The `std::copy` function in C++ is used to copy elements from one range to another efficiently.
#include <algorithm>
#include <vector>
#include <iostream>
int main() {
std::vector<int> source = {1, 2, 3, 4, 5};
std::vector<int> destination(5);
std::copy(source.begin(), source.end(), destination.begin());
for (int n : destination) {
std::cout << n << ' '; // Output: 1 2 3 4 5
}
return 0;
}
What is std::copy?
`std::copy` is a powerful function provided by the C++ Standard Library that streamlines the process of copying elements from one range to another. Its primary purpose is to facilitate the transfer of data between different containers or within the same container. Understanding how to effectively use `std::copy` can significantly improve the efficiency of your code.
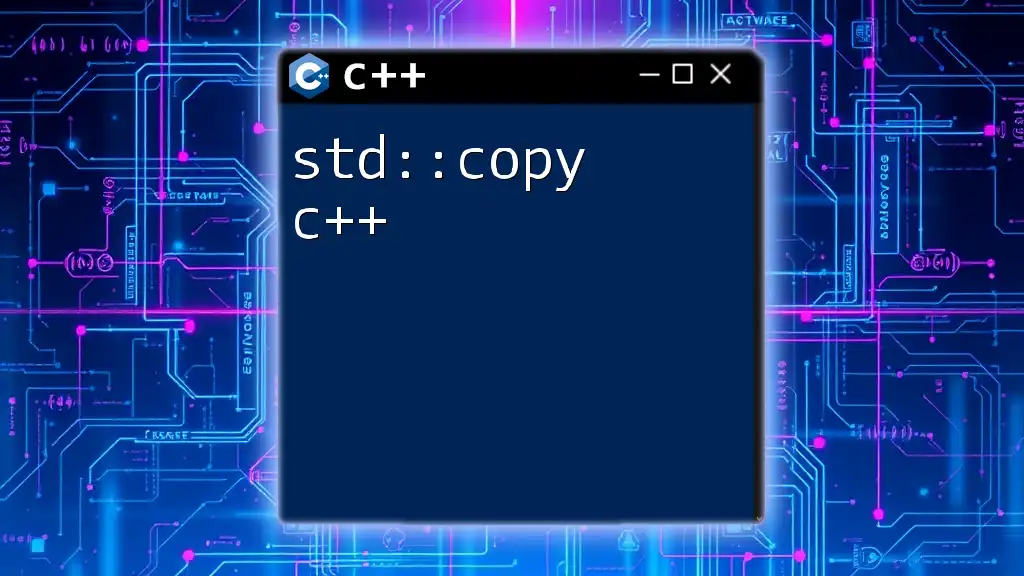
When to Use std::copy?
You may find yourself needing to copy data in various programming scenarios, such as:
- When you need to duplicate elements from an array into another array or from one vector to another.
- When transforming or processing a collection of objects and you want to keep the original intact.
Using `std::copy` is often preferred over manual copying methods like loops due to its concise syntax and efficiency. By leveraging iterator capabilities, `std::copy` can handle various types of containers seamlessly.
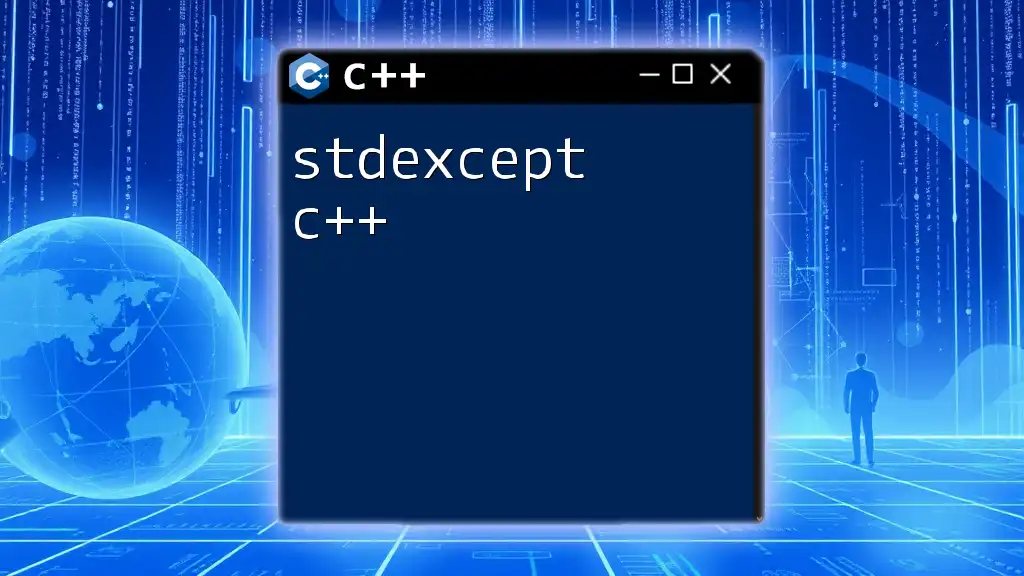
Syntax of std::copy
Function Prototype
The syntax for `std::copy` can be expressed as follows:
template< class InputIt, class OutputIt >
OutputIt std::copy( InputIt first, InputIt last, OutputIt d_first );
Parameters Explained
- InputIt first: This parameter specifies the starting point of the range to copy from—essentially the beginning of the source data.
