In C++, a struct constructor is a special member function that initializes an object's attributes when it is created, as demonstrated in the following example:
#include <iostream>
using namespace std;
struct Point {
int x, y;
// Constructor
Point(int xCoord, int yCoord) : x(xCoord), y(yCoord) {}
};
int main() {
Point p(5, 10); // Creating an object of Point using the constructor
cout << "Point coordinates: (" << p.x << ", " << p.y << ")" << endl;
return 0;
}
What is a Struct in C++?
A struct in C++ is a user-defined data type that allows grouping variables of different types into a single unit. Unlike classes, which can have private and public access specifiers, structs default to public access, making them simple and easy to use for data aggregation.
Structs are particularly useful when you want to represent a composite data structure with multiple attributes.
Example of a Simple Struct
Here's a simple example to illustrate how to define a struct to represent a person:
struct Person {
std::string name;
int age;
};
In this example, the `Person` struct contains two members: a `std::string` for the name and an `int` for the age.
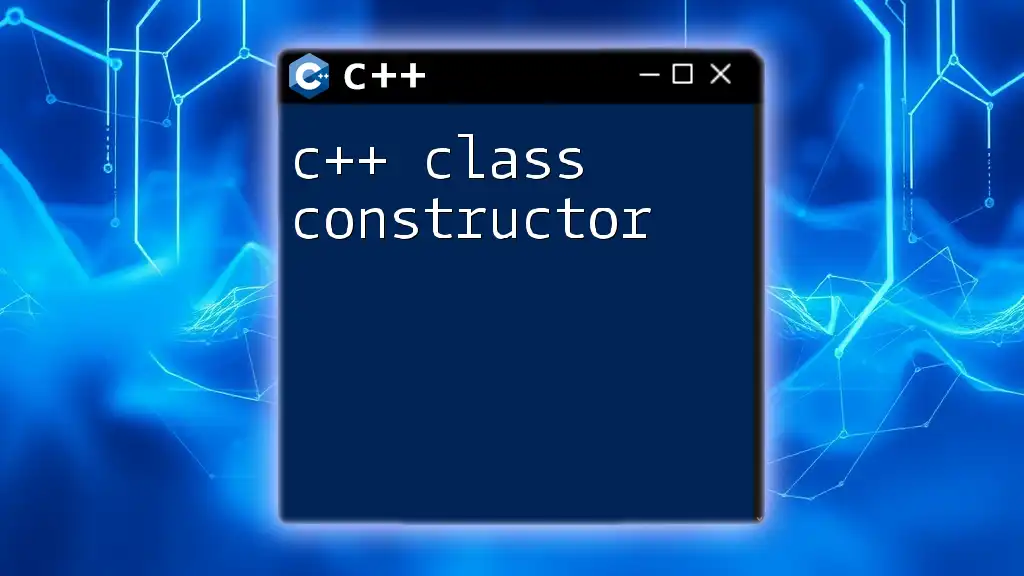
Understanding Constructors
A constructor in C++ is a special member function that is automatically called when an object of a class or struct is created. The primary role of constructors is to initialize the object's member variables.
Constructors come in various types:
- Implicit Constructors: Automatically generated by the compiler if no user-defined constructor is provided.
- Explicit Constructors: Defined by the programmer, including default, parameterized, and copy constructors.
