A C++ destructor segfault occurs when trying to access or delete memory that has already been freed, typically due to improper memory management.
Here’s a simple example to illustrate a potential segfault in a destructor:
#include <iostream>
class MyClass {
public:
MyClass() {
data = new int(42);
}
~MyClass() {
delete data; // Correctly releasing memory
// Uncommenting the next line would cause a segfault if accessed after deletion
// std::cout << *data << std::endl;
}
private:
int* data;
};
int main() {
MyClass obj;
return 0;
}
Understanding Destructors in C++
What is a Destructor?
A destructor is a special member function in C++ that is automatically invoked when an object goes out of scope or is explicitly deleted. The primary role of a destructor is to release resources that the object may have acquired during its lifetime. This includes deallocating memory, closing file handles, or freeing any other resources that might lead to memory leaks.
The syntax for a destructor is straightforward. It follows the same naming convention as the class but precedes it with a tilde (~). For example:
class MyClass {
public:
MyClass() {
// Constructor code
}
~MyClass() {
// Destructor code
}
};
You can define both default destructors and custom destructors. The former is provided automatically by the compiler if you do not define one, while the latter gives you situational control over resource cleanup.
Importance of Destructors
Destructors play a crucial role in resource management. When objects are no longer needed, destructors ensure that any associated resources are properly released. Failure to use destructors effectively can lead to severe problems, including memory leaks, which occur when allocated memory is not returned to the system, eventually exhausting available memory.
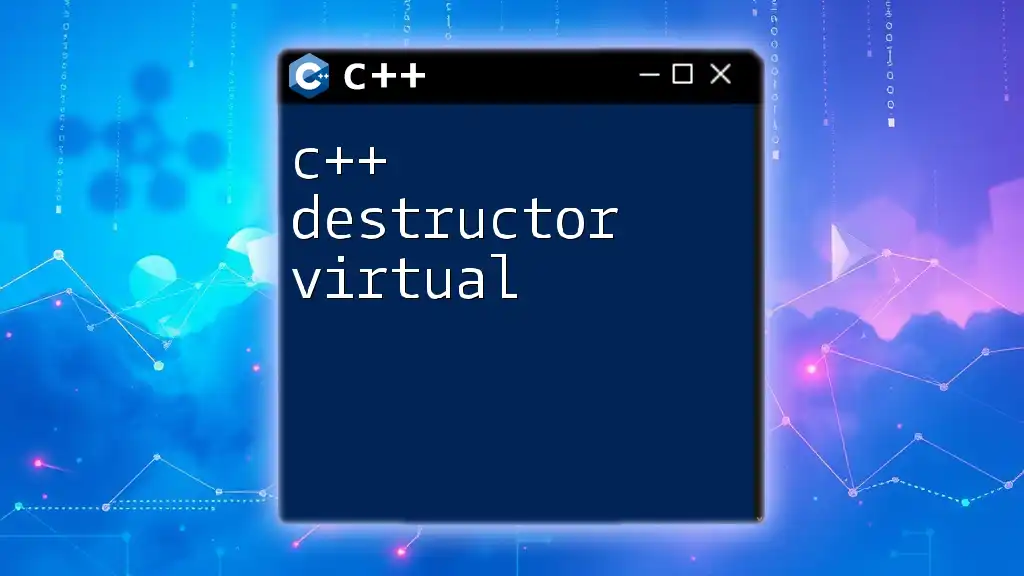
Common Causes of Segfaults in Destructors
What is a Segmentation Fault?
A segmentation fault, often referred to as a segfault, is an error that occurs when a program attempts to access a restricted area of memory. This typically results from a memory access violation, such as trying to use a pointer that points to memory that has already been deallocated. In the context of destructors, segfaults are often directly related to improper memory management practices.
Mismanagement of Dynamic Memory
One of the most common causes of segfaults involving destructors is the mismanagement of dynamic memory. When memory is allocated dynamically (using `new`), it must eventually be deallocated using `delete`. Failing to do so leads to memory leaks, which can contribute to segmentation faults in long-running applications.
class MyClass {
public:
int* ptr;
MyClass() {
ptr = new int(42); // Dynamic memory allocation
}
~MyClass() {
// No deallocation
}
};
In the example above, although `ptr` is dynamically allocated, no destructor is implemented to deallocate `ptr`, leading to a memory leak.
Double Deletion of Pointers
Double deletion occurs when a pointer is deleted more than once. In C++, this can happen easily if not handled correctly. Deleting a pointer again after it has already been deleted can lead to segfaults.
class MyClass {
public:
int* ptr;
MyClass() {
ptr = new int(42);
}
~MyClass() {
delete ptr; // First deletion
delete ptr; // Second deletion - causes segfault
}
};
In the code snippet above, attempting to delete `ptr` a second time will lead to undefined behavior, often resulting in a segmentation fault.