- InputIt last: This indicates the endpoint of the source data. The copy will occur until this point.
- OutputIt d_first: This is where the copied elements will be written to. It indicates the start of the destination range.
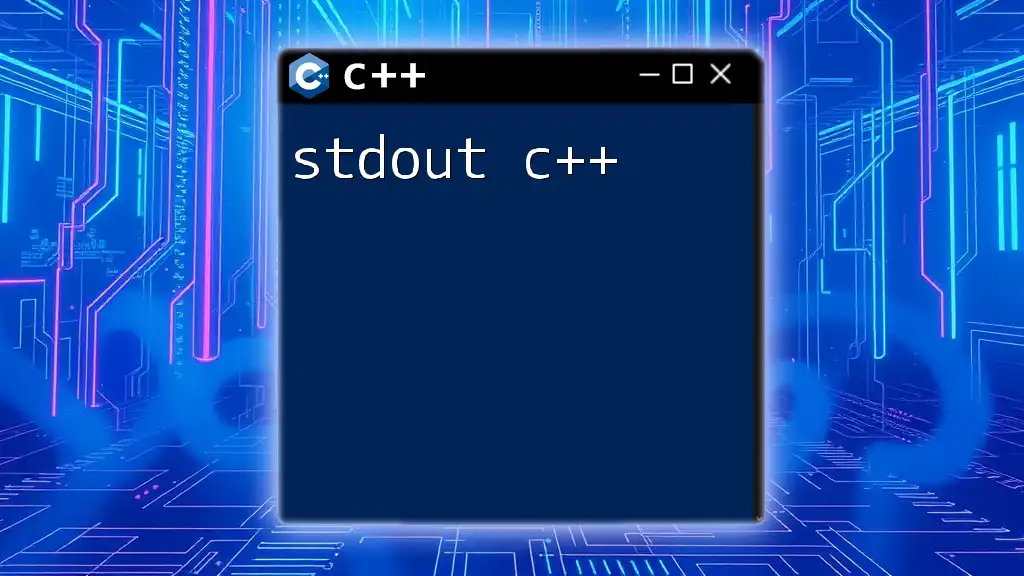
How to Use std::copy
Basic Example
Let's illustrate `std::copy` with a straightforward example that uses arrays:
#include <iostream>
#include <algorithm>
#include <iterator>
int main() {
int source[] = {1, 2, 3, 4, 5};
int dest[5];
std::copy(std::begin(source), std::end(source), std::begin(dest));
for (int n : dest) {
std::cout << n << ' ';
}
return 0;
}
Explanation
In this example:
- We define an integer array named `source` and an equally sized `dest` array.
- `std::copy` initiates copying from the initial element of `source` to the final element, placing the result into `dest`.
- The `for` loop then displays the contents of `dest`, ensuring that the data was copied correctly.
