In C++, a constructor is a special member function that initializes an object when it is created, while a destructor cleans up when the object is no longer needed.
Here’s a simple example:
#include <iostream>
class Example {
public:
Example() { // Constructor
std::cout << "Constructor called!" << std::endl;
}
~Example() { // Destructor
std::cout << "Destructor called!" << std::endl;
}
};
int main() {
Example obj; // Object creation, constructor is called
return 0; // Object destruction, destructor is called
}
What is a Constructor in C++?
Constructors are special member functions in C++ that are automatically called when an object of a class is created. Their primary purpose is to initialize the object's properties and allocate resources necessary for the object to perform its functions.
Types of Constructors
Default Constructor
A default constructor is invoked when an object is created without any parameters. If no constructor is defined, C++ provides a default constructor automatically.
class MyClass {
public:
MyClass() {
// Initialization code
cout << "Default Constructor Called" << endl;
}
};
In this example, when an instance of `MyClass` is created, the default constructor is called to initialize the object.
Parameterized Constructor
A parameterized constructor allows you to pass parameters during object creation. This enables you to set values that will initialize the object.
class MyClass {
public:
int value;
MyClass(int v) {
value = v; // Use value for initialization
cout << "Parameterized Constructor Called with value: " << value << endl;
}
};
When creating an object of `MyClass`, you can specify an integer that will be assigned to `value`.
Copy Constructor
A copy constructor is called when a new object is created as a copy of an existing object. It takes a reference to an object of the same class as a parameter and creates a new object based on it.
class MyClass {
public:
int value;
MyClass(const MyClass &obj) {
value = obj.value; // Copy the data from obj
cout << "Copy Constructor Called with value: " << value << endl;
}
};
This is crucial in scenarios where you want to duplicate an object without altering the original.

What is a Destructor in C++?
Destructors are also special member functions, but they are invoked automatically when an object goes out of scope or is explicitly deleted. Their main function is to perform cleanup, freeing resources that the object may have acquired during its lifetime.
Characteristics of Destructors
A destructor is unique in several ways:
- There can only be one destructor per class.
- Destructors cannot be overloaded, which means you cannot define multiple destructors with different parameters.
- The destructor has the same name as the class but prefixed with a tilde (`~`).
Code Example of Destructor
Here’s a simple example demonstrating a destructor:
class MyClass {
public:
MyClass() {
// Initialization code
cout << "Constructor Called" << endl;
}
~MyClass() {
// Cleanup code
cout << "Destructor Called" << endl;
}
};
In this example, when an object of `MyClass` is destroyed, the destructor is called to perform any necessary cleanup.

Constructor vs Destructor in C++
Understanding the difference between constructors and destructors is key to effective C++ programming.
- Constructors are responsible for initializing an object upon creation, while destructors handle the cleanup of the object before it is destroyed.
- Constructors can be overloaded (you can have multiple constructors within the same class), while destructors cannot be.
Lifecycle of an Object
The lifecycle of an object in C++ can be summarized as follows:
- Creation: When an object is created, a constructor is invoked.
- Usage: The object is used during its lifetime, executing its methods and accessing its properties.
- Destruction: When the object goes out of scope or is deleted, the destructor is automatically invoked to free resources.
Visualizing the lifecycle helps to understand when each function is called, ensuring efficient resource management.
