`std::async` in C++ is a standard library function that allows you to run tasks asynchronously, returning a `std::future` that can be used to fetch the result when it's ready.
#include <iostream>
#include <future>
int main() {
// Launch a task asynchronously
std::future<int> result = std::async([]() {
std::this_thread::sleep_for(std::chrono::seconds(2)); // Simulate work
return 42;
});
// Do some other work while the task runs
std::cout << "Doing other work..." << std::endl;
// Get the result of the async task
std::cout << "Result: " << result.get() << std::endl; // Blocks until the result is ready
return 0;
}
What is std::async?
Definition and Purpose
`std::async` is a powerful feature in C++ introduced in C++11 that allows developers to run functions asynchronously, meaning that the program can continue executing other tasks while waiting for the result of the asynchronous function call. This makes `std::async` an excellent tool for enhancing performance, responsiveness, and scalability in applications, particularly when dealing with time-consuming operations that can be executed in the background.
Key Features of std::async
- Convenience: One of the standout features of `std::async` is how it abstracts away thread management. By using this function, developers do not have to directly deal with thread creation, synchronization, or destruction.
- Flexibility: `std::async` can accept multiple callable types, including normal functions, function pointers, and lambda expressions, providing versatility in its use.
- Return Types: When you call `std::async`, it returns an object of type `std::future`, which allows you to get the result of the asynchronous computation after it's finished.
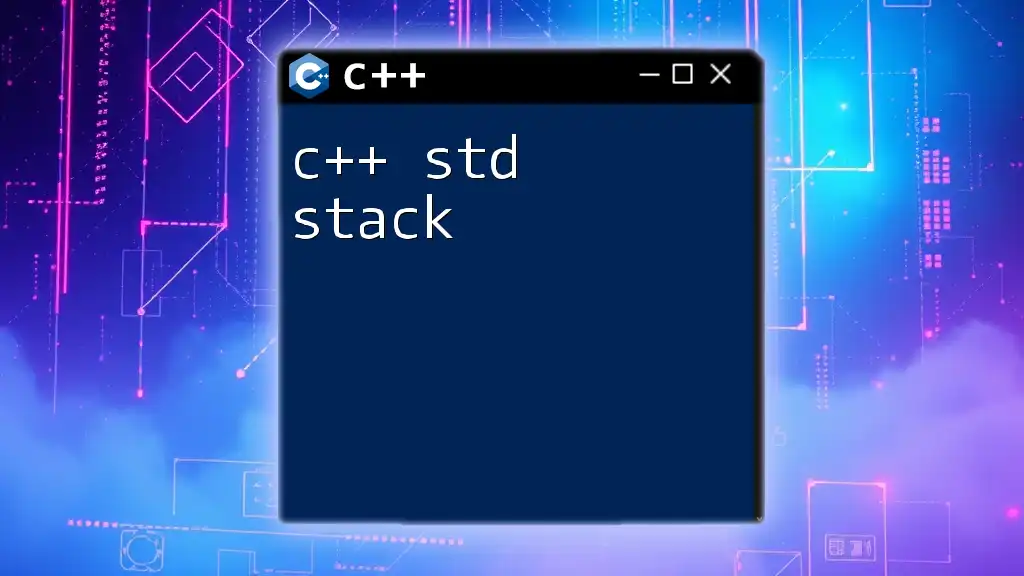
How to Use std::async
Basic Syntax of std::async
The basic syntax for invoking `std::async` is as follows:
std::future<ReturnType> result = std::async(std::launch::policy, Callable, args...);
- ReturnType: This is the type that will be returned by the callable object.
- launch::policy: This optional parameter specifies how the asynchronous task should be executed.
- Callable: This can be a function pointer, a functor, or a lambda expression.
- args: Additional arguments that are passed to the callable.
Launch Policies
The power of `std::async` comes from its ability to choose between two main launch policies:
Explanation of Launch Policies
- std::launch::async: Requests the function to execute asynchronously and immediately. This can create a new thread for executing the function.
