C++ `stdout` is the standard output stream used to display output to the console, and can be utilized using the `cout` object from the iostream library.
Here’s a simple code snippet demonstrating the usage of `cout`:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Standard Output in C++
What is Standard Output?
Standard output refers to the default output stream in a C++ program that usually displays output to the console or terminal. In C++, streams are sequences of characters that can be used to send and receive data. The standard output plays a vital role in displaying the results or status of a program to the user. Understanding `stdout` is essential since it is the method most developers use to communicate with users through printed output.
Unlike `stdout`, which is always directed to the console by default, alternative methods like file output or logging can be used for other applications. Familiarity with these differences allows developers to choose the appropriate output method based on their program's needs.
The Role of stdout in C++
In C++, `stdout` is associated with `std::cout`, an instance of the output stream class defined in the `<iostream>` header file. This object is crucial for sending formatted output to the console, making it easier for users to interact with and understand program output.
To utilize `stdout`, ensure you include the appropriate header at the beginning of your C++ file:
#include <iostream>
By leveraging `std::cout`, you can utilize the full power of C++ output functionalities.
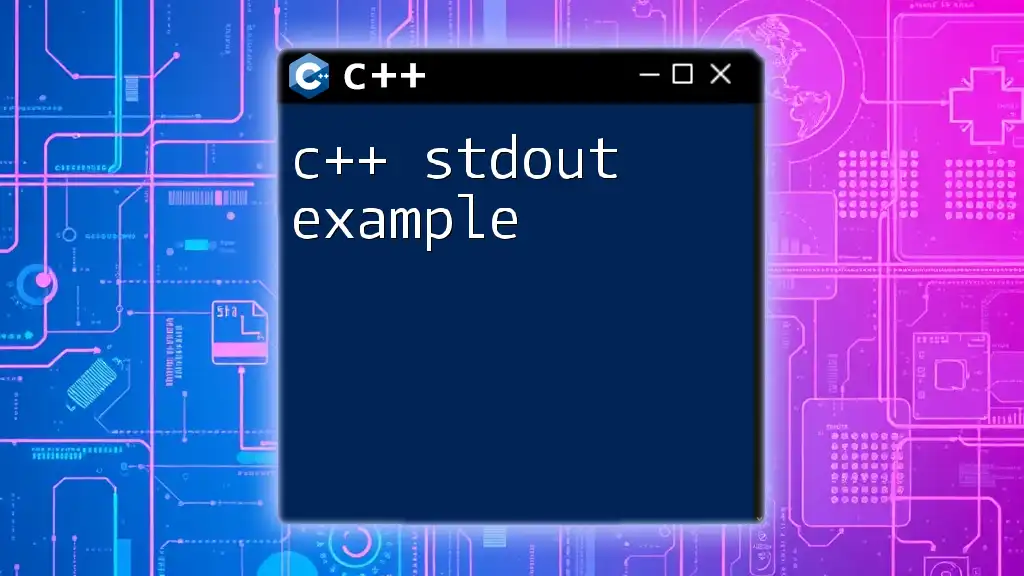
Getting Started with stdout in C++
Setting Up Your Environment
Before diving into the world of `c++ stdout`, you need to set up a development environment. Popular Integrated Development Environments (IDEs) like Visual Studio, Code::Blocks, and Eclipse can help you easily compile and run your C++ code. Once you are set up, create a new C++ source file to start working with `stdout`.
Basic Syntax
To output data using `stdout`, you need to write a simple program that demonstrates fundamental syntax. Here’s an example of how to print "Hello, World!" using `std::cout`:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this example, `std::cout` is used to send the string to `stdout`, and `std::endl` is used to end the line, flush the output buffer, and move the cursor to the next line.
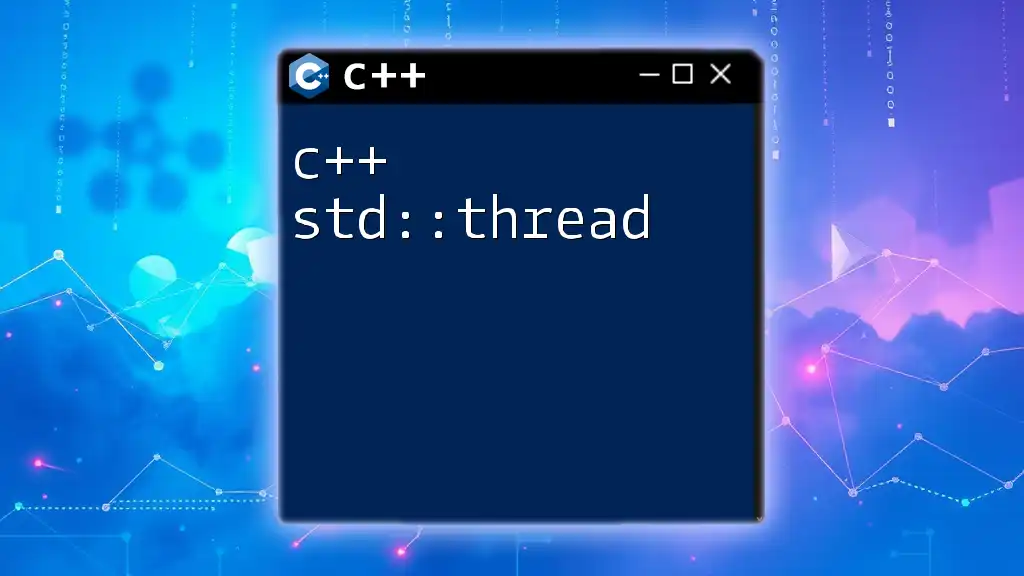
Using stdout with Formatting
Stream Manipulators
C++ offers stream manipulators that enable you to affect the format of the output. Three commonly used manipulators are `std::endl`, `std::flush`, and `std::setw()`.
