A C++ copy constructor is a special constructor that initializes a new object as a copy of an existing object, ensuring that all data members are properly copied.
class MyClass {
public:
int value;
// Copy constructor
MyClass(const MyClass &obj) {
value = obj.value;
}
};
Understanding Copy Constructors
What is a Copy Constructor?
A C++ copy constructor is a special member function responsible for creating a new object as a copy of an existing object. It plays a crucial role in object-oriented programming, particularly when dealing with classes containing dynamically allocated resources, such as pointers.
Key Characteristics of a copy constructor include:
- It is a member function of the class.
- It takes a reference to a constant object of the same class as a parameter.
- It is invoked automatically when an object is copied, either explicitly or implicitly.
When is a Copy Constructor Invoked?
A copy constructor is automatically called in the following situations:
- When initializing a new object with an existing object.
- When passing objects to functions by value, which necessitates creating a copy of the passed object.
- When a function returns an object by value.
Syntax of a Copy Constructor
The basic syntax of a copy constructor follows this format:
class ClassName {
public:
ClassName(const ClassName &obj);
};
The parameter is a constant reference to another object of the same class, ensuring that the original object remains unchanged during the copying process.

Defining a Copy Constructor
Implementation Steps
Implementing a copy constructor includes understanding the difference between memberwise (shallow) copy and deep copy. The former simply copies the member values, while the latter duplicates the memory allocated for pointers to avoid dangling pointers or unintended memory access.
Example of a Simple Copy Constructor
Here is a straightforward example of a C++ copy constructor:
class MyClass {
private:
int *data;
public:
MyClass(int value) {
data = new int(value);
}
// Copy Constructor
MyClass(const MyClass &obj) {
data = new int(*(obj.data)); // Performs deep copy
}
~MyClass() {
delete data; // Destructor for cleanup
}
};
In this code, the copy constructor ensures that a new integer is allocated for each copy of `MyClass`, preventing issues related to shared access of dynamically allocated memory.
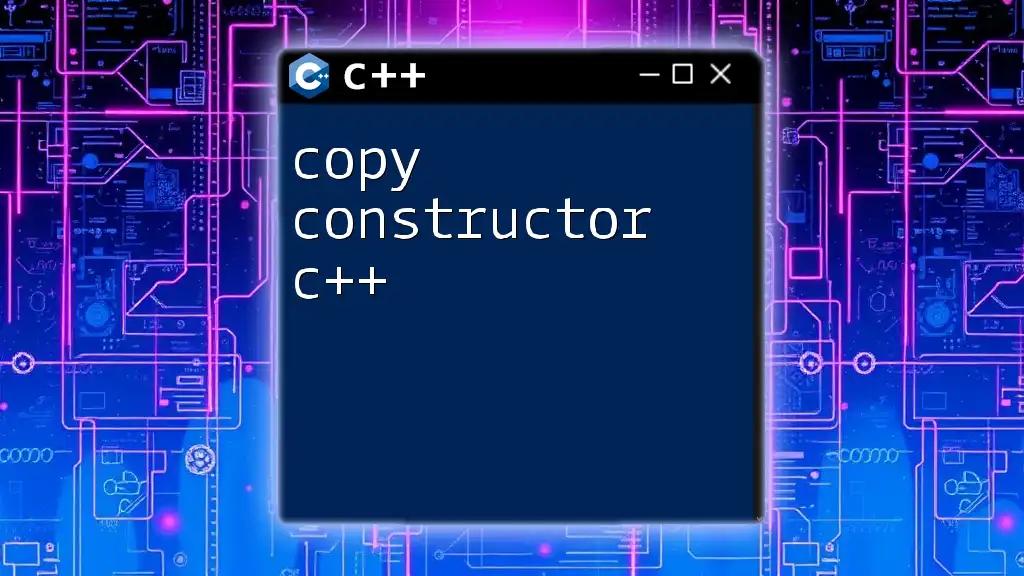
Comparing Copy Constructor and Default Copying Behavior
Default Copy Behavior
If a copy constructor is not explicitly defined, the compiler generates a default copy constructor that performs a memberwise copy. This can lead to issues with resource management, especially when a class contains pointers.
Shallow Copy vs. Deep Copy
Shallow Copy occurs when the default copy behavior is used. In this case, both the original and copied object share the same memory addresses for pointer members, which can lead to problems such as double deletions when both objects are destructed.
Deep Copy, on the other hand, entails creating an independent copy of the dynamically allocated memory. This is vital for safe memory management and avoiding unintended side effects, especially in classes that manage resources directly.
