In C++, the `switch` statement allows for multi-way branching based on the value of a variable, and the `default` case is executed if none of the specified `case` constants match.
#include <iostream>
int main() {
int value = 3;
switch (value) {
case 1:
std::cout << "Value is 1" << std::endl;
break;
case 2:
std::cout << "Value is 2" << std::endl;
break;
default:
std::cout << "Value is neither 1 nor 2" << std::endl;
break;
}
return 0;
}
Understanding the Switch Statement in C++
What is the Switch Statement?
The switch statement in C++ is a control structure that allows you to evaluate a variable and execute corresponding codes based on its value. Unlike the if-else statement, which checks conditions sequentially, the switch statement provides a clearer and more efficient means to handle multiple potential values. This efficiency in managing multiple conditions can greatly enhance both code readability and performance.
How to Use Switch in C++
The syntax of the switch statement follows this structure:
switch(expression) {
case constant1:
// Code to be executed if the expression equals constant1
break;
case constant2:
// Code to be executed if the expression equals constant2
break;
// Additional cases...
default:
// Code to be executed if the expression doesn't match any cases
}
In this syntax:
- expression is evaluated once and compared against different case constants.
- Each case represents a potential match for the expression.
- The break statement is crucial as it prevents execution from falling through to subsequent cases unless explicitly intended.
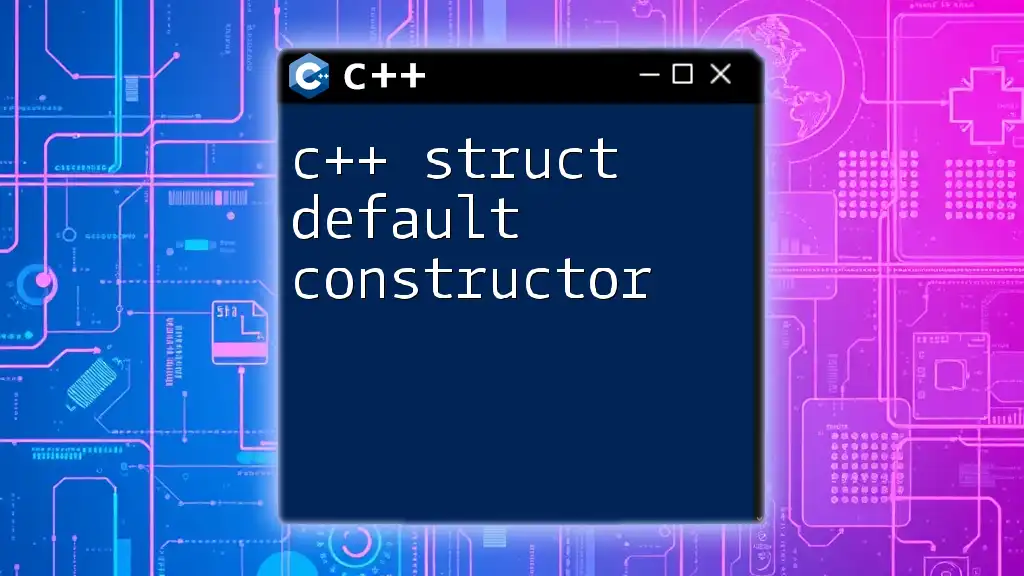
Exploring the Switch Default in C++
What is the Default Case?
The default case in a switch structure acts as a fallback option. It is executed when none of the specified cases match the evaluated expression. Having a default case is invaluable for robust coding, as it helps manage unexpected values and enhance program resilience.
Syntax of Switch Default Case in C++
The default case can be added to a switch statement like this:
default:
// Code to be executed if no cases match
break;
The default case can appear anywhere within the switch statement, although it is often placed at the end for clarity. The structure remains clear and easy to read, even with the inclusion of a default case.
Example of Switch Statement with Default Case
Consider this practical example:
#include <iostream>
using namespace std;
int main() {
int value;
cout << "Enter a number between 1 and 5: ";
cin >> value;
switch (value) {
case 1:
cout << "You entered one." << endl;
break;
case 2:
cout << "You entered two." << endl;
break;
case 3:
cout << "You entered three." << endl;
break;
case 4:
cout << "You entered four." << endl;
break;
case 5:
cout << "You entered five." << endl;
break;
default:
cout << "Number is out of range." << endl; // Default case
break;
}
return 0;
}
In the code provided:
- When the user inputs a number within the range, the corresponding message is displayed.
- If the input number is outside the defined cases (1-5), the default message `"Number is out of range."` is shown, effectively capturing unexpected inputs.
