In C++, `std::pair` is a simple container that stores two heterogeneous objects as a single unit, which can be useful for returning multiple values from a function or storing related data pairs.
Here’s a code snippet demonstrating the usage of `std::pair`:
#include <iostream>
#include <utility> // for std::pair
int main() {
std::pair<int, std::string> student(1, "John Doe");
std::cout << "ID: " << student.first << ", Name: " << student.second << std::endl;
return 0;
}
Understanding std::pair in C++
What is a std::pair?
The std::pair in C++ is a part of the Standard Library, specifically defined in the `<utility>` header. It is a simple data structure that combines two values (which can be of different types) into a single unit. The values stored in a std::pair can be accessed and manipulated easily and can serve as a lightweight way to group two objects together without creating a full custom struct or class.
Syntax of std::pair
To declare a std::pair, you use the following syntax:
std::pair<Type1, Type2>
Where `Type1` is the type of the first element and `Type2` is the type of the second element. For example, `std::pair<int, std::string>` declares a pair where the first element is an integer and the second is a string.
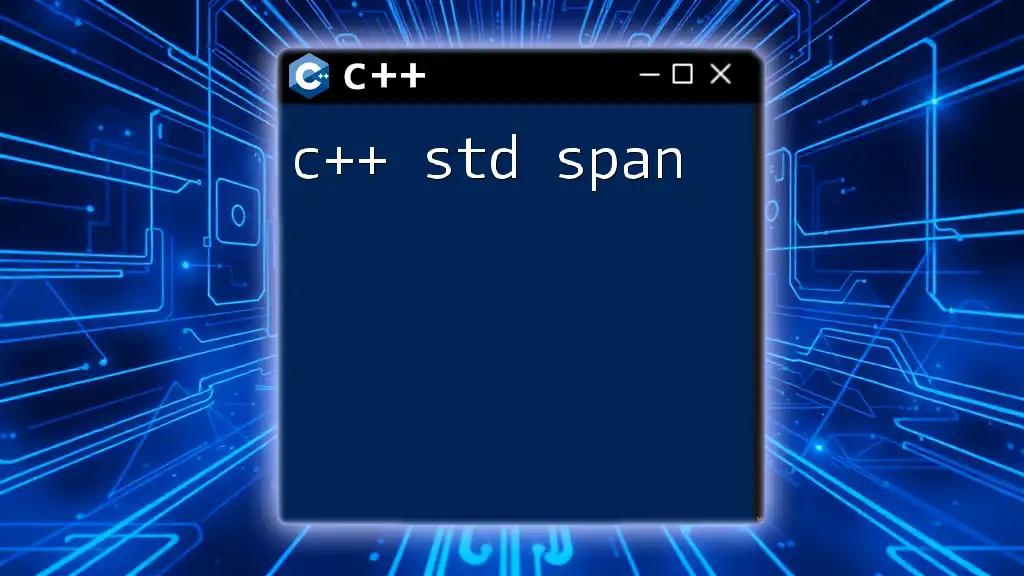
Creating and Initializing std::pair
Creating a std::pair
You can create a std::pair using two methods: the default constructor or the parameterized constructor.
-
Default Constructor: This creates a pair where both elements are initialized to their default values.
std::pair<int, std::string> p1;
-
Parameterized Constructor: Directly initializes the pair with the specified values.
std::pair<int, std::string> p2(1, "Hello");
Initializing std::pair
You can also initialize a std::pair using `std::make_pair`, which deduces the types of the elements automatically. This method is convenient and prevents type mismatch errors.
auto p3 = std::make_pair(2, "World");

Accessing Elements in std::pair
Using Public Data Members
The std::pair class has two public data members: `first` and `second`, which allow direct access to the contained values.
std::cout << "First: " << p2.first << ", Second: " << p2.second << std::endl;
In this example, you will print the values contained in the pair `p2`.
Using std::get()
Alternatively, you can use the `std::get` function to access the elements of the pair using their index. This is a more type-safe way to retrieve the elements.
std::cout << "First: " << std::get<0>(p3) << ", Second: " << std::get<1>(p3) << std::endl;
By specifying the index, you can directly access the desired element while maintaining type safety.

Modifying Elements in std::pair
Changing Values
You can easily modify the values of a std::pair after its creation. Both elements, `first` and `second`, can be assigned new values.
p1.first = 10;
p1.second = "Updated";
This flexibility allows you to maintain and update pairs as your program executes.