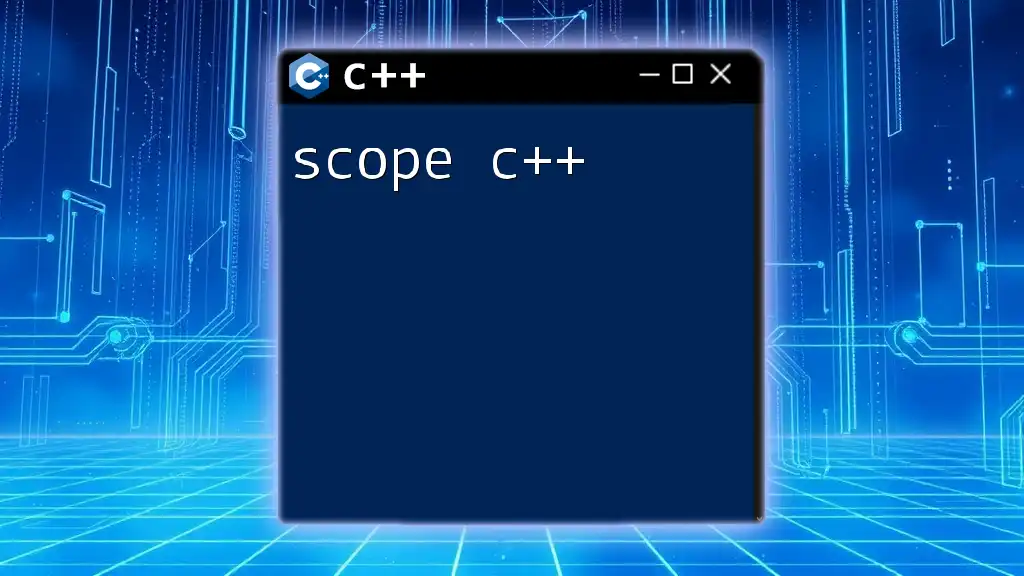
Working with Different Data Types
Copying Arrays
The previous example demonstrated copying from one simple array to another. This method can be applied to arrays of various data types, including integers, doubles, and characters.
Copying Vectors
To illustrate the utility of `std::copy` in more dynamic structures, consider using `std::vector`:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> source = {1, 2, 3, 4, 5};
std::vector<int> dest(source.size());
std::copy(source.begin(), source.end(), dest.begin());
for (const auto &n : dest) {
std::cout << n << ' ';
}
return 0;
}
Copying Custom Objects
`std::copy` is versatile enough to work with user-defined types, allowing for greater flexibility in your applications:
struct Point {
int x, y;
};
int main() {
Point source[] = {{1, 2}, {3, 4}, {5, 6}};
Point dest[3];
std::copy(std::begin(source), std::end(source), std::begin(dest));
// Output interaction for the dest array can be added here
}
Output Interaction
Make sure to test the integrity of copied data by implementing output interactions after copying, as demonstrated in previous examples.
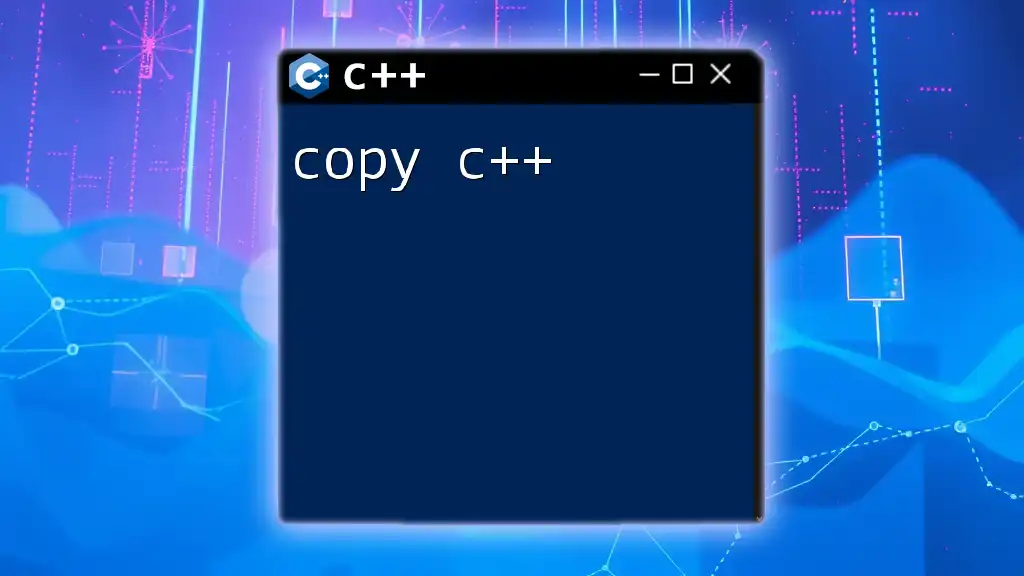
Handling Different Iterators
When using `std::copy`, understanding the types of iterators is crucial:
Input Iterators
These iterators allow read-only access and are important when the source data should not be modified.
