined in the `<utility>` header of the C++ Standard Library and plays an essential role in managing resources effectively by enabling swappable objects, which are critical for tasks like sorting and rearranging data.
By utilizing `std::swap`, you can swap the values of two variables without the overhead of manually transferring data. This improves both performance and code clarity, aligning with the C++ philosophy of maintaining efficient resource management.

The Syntax of std::swap
The basic syntax of `std::swap` is as follows:
namespace std {
template <typename T>
void swap(T& a, T& b) noexcept;
}
In this declaration:
- `T& a` and `T& b` represent the two variables whose values you wish to swap. They are passed by reference, allowing the function to modify the original variables.
- The `noexcept` keyword signifies that `std::swap` will not throw exceptions, which can enhance performance in certain situations by allowing optimizers to make assumptions about exception safety.
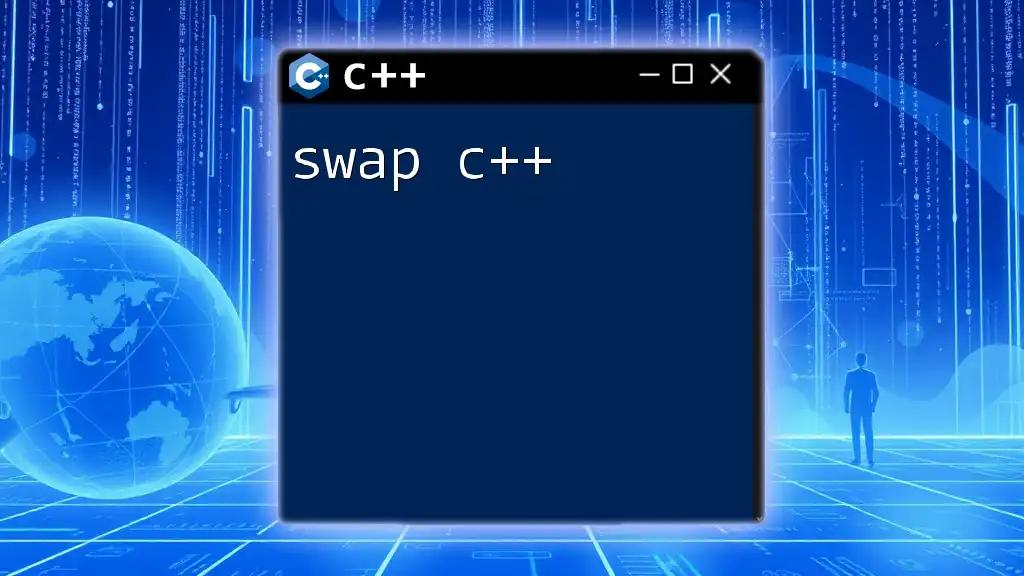
How std::swap Works Under the Hood
To comprehend how `std::swap` operates, consider that it typically relies on three simple steps:
- Copy the value of the first variable to a temporary variable.
- Assign the value of the second variable to the first variable.
- Assign the value from the temporary variable back to the second variable.
In modern C++ (C++11 and newer), thanks to move semantics, `std::swap` can perform these operations very efficiently by transferring ownership of resources rather than duplicating them, making it ideal for managing heap-allocated memory or other resources.

Using std::swap with Different Data Types
Built-in Types
Using `std::swap` is straightforward with fundamental data types. Here's a simple example:
int a = 5;
int b = 10;
std::swap(a, b);
After executing this code, the values will be swapped, so `a` will be `10` and `b` will be `5`. The beauty of `std::swap` is that it abstracts away the manual work of swapping values, leading to cleaner and more maintainable code.
User-defined Types
When working with user-defined types, you must ensure that `std::swap` is appropriately overloaded. Here's an example of a simple class and how to use `std::swap` with it:
class MyClass {
public:
int value;
MyClass(int v) : value(v) {}
};
For this class, you can use `std::swap` directly, assuming it has no internal pointers. However, if your class contains dynamically allocated resources (like pointers), you should implement a custom swap for efficiency, as shown later in this article.

