In C++, you can prevent the use of the copy constructor by declaring it as private in your class definition, which prevents any object from being copied.
class NoCopy {
private:
NoCopy(const NoCopy&); // Private copy constructor
public:
NoCopy() {}
};
Understanding the Copy Constructor
What is a Copy Constructor?
A copy constructor is a special constructor in C++ used to create a new object as a copy of an existing object. Its primary purpose is to initialize an object with the values from another object of the same class. By default, the compiler provides a copy constructor, which performs a shallow copy, meaning it copies the values of the member variables directly. This can lead to problems, especially when managing resources like dynamic memory or file handles.
Here’s a simple example showcasing the default copy constructor:
class Sample {
public:
int x;
Sample(int val) : x(val) {}
};
Sample original(5);
Sample copy = original; // Calls the default copy constructor
Copy Constructor vs. Move Constructor
With the introduction of C++11, move semantics became available, allowing for more efficient transfer of resources. A move constructor enables the transfer of resources from one object to another without copying the underlying data, thus preventing resource duplication.
Understanding the distinction between copy constructors and move constructors is essential in modern C++ programming. While a copy constructor may lead to deep copying and potential inefficiencies, a move constructor, by contrast, can improve performance drastically, especially for containers or classes managing dynamic memory.
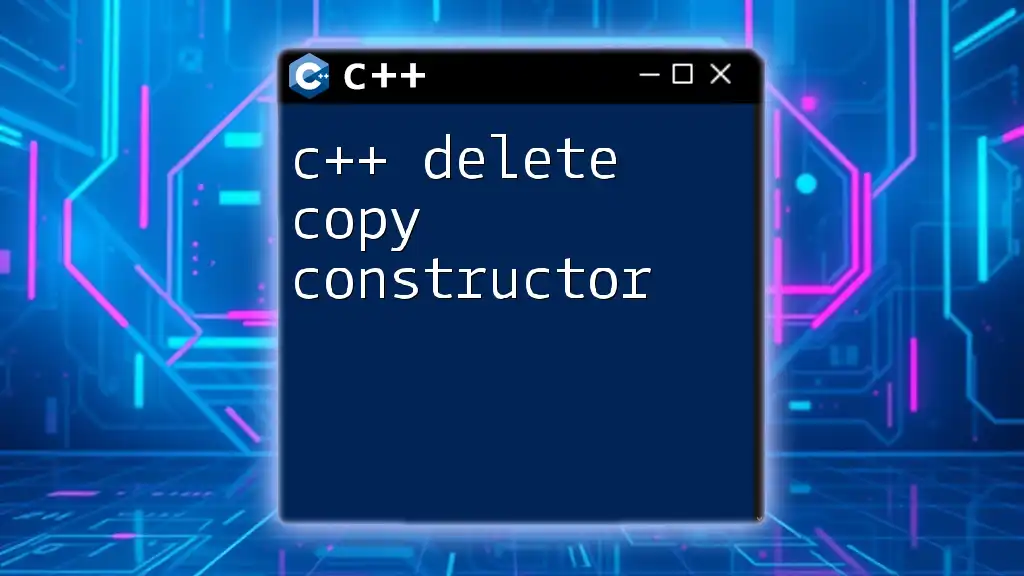
Preventing Copy Construction
Why Prevent Copy Construction?
In certain scenarios, copying objects can lead to significant issues. For instance, if a class manages a resource such as memory that should have a single owner, allowing copy operations can cause multiple instances to manage the same resource. This often results in dangling pointers and double-free errors during deallocation.
By preventing copy construction, you ensure that your class remains robust and behaves predictably, particularly in complex or resource-intensive applications.
Implementing Deleted Copy Constructor
One straightforward method to prevent copy construction in a class is to delete its copy constructor. This approach makes it clear to users of your class that they cannot create copies.
class NonCopyable {
public:
NonCopyable() = default;
NonCopyable(const NonCopyable&) = delete; // Prevent copy construction
NonCopyable& operator=(const NonCopyable&) = delete; // Prevent assignment
};
With the `= delete` syntax, attempts to use the copy constructor or copy assignment operator will generate a compilation error, effectively communicating the intent that objects of this class should not be copied.
Using Private Copy Constructor
Another technique is to declare the copy constructor as private. This will restrict access and prevent users of the class from copying it.
class PrivateCopy {
private:
PrivateCopy(const PrivateCopy&); // Declared but not defined
};
By making the copy constructor private without providing a definition, you effectively disable copy construction while still allowing other member functions and friends to have access if needed.
Combining with `std::unique_ptr`
Using smart pointers, particularly `std::unique_ptr`, inherently prevents copy construction. A `std::unique_ptr` is designed to maintain exclusive ownership of the object it points to, preventing copying.
#include <memory>
class ResourceHandler {
public:
ResourceHandler() : resource(std::make_unique<int>(42)) {}
ResourceHandler(const ResourceHandler&) = delete; // Prevent copy
ResourceHandler& operator=(const ResourceHandler&) = delete; // Prevent assignment
private:
std::unique_ptr<int> resource;
};
In this example, a `ResourceHandler` class uses `std::unique_ptr` to manage the lifetime of a dynamically allocated integer. Since `std::unique_ptr` cannot be copied (only moved), copying `ResourceHandler` becomes impossible.
