`std::apply` is a C++ standard library function that unpacks a tuple of arguments and calls a callable object (like a function or a lambda) with those arguments, enabling concise and flexible function calls.
Here’s a simple example:
#include <iostream>
#include <tuple>
#include <functional>
void printSum(int a, int b) {
std::cout << "The sum is: " << (a + b) << std::endl;
}
int main() {
std::tuple<int, int> args(3, 4);
std::apply(printSum, args); // Calls printSum(3, 4)
return 0;
}
What is std::apply?
The `std::apply` function in C++ is a utility designed to simplify the process of applying callable objects (like functions or functors) to the elements of a tuple. With C++ acquiring new features with each revision, `std::apply` is a part of C++17, providing a robust way to work with tuple-like objects conveniently.
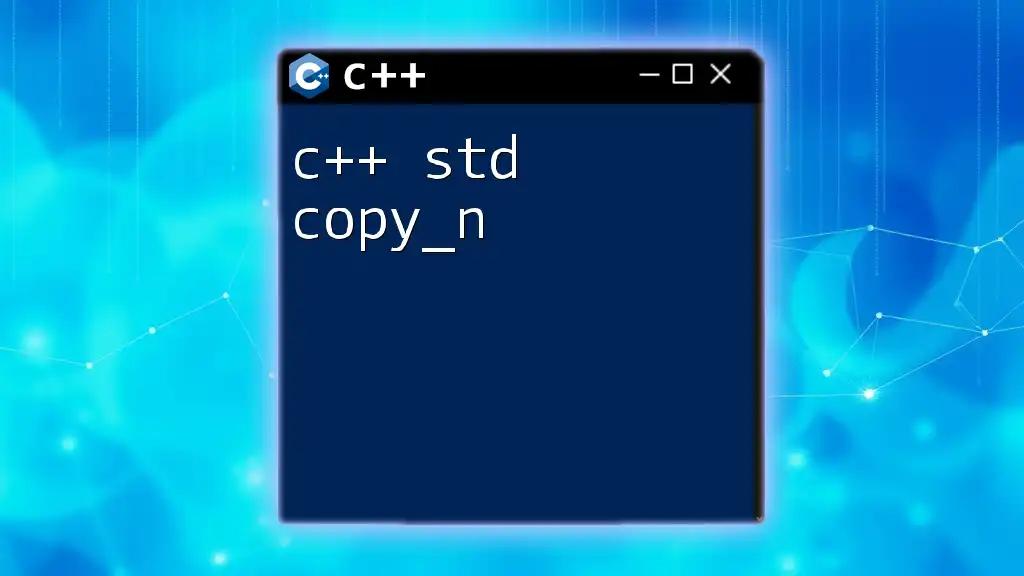
Why Use std::apply?
Using `std::apply` improves code readability and maintainability. It allows developers to avoid boilerplate code when calling functions with multiple parameters stored in a tuple. By leveraging this feature, you can focus more on your application's logic rather than managing the complexity of argument passing manually.
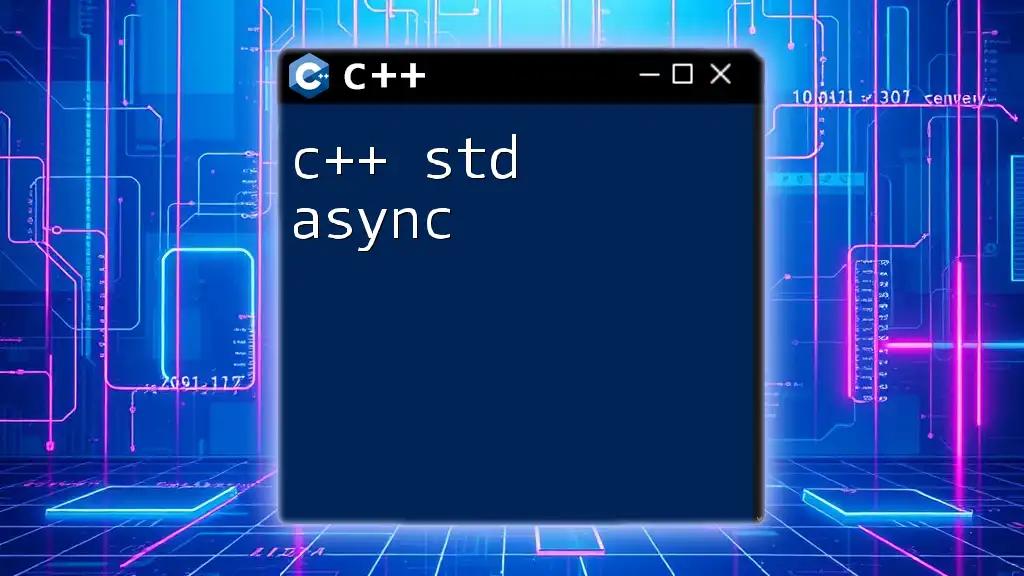
Understanding Tuples and Callable Objects in C++
What are Tuples?
