A C++ matrix is a two-dimensional array that allows you to store and manipulate data in rows and columns, facilitating various mathematical operations.
#include <iostream>
using namespace std;
int main() {
const int rows = 3, cols = 3;
int matrix[rows][cols] = { {1, 2, 3}, {4, 5, 6}, {7, 8, 9} };
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
cout << matrix[i][j] << " ";
}
cout << endl;
}
return 0;
}
Understanding Matrix Basics
What is a Matrix?
A matrix is a mathematical structure that consists of a collection of numbers arranged in a fixed number of rows and columns. In programming, especially in C++, matrices are often used for sophisticated calculations and data representations. For example, a matrix can represent a system of linear equations or be utilized in graphics programming to perform transformations.
Types of Matrices
There are several different types of matrices commonly encountered in C++ programming:
- Row Matrix: A matrix that consists of a single row.
- Column Matrix: A matrix that consists of a single column.
- Square Matrix: A matrix with an equal number of rows and columns (e.g., 3x3, 4x4).
- Rectangular Matrix: A matrix with differing numbers of rows and columns (e.g., 2x3).
- Diagonal Matrix: A square matrix where all off-diagonal elements are zero.
- Identity Matrix: A special diagonal matrix where all diagonal elements are one.
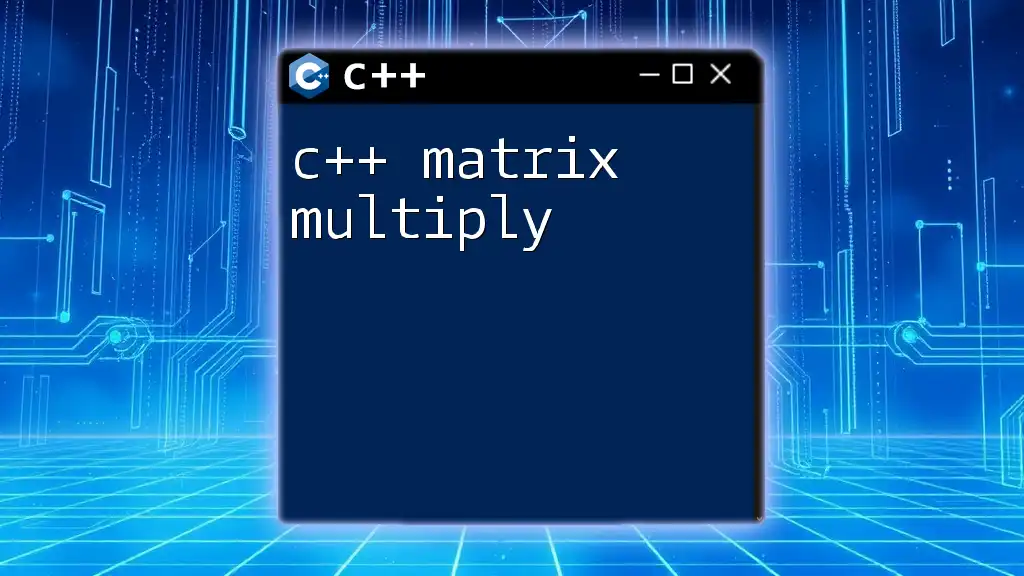
Implementing Matrices in C++
Declaring a Matrix
When working with matrices in C++, one can choose between static or dynamic allocation.
Static Matrix Declaration
Static matrices are declared with a fixed size, which is determined at compile time.
const int ROWS = 3;
const int COLS = 3;
int matrix[ROWS][COLS];
Dynamic Matrix Allocation
Dynamic matrices allow developers to create matrices of variable sizes during runtime using pointers.
int** createMatrix(int rows, int cols) {
int** matrix = new int*[rows];
for (int i = 0; i < rows; i++) {
matrix[i] = new int[cols];
}
return matrix;
}
Accessing Matrix Elements
Accessing elements in a matrix involves using the appropriate indices.
matrix[0][0] = 1; // Assigning value 1 to the first element
int value = matrix[0][0]; // Retrieving the first element
Matrix Operations
Matrix Addition
To add two matrices, their corresponding elements are summed.