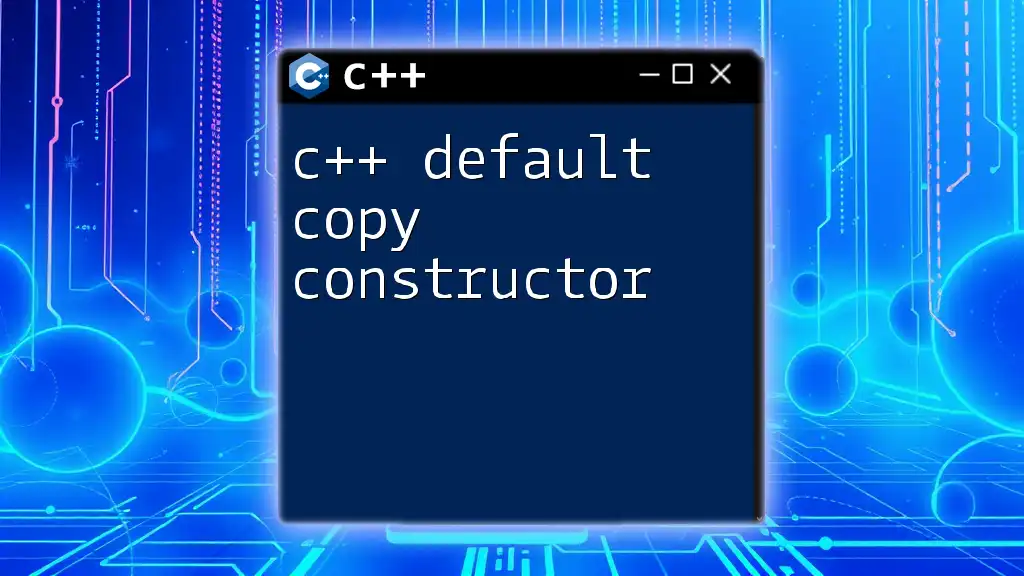
Alternative Approaches
Using Move Semantics
In cases where you still want to allow transferring of resources but want to prevent copying, implementing move semantics is an effective strategy.
When you create a move constructor, you permit the transfer of resources from one instance to another while leaving the moved-from object in a valid state.
class Movable {
public:
Movable() : data(new int[10]) {}
Movable(Movable&& other) noexcept : data(other.data) {
other.data = nullptr; // Nullify the source
}
Movable& operator=(Movable&& other) noexcept {
if (this != &other) {
delete[] data; // Clean up current resource
data = other.data; // Move the resource
other.data = nullptr; // Nullify the source
}
return *this;
}
private:
int* data;
};
In this example, the `Movable` class supports move operations while preventing copies, allowing for efficient resource management without duplication.
Utilizing Copy-and-Swap Idiom
The Copy-and-Swap idiom is another advanced method that enables copy-like behavior while preventing direct copying of resources.
class CopyAndSwap {
public:
CopyAndSwap() {}
CopyAndSwap(const CopyAndSwap&) = delete; // Disable copy
CopyAndSwap& operator=(CopyAndSwap other) { // Copy-and-swap
swap(*this, other);
return *this;
}
friend void swap(CopyAndSwap& first, CopyAndSwap& second) noexcept {
std::swap(first.data, second.data); // Swap contents
}
private:
int* data;
};
In this implementation, the `operator=` function takes its parameter by value, which requires a copy. However, since copying has been disabled, the only way to assign is through the swap, allowing for much safer handling of resource management.
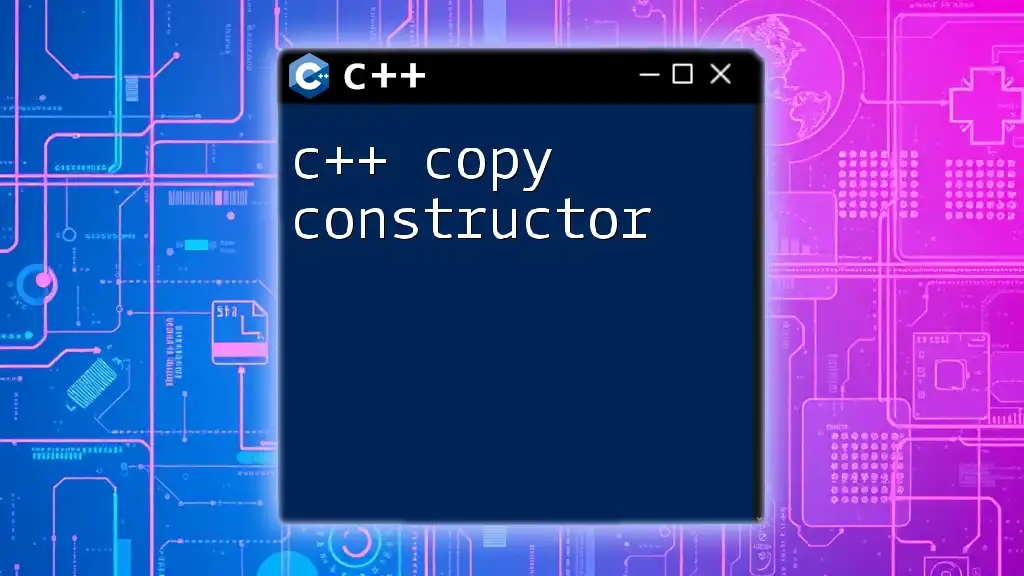
Conclusion
In C++, understanding how to effectively prevent copy construction is crucial to building robust and maintainable software. By employing techniques such as deleting the copy constructor, making it private, or utilizing smart pointers, developers can ensure that their classes manage resources correctly without the risk of unintended copies.
Implementing move semantics and utilizing idioms like Copy-and-Swap can further enhance performance and safety by allowing efficient handling of resources without the drawbacks of traditional copying. Always keep in mind best practices when designing classes, and embrace C++’s features to write clean and efficient code.