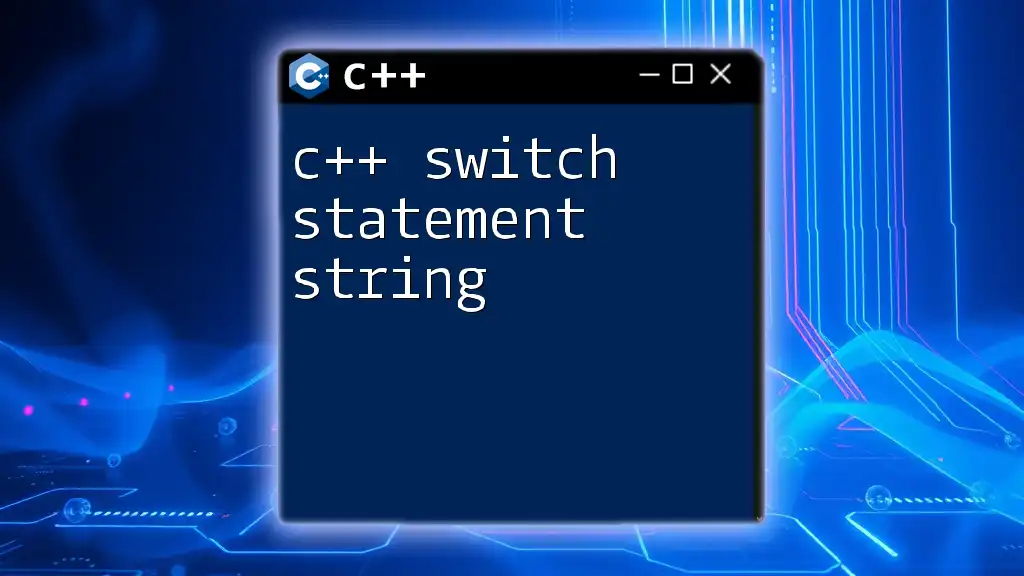
Best Practices When Using Switch Default
When Should You Use a Default Case?
Employing a default case is essential when you anticipate user input or data that could potentially fall outside the defined range of acceptable values. By using a default case, you can ensure your application behaves predictably, regardless of user errors or unexpected conditions, thereby improving overall user experience.
Avoiding Common Mistakes
While working with switch statements, several pitfalls can arise:
- Forgetting the `break` statement: If you omit the break statement after a case, execution will continue into subsequent cases, which may cause unexpected behavior.
- Neglecting the default case: Always consider including a default case to handle unanticipated values. This will make your application more robust and user-friendly.
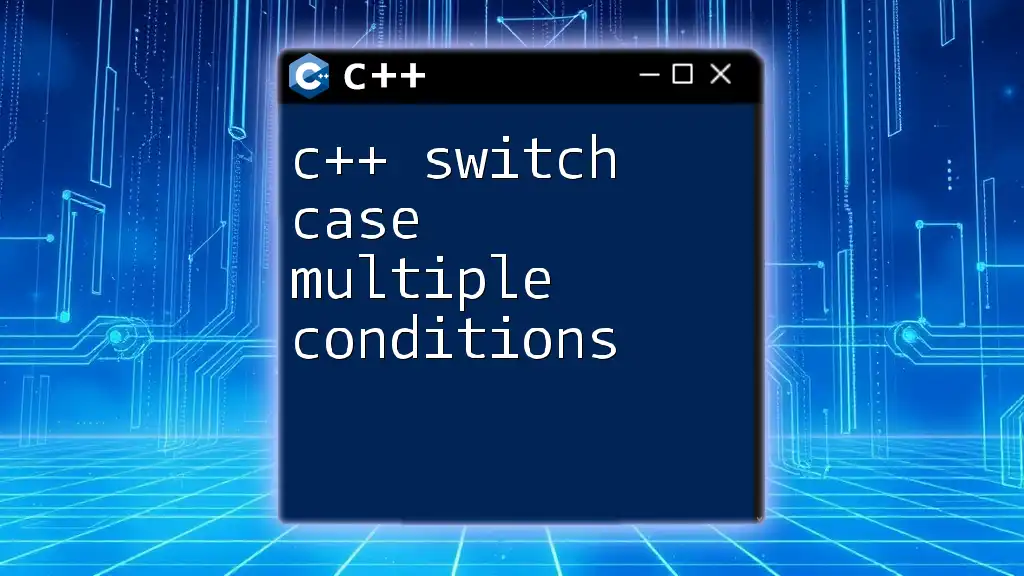
Advanced Features of C++ Switch
Using Switch with Strings in C++
In C++, the switch statement does not natively support strings; it works primarily with integral types (like int or char). If you need switch-like functionality for string comparisons, consider using `if-else` statements or newer data structures like `std::map` or `std::unordered_map`. Here's an example of how you might simulate a switch statement using `if-else` for strings:
string value;
cout << "Enter a color (red, blue, green): ";
cin >> value;
if (value == "red") {
cout << "You chose red!" << endl;
} else if (value == "blue") {
cout << "You chose blue!" << endl;
} else if (value == "green") {
cout << "You chose green!" << endl;
} else {
cout << "Invalid color!" << endl; // Default-like handling
}
This approach effectively manages string comparisons, allowing for flexible input handling.