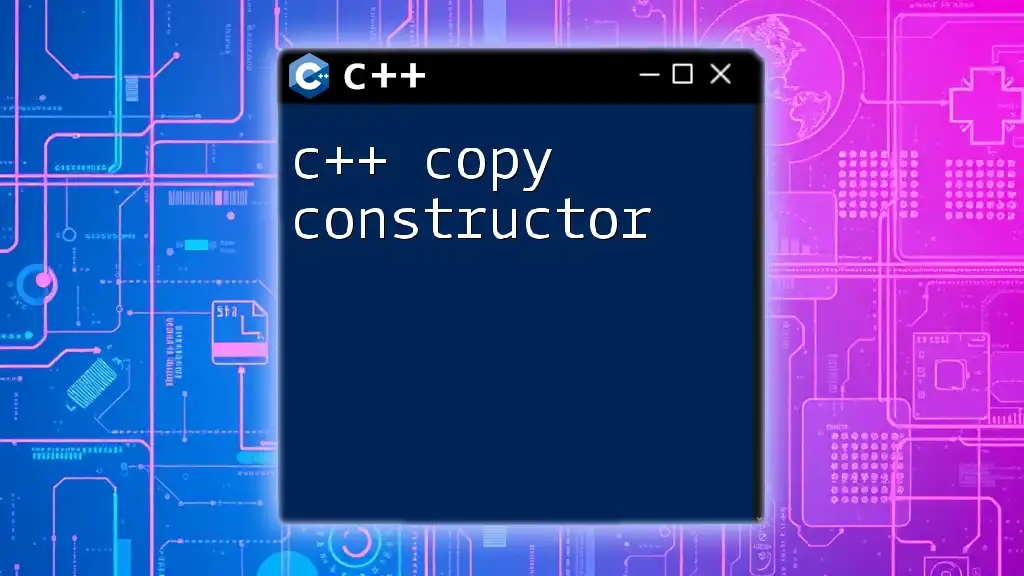
Types of Constructors for Structs
Default Constructor
A default constructor does not take any parameters. It is used to initialize objects with default values. When no constructor is explicitly defined, the compiler provides an implicit default constructor.
Example of a Default Constructor
The following example demonstrates how to define a default constructor within a `Person` struct:
struct Person {
std::string name;
int age;
Person() {
name = "Unnamed";
age = 0;
}
};
In this example, creating a `Person` object with this constructor will initialize the `name` to "Unnamed" and `age` to 0.
Parameterized Constructor
A parameterized constructor allows you to initialize member variables with specific values when the object is created. This type of constructor is more flexible than the default constructor.
Example of a Parameterized Constructor
Here’s how you implement a parameterized constructor in the `Person` struct:
struct Person {
std::string name;
int age;
Person(std::string n, int a) {
name = n;
age = a;
}
};
This constructor allows the user to specify the `name` and `age` of a `Person` object at the time of creation:
Person person2("Alice", 30); // Creates a Person named Alice who is 30 years old.
Copy Constructor
A copy constructor initializes a new object as a copy of an existing object. It is crucial for proper copying and memory management, especially when your struct contains pointers or dynamic memory.
Example of a Copy Constructor
Here is an example of adding a copy constructor to the `Person` struct:
struct Person {
std::string name;
int age;
Person(const Person &p) {
name = p.name;
age = p.age;
}
};
In this case, creating a new `Person` object from an existing one utilizes the copy constructor:
Person person3 = person2; // person3 is a copy of person2.
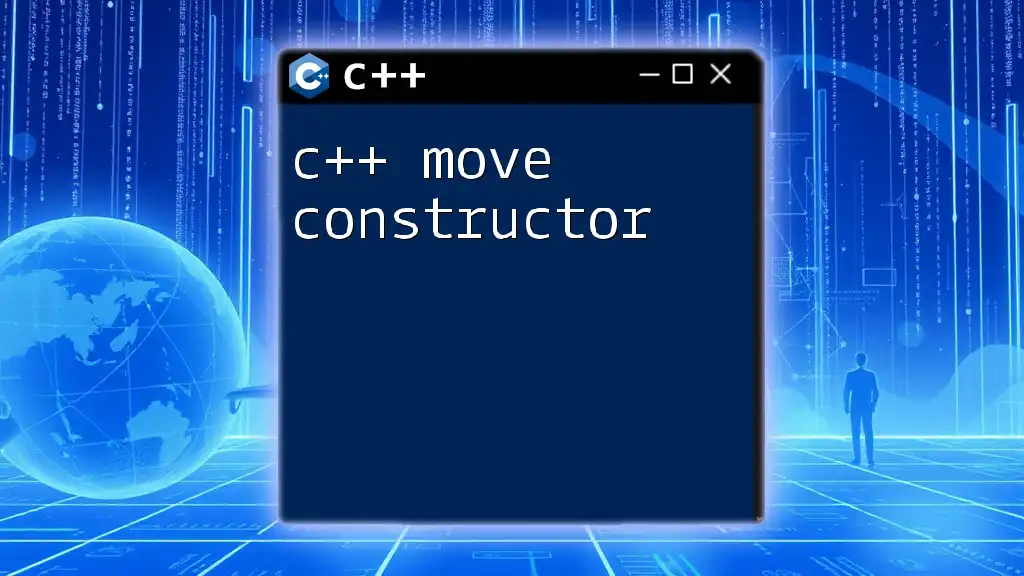
Initializing Structs Using Constructors
When it comes to initialization, constructors simplify the process.