Advantages of Using std::swap
Efficiency
One of the primary benefits of using `std::swap` is efficiency. Consider this: manually swapping values might involve multiple assignments, potentially leading to extra overhead. However, `std::swap`, particularly with modern C++, optimizes resource management by using move semantics, which can significantly reduce copying and improve performance.
Code Clarity
Another compelling reason to use `std::swap` is that it enhances code clarity. Instead of writing verbose logic to exchange two values, you can express your intent succinctly:
std::swap(x, y);
This single line is self-explanatory and less error-prone compared to writing a manual swap function.
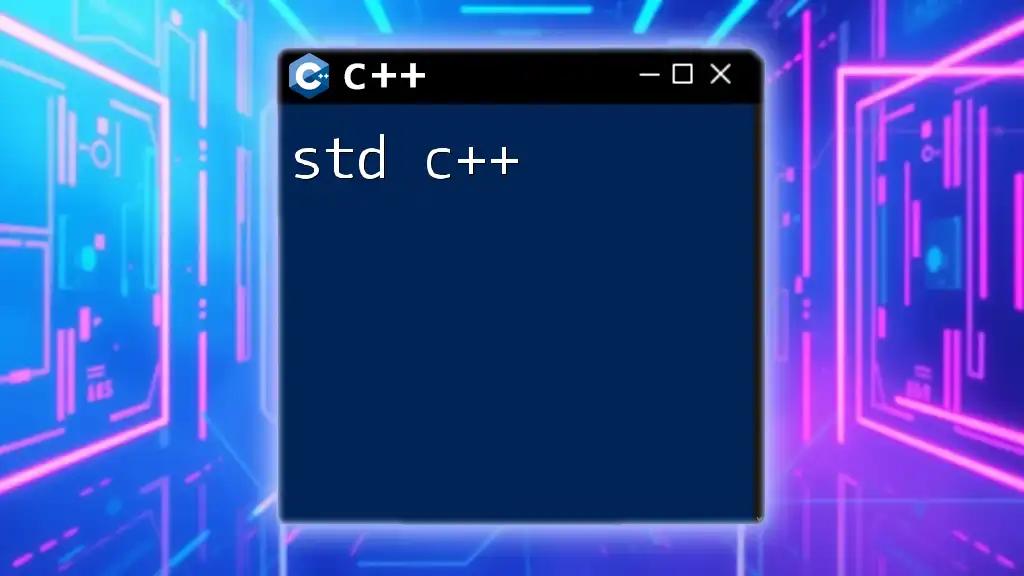
Implementing Custom Swaps for User-defined Types
Utilizing the Copy-and-Swap Idiom
To manage user-defined types appropriately, especially those that manage resources, you can use the copy-and-swap idiom. The idiom provides exception safety and simplifies your code. Here’s an illustrative example:
class MyClass {
public:
int* data;
MyClass(int value) : data(new int(value)) {}
~MyClass() { delete data; }
// Copy constructor
MyClass(const MyClass& other) : data(new int(*other.data)) {}
// Swap function
friend void swap(MyClass& first, MyClass& second) noexcept {
using std::swap; // Enable ADL
swap(first.data, second.data);
}
};
In this example, the custom swap function uses `std::swap` to exchange the `data` pointers, ensuring that the memory is handled appropriately without duplicates. This approach not only improves performance but also maintains exception safety, since swapping does not throw.

Practical Examples of std::swap
Swapping Integers
Here’s a basic illustration:
int x = 2, y = 3;
std::swap(x, y);
After running this code, `x` will be `3`, and `y` will be `2`. This simple use case demonstrates how easily `std::swap` can manage basic types.
Swapping Pointers
Using `std::swap` with pointers can simplify memory management as well. For instance:
int* ptr1 = new int(10);
int* ptr2 = new int(20);
std::swap(ptr1, ptr2);
Post-swap, `ptr1` now points to `20`, while `ptr2` points to `10`. This can be helpful when handling resources that rely on pointer semantics.
Swapping Containers
`std::swap` is also extremely useful with STL containers. Here’s how you can swap entire vectors, which can be more efficient than manual element-by-element swaps:
std::vector<int> vec1 = {1, 2, 3};
std::vector<int> vec2 = {4, 5, 6};
std::swap(vec1, vec2);
In this case, the contents of `vec1` and `vec2` are exchanged effectively, saving on the overhead of copying individual elements.

