The `std::setfill` manipulator in C++ is used to specify a character that will fill in the empty spaces when formatting output with `std::setw`.
Here's a code snippet demonstrating its usage:
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::setfill('*') << std::setw(10) << 42 << std::endl;
return 0;
}
Understanding C++ Setfill
Definition of `setfill`
In C++, `setfill` is a manipulator that allows you to specify a fill character used by the output stream when the values being displayed don't completely fill the specified width. For instance, if you're trying to print a number within a set width, you might want to fill in the extra spaces with a specific character, such as a zero or a period. The function is part of the `<iomanip>` library.
Purpose of `setfill`
The primary purpose of `setfill` is to enhance the formatting of outputs in a controlled way, which is especially useful in creating aligned tables and organized displays. The ability to specify fill characters can produce cleaner, more readable outputs, which is invaluable in user interfaces and reporting.
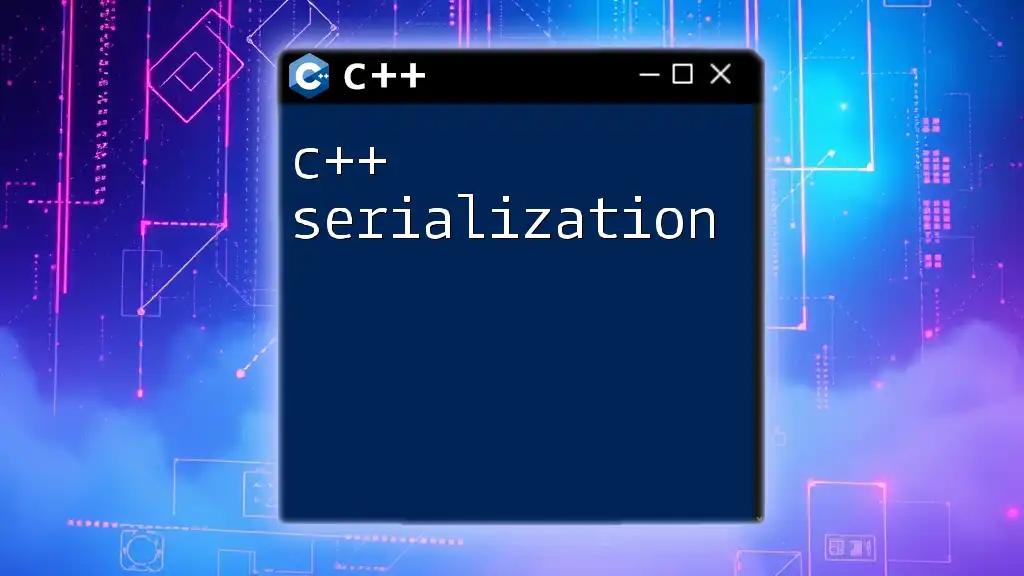
Using `setfill` in C++
Including the Necessary Header Files
To utilize `setfill`, you must include the `<iomanip>` and `<iostream>` libraries in your program. This allows you to access the formatting tools provided by C++.
#include <iostream>
#include <iomanip>
Basic Syntax and Examples
Setting Fill Characters
The syntax for `setfill` is straightforward:
std::cout << std::setfill('fill_character');
For example, if you want to fill with zeros:
std::cout << std::setfill('0') << std::setw(5) << 42; // Outputs: 00042
In this snippet, `std::setw(5)` sets the width to 5, and the `setfill('0')` ensures that the empty spaces are filled with zeros.
Combining `setfill` with `setw`
Explanation of `setw`
The `setw` manipulator is responsible for setting the width of the output. When combined with `setfill`, it can produce neatly formatted strings and numbers that stand out.
Example: Combining `setfill` with `setw`
Here’s how you can seamlessly integrate both manipulators:
std::cout << std::setfill('*') << std::setw(10) << 123; // Outputs: *****123
In this example, the width is set to 10, and the remaining spaces are filled with asterisks, showcasing the power of formatting in C++.
