In C++, `std::tie` is a utility function that allows you to unpack values from a tuple or a pair into individual variables, simplifying the extraction of multiple returned values.
Here’s a code snippet demonstrating its usage:
#include <iostream>
#include <tuple>
std::tuple<int, double> getValues() {
return std::make_tuple(5, 3.14);
}
int main() {
int x;
double y;
std::tie(x, y) = getValues();
std::cout << "x: " << x << ", y: " << y << std::endl;
return 0;
}
Understanding the Purpose of std::tie
`std::tie` is a powerful utility in C++ that allows you to create tuple-like structures while maintaining direct references to original variables. This function is particularly useful when you want to unpack or obtain multiple values from a function call without the need for creating a separate tuple. It collaborates beautifully with C++'s standard library, making your code cleaner and often more efficient by avoiding unnecessary copying of values.

What are Tuples?
In C++, tuples are fixed-size collections of heterogeneous values, defined in the `<tuple>` header. They allow you to group multiple items into a single entity while preserving their types. For example, you can define a tuple representing a person's details that includes an integer and a string:
std::tuple<int, std::string> person = std::make_tuple(30, "Alice");
This tuple stores the age and name of the person in a structured manner. Tuples are very handy when you need to return multiple values from a function, keeping your code organized.
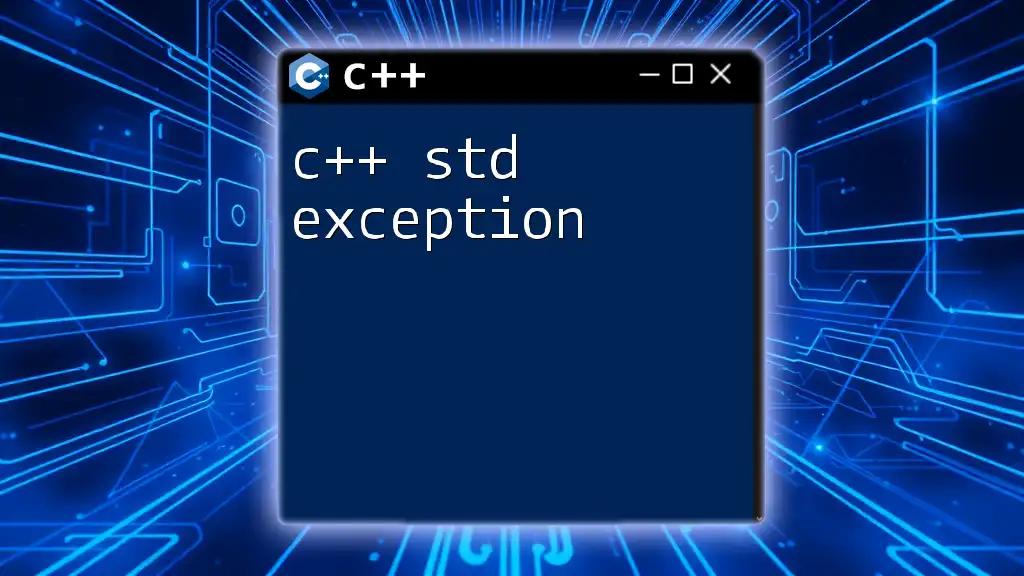
How std::tie Differs from std::tuple
While tuples provide a way to bundle multiple values together, `std::tie` has a unique advantage: it creates references to existing variables rather than producing a new copy of the data. This is particularly beneficial when performance matters, as it avoids the overhead associated with copying large objects.
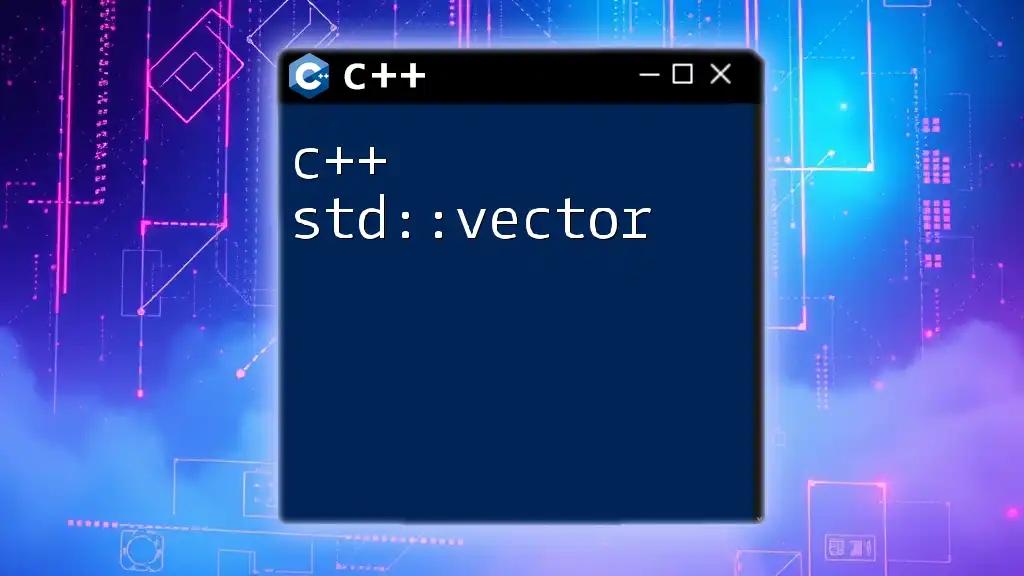
Basic Syntax of std::tie
The syntax for `std::tie` is straightforward; it typically follows this structure:
std::tie(variable1, variable2) = std::make_tuple(value1, value2);
Here, `variable1` and `variable2` will be assigned the values from the tuple, effectively "unpacking" it.
