A deep copy copy constructor in C++ creates a new object as a copy of an existing object, ensuring that all dynamic memory is duplicated rather than merely copying pointer references.
#include <iostream>
#include <cstring>
class DeepCopyExample {
private:
char* data;
public:
// Constructor
DeepCopyExample(const char* value) {
data = new char[strlen(value) + 1];
strcpy(data, value);
}
// Deep copy copy constructor
DeepCopyExample(const DeepCopyExample& other) {
data = new char[strlen(other.data) + 1];
strcpy(data, other.data);
}
// Destructor
~DeepCopyExample() {
delete[] data;
}
void display() const {
std::cout << data << std::endl;
}
};
int main() {
DeepCopyExample original("Hello, World!");
DeepCopyExample copy = original; // Calls deep copy copy constructor
copy.display();
return 0;
}
Understanding Object Copying in C++
In C++, copying objects involves duplicating their data members. This can be accomplished through either a shallow copy or a deep copy.
-
Shallow copy duplicates only the values of the data members, which can lead to issues when the data members themselves are pointers pointing to dynamically allocated memory. When a shallow copy is made, both source and copied objects point to the same memory location, which can result in accidental modifications or double deletions.
-
Deep copy, on the other hand, creates a new instance of dynamically allocated memory for the copied object, ensuring that each object manages its own memory independently. This is crucial for maintaining data integrity and avoiding memory leaks.
Understanding the distinction is vital for effective memory management in C++ and for implementing a deep copy copy constructor correctly.
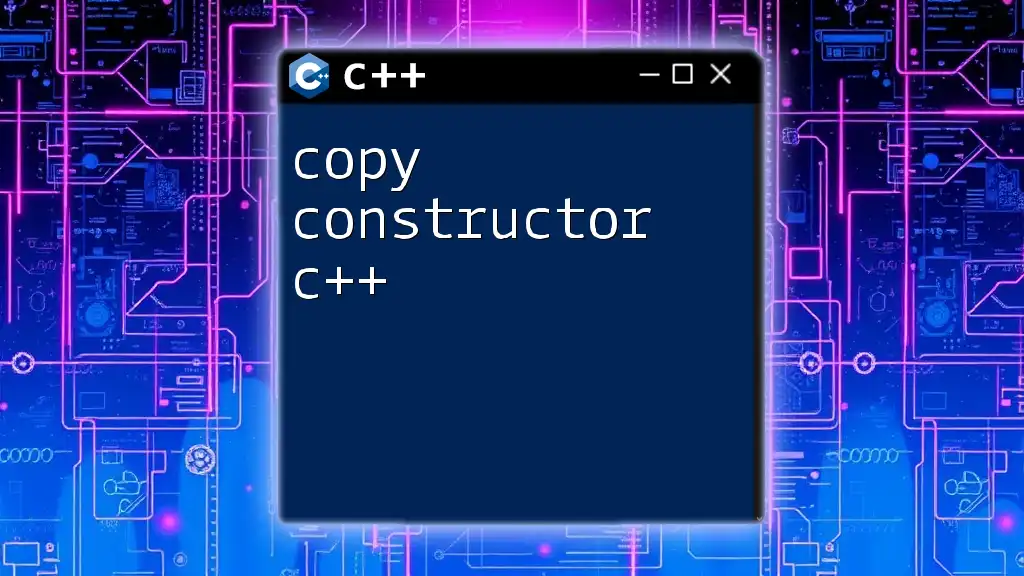
What is a Copy Constructor?
Definition and Purpose of Copy Constructors
A copy constructor is a special constructor in C++ that initializes a new object as a copy of an existing object. This is essential for classes that manage resource ownership, particularly when dealing with dynamic memory.
Syntax of a Copy Constructor
The basic syntax for defining a copy constructor is as follows:
ClassName(const ClassName& other);
The parameter is a reference to another object of the same class, and the constructor typically handles the allocation and copying of resources.