- std::launch::deferred: Indicates that the function will only be run when the result is explicitly requested (i.e., via `get()`). This can save resources if the computation may not be necessary.
- You can also combine both policies allowing the system to decide which to use based on current conditions.
Code Example: Basic Usage
Here’s a simple example demonstrating `std::async` in action:
#include <iostream>
#include <future>
#include <chrono>
int asyncFunction() {
std::this_thread::sleep_for(std::chrono::seconds(2));
return 42;
}
int main() {
std::future<int> result = std::async(std::launch::async, asyncFunction);
std::cout << "Waiting for result..." << std::endl;
std::cout << "Result: " << result.get() << std::endl;
return 0;
}
In this example, the program starts `asyncFunction()` asynchronously, allowing the main thread to continue executing. The `get()` function on the `future` object will block if the result is not ready yet, but in this case, it will wait for the `asyncFunction` to complete and return the result.
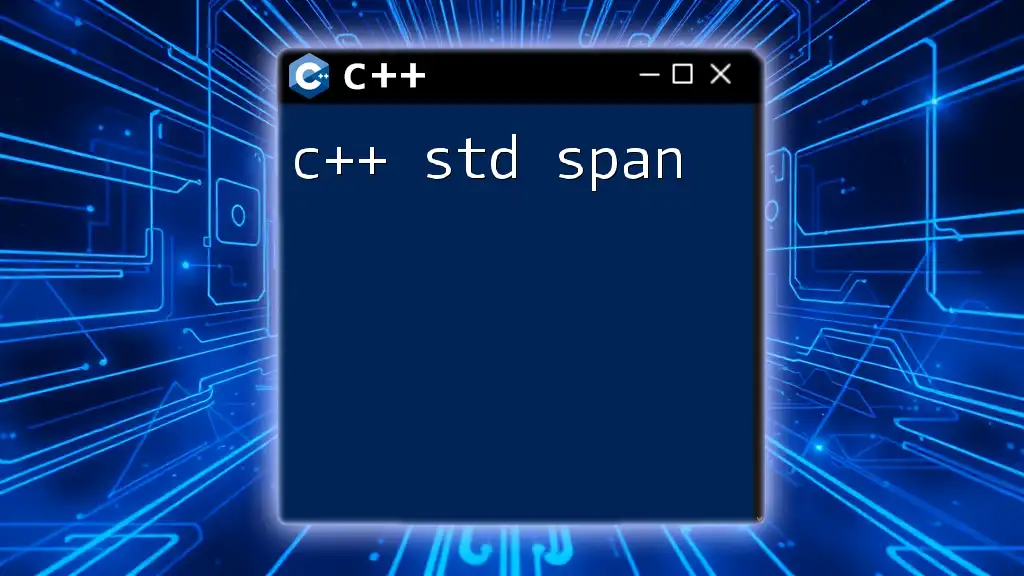
Benefits of Using std::async
Simplified Code Structure
Using `std::async` can lead to code that is easier to read and understand. Instead of managing threads manually, you can focus on defining the work to be done and where the results will go, which consequently reduces the chances of threading bugs.
Handling Long-Running Operations
Another significant advantage is the ability to perform long-running tasks without blocking the main thread. For instance, in a user interface application, if a background calculation takes a few seconds, `std::async` can perform that while keeping the application responsive to user input.
Exception Handling with Futures
An essential feature of `std::future` is its ability to capture exceptions thrown by asynchronous operations.
Consider the following code snippet:
std::future<void> asyncOp = std::async([] {
throw std::runtime_error("An error occurred");
});
try {
asyncOp.get();
} catch (const std::exception& e) {
std::cout << e.what() << std::endl;
}
Here, if the asynchronous operation throws an exception, it can be caught and handled in the main thread, enabling robust error management.