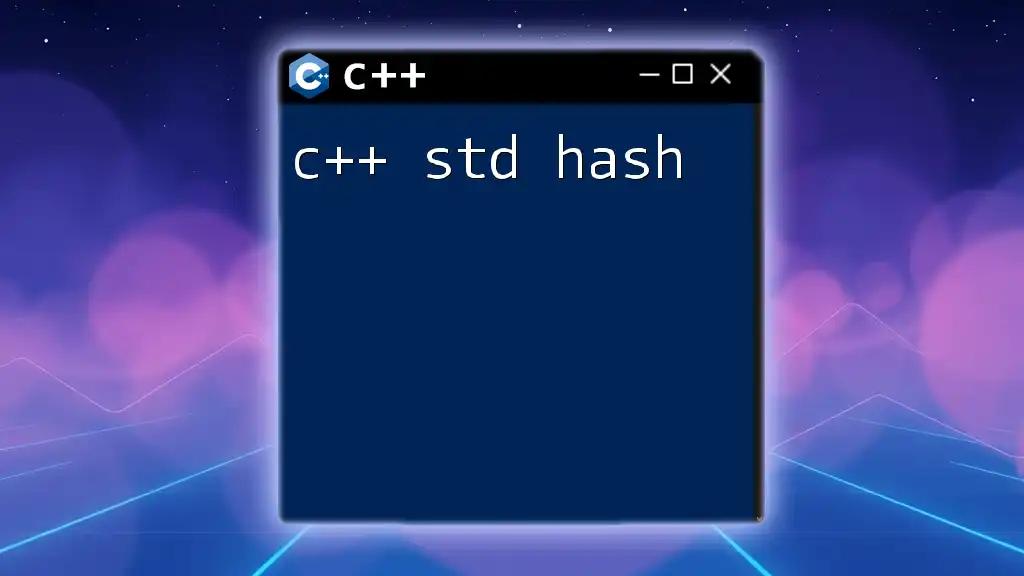
Using std::pair in Functions
Passing std::pair to Functions
You can pass std::pair objects to functions, enabling you to handle multiple related values elegantly.
void printPair(std::pair<int, std::string> p) {
std::cout << p.first << ", " << p.second << std::endl;
}
This function will print the contents of the pair passed to it.
Returning std::pair from Functions
Using std::pair as a return type from functions is beneficial when you need to return two related values.
std::pair<int, int> add(int a, int b) {
return std::make_pair(a, b);
}
Here, the `add` function returns a pair containing the arguments provided, encapsulating two outputs within a single return type.
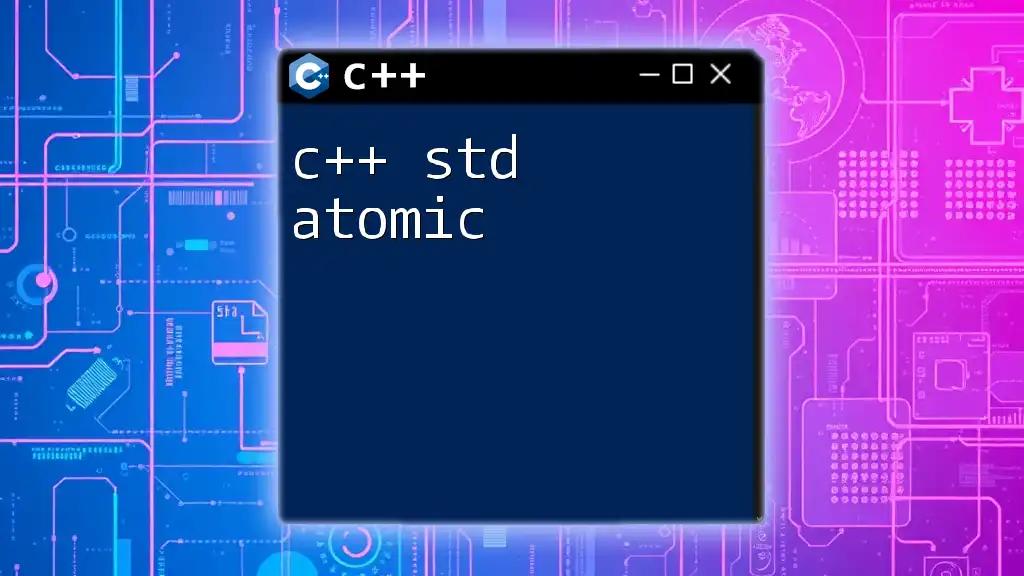
std::pair in Containers
Using std::pair with STL Containers
std::pair is particularly useful when working with Standard Template Library (STL) containers, such as maps.
std::map<int, std::string> myMap;
myMap.insert(std::make_pair(1, "One"));
In this example, `std::pair` allows you to deposit both a key-value pair into the map succinctly.