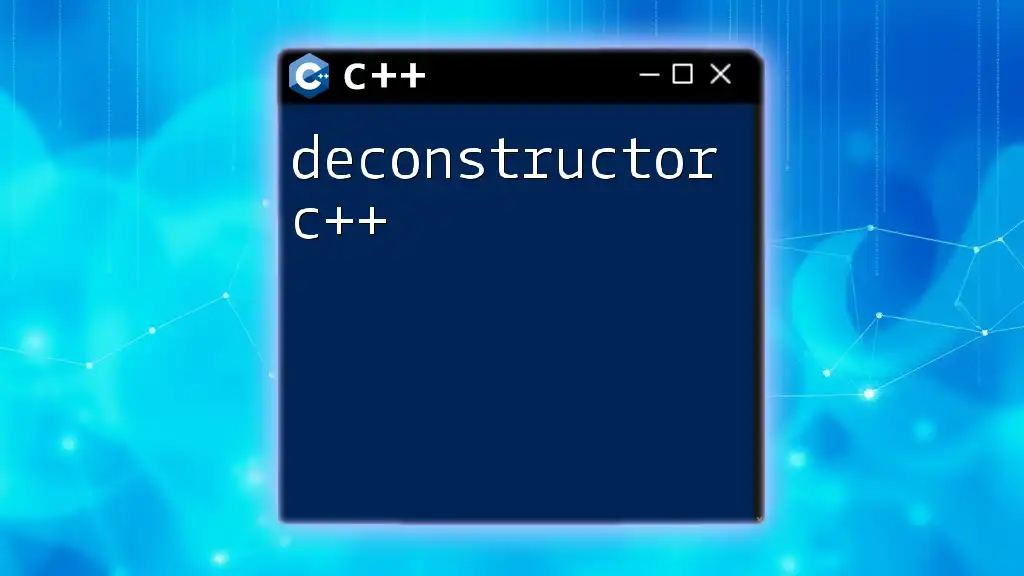
Shallow Copy vs. Deep Copy
Defining Shallow Copy
Shallow copying can be illustrated through an example:
class ShallowCopyExample {
public:
int* data;
ShallowCopyExample(int value) {
data = new int(value);
}
// Shallow copy constructor
ShallowCopyExample(const ShallowCopyExample& other) {
data = other.data; // both objects point to same memory
}
~ShallowCopyExample() {
delete data; // issue: double deletion if both are destructed
}
};
In this case, if two objects of `ShallowCopyExample` are created, deleting one can lead to an attempt to delete memory that has already been released when the other is destructed.
Understanding Deep Copy
Deep copying, in contrast, ensures that a new copy of the dynamically allocated memory is created. This is generally achieved through a user-defined copy constructor.
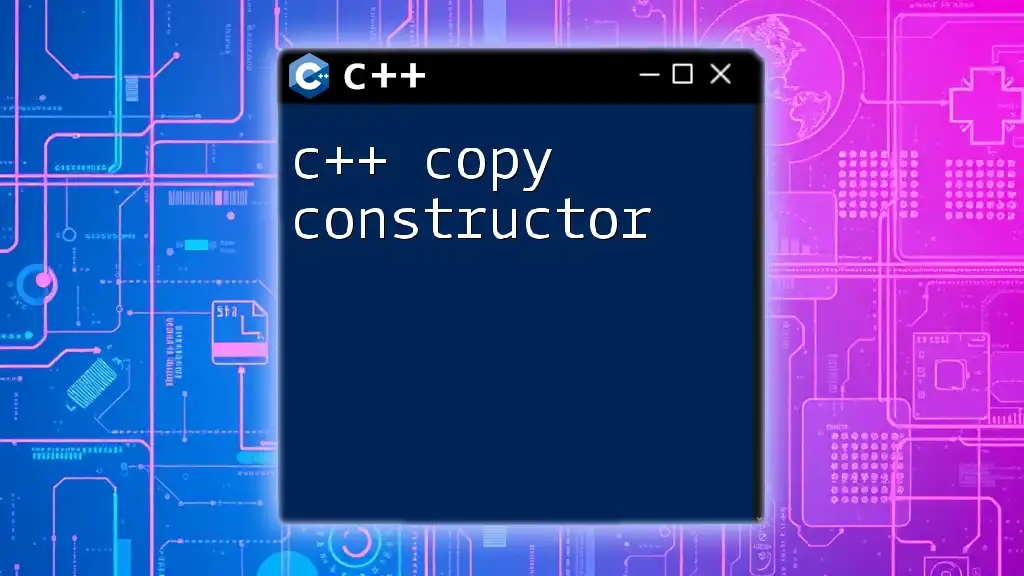
Implementing a Deep Copy Constructor in C++
Step-by-Step Guide to Creating a Deep Copy Constructor
To implement a deep copy constructor, follow these steps:
- Identify Dynamic Resources: Review the data members of the class and identify which ones point to dynamically allocated memory.
