C++ pairs are a simple way to store two related values together as a single unit, often used for associative containers like maps.
#include <iostream>
#include <utility>
int main() {
std::pair<int, std::string> myPair(1, "example");
std::cout << "First: " << myPair.first << ", Second: " << myPair.second << std::endl;
return 0;
}
What is a Pair in C++?
A pair in C++ is a simple yet powerful data structure defined in the Standard Template Library (STL) that allows you to store two heterogeneous values together. It serves as a convenient way to group two related values. For instance, when you want to store a student's ID along with their name, you can use a pair to encapsulate both pieces of data efficiently.
Understanding C++ pairs is paramount for enhancing your programming capabilities, especially when you want to return multiple values from functions or create complex data structures.

Basic Structure of a Pair
The C++ pair is implemented in the `<utility>` header file. It is defined as:
template <class T1, class T2>
struct pair;
This means that a pair can hold two values of potentially different types, where `T1` is the type of the first element and `T2` is the type of the second element.
Creating Pairs in C++
You can create pairs in various ways.
Using `std::make_pair()`
Using `std::make_pair()` is the most common method for creating a pair. Here's how you can create a pair containing an integer and a string:
#include <iostream>
#include <utility>
int main() {
std::pair<int, std::string> myPair = std::make_pair(1, "Hello");
std::cout << myPair.first << ", " << myPair.second << std::endl;
return 0;
}
In this example, `myPair.first` holds the integer `1`, while `myPair.second` contains the string "Hello".
Direct Initialization
Another way to create pairs is through direct initialization. Here's how to do it:
std::pair<int, std::string> anotherPair(2, "World");
In this example, `anotherPair` is directly initialized with the values `2` and "World".

Accessing Pair Elements
Accessing the elements of a C++ pair is straightforward. You can use the member variables `first` and `second` to retrieve the values stored in the pair. Here's an example:
std::cout << "First: " << anotherPair.first << ", Second: " << anotherPair.second << std::endl;
This will print the values encapsulated in the pair: `First: 2, Second: World`.

Modifying Pair Elements
Pairs are mutable, meaning you can change their values. You can simply assign new values to the `first` and `second` members as shown below:
anotherPair.first = 3;
anotherPair.second = "Modified";
After this operation, the values of `anotherPair` will be updated to `3` and "Modified".