Output Iterators
These iterators enable writing to a range and can support operations that modify the destination container.
Random Access Iterators
Random access iterators combine the capabilities of both input and output iterators. They provide more flexibility and can contribute to improved performance for indexed access.
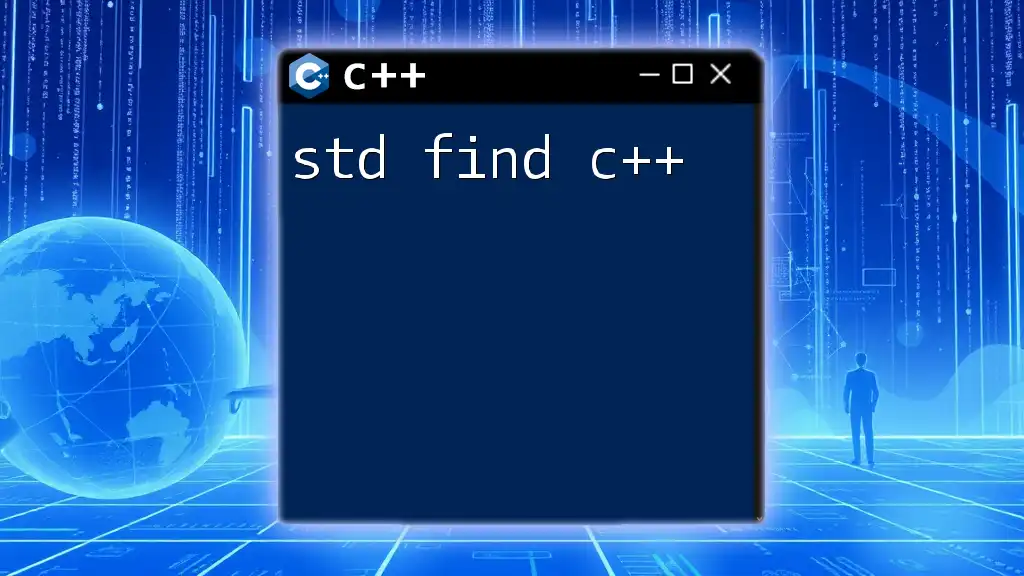
Advanced Usage of std::copy
Copied Elements with Transformations
Combining `std::copy` with `std::transform` can enhance functionality. Here’s an example that shows how to modify elements while copying:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> source = {1, 2, 3, 4, 5};
std::vector<int> dest(source.size());
std::transform(source.begin(), source.end(), dest.begin(), [](int n) { return n * 2; });
for (const auto &n : dest) {
std::cout << n << ' ';
}
return 0;
}
Working with std::back_inserter
`std::back_inserter` can be a handy tool when your destination container does not have predefined space:
#include <vector>
#include <algorithm>
#include <iterator>
std::vector<int> source = {1, 2, 3, 4, 5};
std::vector<int> dest;
std::copy(source.begin(), source.end(), std::back_inserter(dest));
Performance Considerations
When using `std::copy`, be mindful of memory management. It performs a shallow copy, which could lead to issues if your data type involves pointers or dynamic memory. Understanding the difference between deep and shallow copies can be crucial when copying or manipulating complex data types.