- Write the Copy Constructor: Ensure that during the copy process, new memory is allocated for these members.
- Code Example: Here’s how a deep copy constructor might look:
class DeepCopyExample {
public:
int* data;
DeepCopyExample(int value) {
data = new int(value);
}
// Deep copy constructor
DeepCopyExample(const DeepCopyExample& other) {
data = new int(*other.data); // allocate new memory and copy the value
}
~DeepCopyExample() {
delete data; // prevent memory leaks
}
};
In this implementation, the `DeepCopyExample` class creates a new integer in memory whenever a copy of the object is requested, safeguarding against unintended modifications.
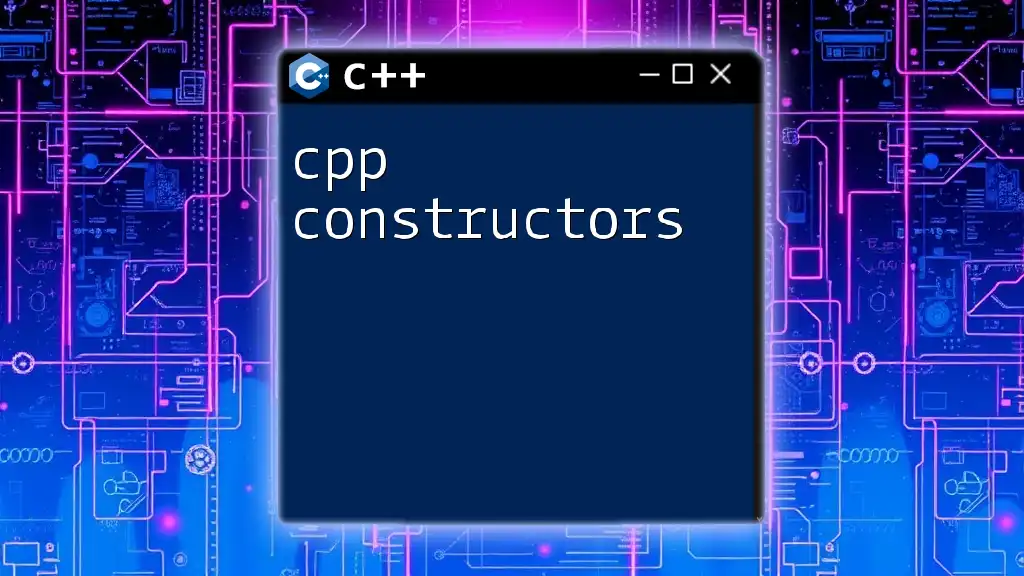
Practical Example of Deep Copy
Creating a Simple Class with Dynamic Allocation
Let’s explore a concrete example with a simple class consisting of dynamic allocation:
class MyClass {
public:
int* value;
MyClass(int val) {
value = new int(val);
}
// Deep copy constructor
MyClass(const MyClass& other) {
value = new int(*other.value); // deep copy
}
// Destructor
~MyClass() {
delete value; // clean up memory
}
};
// Usage example
int main() {
MyClass obj1(10); // Create obj1
MyClass obj2 = obj1; // Use copy constructor
*obj2.value = 20; // Change obj2's value
// obj1.value remains 10
}
In this example, we see that modifying `obj2`'s `value` does not impact `obj1`, demonstrating the effectiveness of deep copying.
Testing the Deep Copy Functionality
When creating objects, ensure to verify that the deep copy functionality is intact. This can be observed through practical use cases, such as monitoring values before and after modifications.