Nested Switch Statements
Nested switch statements allow for handling complex scenarios where the value from one switch may lead to further conditions. For example:
switch (outerValue) {
case 1:
switch (innerValue) {
case 1:
cout << "Outer is 1, Inner is also 1." << endl;
break;
// Other cases...
}
break;
// Other outer cases...
}
This structure is helpful in situations where multiple levels of conditions exist, providing a powerful tool for complex decision-making in code.
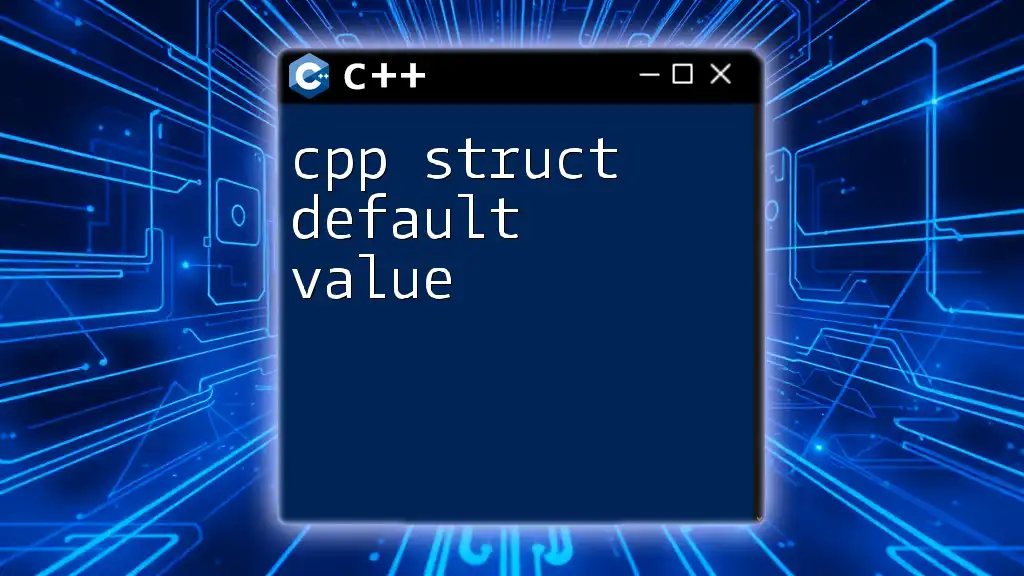
Conclusion
Recap of Key Takeaways
Understanding the c++ switch default is crucial for effective programming. It enhances control flow management, safeguards against unexpected inputs, and contributes significantly to code clarity and maintainability.
Further Learning Resources
To solidify your understanding and delve deeper into advanced C++ concepts, consider exploring books and online platforms that offer tutorials and exercises on C++ programming. They can provide invaluable insights into best practices and advanced techniques.
Encouraging Engagement
We encourage you to leave comments or questions about your experiences with the switch statement in C++. Sharing your examples helps foster a vibrant learning community.
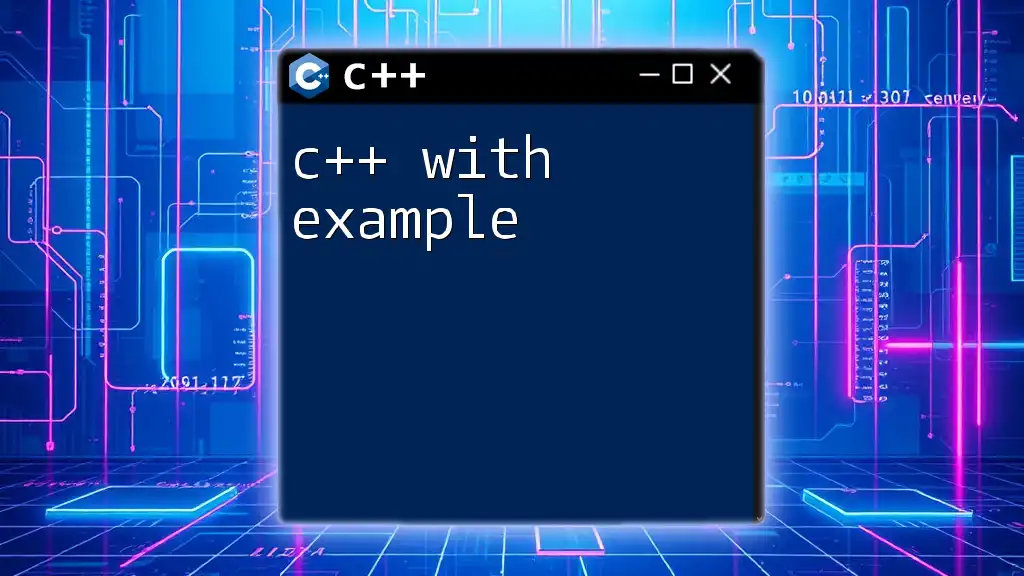
Call to Action
Join the conversation by sharing your thoughts and examples of using switch statements and default cases in C++. Don't forget to subscribe to our newsletter for more concise C++ tips and tricks that can elevate your programming skills!