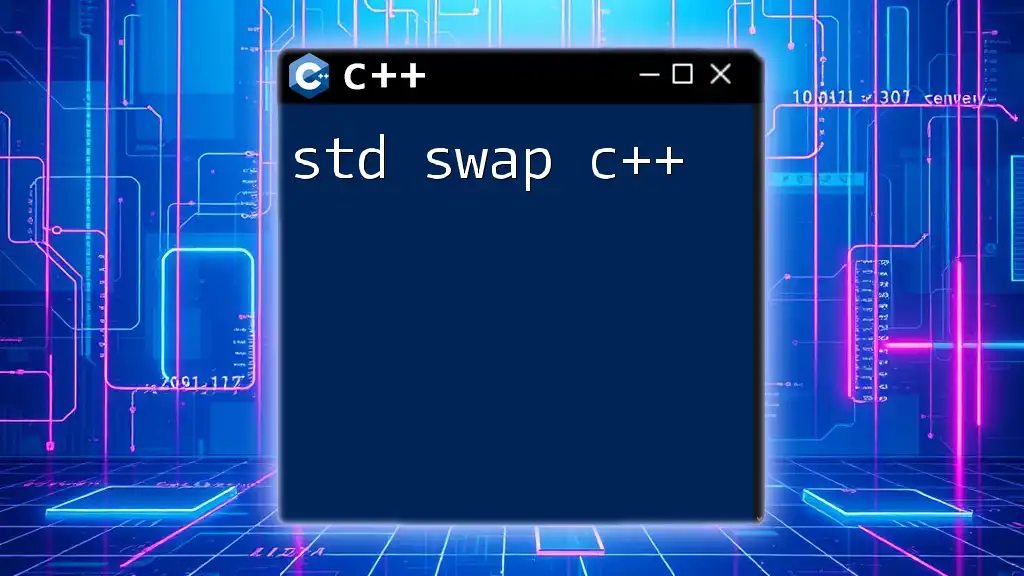
Common Pitfalls
While `std::copy` is straightforward, there are potential pitfalls to consider:
- If the destination is smaller than the source, accessing out-of-bounds memory can lead to undefined behavior.
- Carelessly managing container sizes can result in runtime exceptions.
Example of Incorrect Usage
std::vector<int> source = {1, 2, 3};
std::vector<int> dest;
std::copy(source.begin(), source.end(), dest.begin()); // This will cause an error!
When using `std::copy`, ensure that the destination has been appropriately sized to accommodate the incoming elements.
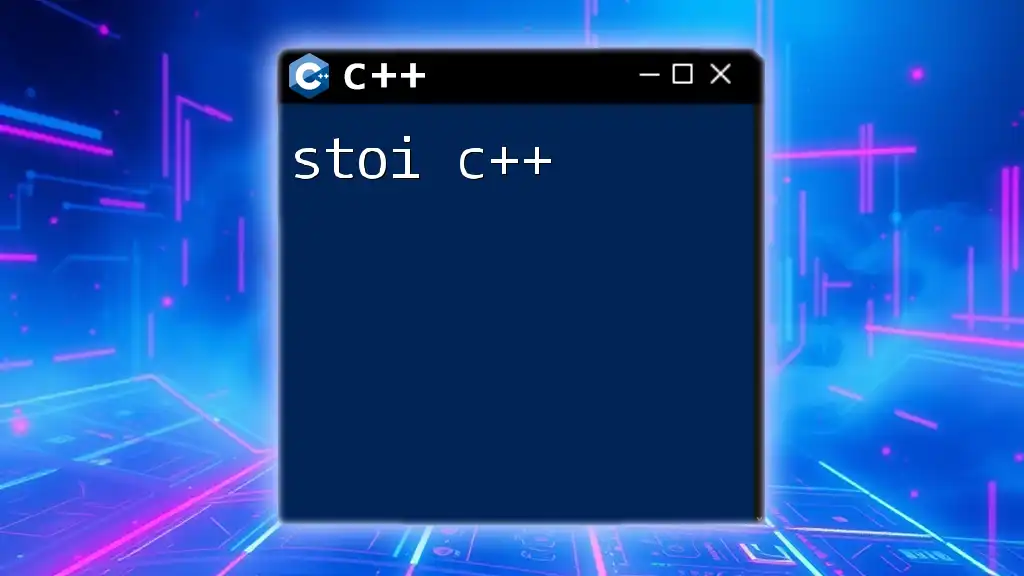
Conclusion
In summary, `std::copy` is an essential function in C++ that allows for effective data management across various types of containers. Its prolific usage can drastically reduce the amount of code needed for copying tasks while enhancing performance and readability. Mastering `std::copy` will serve you well in future C++ projects.