Here’s an example that illustrates their use:
#include <iostream>
#include <iomanip> // Required for std::setw
int main() {
std::cout << std::setw(10) << "Name" << std::endl;
std::cout << std::setw(10) << "Alice" << std::endl;
std::cout << std::setw(10) << "Bob" << std::endl;
return 0;
}
In this snippet, `std::setw(10)` specifies that the next output should be at least 10 character spaces wide. This enhances the readability of output data.
Formatting Output
When working with different data types, formatting becomes crucial for clarity. Here’s how you can format a floating-point number:
#include <iostream>
#include <iomanip>
int main() {
double pi = 3.14159;
std::cout << std::fixed << std::setprecision(2) << pi << std::endl;
return 0;
}
In this code, `std::fixed` ensures the number is displayed in fixed-point notation, while `std::setprecision(2)` limits the printed value to two decimal places, making it clear and easy to understand.
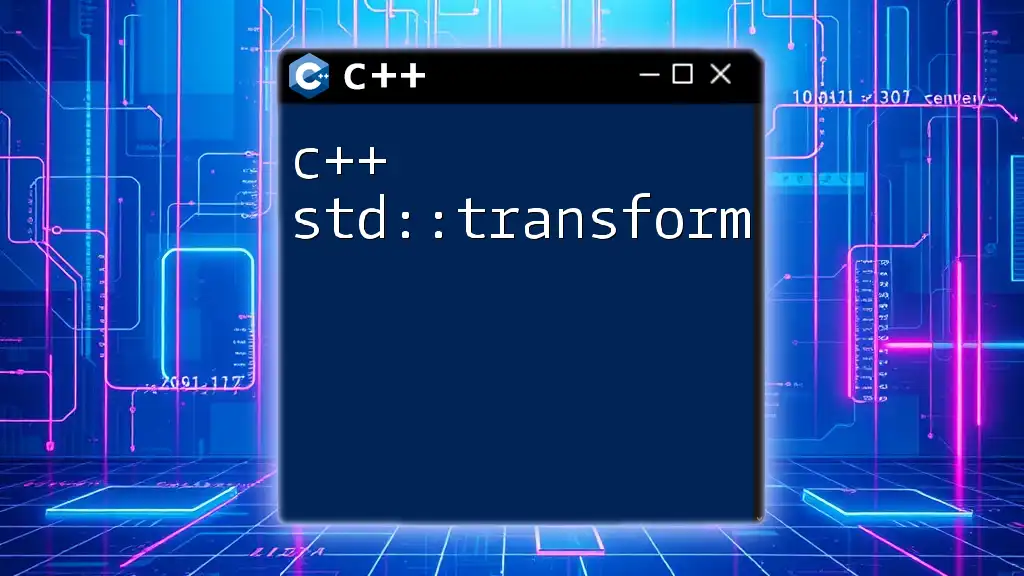
Advanced Usage of stdout
Redirecting Output
One powerful feature of using `stdout` is the ability to redirect its output to a file. This enables you to save output for analysis or record-keeping without modifying your program.
You can redirect the output of your C++ program to a file in the command line like this:
./my_program > output.txt
In this command, `output.txt` will contain everything that would normally be printed to the console.
Flushing stdout
Flushing the output buffer is essential when you want to ensure that all buffered output is sent to `stdout`. Flushing can be especially helpful in real-time applications where immediate feedback is necessary. Here’s an example that highlights the significance of flushing:
#include <iostream>
#include <thread> // Required for std::this_thread::sleep_for
#include <chrono> // Required for std::chrono
int main() {
std::cout << "Processing";
std::cout.flush(); // Ensure "Processing" is printed immediately
std::this_thread::sleep_for(std::chrono::seconds(2));
std::cout << " Done!" << std::endl;
return 0;
}
In this example, `std::flush()` makes sure that the text "Processing" is displayed immediately, even before the program pauses for two seconds. This characteristic is vital in scenarios where feedback is critical.