A tuple in C++ is a fixed-size collection of elements, where each element can be of different types. Consider it as a lightweight data structure that helps group different data types together.
Example of Tuples in C++:
#include <tuple>
#include <iostream>
std::tuple<int, double, std::string> myTuple(1, 3.14, "Hello");
Callable Objects in C++
In C++, a callable object can be any entity that can be "called" like a function. This includes:
- Functions
- Function pointers
- Functors (objects that overload the `operator()`)
The versatility of callable objects allows you to write more abstract and reusable code.
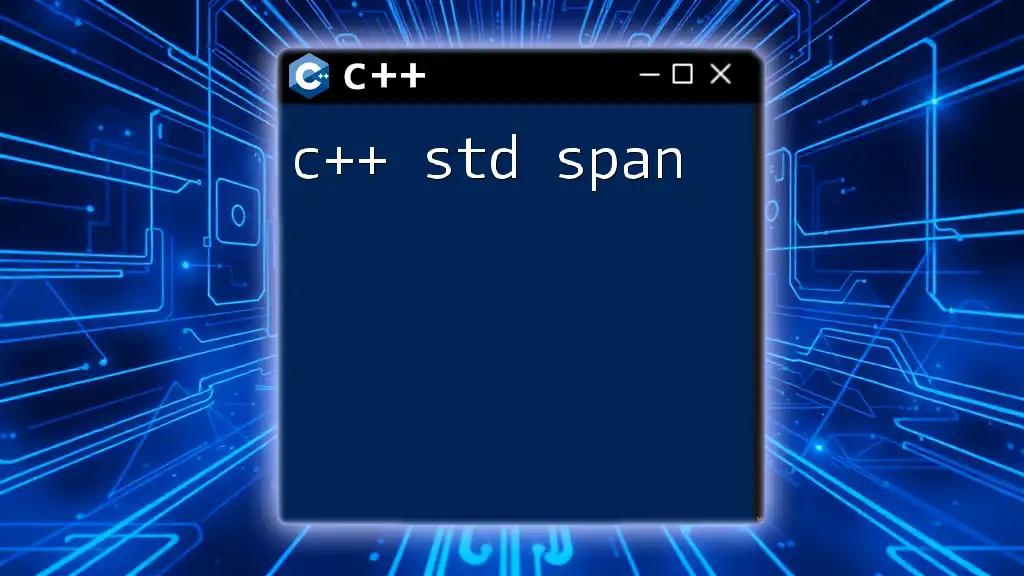
The Syntax of std::apply
Basic Syntax Explanation
The fundamental syntax for using `std::apply` is as follows:
std::apply(Function, Tuple);
- Function: The callable object you want to apply.
- Tuple: The tuple containing arguments to be passed to the callable object.
Header File Requirement
To use `std::apply`, ensure you include the appropriate header file:
#include <tuple>
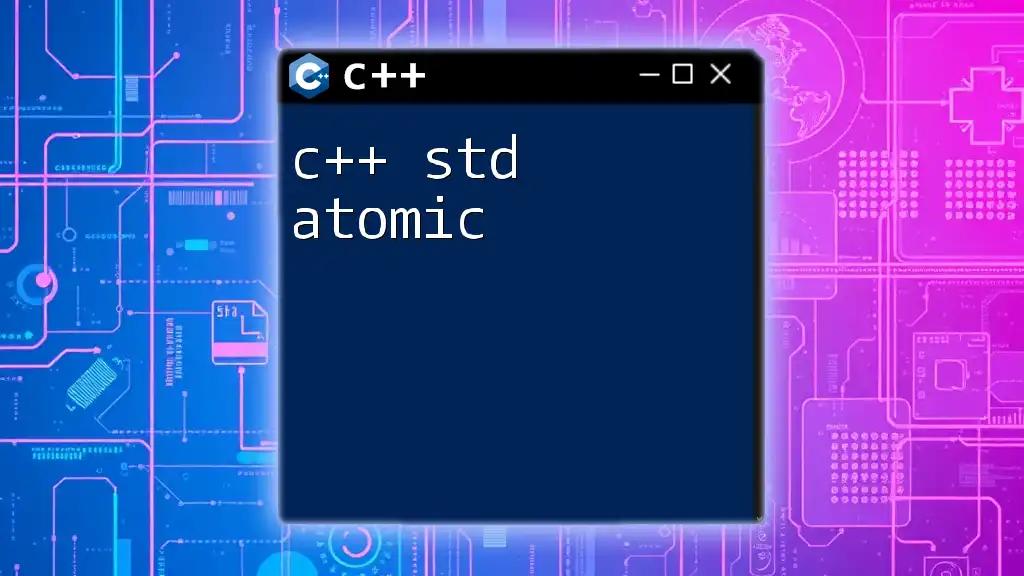
Deep Dive into std::apply Mechanics
How std::apply Works
The magic of `std::apply` lies in its ability to unpack tuple elements and pass them as separate arguments to the specified callable object. Internally, it uses template magic to handle different callable types and argument counts, allowing it to be both flexible and type-safe.
