A C++ struct is a user-defined data type that allows the grouping of variables under a single name for more organized code.
struct Person {
std::string name;
int age;
};
Person john;
john.name = "John Doe";
john.age = 30;
Understanding Structs
Definition of Struct in C++
In C++, a struct is a user-defined data type that allows you to group related variables under a single name. You can think of a struct as a blueprint for creating complex data types that can hold multiple attributes. Unlike classes, which are also user-defined types in C++, structs have certain key differences. Notably, all members of a struct are public by default. This aspect makes structs an ideal choice for plain data structures where you simply want to group related fields together.
Key Characteristics of Structs
- Public by Default: Members of a struct can be accessed directly without any qualifiers unless specified otherwise. This makes it easier to work with for simple data aggregation.
- Data Aggregation: A struct can hold different data types, making it versatile for grouping various characteristics of an object.
- Use Cases: Structs are particularly useful in scenarios where you want to model simple entities instead of creating full-fledged classes. For example, you might choose a struct to represent a point in a 2D space without the overhead of additional class features like methods or encapsulation.
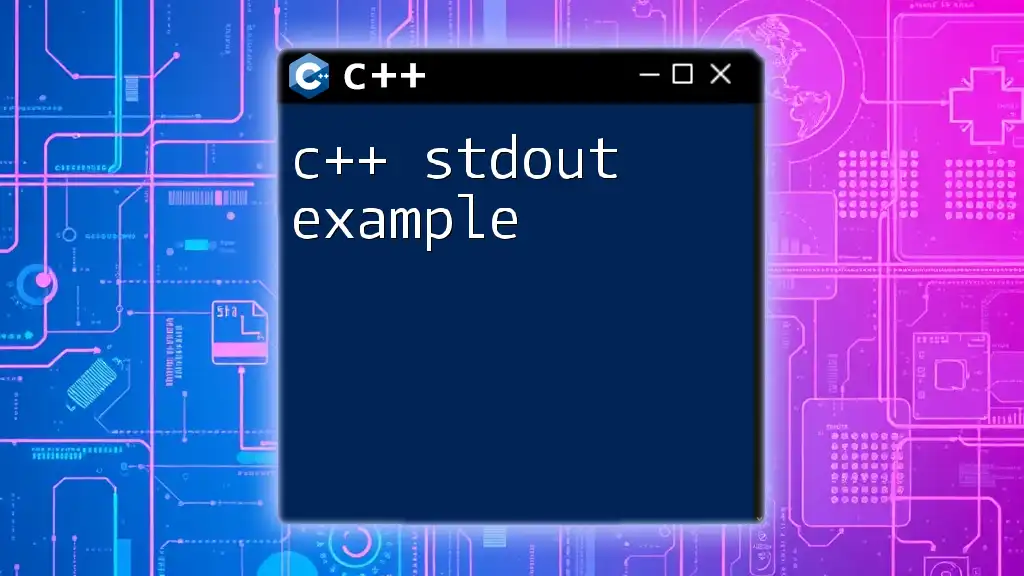
Declaring a Struct
Basic Syntax
To declare a struct in C++, you start with the keyword `struct`, followed by the name of the struct, and then encapsulate the member variables within curly braces. The basic syntax looks as follows:
struct StructName {
dataType member1;
dataType member2;
// more members
};
Example of a Simple Struct
Here is a practical example of how to declare a struct named `Person`:
struct Person {
std::string name;
int age;
};
In this snippet, we define a struct called Person that contains two members: a `std::string` for the person's name and an `int` for their age.