Code Example:
void addMatrices(int a[ROWS][COLS], int b[ROWS][COLS], int result[ROWS][COLS]) {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
result[i][j] = a[i][j] + b[i][j];
}
}
}
Matrix Subtraction
Similarly, subtracting one matrix from another involves subtracting corresponding elements.
Code Example:
void subtractMatrices(int a[ROWS][COLS], int b[ROWS][COLS], int result[ROWS][COLS]) {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
result[i][j] = a[i][j] - b[i][j];
}
}
}
Matrix Multiplication
Matrix multiplication requires a careful alignment of rows and columns. The number of columns in the first matrix must equal the number of rows in the second matrix.
Important Considerations: Ensure the dimensions are compatible to perform multiplication.
Code Example:
void multiplyMatrices(int a[ROWS][COLS], int b[COLS][ROWS], int result[ROWS][ROWS]) {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < ROWS; j++) {
result[i][j] = 0;
for (int k = 0; k < COLS; k++) {
result[i][j] += a[i][k] * b[k][j];
}
}
}
}
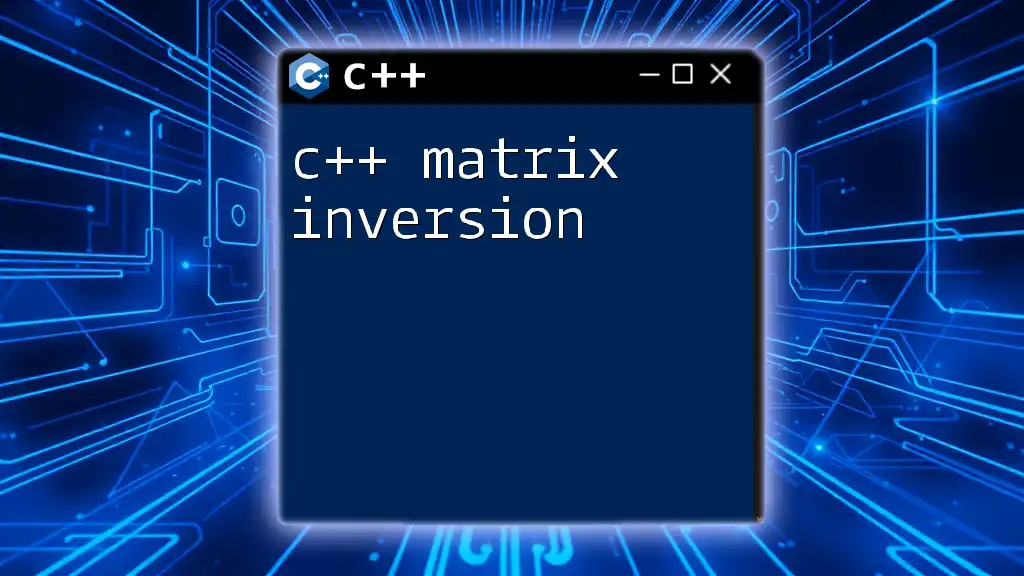
Advanced Matrix Techniques
Transposing a Matrix
Transposing a matrix involves swapping its rows with columns. For instance, the element at position (i, j) becomes (j, i).
Code Example:
void transposeMatrix(int original[ROWS][COLS], int transposed[COLS][ROWS]) {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
transposed[j][i] = original[i][j];
}
}
}
Determinants and Inverses
A determinant is a special number calculated from a square matrix that provides various properties of the matrix. Calculating the inverse of a matrix is significant in solving systems of equations, but not all matrices have inverses.
Code Example for Finding Determinant:
int determinant(int matrix[2][2]) {
return (matrix[0][0] * matrix[1][1]) - (matrix[0][1] * matrix[1][0]);
}
Code Example for Finding Inverse:
void inverseMatrix(int matrix[2][2], float inverse[2][2]) {
int det = determinant(matrix);
if (det != 0) {
inverse[0][0] = matrix[1][1] / float(det);
inverse[0][1] = -matrix[0][1] / float(det);
inverse[1][0] = -matrix[1][0] / float(det);
inverse[1][1] = matrix[0][0] / float(det);
}
}
Matrix Storage Techniques
Matrices can be stored in several ways, the most common being a conventional 2D array. However, dynamic memory with vectors (from the C++ Standard Library) provides benefits like automatic management of memory and flexibility in size.