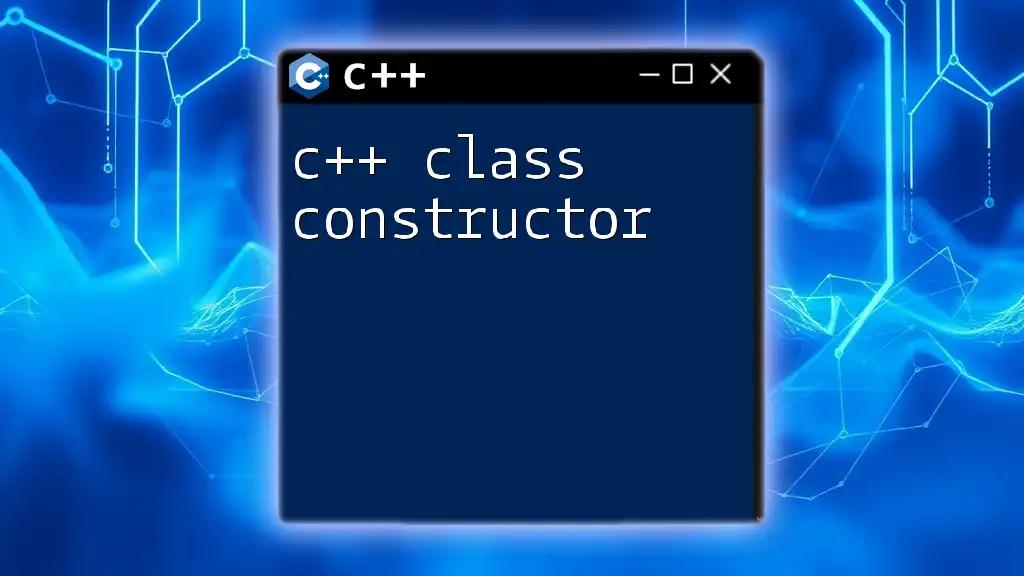
Constructors and Destructors in C++: Practical Applications
Constructors and destructors play a crucial role in real-world applications, particularly in resource management. For instance, when dealing with dynamic memory allocation or file handling, they ensure resources are appropriately allocated and freed.
Example: Managing Resources
Suppose you are managing a file resource. The following example illustrates the importance of both constructors and destructors in this context:
class FileHandler {
private:
FILE *fp;
public:
FileHandler(const char *filename) {
fp = fopen(filename, "r");
if (fp == nullptr) {
cout << "Could not open file" << endl;
}
}
~FileHandler() {
if (fp != nullptr) {
fclose(fp);
cout << "File closed" << endl;
}
}
};
Here, the constructor opens a file, and the destructor ensures that the file is properly closed when the `FileHandler` object is destroyed.

Best Practices for Using Constructors and Destructors
Creating constructors and destructors is not just about writing code; it’s essential to implement them effectively. Here are some best practices:
Avoiding Resource Leaks
Always define destructors when your class manages resources such as memory or file handles. Neglecting to do so can lead to resource leaks, where resources are allocated but never released.
Exception Safety
Handling exceptions during object construction is crucial. If an exception is thrown in a constructor, any resources allocated in that constructor must be cleaned up to prevent memory leaks.
Consider using smart pointers (like `std::unique_ptr` or `std::shared_ptr`) instead of raw pointers, as they automatically handle memory allocation and deallocation.
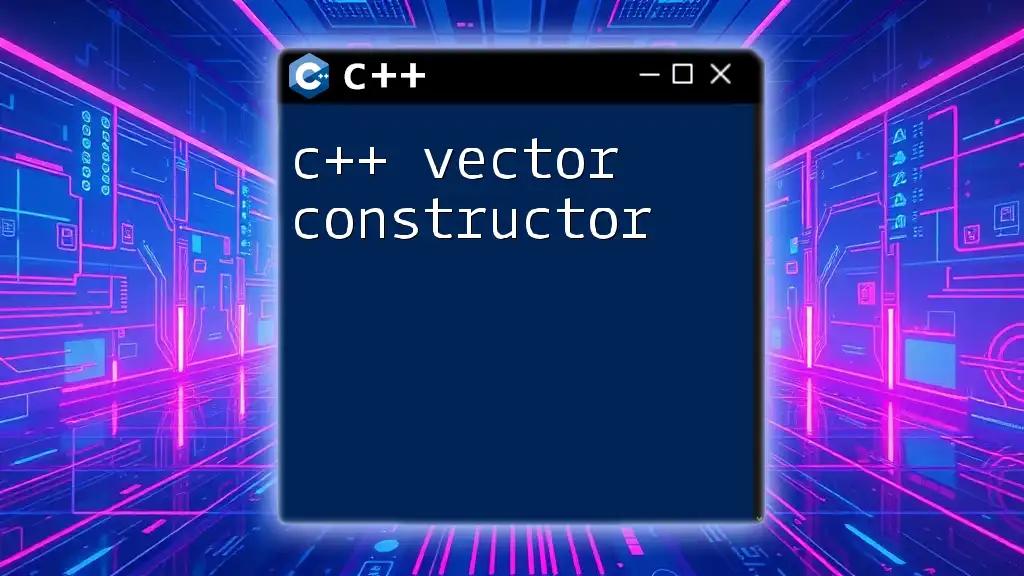
Common Mistakes in C++ Constructors and Destructors
Even experienced developers can stumble into common pitfalls when working with constructors and destructors.
