The `std::fill` function in C++ is used to assign a specified value to a range of elements in a container, effectively filling it with that value.
#include <vector>
#include <algorithm>
#include <iostream>
int main() {
std::vector<int> vec(5); // Create a vector of 5 elements
std::fill(vec.begin(), vec.end(), 10); // Fill the vector with the value 10
for(int num : vec) {
std::cout << num << " "; // Output: 10 10 10 10 10
}
return 0;
}
Understanding the Basics of std::fill
What is std::fill?
The `std::fill` function is a part of the C++ Standard Library, specifically found under the algorithm header. Its primary purpose is to fill a range of elements with a specific value. This is especially useful in situations where you want to initialize a collection or reset the contents of an existing one without needing to iterate through the elements manually. The efficiency and compactness of `std::fill` make it a valuable tool in C++ programming.
Syntax of std::fill
The general syntax for `std::fill` is:
std::fill(BidirectionalIterator first, BidirectionalIterator last, const T& value);
- first: This is the beginning iterator for the range to fill.
- last: This is the ending iterator, which is one past the last element to fill.
- value: The value used to fill the specified range.
This function does not return a value, as its purpose is to modify the range specified by the iterators.
Key Concepts
To effectively use `std::fill`, a solid understanding of iterators is essential. Iterators in C++ are a versatile way to represent a position within a range, allowing manipulation of data structures such as arrays and vectors without needing to use direct indexing. Additionally, the value type that you fill the elements with matters; `std::fill` can accept any type that can be assigned to the elements in the specified range.
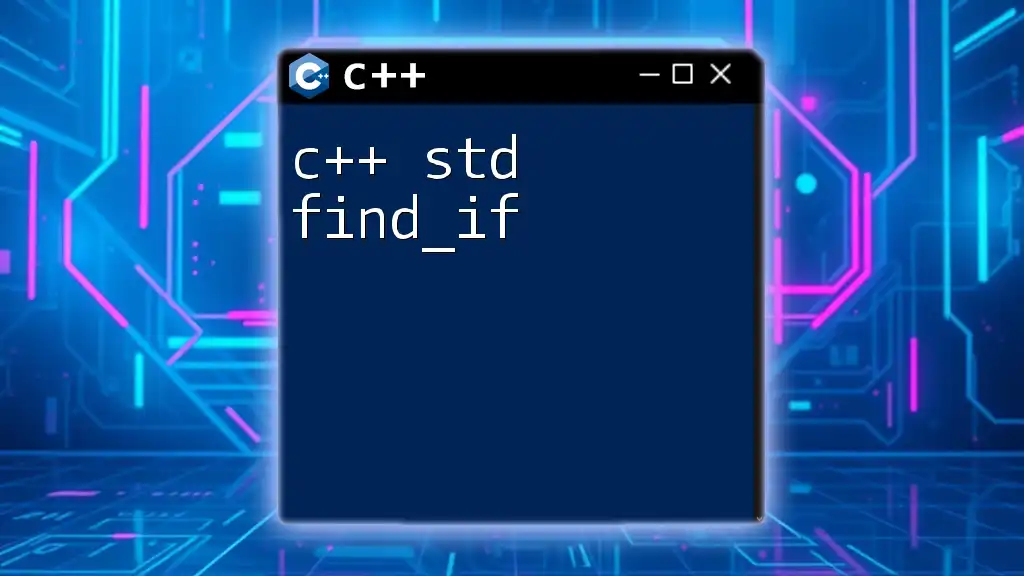
How to Use C++ std::fill
Basic Example of std::fill
A simple demonstration of `std::fill` can be seen in filling an array with a specific value.
#include <iostream>
#include <algorithm>
int main() {
int arr[5];
std::fill(arr, arr + 5, 10); // Filling the array with the value 10
for (int i : arr) {
std::cout << i << " "; // Outputs: 10 10 10 10 10
}
return 0;
}
In the example above, we use `std::fill` to set every element in the array `arr` to the value 10. The expression `arr + 5` points to the end of the array, allowing `std::fill` to know when to stop.