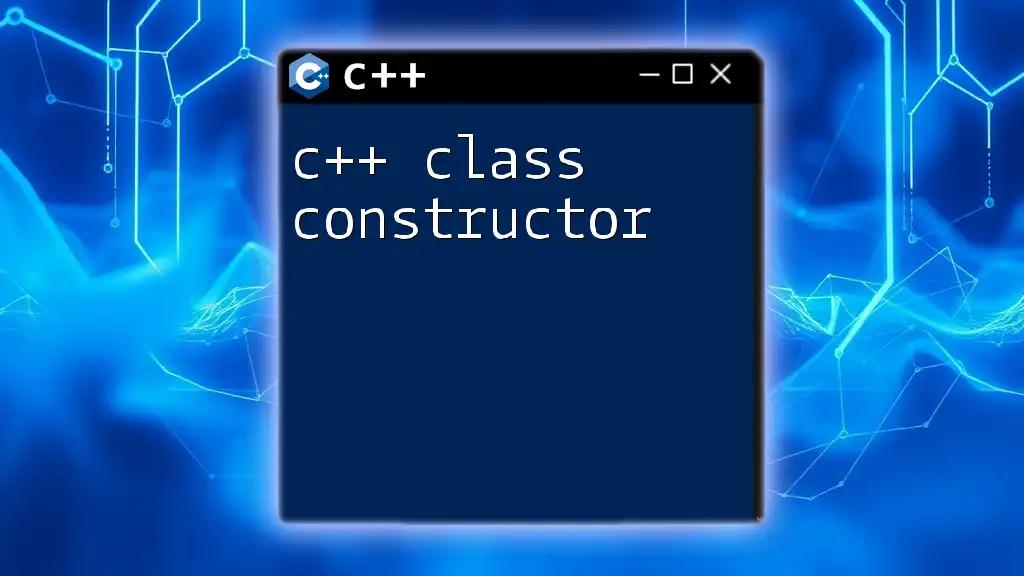
Best Practices for Implementing Copy Constructors
When to Implement Your Own Copy Constructor
You should implement a custom copy constructor when:
- Your class includes dynamically allocated memory.
- You need to manage complex resources that require unique ownership semantics.
- You want to prevent potential issues caused by the compiler-generated shallow copy.
Common Pitfalls to Avoid
Be mindful of common mistakes when writing copy constructors:
- Neglecting to copy dynamically allocated memory: Always ensure you allocate new memory for the copied object to avoid both objects pointing to the same memory location.
- Forgetting to declare a destructor: If your class allocates resources, implementing a destructor is essential to avoid memory leaks or undefined behavior when objects go out of scope.
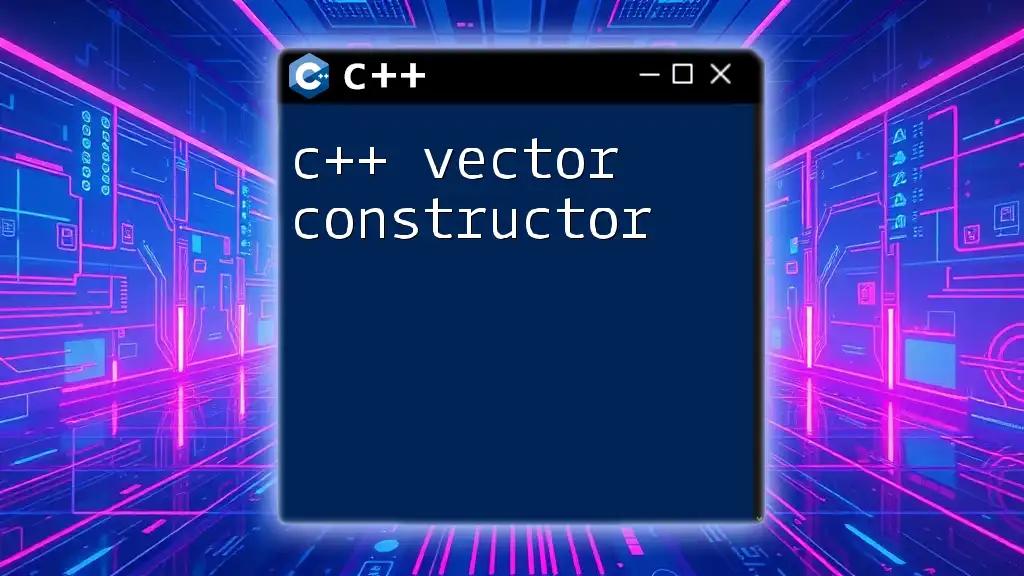
Copy Constructor in Practice
Real-World Example
Consider the following `Resource` class that demonstrates the proper implementation of a copy constructor:
class Resource {
private:
int size;
int *data;
public:
Resource(int s) : size(s) {
data = new int[size];
}
// Copy Constructor
Resource(const Resource &res) {
size = res.size;
data = new int[size];
for (int i = 0; i < size; i++) {
data[i] = res.data[i]; // Deep copy of the data
}
}
~Resource() {
delete[] data; // Cleanup memory
}
// Additional methods...
};
Utilizing the Copy Constructor in Code
The following example demonstrates creating an object and leveraging the copy constructor:
int main() {
Resource res1(5); // Creates an object with size 5
Resource res2 = res1; // Invokes copy constructor to create a copy
}
Here, `res2` is initialized as a copy of `res1`. The copy constructor ensures that `res2` has its own distinct copy of the data, safeguarding against unintended memory corruption or leaks.

Alternatives to Copy Constructors
Move Semantics
Introduced in C++11, move semantics offer an efficient alternative to using copy constructors. A move constructor transfers ownership of resources, meaning that it does not create a copy but rather reassigns pointers or resources from one object to another.
A brief example of a move constructor might look like this:
class MyClass {
public:
MyClass(MyClass &&obj) noexcept {
// Move resources from obj to *this
}
};
Using move constructors can significantly improve performance by minimizing unnecessary copying.

Conclusion
Understanding the C++ copy constructor is essential for effective memory management in C++. By defining your copy constructors when needed, recognizing the differences between shallow and deep copies, and avoiding common pitfalls, you can ensure robust and efficient code.
Embracing best practices will not only lead to better resource management but also enhance your coding skills in C++. Start experimenting with different implementations of copy constructors to deepen your understanding, and consider sharing your experiences or questions for further discussion.