Returning Multiple Values from Functions
Using `std::tie` is a perfect way to return multiple values from a function succinctly. Here's a clear example:
std::tuple<int, double> func() {
return std::make_tuple(100, 5.5);
}
int main() {
int x;
double y;
std::tie(x, y) = func();
// x will be 100, y will be 5.5
}
In this example, we create a function that returns a tuple. By using `std::tie`, we can directly unpack the tuple’s values into our variables `x` and `y`, improving code clarity and conciseness.
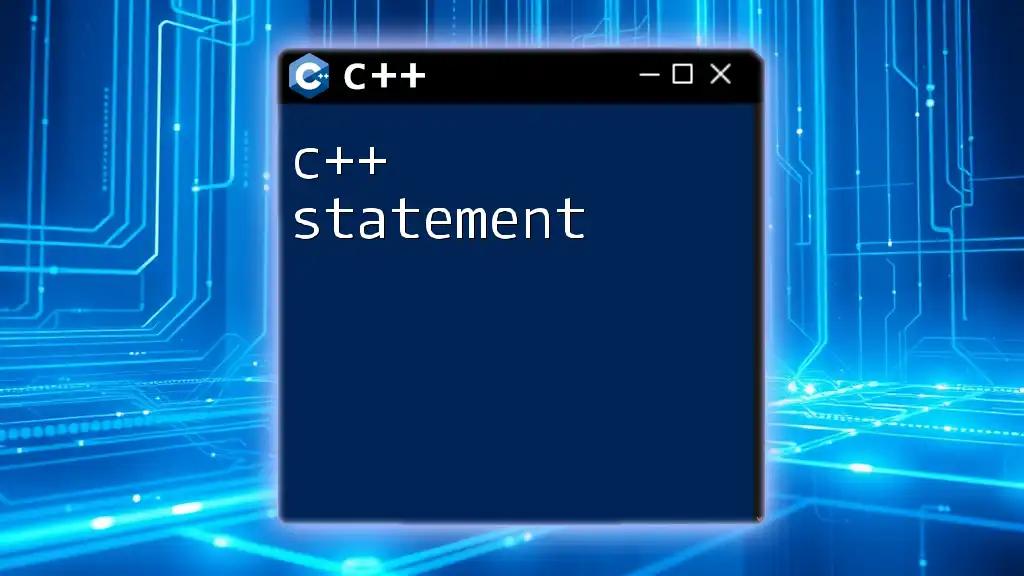
Unpacking Values with std::tie
One of the primary uses of `std::tie` is to unpack values cleanly. Consider a situation where you have a tuple containing an employee's ID and name:
std::tuple<int, std::string> employee = std::make_tuple(284, "John Doe");
int id;
std::string name;
std::tie(id, name) = employee;
In the above code, we unpack the tuple `employee` into the `id` and `name` variables. This method keeps your code organized as it directly maps the tuple's elements to meaningful variable names.

Conditional Assignments with std::tie
Another scenario where `std::tie` shines is with conditional assignments. For instance:
bool success;
int result;
std::tie(success, result) = performOperation();
Here, `performOperation()` may return a tuple where the first element indicates whether the operation was successful, and the second element gives the resulting value. `std::tie` lets you assign both variables in a single line, enhancing code readability.
