The `std::atomic` is a C++ template class that provides a way to perform atomic operations on data types, ensuring thread safety without the use of mutexes.
Here's a simple code snippet demonstrating its usage:
#include <iostream>
#include <atomic>
#include <thread>
std::atomic<int> counter(0);
void increment() {
for (int i = 0; i < 1000; ++i) {
counter++;
}
}
int main() {
std::thread t1(increment);
std::thread t2(increment);
t1.join();
t2.join();
std::cout << "Final counter value: " << counter.load() << std::endl;
return 0;
}
What is std::atomic?
C++ provides a way to perform operations on shared data without the need for explicit locks through the `std::atomic` type. This enables developers to safely manage shared variables in concurrent environments, ensuring that operations on these variables are performed atomically. Essentially, an atomic operation is one that completes fully or not at all, preventing any intermediate states from being observed.

Why Use std::atomic?
Using `std::atomic` offers several advantages:
- Performance: Typically, atomic operations are faster than acquiring and releasing mutex locks, as they eliminate the overhead associated with locking mechanisms.
- Safety: With atomic operations, you can avoid common concurrency problems like data races, where multiple threads read and write shared data simultaneously, leading to undefined behavior.
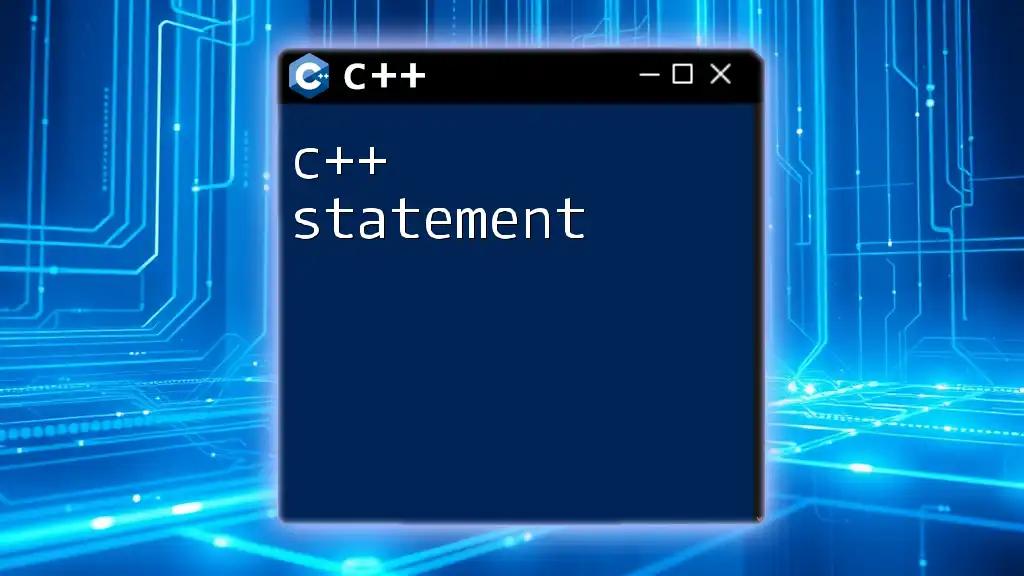
Understanding Atomic Operations
Defining Atomic Operations
Atomic operations guarantee that certain operations will complete without interruption. When multiple threads access the same memory location concurrently, they could interfere with each other's operations, leading to inconsistent results. By utilizing `std::atomic`, you ensure that these operations occur safely.
Key Characteristics of Atomic Variables
Creating lock-free and wait-free algorithms is where `std::atomic` shines.
- Lock-Free: At least one thread can make progress at any time, ensuring that not all threads are stalled.
- Wait-Free: Every thread can complete its operation in a bounded number of steps, providing the highest level of guarantee for responsiveness.
Memory Model in C++
The C++ memory model defines how operations on different threads interact with one another. `std::atomic` is designed to work seamlessly within this model, allowing for a more predictable interaction when multiple threads are involved.
Memory Ordering Overview
Memory ordering is crucial in atomic operations as it affects visibility and execution order of shared variables across threads. Familiarizing yourself with memory orderings will help you write more efficient and safer multi-threaded code.

Getting Started with std::atomic
Including the Header
To leverage the functionalities of `std::atomic`, include the appropriate header at the top of your file:
#include <atomic>
Creating Atomic Variables
You can declare atomic variables similarly to regular variables, but with the `std::atomic` template. For example:
std::atomic<int> counter(0);
Here, `counter` is an atomic integer initialized to zero, ready to be safely used in a multi-threaded context.
Supported Data Types
The `std::atomic` template can work with various data types.
Integral Types
You can use atomic types for the built-in integral types such as `int`, `long`, `bool`, etc.:
std::atomic<bool> flag(false);
std::atomic<long> balance(1000);
User-Defined Types
Although `std::atomic` primarily supports integral types, it can also be used with certain user-defined types. To ensure proper atomic access, the user-defined type must be trivially copyable.