Dangling Pointers
A dangling pointer refers to a pointer that does not point to a valid object of the appropriate type, either because the object was deleted or it went out of scope. Dangling pointers can pose a significant risk, especially if the memory they point to is accessed after deallocation.
class MyClass {
public:
int* ptr;
MyClass() {
ptr = new int(42);
}
~MyClass() {
delete ptr; // Properly deleted
}
};
void example() {
MyClass obj;
int* danglingPtr = obj.ptr; // Dangling pointer after obj goes out of scope
}
In this example, `danglingPtr` points to a now-deleted memory location after `obj` falls out of scope, which can lead to segfaults when accessed.
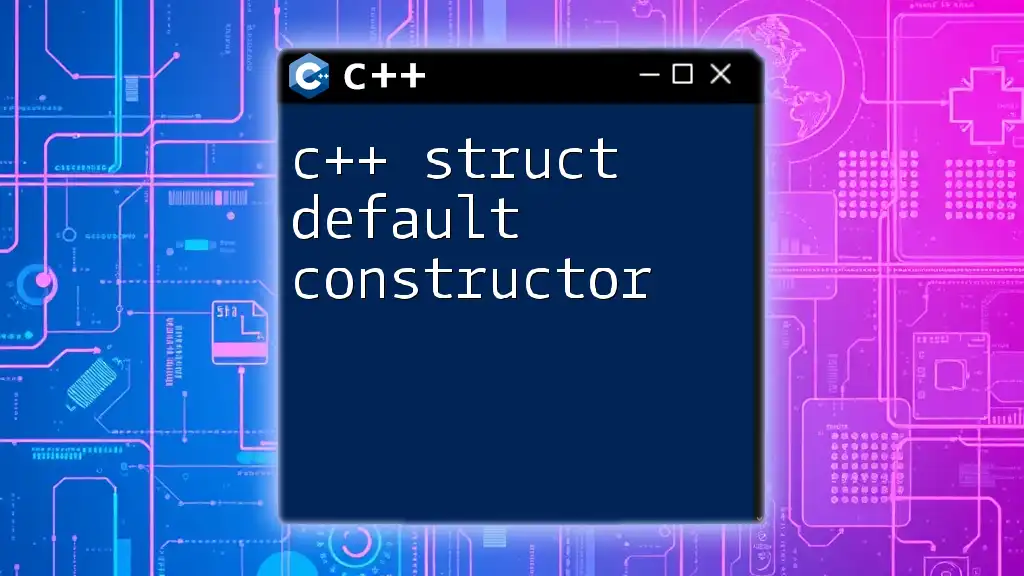
Real-World Scenarios of Destructor Segfaults
Case Study: Improper Resource Release
Consider the following scenario where resources are not properly managed:
class Resource {
public:
Resource() {
// Acquire resources
}
~Resource() {
// Forgetting to release resources can lead to leaks
}
};
In this example, if the resources are not released properly in the destructor, the application will suffer from memory leaks, leading to instability and potentially a segfault during later operations. The recommendation here is to always ensure that resources are appropriately handled in your destructor.
Case Study: Circular References
Circular references happen when two or more objects reference each other. When destructors attempt to release these references, they can end up stuck in a loop or trying to access already deleted memory, which can lead to segfaults.
class Node {
public:
Node* next;
Node() : next(nullptr) {}
~Node() {
delete next; // This can lead to issues if there's a circular reference
}
};
To avoid this trap, one must break the circular reference by ensuring that at least one of the references is nullified before deletion in the destructor.
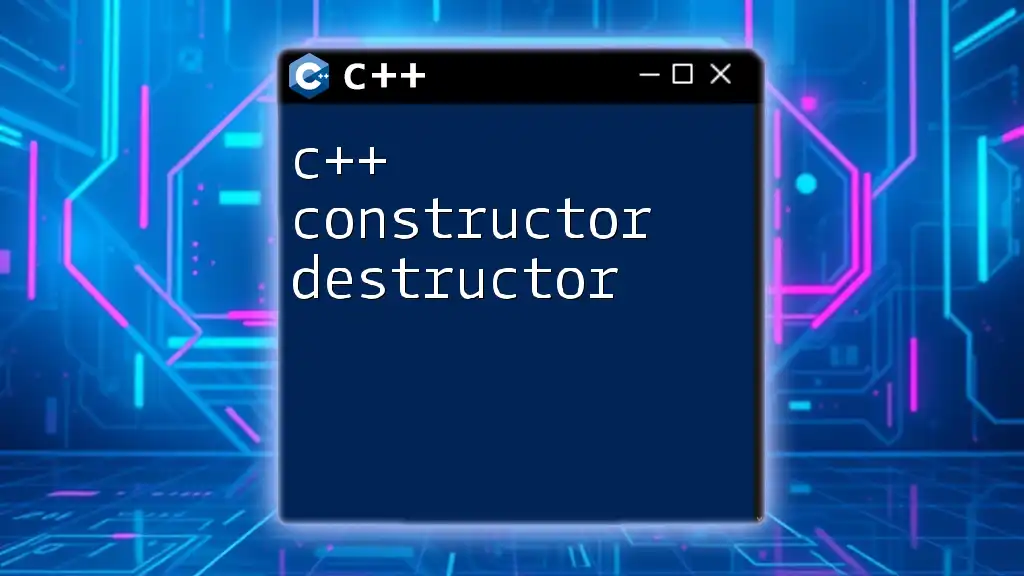
Best Practices in Implementing Destructors
Rule of Three
The Rule of Three in C++ states that if a class defines one of the following: a destructor, a copy constructor, or a copy assignment operator, it should probably define all three. This establishes a strong guarantee that resources are managed consistently.