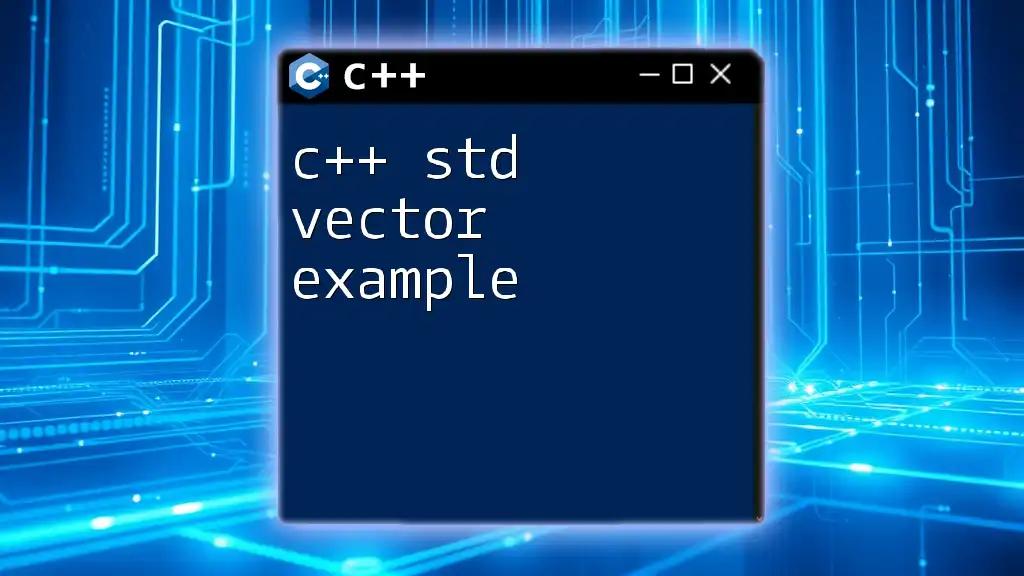
Efficiency of std::tie
Efficiency is a crucial consideration in programming. When using `std::tie`, there’s a significant reduction in overhead since it deals with references rather than copies. This efficiency becomes paramount in performance-critical applications, particularly when handling complex data structures or large objects.
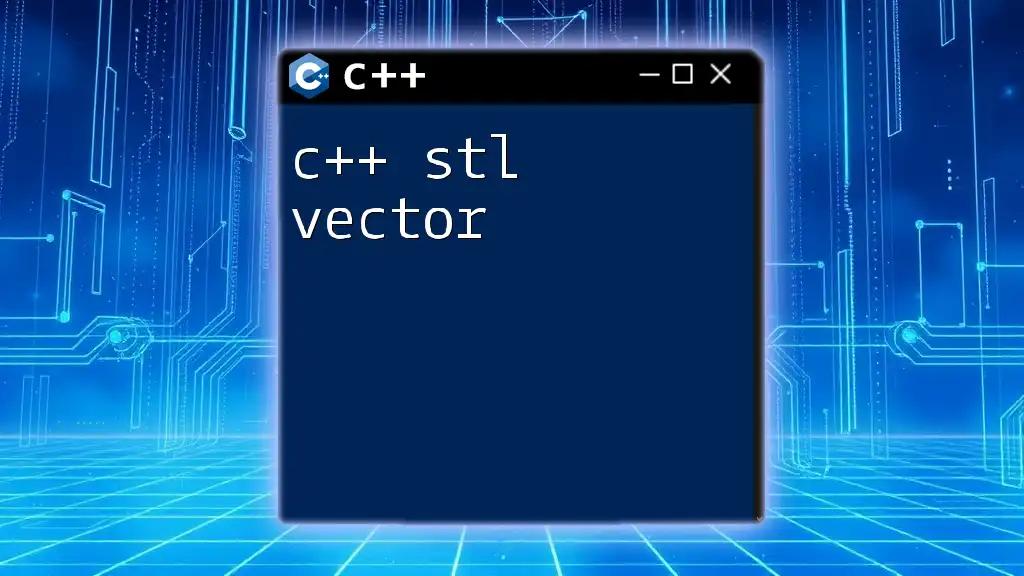
Using std::tie Incorrectly
Despite its power, programmers often misuse `std::tie`. Common mistakes include trying to unpack more values than provided. For example:
int x;
std::tie(x) = std::make_tuple(10, 20); // Error: too many values
Here, this code will lead to a compile-time error as there are too many values in the tuple for the single variable. It's essential to match the number of unpacked variables with the values provided in the tuple.

When to Use std::tie
To use `std::tie` effectively, keep these guidelines in mind:
- Use it for unpacking: When you have multiple return values from a function.
- Maintain readability: Group together related results in a single line.
- Avoid excessive copying: When handling large objects, utilize references for better performance.