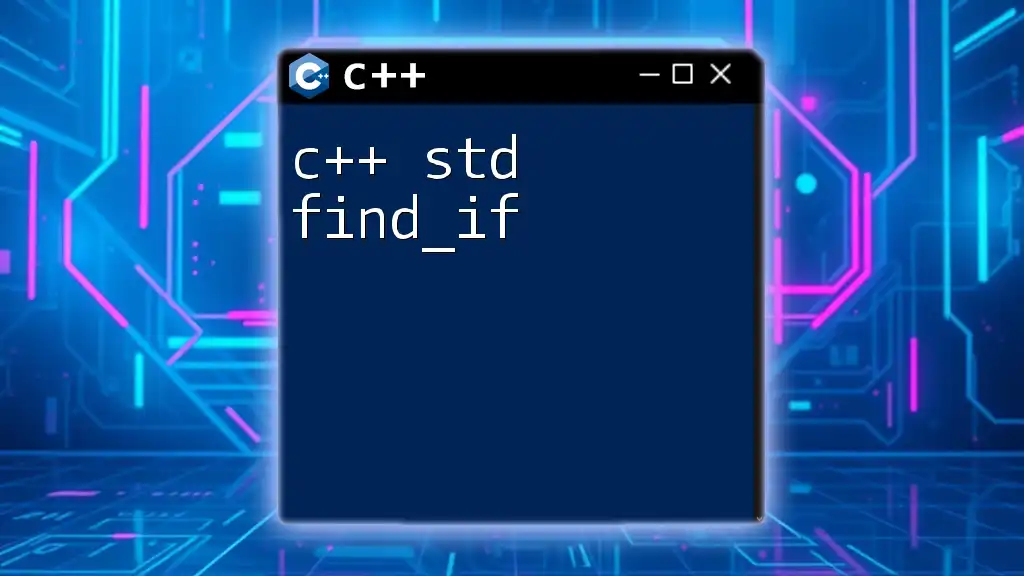
Common Use Cases for std::async
Background Tasks
`std::async` is ideal for running background tasks where immediate results are not required. For example, loading resources in the background while maintaining application performance can dramatically improve user experience.
Parallelizing Computations
When you have a heavy computational task that can be divided into smaller tasks, `std::async` can be a perfect fit.
Here’s an example of summing an array of integers asynchronously:
#include <vector>
#include <numeric>
#include <iostream>
#include <future>
int sum_array(const std::vector<int>& vec) {
return std::accumulate(vec.begin(), vec.end(), 0);
}
int main() {
std::vector<int> data = {1, 2, 3, 4, 5};
auto futureSum = std::async(std::launch::async, sum_array, data);
std::cout << "Sum: " << futureSum.get() << std::endl;
return 0;
}
In this example, the summation of the vector happens in a separate thread. By using `std::async`, we offload this computation while allowing the main thread to remain available for other tasks.
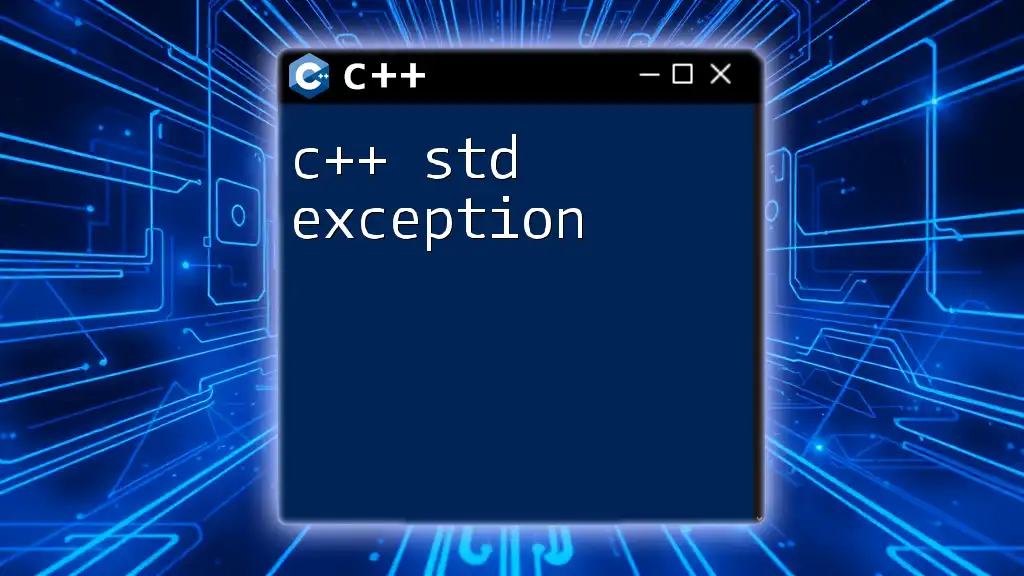
Things to Keep in Mind
Performance Considerations
While `std::async` simplifies asynchronous programming, it is essential to be aware of its performance implications. The overhead of managing threads may not always pay off, especially for lightweight tasks. Always consider whether the complexity introduced by multithreading is justified for the scenario at hand.