Practical Uses of C++ Pairs
Pair as Function Return Types
One of the practical applications of C++ pairs is returning multiple values from a function. For instance, consider a function that determines the minimum and maximum of two numbers:
std::pair<int, int> minMax(int a, int b) {
return std::make_pair(a < b ? a : b, a > b ? a : b);
}
Here, the function `minMax` returns a pair containing the minimum and maximum values. This allows you to fetch multiple outputs from a single function call conveniently.
Storing Key-Value Pairs
Pairs are well-suited for storing key-value relationships, such as in associative arrays and maps. For example:
#include <map>
std::map<std::string, int> scores;
scores.insert(std::make_pair("Alice", 95));
In this code, a map called `scores` is created, linking the string "Alice" to the integer `95`, effectively associating a student's name with their score.
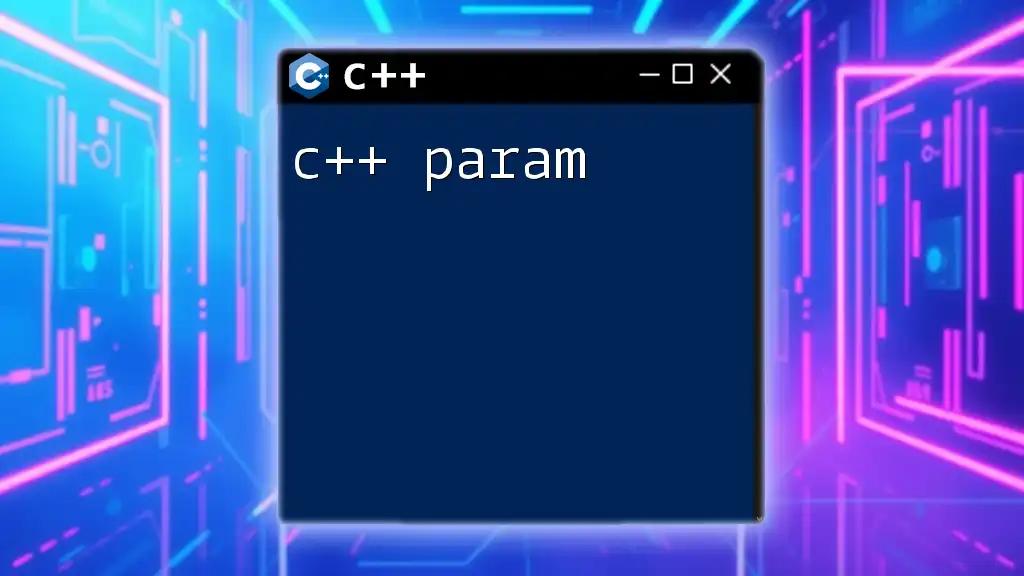
Using Pairs in STL Containers
Using Pairs in Vectors
You can also store pairs in STL containers such as vectors. Below is an example of how to implement a vector containing pairs:
#include <vector>
std::vector<std::pair<int, int>> pairVector;
pairVector.push_back(std::make_pair(1, 2));
In this example, `pairVector` now contains a pair `(1, 2)`.
Sorting Pairs
Sorting pairs is a common task. When sorting a vector of pairs, it automatically uses the first element for comparison, falling back to the second if the first elements are equal. Here’s a basic example:
std::sort(pairVector.begin(), pairVector.end());
This sorts the `pairVector` based on the `first` and `second` elements of the pairs.
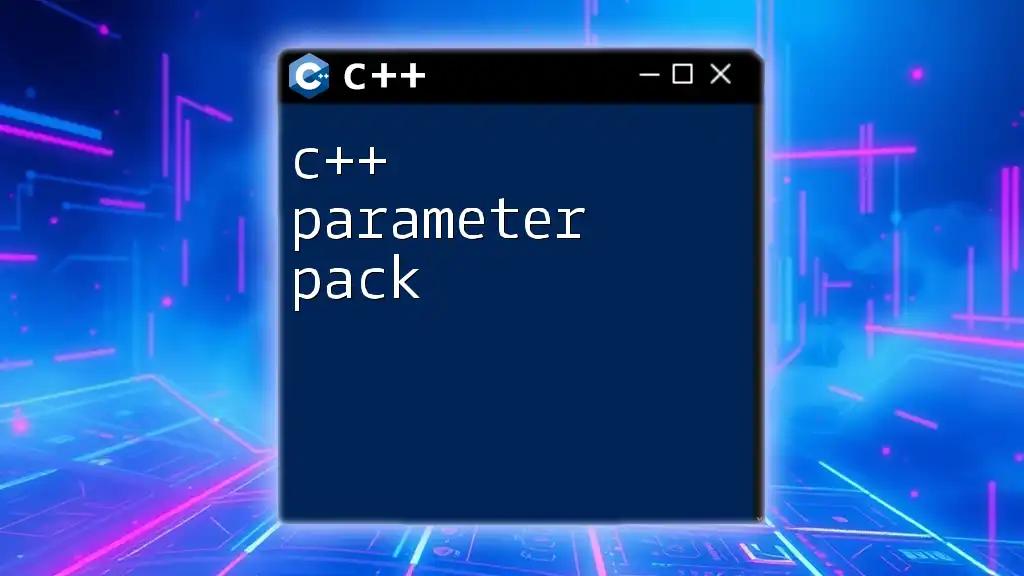
Comparison Operators for Pairs
C++ pairs can be compared using relational operators such as `<`, `>`, `==`, etc. The comparison occurs lexicographically based on the first element, then the second element if necessary. Thus, pairs can be directly used in data structures that require comparisons.

Limitations of Pairs
While C++ pairs provide a simple way to group two values, they do have limitations. They should not replace structures or classes when dealing with more complex data compositions. When you need to group more than two data points or share meaningful context, consider using a `struct` or a `std::tuple`.