Using Default Constructor
You can create a `Person` object using the default constructor like this:
Person person1; // Invokes the default constructor
`person1` will have default values assigned by the default constructor.
Using Parameterized Constructor
To create a `Person` object with specific values, use the parameterized constructor:
Person person2("Alice", 30); // Invokes the parameterized constructor
Here, `person2` is instantiated with a name and an age.
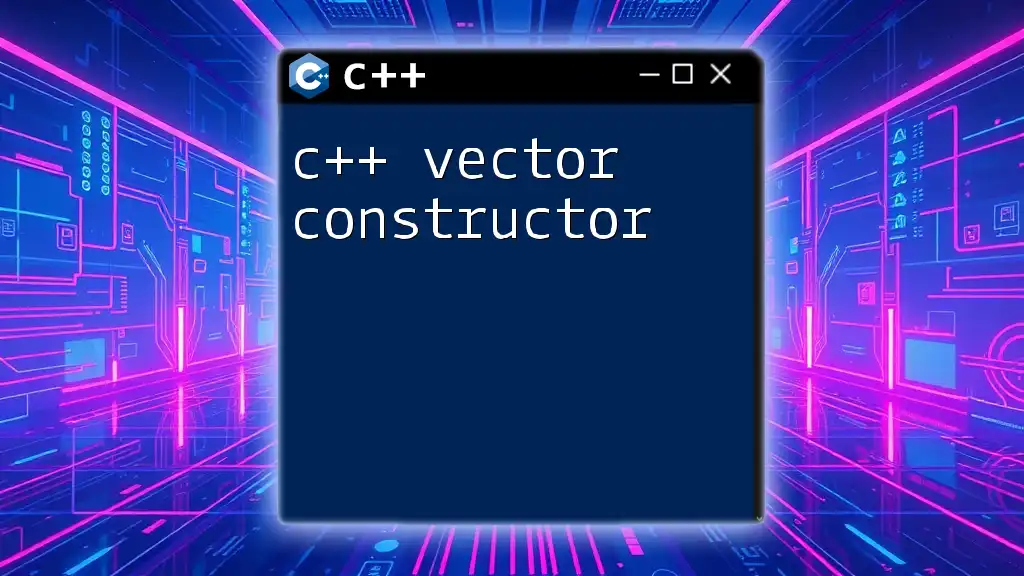
Destructors in Structs
Every struct can have a destructor, which is a member function invoked when an object goes out of scope. Destructors are essential for cleanup, especially if your struct allocates dynamic memory.