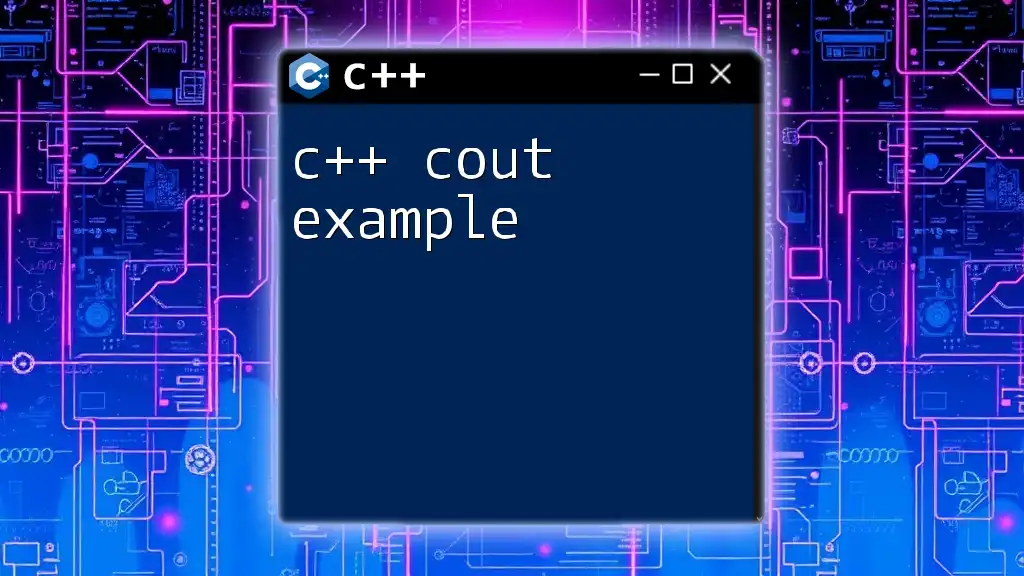
Accessing Struct Members
Dot Operator
To access the members of a struct, you use the dot operator (`.`). For example:
Person person;
person.name = "Alice";
person.age = 30;
In this code, we create an instance of the `Person` struct named `person` and directly assign values to its members, `name` and `age`.
Accessing Members via Pointers
You can also access struct members using pointers. Here's how:
Person *pPerson = &person;
std::cout << pPerson->name << " is " << pPerson->age << " years old.";
In this example, we first create a pointer to the `person` instance and then use the `->` operator to access its members.
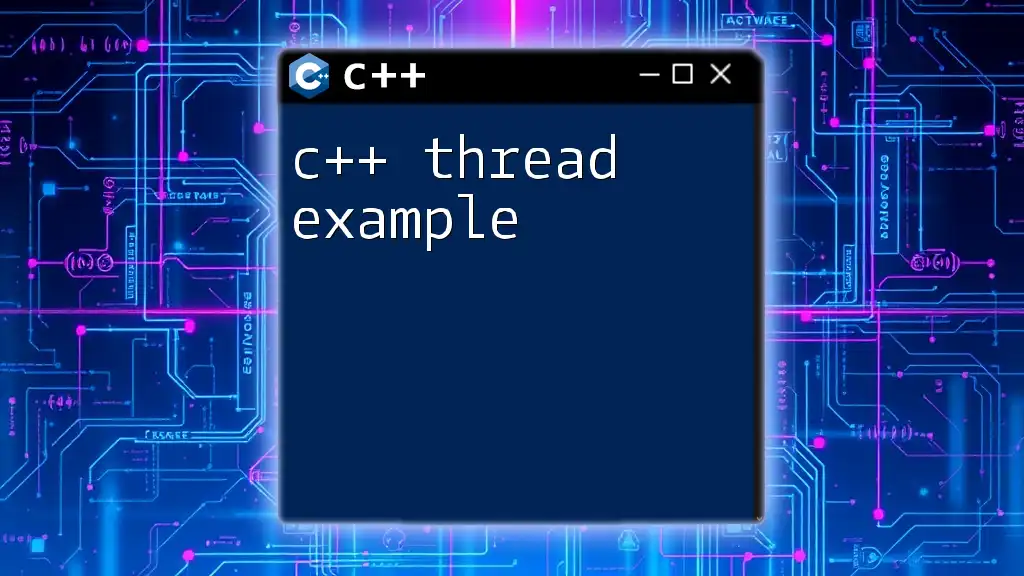
Initializing Structs
Default Initialization
Structs can be initialized at the time of declaration using an initializer list:
Person person{"Bob", 25};
In this case, we are creating an instance of `Person` and initializing its members in a single statement. This method allows for clean and concise code.