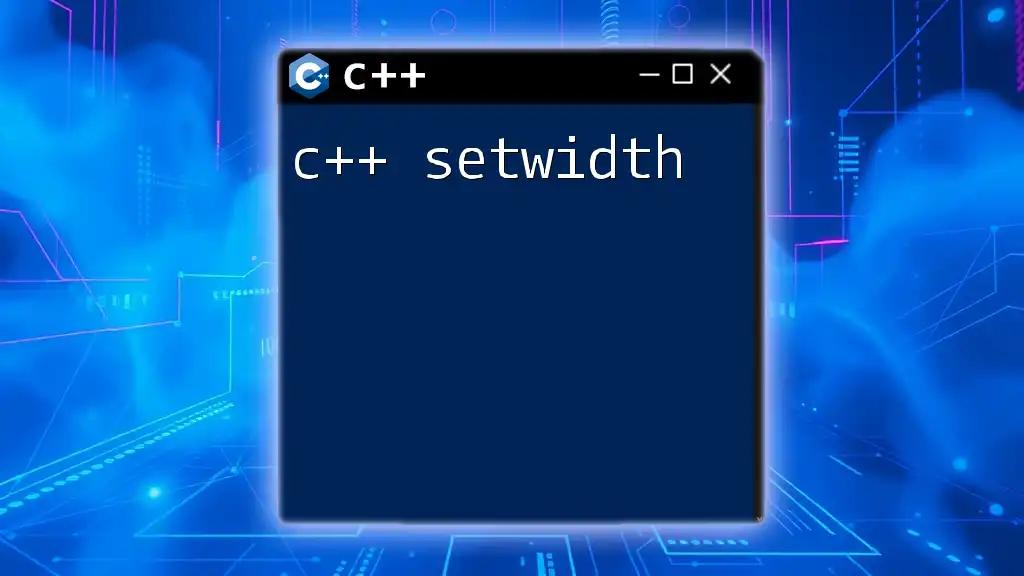
More Advanced Use Cases
Using Multiple Fill Characters
Custom Fill Character Examples
You can easily customize the fill character to anything you desire. Consider the following:
std::cout << std::setfill('-') << std::setw(10) << 7; // Outputs: ------7
In this snippet, the fill character is a dash, illustrating that `setfill` can greatly alter the look of your output.
Output Formatting in Loops
Practical Scenarios
One of the common applications of `setfill` is within loops, especially if you're displaying tables or sets of data:
for (int i = 1; i <= 10; ++i) {
std::cout << std::setfill('0') << std::setw(2) << i << " ";
}
// Outputs: 01 02 03 04 05 06 07 08 09 10
This loop prints numbers from 01 to 10, ensuring that each number is consistently formatted, which aids in clarity.

Common Mistakes and How to Avoid Them
When Does `setfill` Not Work?
Sometimes, you may find that `setfill` doesn't seem to be behaving as expected. A common reason for this is not using it in conjunction with `setw`. Always ensure that a width is specified for the fill character to take effect.
Overriding Previous Settings
Be cautious about the state of the output stream when using `setfill`. Once you set a fill character, it remains until you change it or reset the stream's format. This means if you apply `setfill('*')` followed by another `setfill('#')`, the latter will take precedence.

Performance Considerations
Impact on Output Performance
While using formatting manipulators like `setfill` can enhance the readability of output, it is important to remember that excessive formatting can slow down performance, especially in large loops or with extensive output operations. To maintain efficiency, use formatting judiciously.