Benefits of Using Vectors:
- Dynamic resizing.
- Simplified memory management.
- A rich set of in-built functions for ease of use.
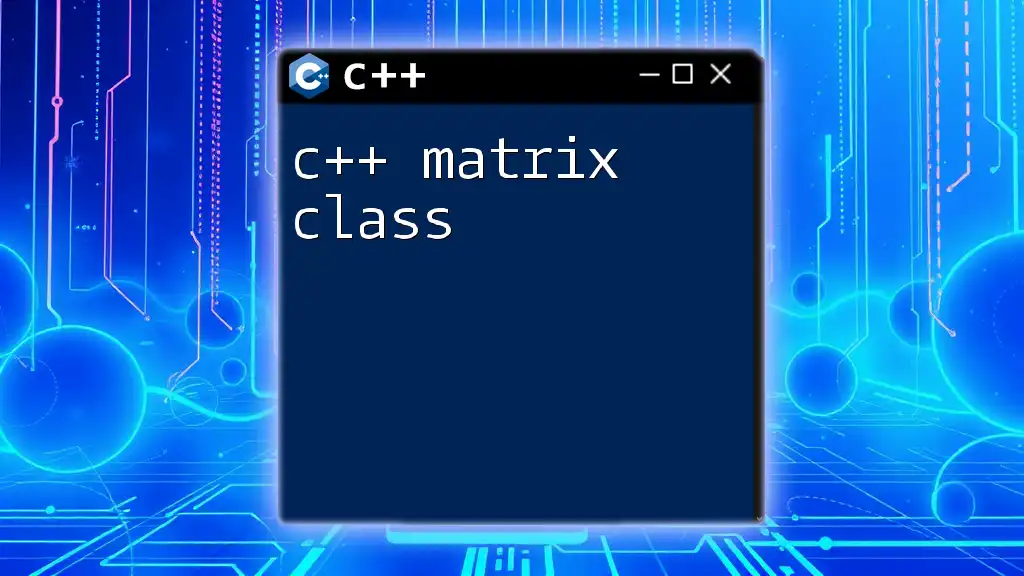
Practical Applications of Matrices in C++
Solving Linear Equations
Matrices are invaluable in solving systems of linear equations using methods like Gaussian elimination or matrix inversion.
Code Example:
// Assume a function that uses previously defined matrix operations
void solveEquations(int a[ROWS][COLS], int b[ROWS]) {
float inverse[ROWS][COLS];
inverseMatrix(a, inverse);
// Further multiplication with b to find solution
}
Graphics and Image Processing
In graphics programming, matrices are used for transformations such as translation, scaling, and rotation of images. These operations can be represented with matrix multiplication.
Code Example:
void applyTransformation(int point[2], int transformation[3][3]) {
int newPoint[3] = {point[0], point[1], 1}; // Homogeneous coordinates
int transformed[3] = {0, 0, 0};
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
transformed[i] += transformation[i][j] * newPoint[j];
}
}
// Now transformed contains the new coordinates
}
Machine Learning and Data Science
In machine learning, data is often represented in matrix form, with features as columns and samples as rows. Algorithms like linear regression and neural networks rely heavily on matrix computations.
Basic Implementations using C++: Using matrices for simple linear regression involves storing data points and calculating coefficients through matrix operations previously discussed.