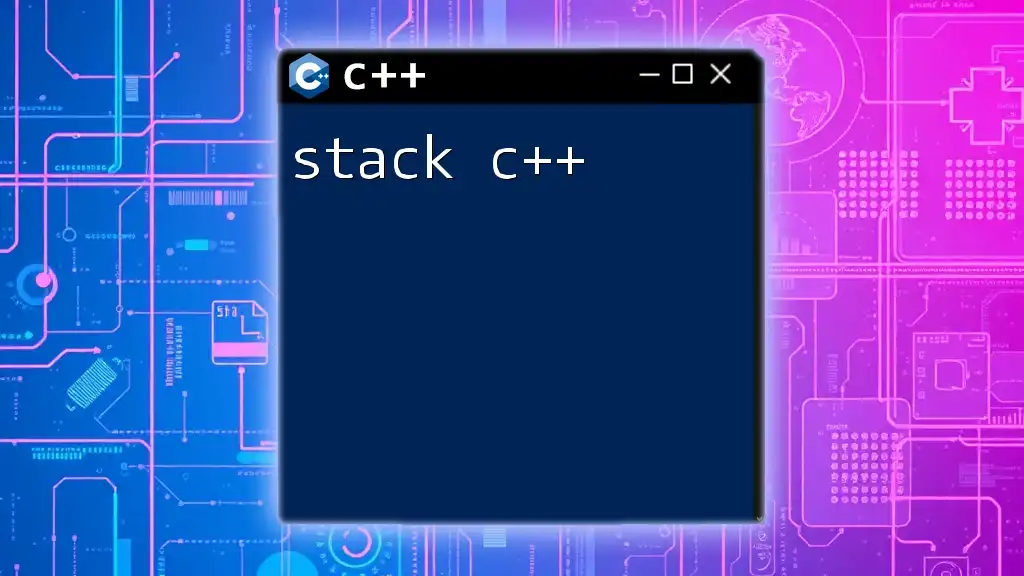
Frequently Asked Questions (FAQs)
What happens if the destination is smaller than the source?
If you attempt to copy more elements than the destination can hold, you risk accessing invalid memory, which leads to undefined behavior. Always ensure your destination container is appropriately sized.
Can I use std::copy with strings?
Absolutely! `std::copy` can be utilized with C++ strings as well, enabling simple and effective copying of characters:
std::string str = "Hello";
std::string copy;
std::copy(str.begin(), str.end(), std::back_inserter(copy));
Is std::copy thread-safe?
`std::copy` itself does not provide thread safety. If you plan to work with multiple threads accessing the same data, it is crucial to implement your synchronization mechanisms to avoid data races and undefined behavior.
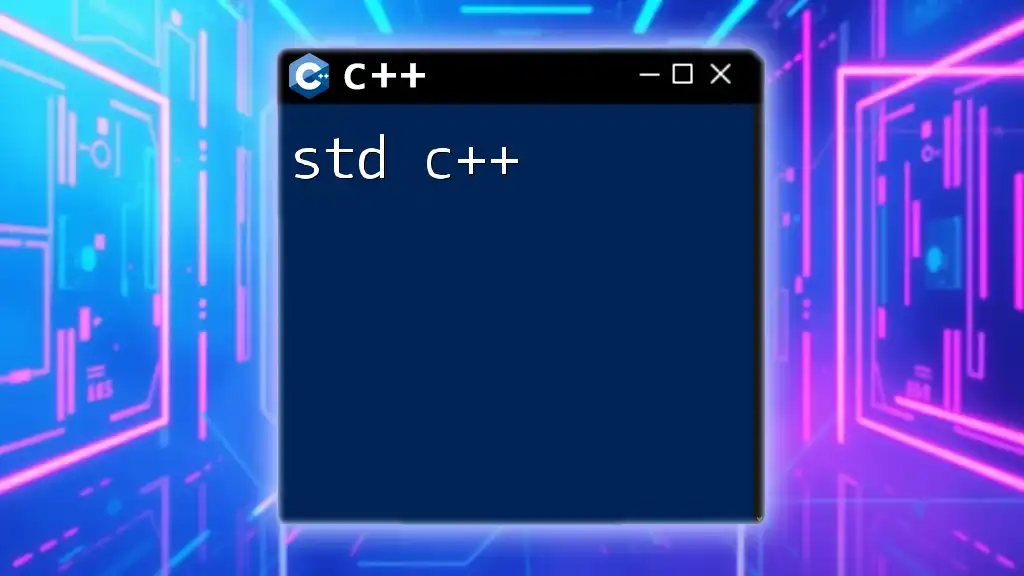
Additional Resources
To further enhance your knowledge of C++, consider exploring the following resources:
- Official C++ documentation on the Standard Template Library (STL)
- Online tutorials and courses that delve deeper into C++ command usage
- Community forums where you can ask questions and share knowledge with fellow C++ enthusiasts
By diving into these resources, you can gain a more profound understanding of how to utilize `std::copy` and other C++ features effectively.