Limitations of std::async
There are a few important limitations to consider. For instance, if you call `get()` on a `std::future` object that was created with `std::launch::deferred`, the underlying callable will execute at that moment, which may not be intended. Additionally, developers must be cautious about the lifespan of the objects passed to an asynchronous call to prevent dangling references.
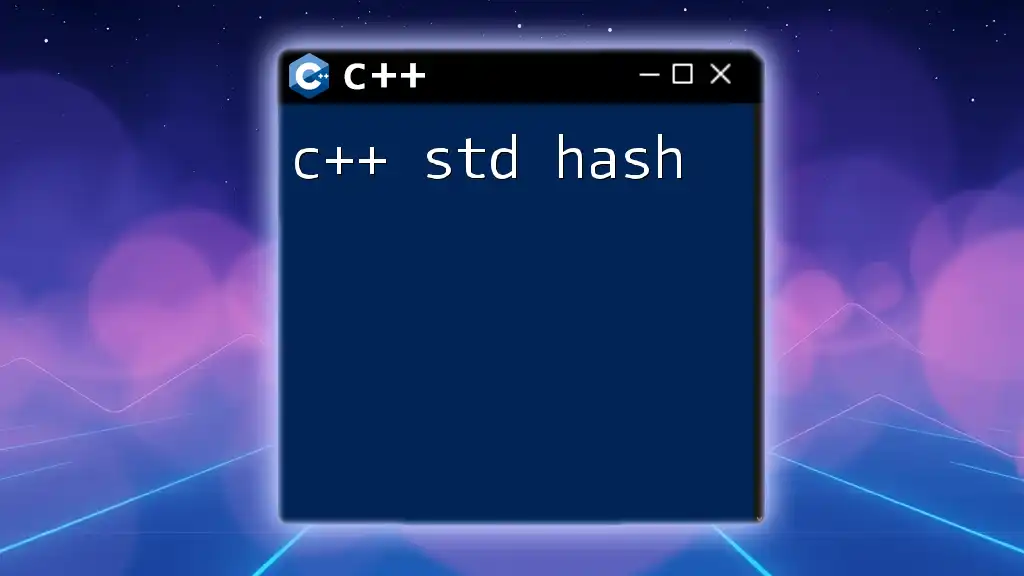
Best Practices
Where to Use std::async
When considering where to apply `std::async`, you should aim for scenarios involving:
- I/O operations that can operate independently of the main thread.
- Long computations that do not require immediate results.
- Situations where overall efficiency can improve with parallel execution.
Tools and Resources
To enhance your experience with `std::async`, consider exploring libraries such as Intel TBB (Threading Building Blocks) or Boost, which provide more advanced threading mechanisms, including thread pools that can complement `std::async` for greater control over thread management.