Using Constructors with Structs
You can enhance the utility of structs by adding constructors, similar to classes. Here’s an example:
struct Car {
std::string brand;
int year;
Car(std::string b, int y) : brand(b), year(y) {}
};
Car car1("Toyota", 2020);
In this case, we define a struct called `Car` that includes a constructor to facilitate initialization. This approach adds functionality while maintaining the simplicity of structs.
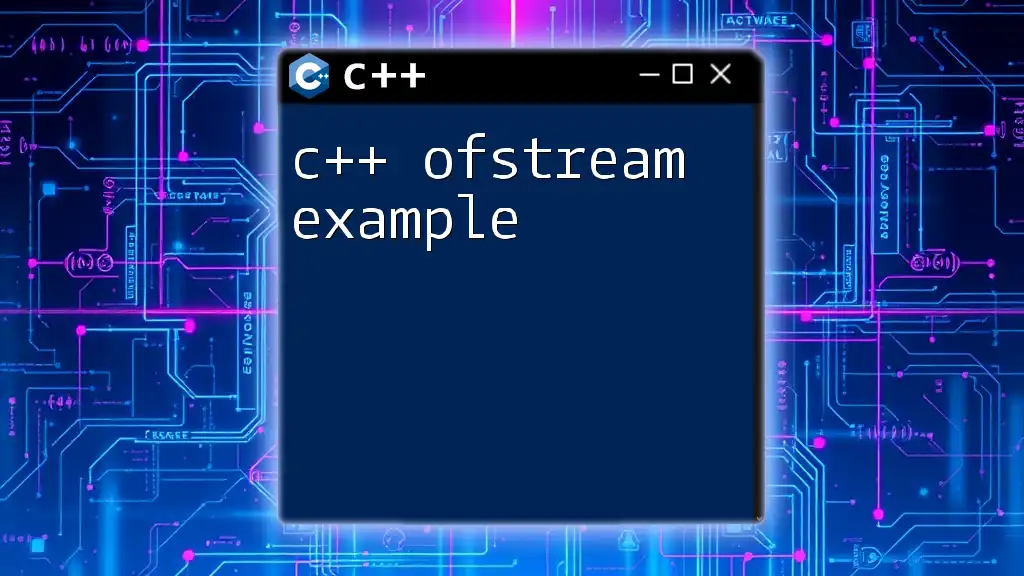
Structs with Functions
Member Functions in Structs
Structs can contain member functions, which can be beneficial for processing the data within the struct. For instance:
struct Rectangle {
int width, height;
int area() {
return width * height;
}
};
Here, the `Rectangle` struct has a member function `area` that calculates the area based on its dimensions, `width` and `height`.
Passing Structs to Functions
You can also pass a struct as an argument to functions. Here’s an example:
void printPerson(const Person& p) {
std::cout << p.name << " is " << p.age << " years old.";
}
By using a reference (`const Person&`), you can pass the struct efficiently without the overhead of copying it. This technique helps in performance-sensitive applications.