class MyClass {
public:
int* ptr;
MyClass() {
ptr = new int(42); // Constructor
}
MyClass(const MyClass& other) {
ptr = new int(*other.ptr); // Copy Constructor
}
MyClass& operator=(const MyClass& other) {
if (this != &other) {
delete ptr; // Cleanup
ptr = new int(*other.ptr); // Copy assignment
}
return *this;
}
~MyClass() {
delete ptr; // Destructor
}
};
By following the Rule of Three, you ensure robust management of dynamically allocated resources and minimize the risk of segfaults.
Smart Pointers as an Alternative
To mitigate common issues associated with raw pointer management, consider utilizing smart pointers. Smart pointers (like `std::unique_ptr`, `std::shared_ptr`, and `std::weak_ptr`) automatically manage the memory they own, significantly reducing the risk of leaks and dangling pointers.
#include <memory>
class MyClass {
public:
std::unique_ptr<int> ptr;
MyClass() : ptr(std::make_unique<int>(42)) {}
// No need for custom destructor or copy semantics
};
Smart pointers automatically handle deallocation when their scope ends, making your code safer and cleaner.
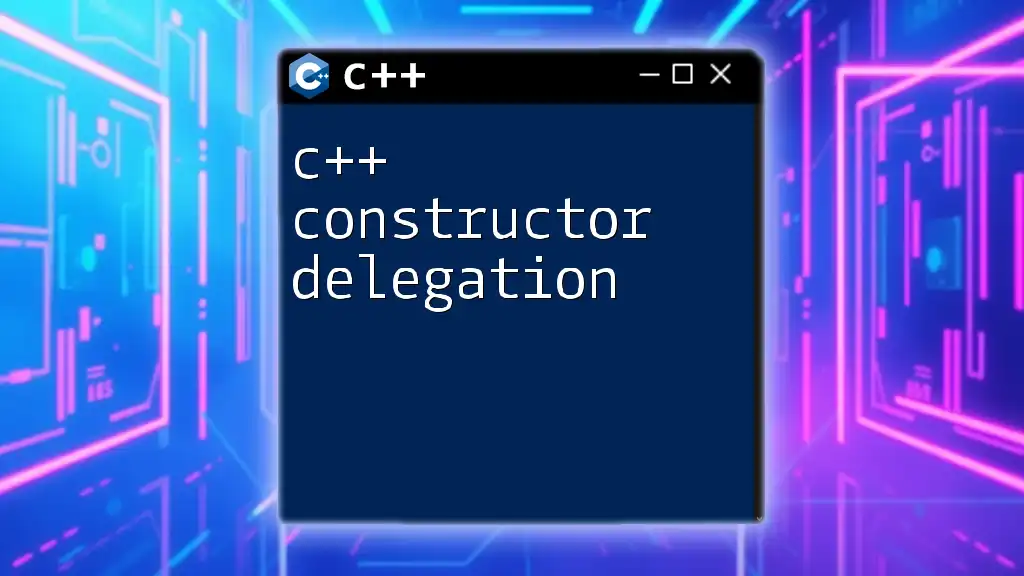
Debugging Segfaults Related to Destructors
Tools and Techniques
When dealing with segmentation faults, employing debugging tools can be invaluable. gdb (GNU Debugger) and the Visual Studio Debugger are powerful tools that can help you step through code execution to locate the precise point of failure. Additionally, memory analysis tools like valgrind can assist in identifying memory leaks and invalid memory accesses.
Common Debugging Steps
To effectively debug segfaults related to destructors, follow these steps:
- Identify the Location: Use a debugger to pinpoint where the segmentation fault occurs.
- Analyze Stack Trace: Examine the stack trace to understand the sequence of function calls leading up to the segfault.