Example of a Destructor
Here’s an example of a destructor in a `Person` struct:
struct Person {
std::string name;
int age;
~Person() {
// Cleanup resources if necessary
// Usually empty for struct unless managing dynamic resources
}
};
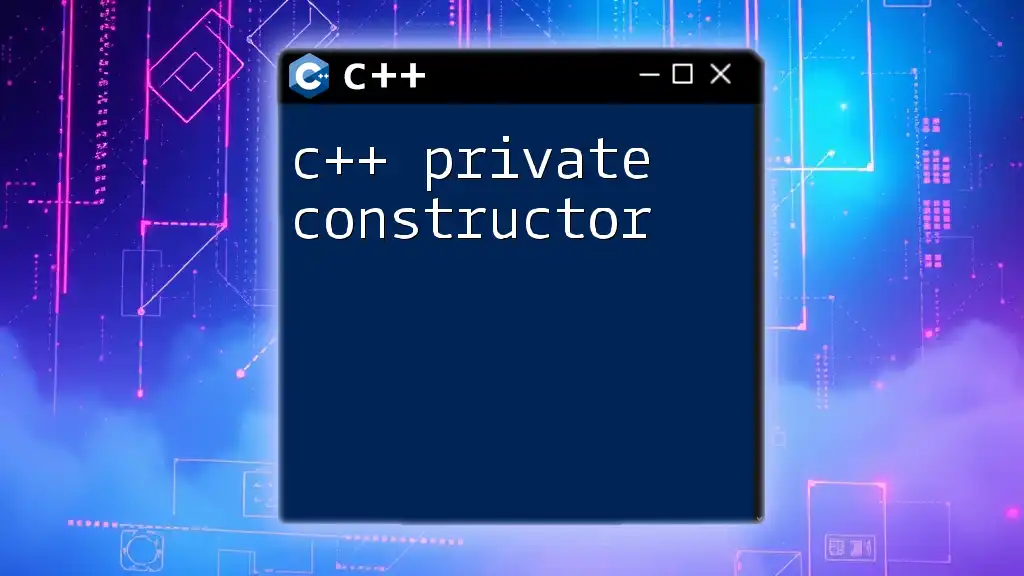
Best Practices for Using Struct Constructors
When working with struct constructors, initialization often proves more effective than assignment. Using initializer lists is highly encouraged for better performance and readability.
Example of an Initializer List
The following demonstrates how to use an initializer list within a constructor:
struct Person {
std::string name;
int age;
Person(std::string n, int a) : name(n), age(a) {}
};
This method initializes the members directly, making the code more efficient.
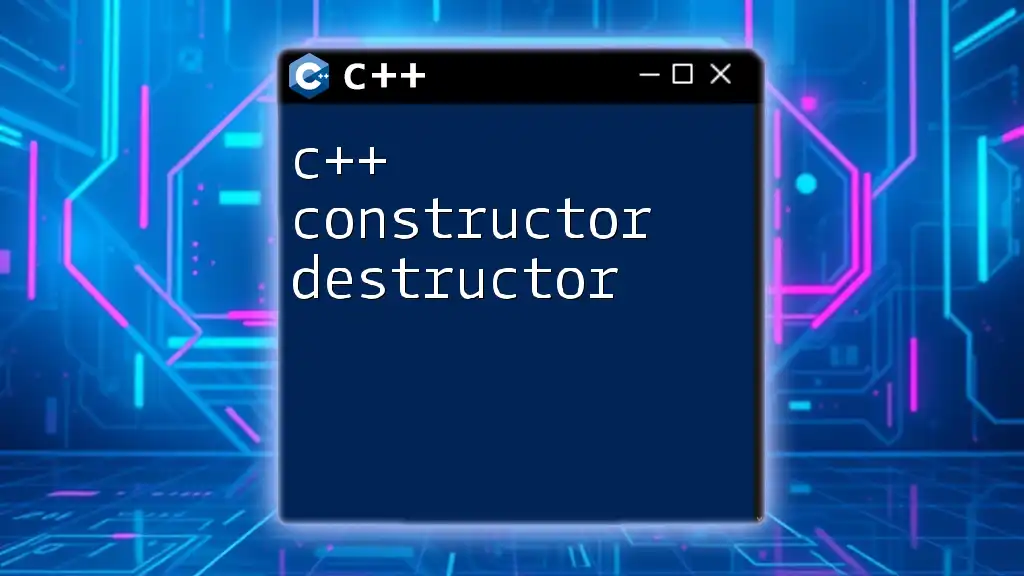
Common Pitfalls and Troubleshooting
When using C++ struct constructors, avoid common pitfalls such as forgetting to define constructors when dealing with dynamic resources or omitting to initialize member variables, which can lead to undefined behaviors or memory leaks. Always ensure that when copying structs, you manage resource ownership properly to prevent issues like double deletion.