Filling an Array
Filling static arrays using `std::fill` offers a clear, efficient method to initialize or reset them. Continuing with arrays, you might see:
#include <iostream>
#include <algorithm>
int main() {
int arr[5];
std::fill(arr, arr + 5, 0); // Filling the array with zeros
for (int i = 0; i < 5; ++i) {
std::cout << arr[i] << " "; // Outputs: 0 0 0 0 0
}
return 0;
}
Using `std::fill` with arrays enhances readability and maintainability, as the intent to fill is clear and concise.
Filling a Vector
Vectors in C++ are dynamic arrays that provide more flexibility. `std::fill` can also be seamlessly used with them:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec(5); // Creating a vector of size 5
std::fill(vec.begin(), vec.end(), 42); // Filling with the value 42
for (int i : vec) {
std::cout << i << " "; // Outputs: 42 42 42 42 42
}
return 0;
}
Here, `vec.begin()` and `vec.end()` return iterators pointing to the first and one past the last elements, respectively. The result is a vector filled with the value 42, showcasing how `std::fill` can simplify operations on vectors.
Filling a Range with std::fill
Filling a specific range within a data structure is easily accomplished with `std::fill`. Consider the following code that fills a portion of a vector:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::fill(vec.begin() + 1, vec.begin() + 4, 99); // Filling elements 2 to 4 with 99
for (int i : vec) {
std::cout << i << " "; // Outputs: 1 99 99 99 5
}
return 0;
}
In this scenario, only the second, third, and fourth elements are filled with the value 99, demonstrating the versatility of `std::fill` in targeting specific segments of a collection.
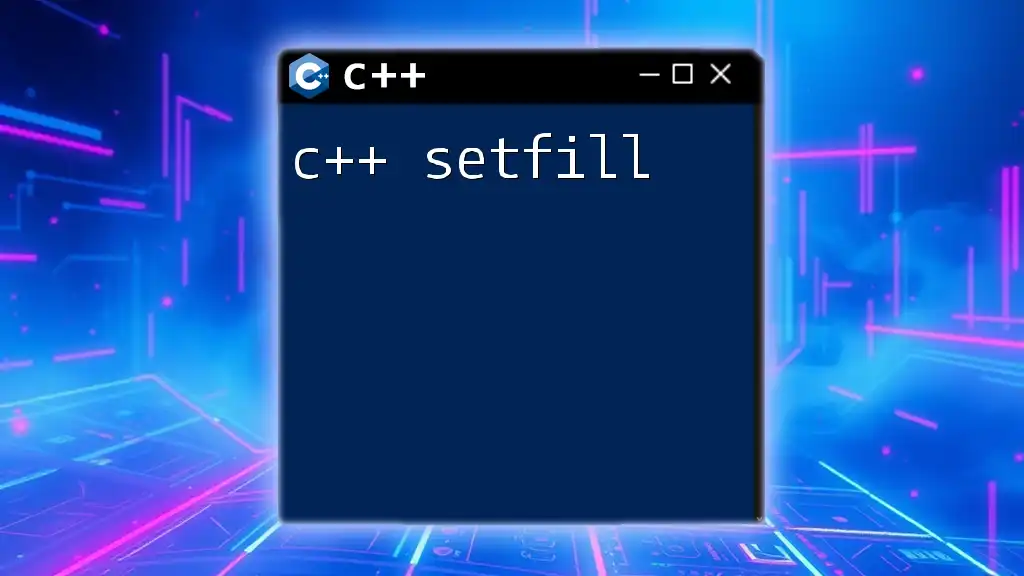
Advanced Usage of C++ std::fill
Using std::fill with Custom Data Types
One of the great features of `std::fill` is its ability to work with custom structures or classes. For example, suppose you have a struct `Point`:
#include <iostream>
#include <algorithm>
#include <array>
struct Point {
int x, y;
};
int main() {
std::array<Point, 3> points;
Point p = {1, 2};
std::fill(points.begin(), points.end(), p); // Filling with custom Point struct
for (const auto& point : points) {
std::cout << "(" << point.x << ", " << point.y << ") "; // Outputs: (1, 2) (1, 2) (1, 2)
}
return 0;
}
In this code, we created an array of `Point` structures and filled it with instances populated with specific values. The ability to use `std::fill` with custom types emphasizes its flexibility and power.