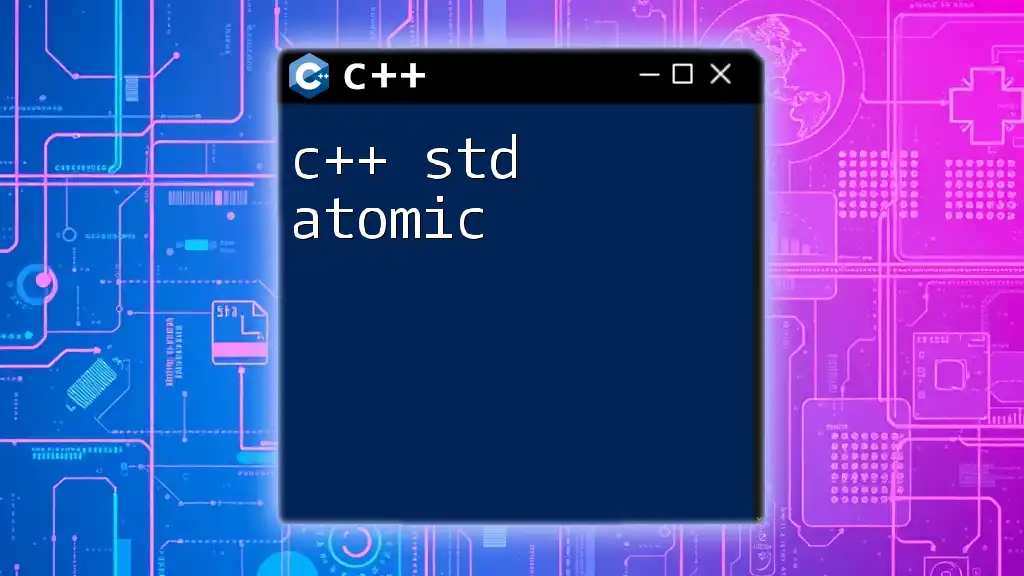
Troubleshooting Common Issues
Common Mistakes with stdout
When working with `c++ stdout`, beginners often make a few common mistakes, such as:
- Not including the `<iostream>` header.
- Confusing `std::cout` with other output methods, leading to runtime errors or incorrect output.
Debugging stdout Output
To debug output issues effectively, consider checking:
- If the output is correctly directed to `stdout` or redirected elsewhere by accident.
- The potential presence of logic errors leading to unexpected results in your output.
It can also be beneficial to use error codes and logging alongside `stdout` for better visibility into your program's operations.
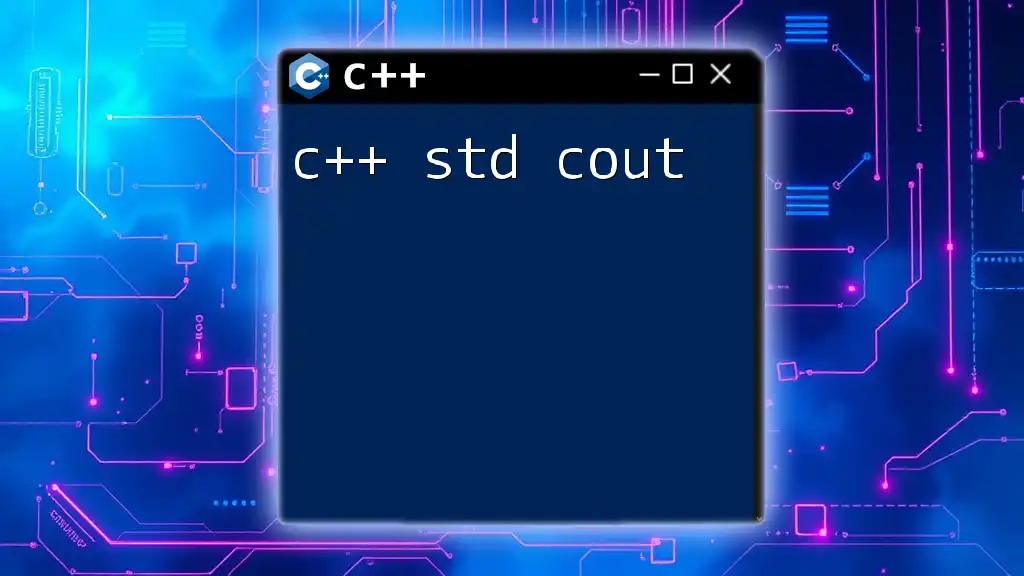
Conclusion
Mastering `c++ stdout` is a fundamental skill for any C++ developer. Understanding how to format, manipulate, and redirect output enhances the user experience and makes debugging smoother. Practicing these concepts by creating small projects will solidify your knowledge and help you effectively communicate with users through your applications. Embrace the power of standard output as you develop your C++ skills further, and push your programming capabilities to new heights!