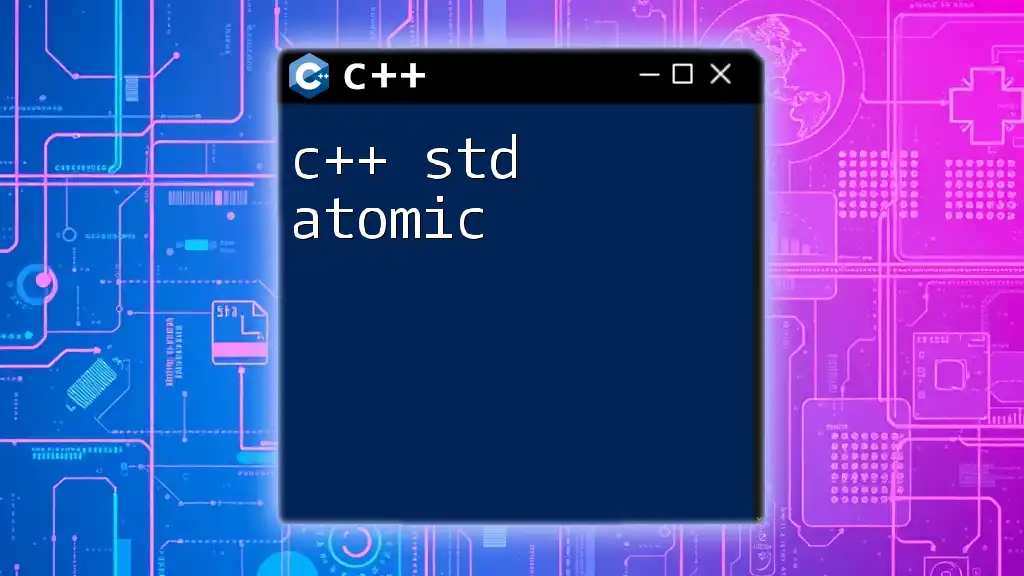
Conclusion
In conclusion, `C++ std::async` is a robust tool for achieving concurrency without the hassle of manual thread management. Through its intuitive interface and support for asynchronous operations, developers can create responsive applications that run efficiently while handling resource-intensive tasks in the background. As you get more familiar with `std::async`, you'll find it an invaluable asset in your C++ programming toolkit.
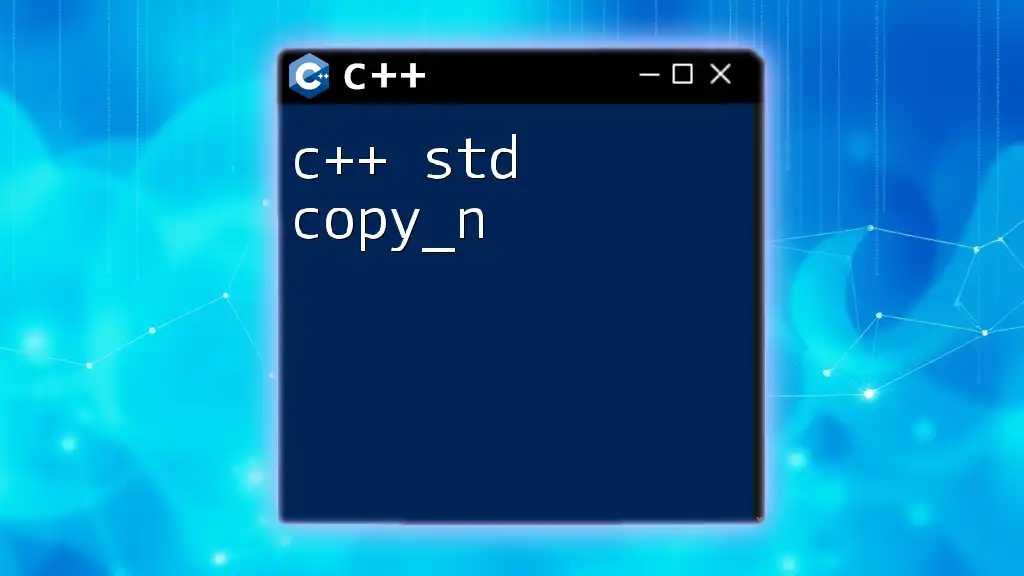
Call to Action
If you found this guide on `C++ std::async` helpful, subscribe for more insights into C++ programming and concurrency techniques. We invite you to join our community for collaborative learning and to dive deeper into the world of advanced C++ features!