Performance Considerations
Understanding the performance implications of using `std::fill` is essential in optimizing code. The time complexity of `std::fill` is O(n), where n is the number of elements in the range to be filled. In terms of performance, `std::fill` is generally faster than manually iterating through each element (O(n) in that case too) due to certain optimizations made by the STL.
Combined Usage with Other Standard Algorithms
`std::fill` can often be used in conjunction with other standard algorithms. For instance, combining `std::copy` and `std::fill` can provide elegant solutions for initializing collections from existing data:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> source = {1, 2, 3};
std::vector<int> dest(3);
std::copy(source.begin(), source.end(), dest.begin());
std::fill(dest.begin() + 1, dest.end(), 100); // Filling from second element onward
for (int i : dest) {
std::cout << i << " "; // Outputs: 1 100 100
}
return 0;
}
Here, we first copy a source vector to a destination vector and then fill part of the destination with a new value. Such combinations enhance code flexibility and maintain readability.
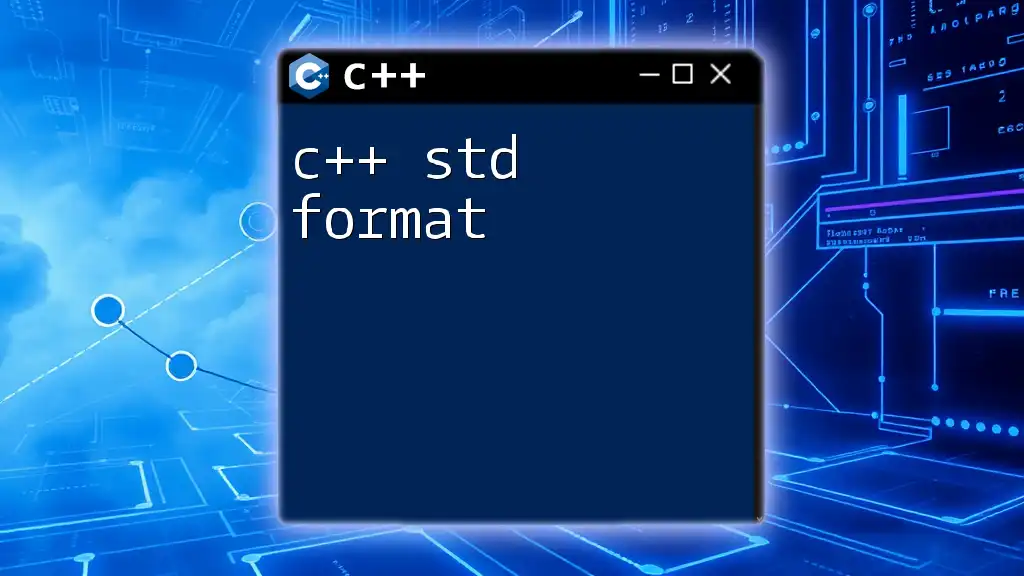
Best Practices for using std::fill
When to Use vs. Not to Use std::fill
Knowing when to use `std::fill` is vital for writing clean and efficient code. You should consider using `std::fill` when:
- Initializing or resetting a data structure.
- You want to preserve the type-safety and efficiency associated with the standard library.
- Filling ranges in complex structures.
However, avoid using it when:
- The operation requires conditional logic or custom filling strategies.
- You only need to modify a small subset of elements based on specific conditions.
Avoiding Common Pitfalls
While `std::fill` is straightforward, common issues can arise:
- Out-of-Bounds Access: Ensure that the iterators passed to `std::fill` do not exceed their bounds. Always validate your ranges.
- Incorrect Value Type: Make sure the value used to fill is compatible with the data type of the elements in the range.
Following best practices and being mindful of these pitfalls ensures a smoother development experience and robust code.