When to Use Pairs
Pairs can be highly useful when you need to combine two logically associated elements, such as coordinates (x, y) or items in a collection (key, value). Their simplicity often makes them more efficient for quick tasks compared to defining a full class or struct.
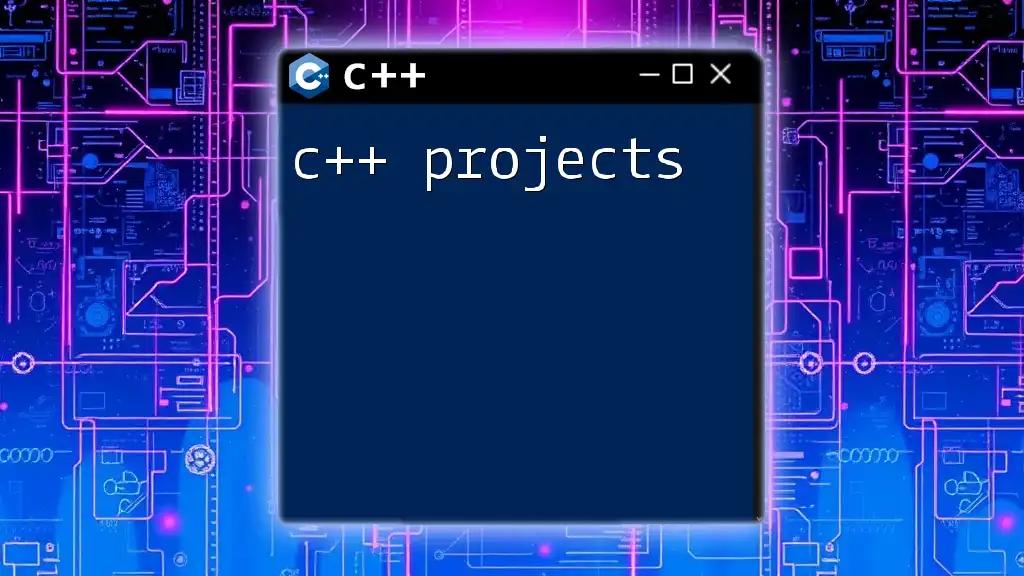
Avoiding Pitfalls
Despite their utility, overusing pairs can lead to code that is hard to read and maintain. Always strive for clarity in your code. If you find yourself using pairs extensively, it might be a sign to switch to a more descriptive data structure.
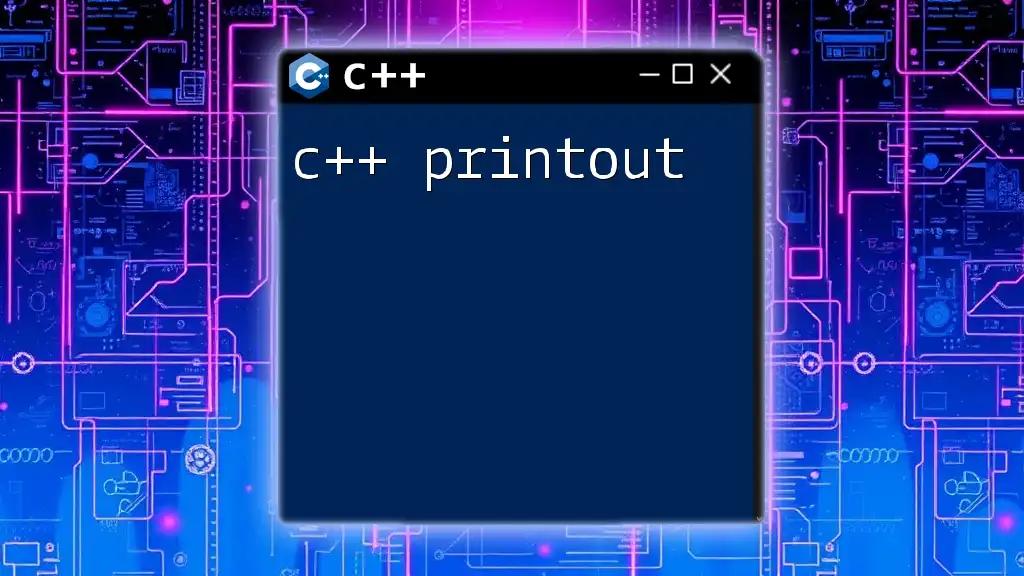
Recap of Key Points about C++ Pairs
C++ pairs are a versatile and efficient way to manage and manipulate two associated data points. They provide a straightforward mechanism for grouping values, simplifying return types for functions, and organizing data in maps and vectors. Understanding how to utilize pairs effectively can significantly enhance your C++ programming skills.
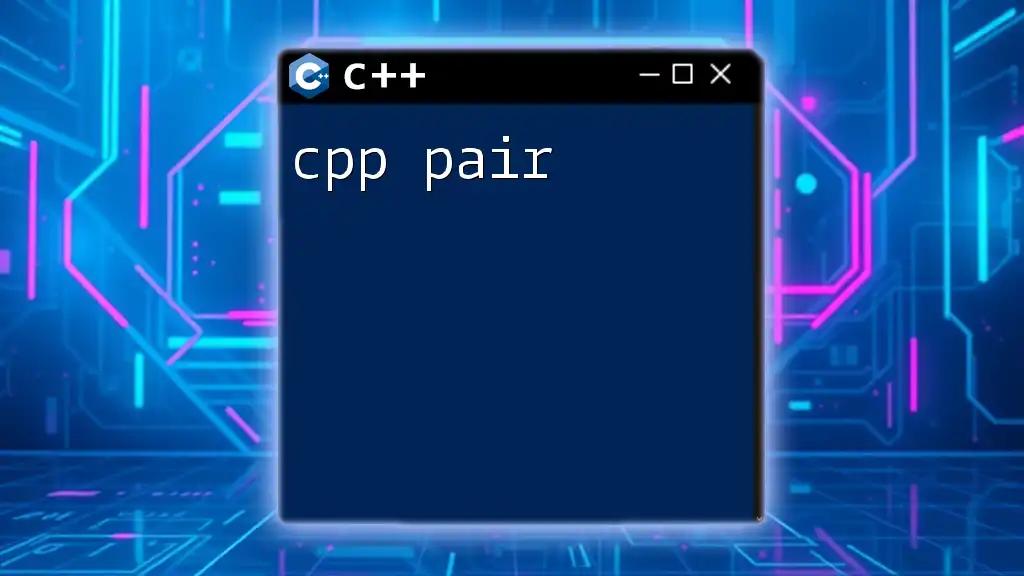
Further Reading
For further exploration of C++ pairs and the STL, visit the official C++ documentation and consider engaging with coding challenges that involve pair manipulations. Experimenting with practical applications will reinforce your knowledge and lead to more efficient programming practices.