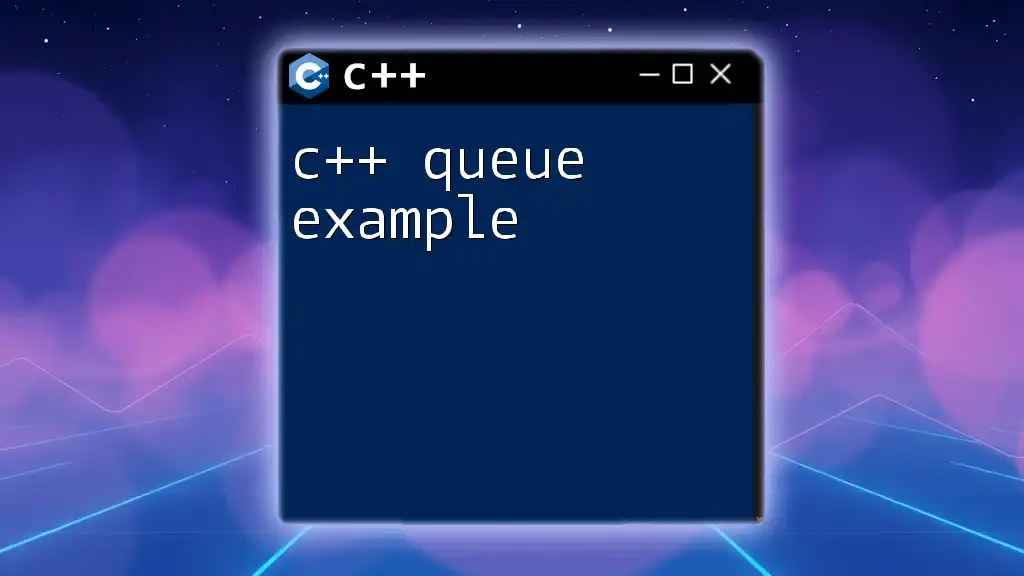
Advanced Struct Usage
Nested Structs
Structs can be nested, enabling you to create more complex data structures. Consider the following example:
struct Address {
std::string street;
std::string city;
};
struct Employee {
std::string name;
Address address;
};
Here, we define an `Address` struct that can be encapsulated within an `Employee` struct. This demonstrates how structs can model real-world relationships effectively.
Structs with Array Members
You can also include arrays as members within a struct:
struct Student {
std::string name;
int grades[5];
};
In this example, the `Student` struct holds an array of integers to represent grades. This allows you to manage multiple related pieces of information in a single entity.
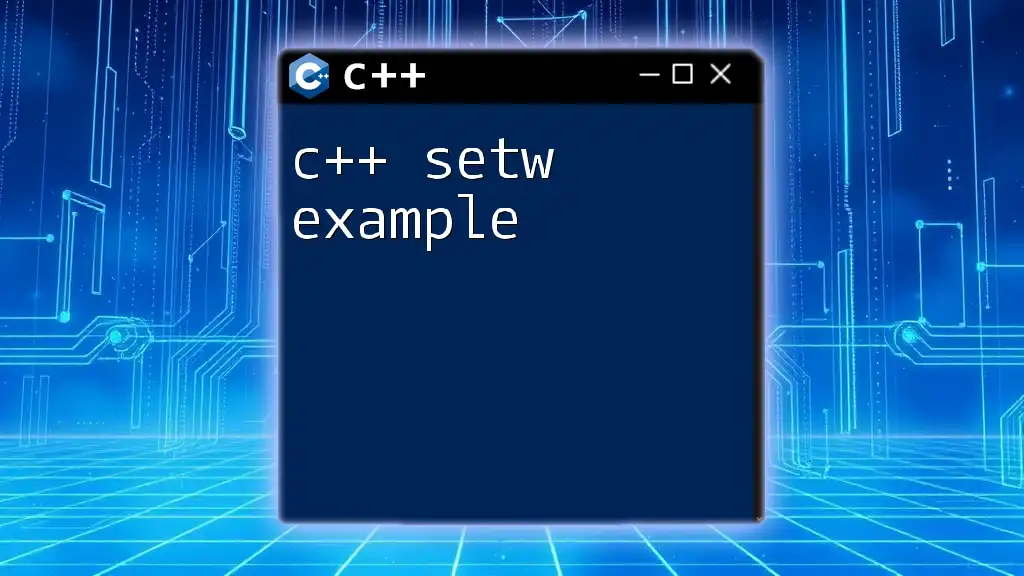
Conclusion
C++ structs are a powerful feature that provides a way to create complex data types with ease. By understanding the nature of structs, their declaration, accessibility, initialization, and advanced usage, you can effectively model data for your applications. Experimenting with structs will enhance your programming skills and broaden your understanding of data structures in C++. Remember, the next time you need a straightforward way to group related data, think of using a struct in your C++ code.