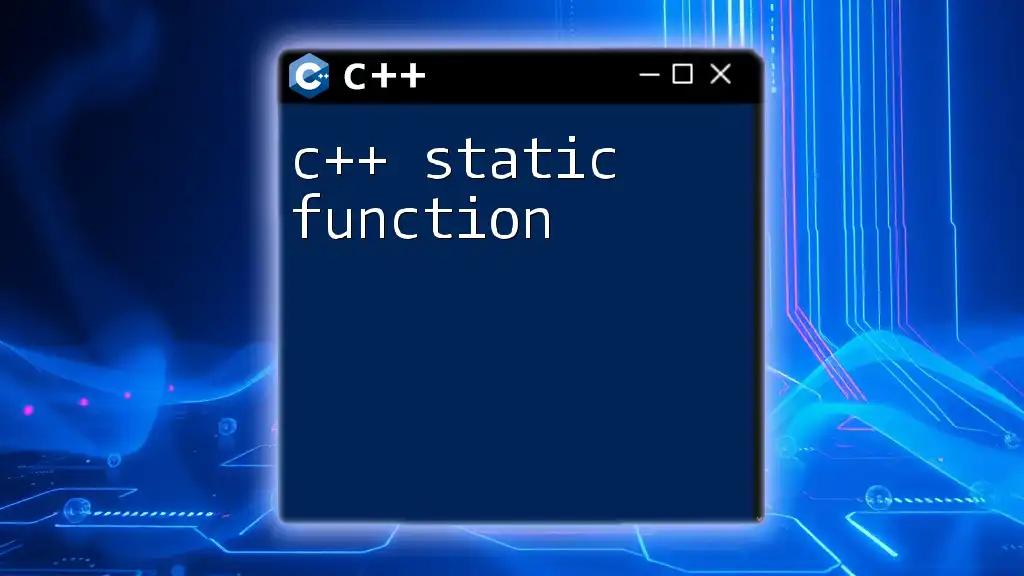
Recap of Key Points
In summary, `std::tie` is a valuable tool in C++ that enables the creation of tuple-like reference pairs. It enhances code clarity while improving efficiency by avoiding unnecessary copies. Whether unpacking multiple return values from functions or facilitating conditional assignments, `std::tie` can streamline your programming.
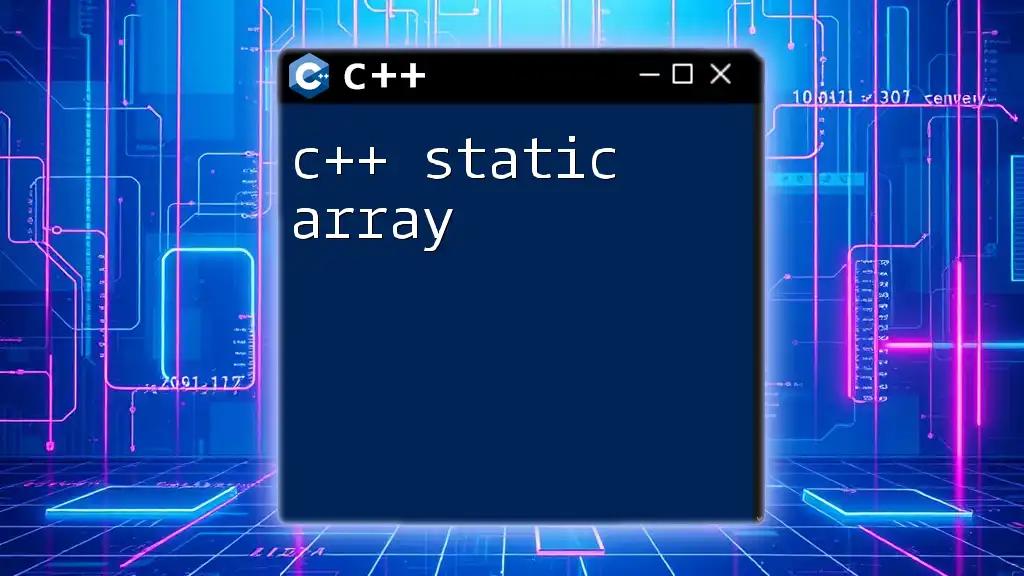
Further Reading and Resources
For those interested in diving deeper into the topic, here are some resources:
- The official C++ documentation for `<tuple>` and `std::tie`
- C++ programming books focusing on modern C++ (C++11 and beyond)
- Online courses that tackle advanced C++ concepts and best practices

Final Thoughts on std::tie
To conclude, understanding and implementing `std::tie` can improve the quality of your C++ code greatly. It allows developers to create structured, efficient, and maintainable code. Don't hesitate to incorporate `std::tie` into your programming toolkit, as it will serve you well in various scenarios, making your codebase cleaner and more efficient.