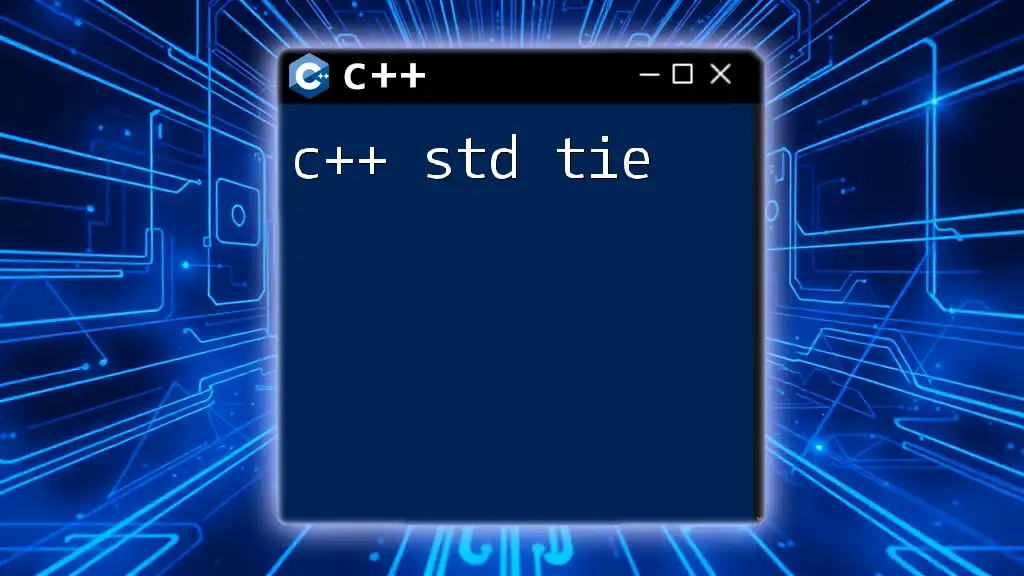
Conclusion
In summary, `C++ std::fill` is a fundamentally useful function that simplifies the process of filling collections with a specific value. Coupled with its ease of use across various data structures—including arrays, vectors, and custom types—`std::fill` enhances both the efficiency and readability of your code. By incorporating these techniques and best practices into your programming strategy, you can elevate your code quality and confidence as a C++ developer.
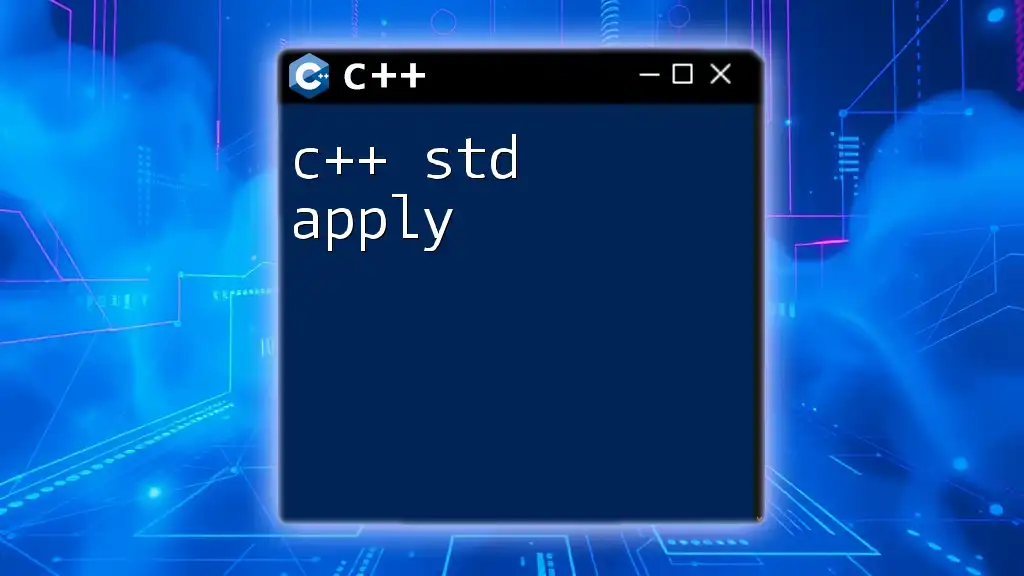
Additional Resources
- Explore the [official C++ Standard Library documentation](https://en.cppreference.com/w/cpp/algorithm/fill) for further details on `std::fill`.
- Recommended reading: "The C++ Programming Language" by Bjarne Stroustrup.
- Engage with online forums and communities, such as Stack Overflow or Reddit's r/cpp, to broaden your understanding and connect with fellow developers.