Common Pitfalls to Avoid
While using `std::swap`, you must be cautious about its application with non-swappable types. For example, if an object cannot be copied or moved (like certain unique pointers without a custom swap implementation), attempting to use `std::swap` could lead to compilation errors. Be vigilant about the types you're working with, and always consider implementing custom swaps for classes that manage unique resources.
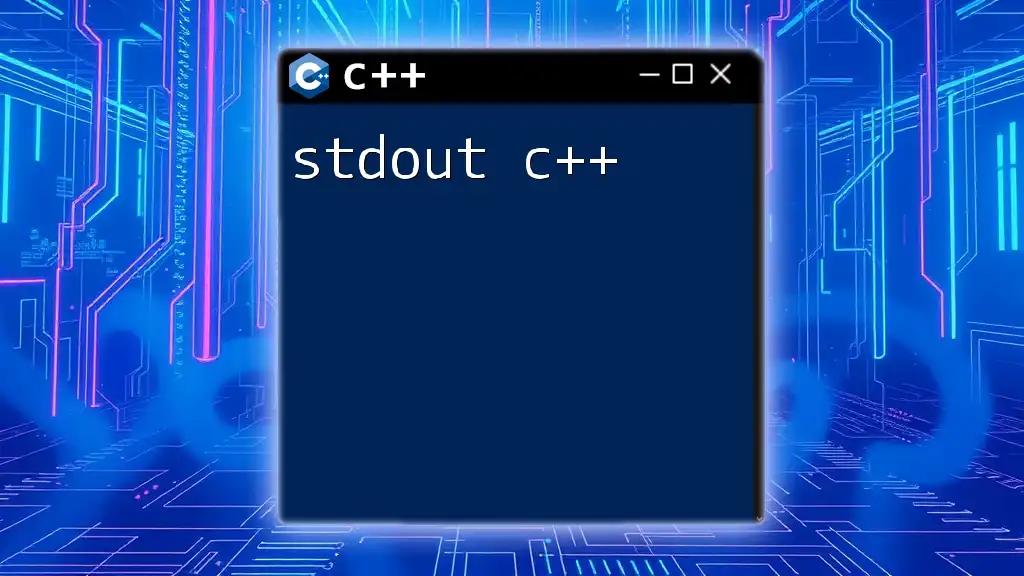
Conclusion
In summary, `std::swap` in C++ is an essential tool for effective resource management and code clarity. Its efficiency in exchanging values and the simplicity it brings to your code cannot be overstated. Whether you're swapping built-in types or customizing it for user-defined types, mastering `std::swap` will improve your coding practice significantly.

Additional Resources
To further explore `std::swap`, check the following resources:
- Official C++ documentation on `<utility>`.
- Recommended C++ literature and online tutorials for best practices in C++ programming.
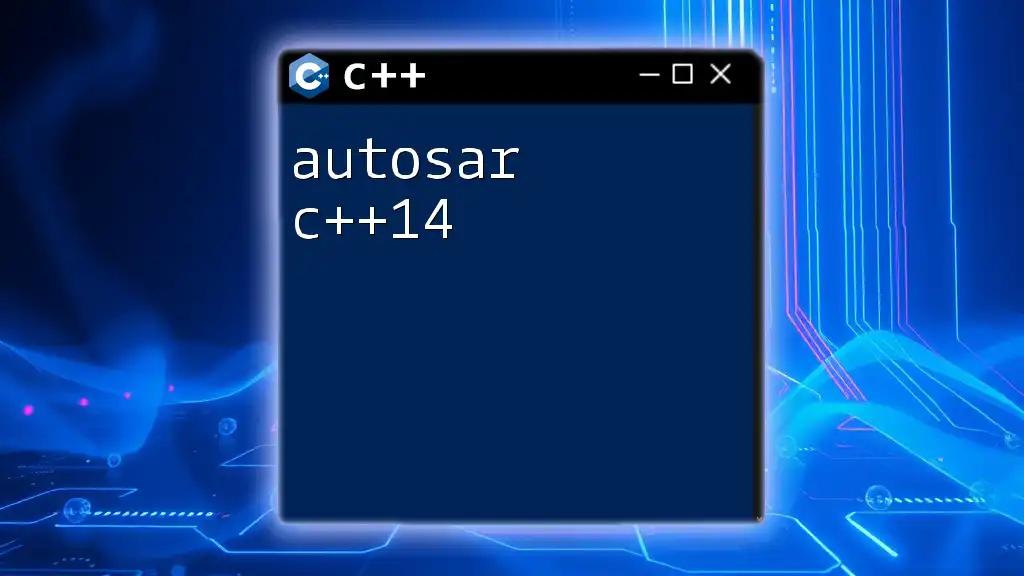
Call to Action
Try implementing `std::swap` in your own projects and explore its full potential. Share your experiences and insights on social media, and let’s enrich our understanding of C++ together!