For example, consider the following struct:
struct MyData {
int a;
int b;
};
std::atomic<MyData> myData;
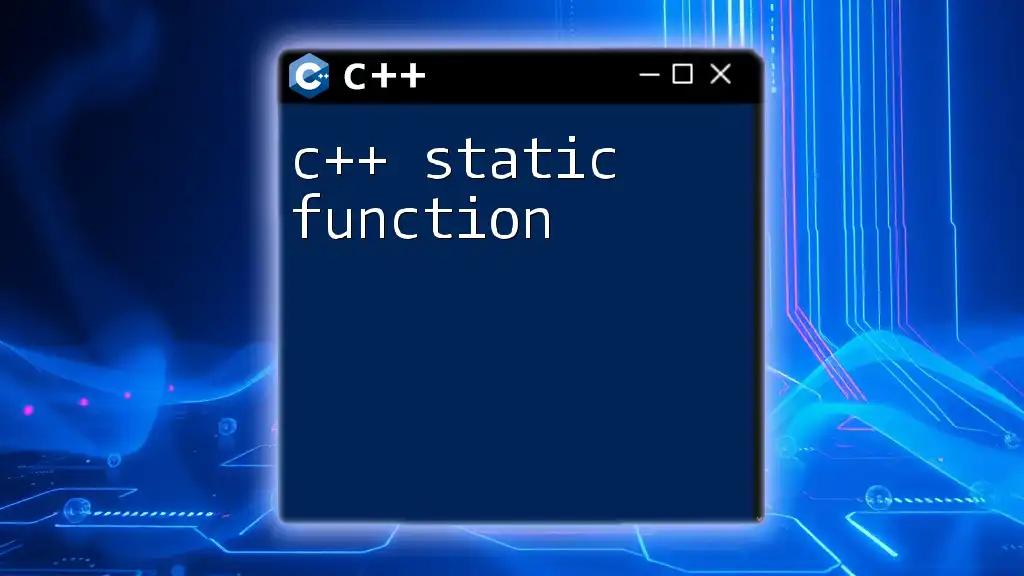
Commonly Used Operations
Load and Store Operations
Two essential operations in `std::atomic` are `load()` and `store()`.
-
Load retrieves the current value:
int value = counter.load();
-
Store sets a new value:
counter.store(value + 1);
Exchange Operations
The `exchange()` method allows you to set a new value while simultaneously returning the old one:
int oldValue = counter.exchange(10);
This operation is atomic and prevents any race conditions that might occur when updating the value.
Increment and Decrement
Atomic operations to increment or decrement values are also available through `fetch_add()` and `fetch_sub()`:
counter.fetch_add(1); // Atomically increments counter by 1
counter.fetch_sub(1); // Atomically decrements counter by 1
These operations ensure that no two threads will increment or decrement the value simultaneously, thereby preserving consistency.
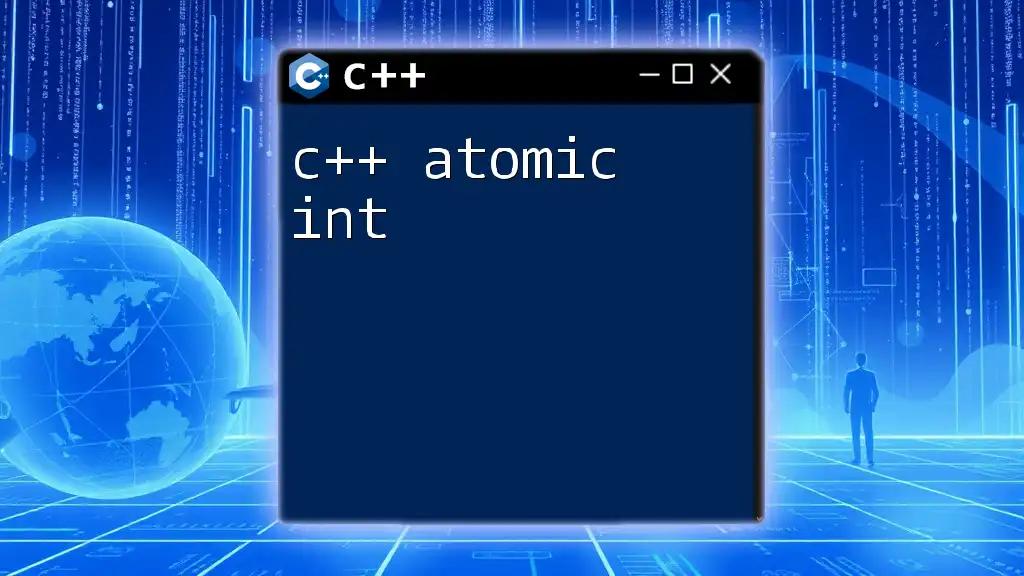
Memory Ordering
Understanding Memory Ordering
Memory ordering specifies how operations on atomic variables are seen by other threads. This is crucial in multi-threaded situations, where the order of operations may not be the same across different threads.