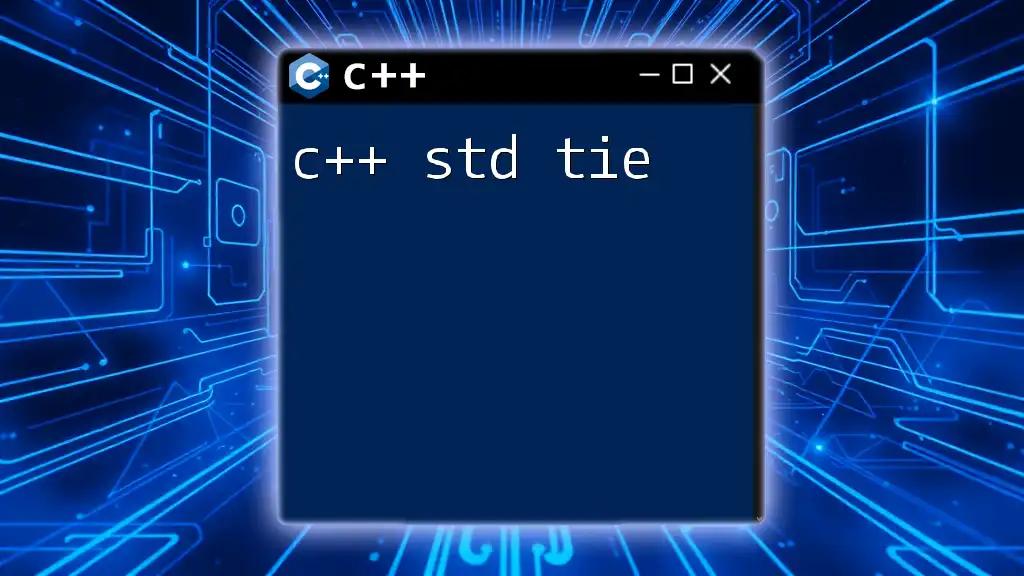
Common Use Cases for std::pair
Pairing Related Data
std::pair excels in scenarios where you need to associate two pieces of related data together. For instance, when implementing a graph, you can store an edge's weight and the two vertex identifiers in a pair.
Handling Return Values
Returning multiple values from a function can be cumbersome with standard return types. Instead, using std::pair can simplify this process. For example, if a function needs to return the coordinates of a point (X, Y), using std::pair enables a more structured approach.

Comparisons with Other Data Structures
std::pair vs std::tuple
While std::pair can hold only two elements, std::tuple enables the storage of multiple elements of various types. Use std::pair when you only need two related values and rely on std::tuple when dealing with larger sets.
std::pair vs Structs
When needing a simple way to group together two related variables, std::pair is often preferred over custom structs for its succinctness and ease of use. However, if more contextual information is required, a struct would be a better choice.
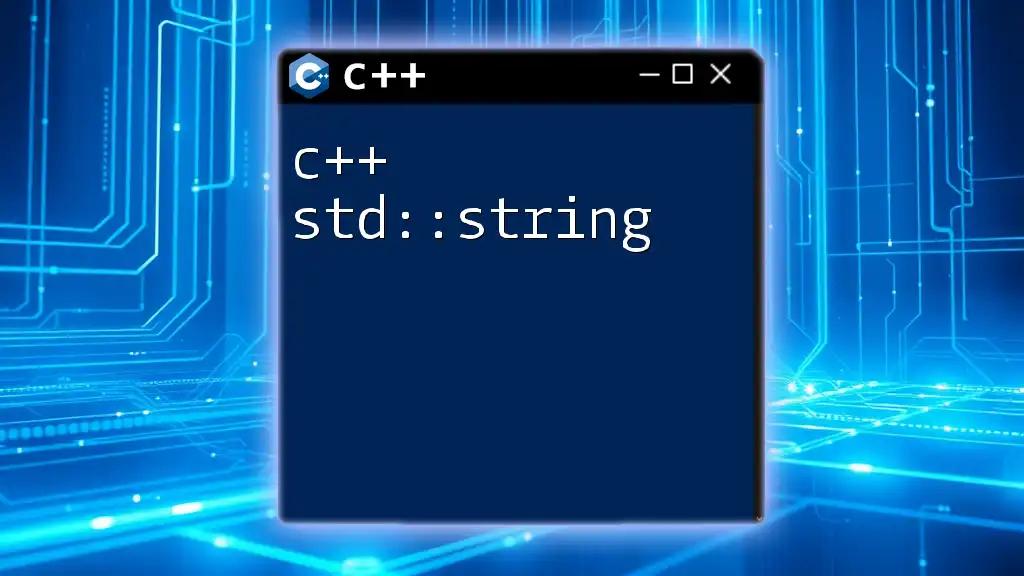
Conclusion
In summary, the c++ std pair is an invaluable member of the Standard Library, simplifying the grouping of two related values. Its ease of use, clear syntax, and flexibility make it ideal for developers looking to streamline their code without unnecessary complexity. Feel encouraged to incorporate std::pair into your own projects and explore its myriad advantages. Practice coding with these examples and deepen your understanding of this useful C++ feature.

Additional Resources
For further exploration, consider reading more about C++ functionalities, experimenting with online compilers, or referencing the official C++ documentation on std::pair to expand your knowledge even further.