Example of Using std::apply
Let’s illustrate its utility with a simple example:
#include <iostream>
#include <tuple>
#include <functional>
void printSum(int a, int b) {
std::cout << "Sum: " << (a + b) << std::endl;
}
int main() {
std::tuple<int, int> numbers(3, 4);
std::apply(printSum, numbers);
return 0;
}
This code snippet defines a function `printSum`, which accepts two integers. By using `std::apply`, we pass the tuple `numbers` directly into the function, eliminating the need for manual unpacking. The output will be:
Sum: 7
Practical Applications of std::apply
Real-World Use Cases
`std::apply` shines especially in scenarios where you frequently work with tuple types. It enables you to streamlines function calls that would otherwise require cumbersome code to extract values from the tuple.
Use Case Example: Database Operations
Consider a situation where you need to insert data into a database. Using tupples, you can structure your database entries neatly and apply them directly:
#include <iostream>
#include <tuple>
#include <string>
void insertIntoDatabase(std::string table, int id) {
std::cout << "Inserting ID: " << id << " into table: " << table << std::endl;
}
int main() {
std::tuple<std::string, int> dbEntry("Users", 42);
std::apply(insertIntoDatabase, dbEntry);
return 0;
}
In this example, we define a function `insertIntoDatabase` that takes a table name and an ID. By using `std::apply`, we streamline the process of inserting database entries.
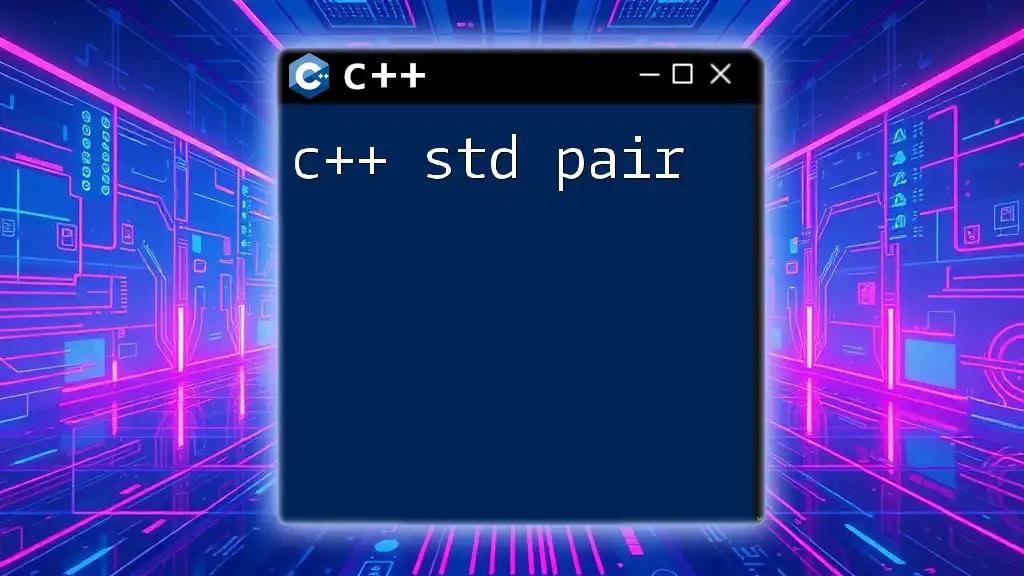
Comparison: std::apply vs Traditional Methods
Using std::apply vs Manual Argument Passing
The traditional method of passing arguments involves manually unpacking the tuple, which can make your code cumbersome and error-prone. When using `std::apply`, you gain:
- Conciseness: Less boilerplate code to write.
- Type Safety: Less room for mismatched argument types.
Performance Considerations
While `std::apply` offers many advantages, it is essential to consider performance implications when used extensively in performance-critical sections of code. However, for the vast majority of applications, the benefits in clarity and maintenance far outweigh the potential costs.

Best Practices with std::apply
When to Use std::apply
- Use std::apply when working frequently with tuples.
- When functions require a varying number of parameters that can be organized as a tuple for clarity.