Forgetting to Define Destructor
Failing to define a destructor when resources are acquired can lead to memory leaks. Over time, these leaks can accumulate, causing performance issues and application crashes.
Improper Usage of Copy Constructors
Copy constructors can introduce intricate issues, especially when managing dynamic resources. Failing to properly copy resources in the copy constructor can lead to double-free errors or shallow copies where both objects attempt to manage the same resource.

Conclusion
Understanding C++ constructors and destructors is vital for writing robust, efficient, and resource-safe C++ programs. Throughout this article, we explored the definitions, types, characteristics, uses, and best practices surrounding constructors and destructors. By mastering these concepts, you can enhance your programming skills and avoid common pitfalls, leading to more efficient and error-free coding.
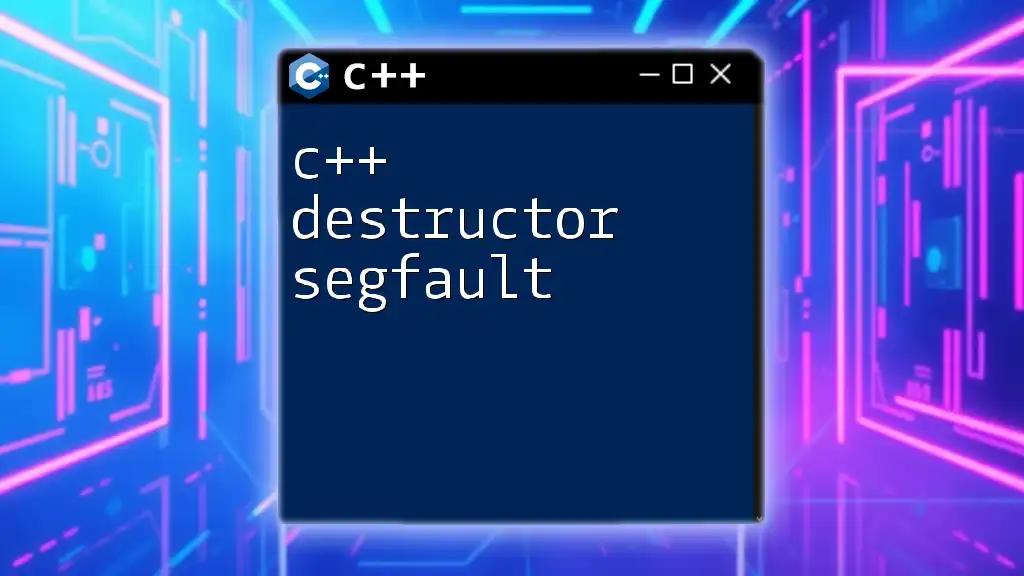
Further Reading and Resources
For those interested in deepening their understanding of C++ constructors and destructors, numerous resources are available, including widely regarded programming books, online courses, and official documentation that provide more in-depth knowledge and practical examples. Engaging with these materials will facilitate a stronger grasp of C++ and its features, enhancing your overall programming prowess.