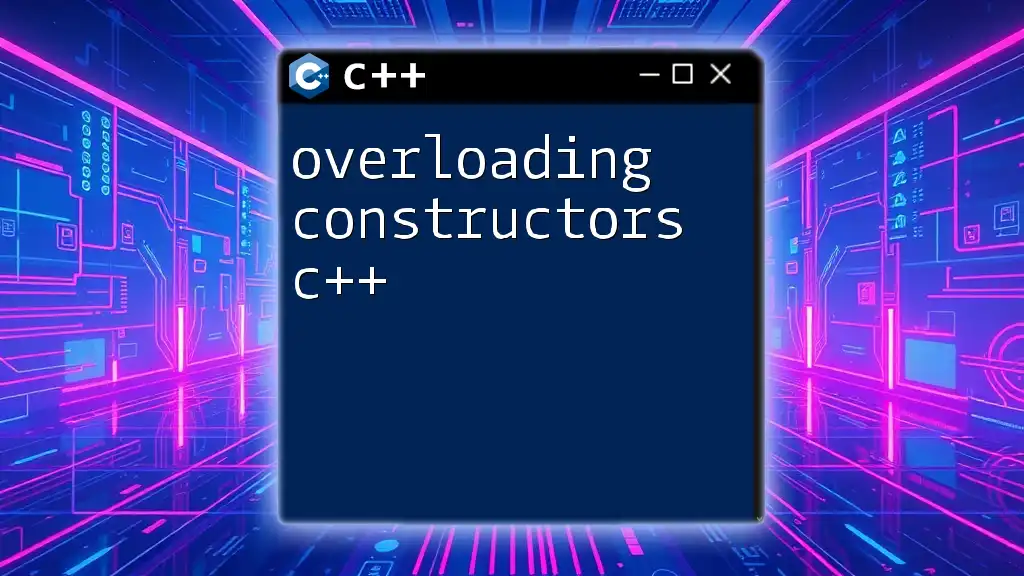
Potential Pitfalls and Best Practices
Common Mistakes When Implementing Deep Copy
- Memory Leaks: Failing to delete previously allocated memory in the destructor can lead to memory leaks.
- Double Deletion: Attempting to delete the same memory more than once can cause program crashes.
Best Practices for Writing a Deep Copy Constructor
To ensure effective memory management:
- Always allocate new memory before copying: This principle prevents issues related to shared memory.
- Implement the Rule of Three: If you define a destructor, copy constructor, or copy assignment operator, you should define all three to manage dynamic resources safely.
Consider employing smart pointers, such as `std::unique_ptr` or `std::shared_ptr`, to handle memory automatically in modern C++. This reduces the complexity associated with manual memory management.
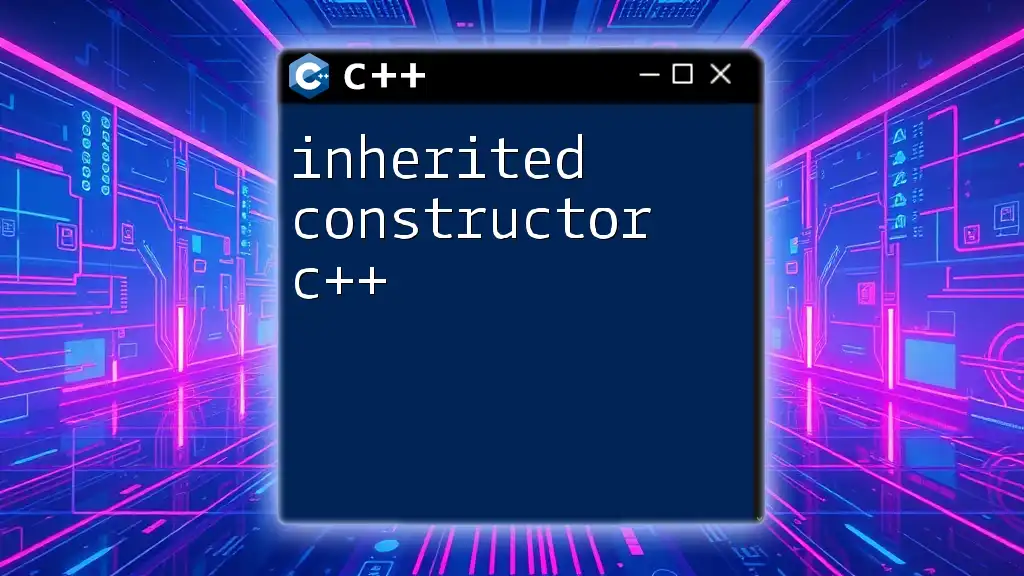
Conclusion
In conclusion, understanding the deep copy copy constructor in C++ is crucial for managing resources effectively. By mastering the implementation of deep copy constructors, developers can prevent potential pitfalls, such as memory leaks and double deletions. This knowledge empowers you to create robust applications that handle dynamic memory safely and efficiently.
Experimenting with deep copy constructors in your own projects is encouraged, as practical application reinforces understanding. For further exploration, consider diving deeper into advanced memory management techniques in C++.