Debugging Tips
Common issues include:
- Mismatched tuple size and argument count.
- Incorrect tuple element types.
Code Maintainability
Utilizing `std::apply` leads to clearer, more maintainable code. Whenever your logic involves tuples, consider adopting `std::apply` for enhanced clarity.
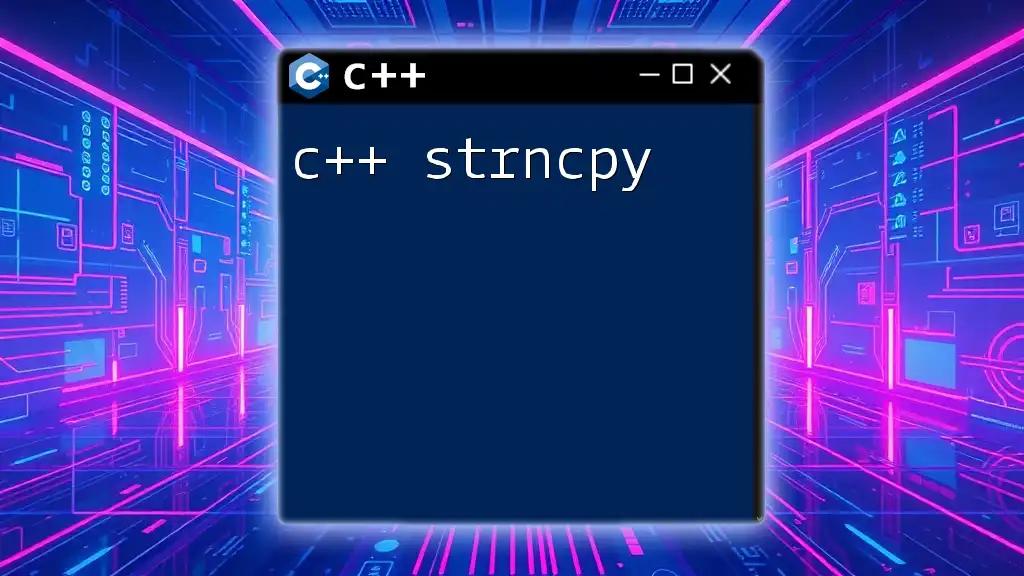
Conclusion
In summary, `std::apply` is a powerful feature in C++ that simplifies the interaction between tuples and callable objects. The ease of applying functions directly to tuples allows for cleaner, more comprehensible code, essential in modern C++ development. Experimenting with this feature can elevate your coding practices and enhance the performance of your applications.
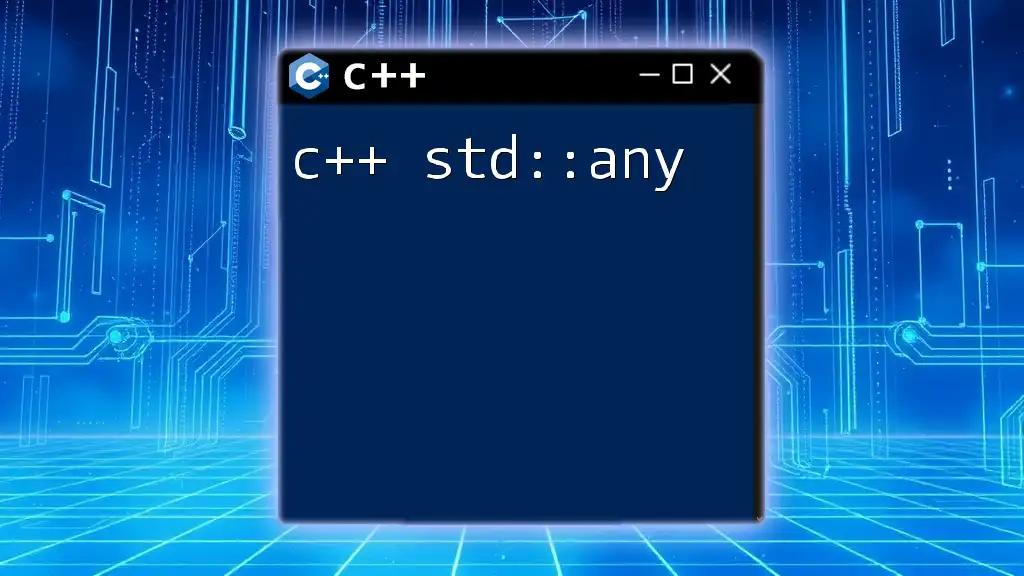
Additional Resources
For further learning, check out the official documentation on `std::apply`, and explore online coding platforms where you can practice this feature hands-on. Embrace this efficient tool to elevate your proficiency in C++.