Types of Memory Orderings
The `std::atomic` template provides several options for memory ordering:
-
Sequential Consistency: This is the default option and ensures all memory operations are seen in a specific sequence across threads, eliminating race conditions.
-
Relaxed: Allows operations to be performed without concerns for the relative ordering with respect to other operations. Use it for performance, but only when you are sure it won’t cause issues.
-
Acquire and Release: These ensure that all prior operations are completed before a subsequent operation (acquire) and enforce all following operations to see the effects of a preceding operation (release).
For instance:
counter.load(std::memory_order_acquire);
counter.store(value, std::memory_order_release);
This guarantees that all reads and writes on the current thread happen before the atomic load/store.
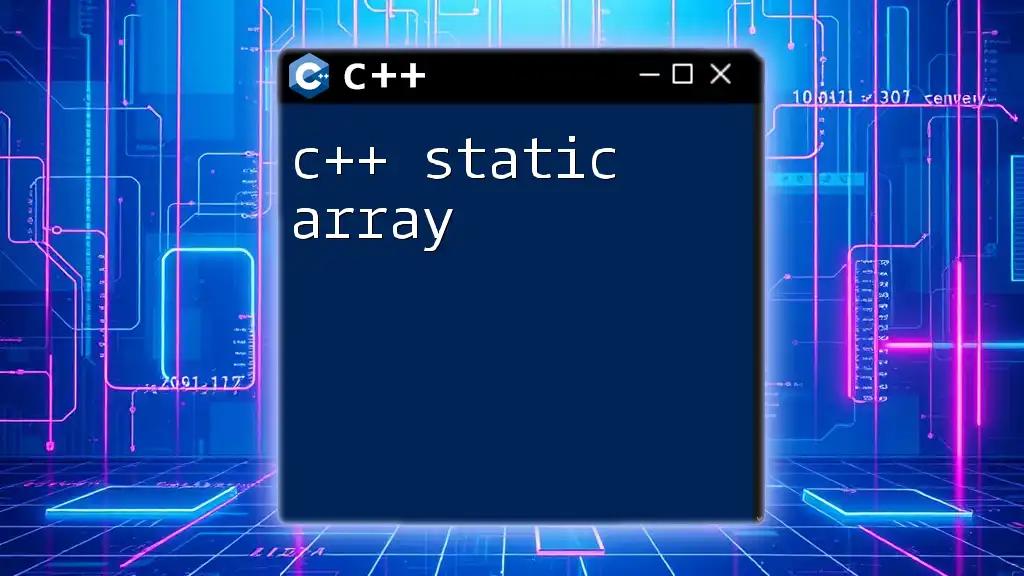
Use Cases for std::atomic
Example Use Case in Multi-threaded Applications
Consider a simple example where multiple threads are incrementing a shared counter. Here’s a basic illustration:
#include <iostream>
#include <thread>
#include <atomic>
std::atomic<int> counter(0);
void incrementCounter(std::atomic<int>& counter) {
for (int i = 0; i < 1000; ++i) {
counter.fetch_add(1);
}
}
int main() {
std::thread t1(incrementCounter, std::ref(counter));
std::thread t2(incrementCounter, std::ref(counter));
t1.join();
t2.join();
std::cout << "Final counter value: " << counter << std::endl;
return 0;
}
This simple program creates two threads, each incrementing the shared `counter` atomic variable 1000 times, demonstrating how `std::atomic` allows safe manipulation of shared resources.
Best Practices for Using std::atomic
When using `std::atomic`, it's essential to understand when to use it over mutexes.
- Use atomics when you have simple data types that can be modified independently.
- For complex manipulations, consider using mutexes to prevent potential race conditions.
- Avoid mixing atomic and non-atomic accesses to the same variable or data structure; it can lead to unexpected behavior.

Conclusion
In summary, `c++ std atomic` provides a vital tool for managing shared data in multi-threaded applications. By understanding atomic operations, memory orderings, and best practices, you can create efficient, safe, and clean concurrent applications. Whether you're working with simple counters or more complex data structures, leveraging the power of `std::atomic` will significantly enhance your C++ programming for multi-threading scenarios.

FAQs
What is the difference between std::atomic and mutex?
While mutexes provide a mechanism to lock resources ensuring only one thread accesses them at a time, `std::atomic` allows multiple threads to access a variable simultaneously with atomic guarantees. Mutexes incur more overhead than atomic operations.
Can std::atomic be used with complex data types?
`std::atomic` can be used with user-defined types if those types are trivially copyable. This means they must only contain types that can be copied using simple memory operations without side effects.
What are the limitations of using std::atomic?
The limitations of `std::atomic` primarily concern its use with data types. Complex data structures and those with internal state management should use mutexes or other synchronization techniques to ensure thread safety. Additionally, there's a learning curve in understanding memory orderings and their implications.