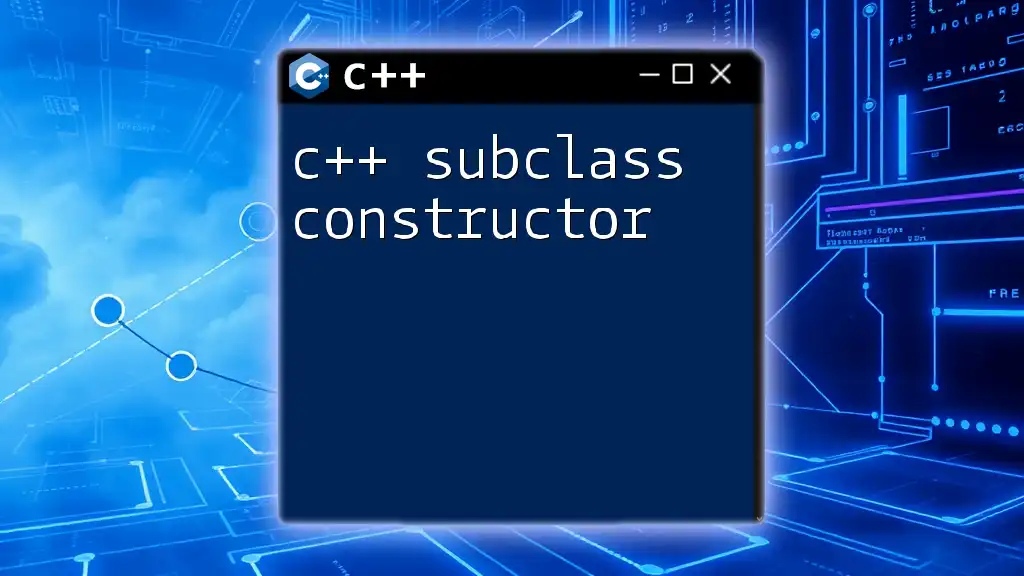
Conclusion
Understanding C++ struct constructors is crucial for effective programming in C++. They enable you to initialize objects easily, leading to cleaner and more maintainable code. Exploring constructors further will provide you with a solid foundation for managing data structures in your applications.
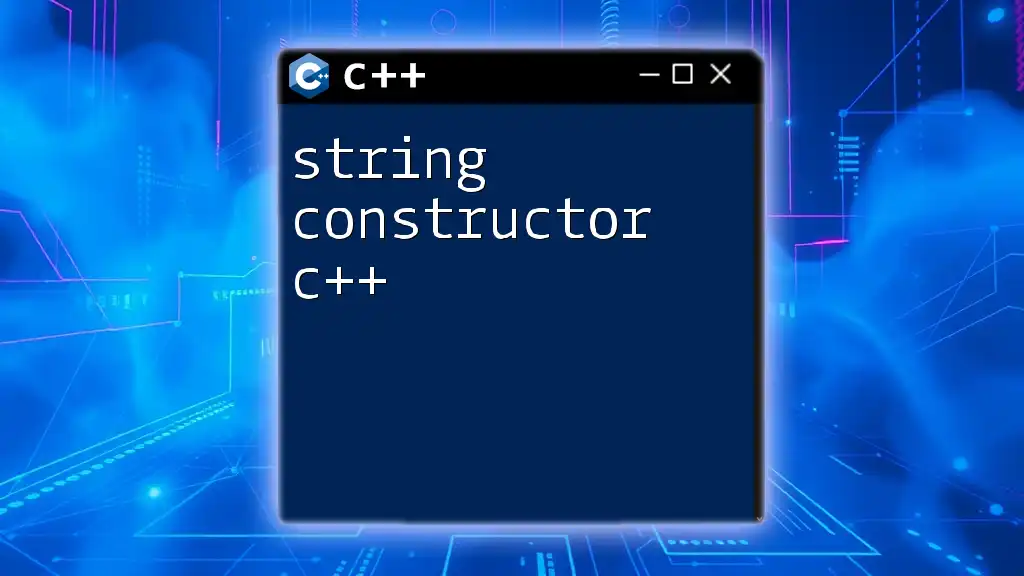
Additional Resources
For further reading and practice, consider delving into:
- C++ Reference documentation.
- Online coding platforms such as Codecademy or LeetCode.
Code Practice Problems
To reinforce what you've learned about `c++ struct constructor`, try implementing struct constructors in small practice coding exercises. Designing structs that mimic real-world entities will deepen your understanding.