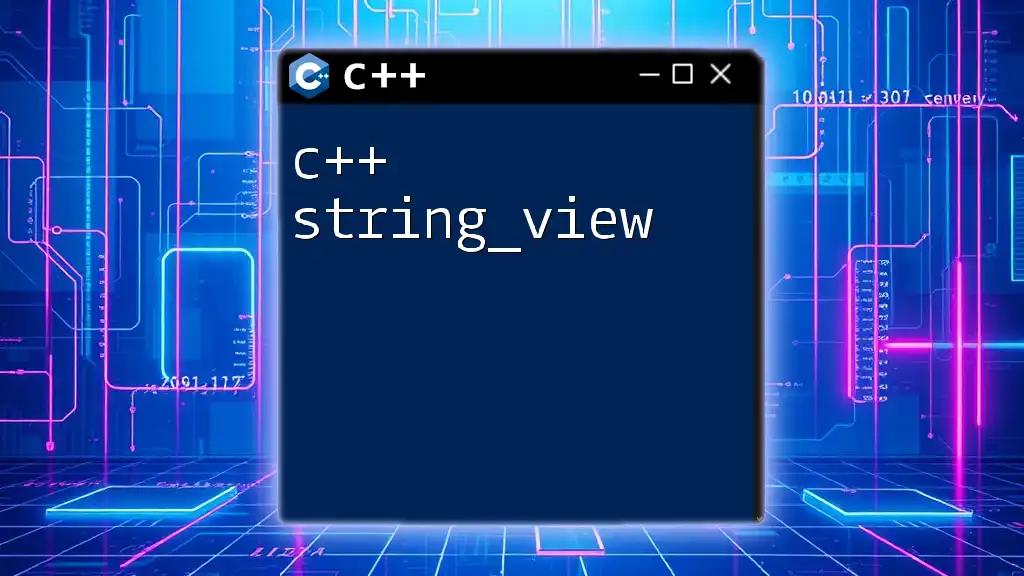
Conclusion
With C++ `setfill`, you have a powerful tool for customizing the appearance of your console output. By understanding and correctly applying `setfill`, combined with `setw`, you can create formatted outputs that are not only functional but also aesthetically pleasing. Experiment with different fill characters and widths to find the best presentation for your data.
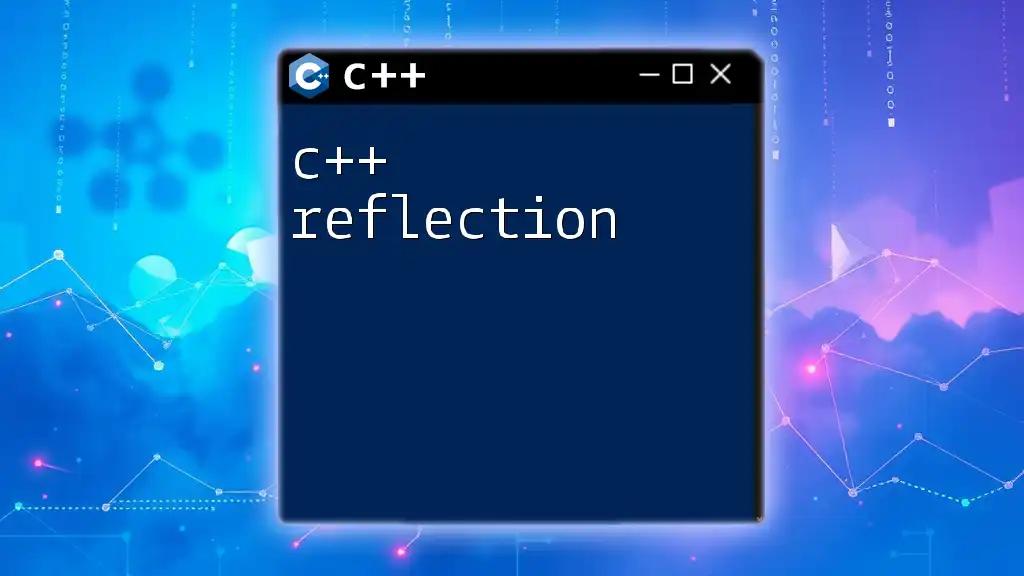
Additional Resources
For further reading on formatting in C++, consider exploring the official C++ documentation and resources that delve into the `<iomanip>` library, giving you deeper insights into its capabilities.

FAQs
What does `setfill` do?
Setfill allows you to specify a fill character used to pad output in an output stream, with its appearance dependent on the specified width denoted by `setw`.
Can you use `setfill` for types other than integers?
While `setfill` is most commonly used with integers, you can also format strings. For instance, appending spaces or other characters can help you align textual data just as effectively.
How do you reset the fill character in C++?
To reset the fill character to its default state (a space), simply use `std::setfill(' ')`. This efficiently restores the default behavior of the output stream.
With a comprehensive understanding of `setfill`, you now have the tools needed to create clean, organized, and visually appealing outputs in your C++ programs!