- Isolate the Problem: Once you identify the problematic code, isolate it to test different scenarios and find the root cause of the segfault.
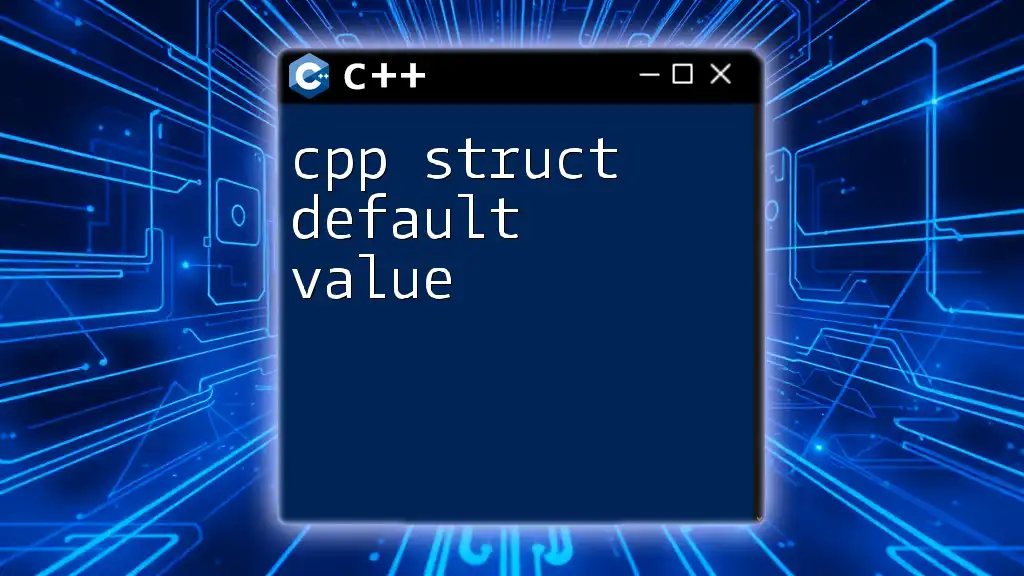
Conclusion
Understanding the intricacies of destructors and their connection to segmentation faults is crucial for C++ programmers. By recognizing common pitfalls such as memory mismanagement, double deletion, and dangling pointers, developers can create safer applications. Remember to adhere to best practices like the Rule of Three and consider using smart pointers whenever possible.
Implementing these strategies will significantly reduce the risks associated with C++ destructor segfaults. Take the time to learn and apply these principles to write robust, memory-safe code, ensuring the successful management of resources in your C++ applications.
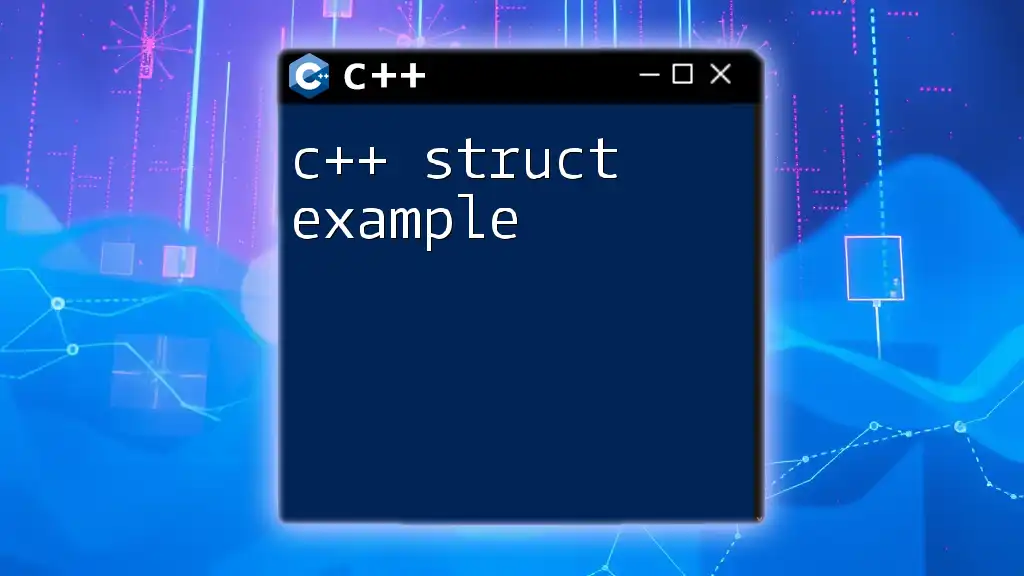
Additional Resources
For further learning and exploration, consider checking out relevant documentation, C++ programming books, and online courses. Engaging in community forums can also provide valuable insights and guidance on mastering memory management and C++ destructors.