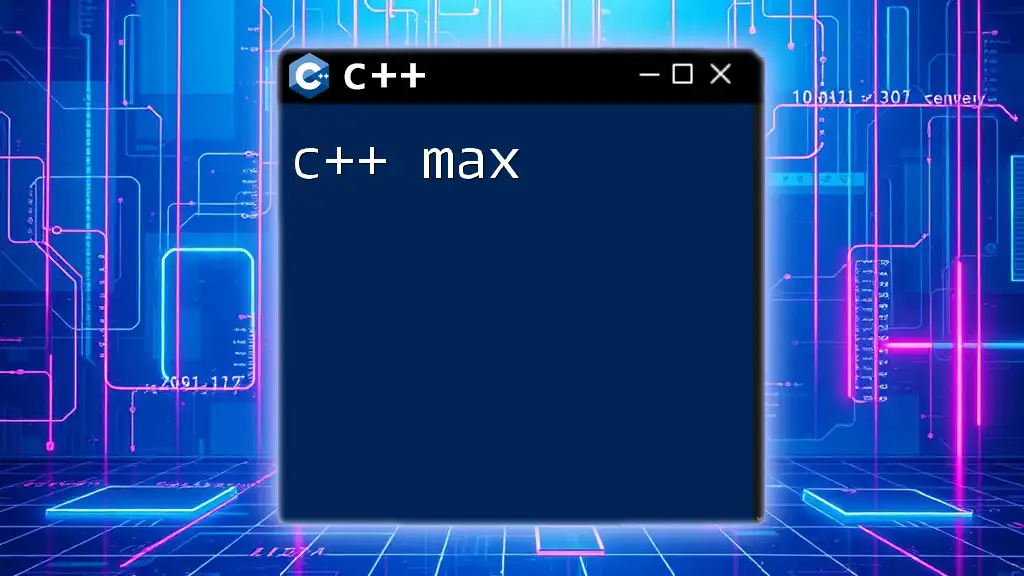
Debugging Common Matrix Issues in C++
Common Errors with Matrix Logic
Programming with matrices in C++ can lead to common pitfalls:
- Index Out of Bounds: Always ensure indices are within the defined bounds of the matrix.
- Memory Leaks with Dynamic Matrices: If using dynamic allocation, ensure to delete allocated memory to prevent memory leaks.
Code Snippet for Error Handling:
if (rowIndex >= ROWS || colIndex >= COLS) {
throw std::out_of_range("Index out of bounds!");
}
Best Practices for Working with Matrices
To optimize memory utilization and performance, consider:
- Implementing proper memory management strategies when using dynamic allocation.
- Choosing between static and dynamic allocation based on application needs (e.g., if matrix size is known ahead of time, static allocation is preferred for efficiency).
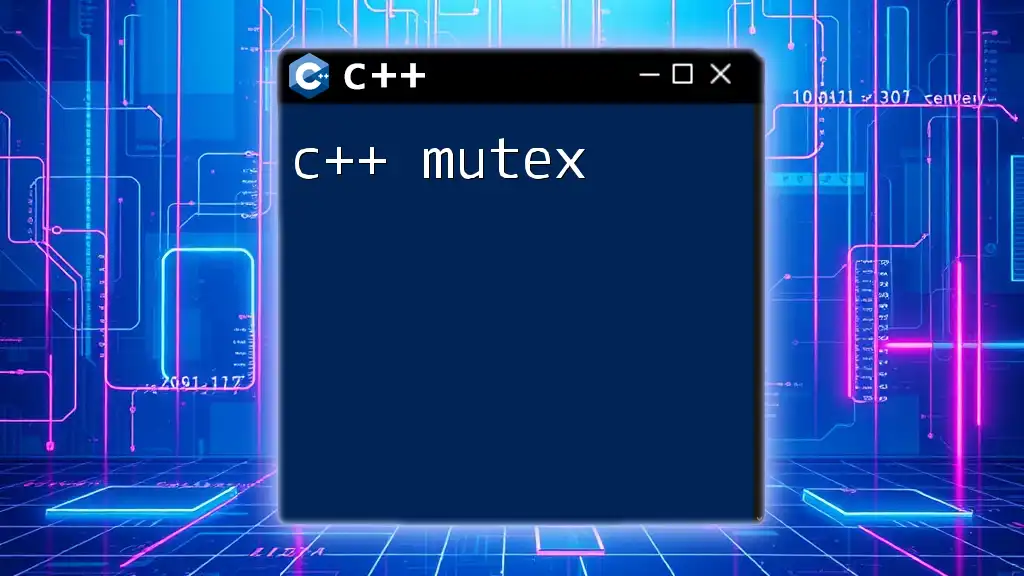
Conclusion
In this comprehensive guide on C++ matrices, we've covered essential matrix types, operations, advanced techniques, and practical applications. The versatility of matrices in programming cannot be overstated, serving critical functions in various domains such as graphics, machine learning, and solving complex mathematical problems. By practicing these concepts through coding examples, you can solidify your understanding and enhance your skills in C++.
Feel encouraged to dive deeper into this topic and explore additional resources available to expand your knowledge!