In C++, the maximum value for an integer can be obtained using the `std::numeric_limits` class from the `<limits>` header, which provides a portable way to retrieve the maximum value for various data types.
#include <iostream>
#include <limits>
int main() {
std::cout << "The maximum value for an int is: " << std::numeric_limits<int>::max() << std::endl;
return 0;
}
Understanding Integer Types in C++
What are Integers?
In C++, integers are whole numbers that can be positive, negative, or zero. They are one of the fundamental data types in C++ and are used to perform arithmetic operations and control program flow. Understanding how integers function is critical for effective programming, especially when dealing with maximum and minimum values.
Different Integer Types in C++
C++ provides several integer types, each with unique characteristics tailored for specific needs:
- Standard Integer Types: These include `int`, `short`, `long`, and `long long`, which vary in size and range of values based on the compiler and system architecture.
Code Snippet: Declaring Different Integer Types
int myInt = 10;
short myShort = 100;
long myLong = 1000L;
long long myLongLong = 10000LL;
- Unsigned Integers: This variation allows only non-negative values, effectively doubling the upper limit of the integer range compared to their signed counterparts. This can be particularly useful when negative numbers are not needed.
Code Snippet: Using Unsigned Integers
unsigned int myUnsignedInt = 20;
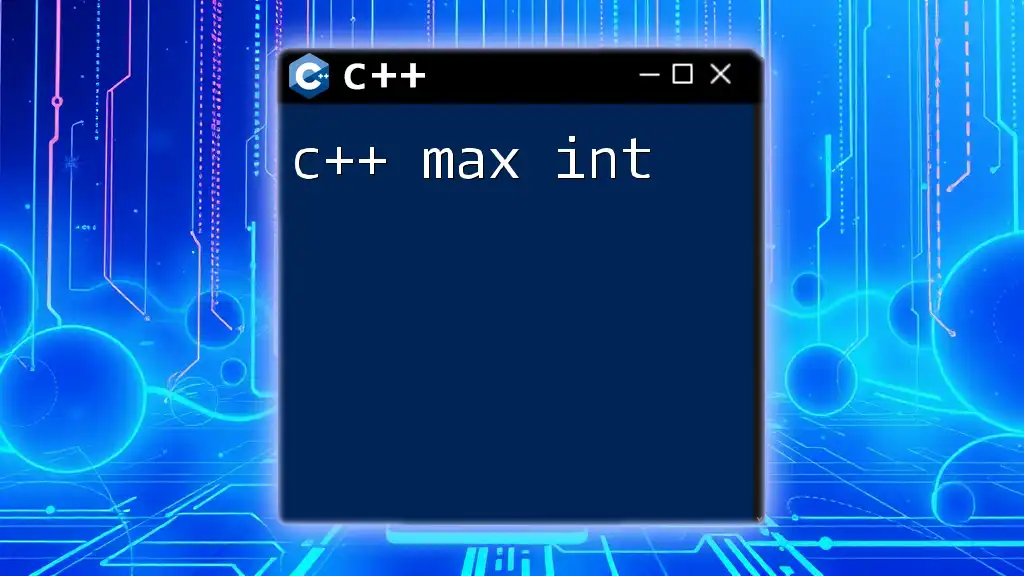
The Concept of Maximum Integer Values
What is Max Integer?
The term maximum integer value refers to the largest value that a specific integer type can hold. Each type has a different max value based on the number of bits allocated. For instance, an `int` might have a maximum value of 2,147,483,647 (for a 32-bit system), while a `long long` can go much higher, allowing for larger computations.
Determining Maximum Values
In C++, developers can easily find the maximum values for various integer types using the `<limits>` header, which provides a standardized way to access these limits.
Utilizing the `<limits>` Header
The `std::numeric_limits` class in the `<limits>` header offers methods to determine numeric properties. It is essential in writing robust programs that handle integer limits wisely.
Code Snippet: Finding Max Integer Values
#include <limits>
#include <iostream>
int main() {
std::cout << "Max int: " << std::numeric_limits<int>::max() << std::endl;
std::cout << "Max short: " << std::numeric_limits<short>::max() << std::endl;
std::cout << "Max long: " << std::numeric_limits<long>::max() << std::endl;
std::cout << "Max long long: " << std::numeric_limits<long long>::max() << std::endl;
return 0;
}
This code outputs the maximum values for each integer type, giving developers critical insight into the limits of their data types.
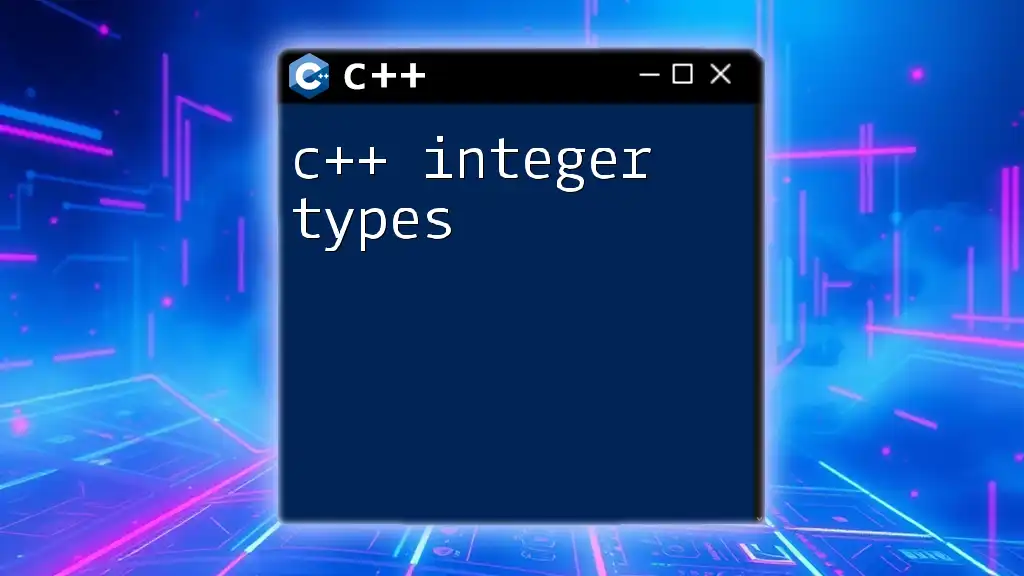
Practical Applications of Max Integer Values
Setting Limits in Programming
Understanding the maximum integer values is crucial when setting boundaries in programming, especially for array indexing and loop boundaries. Failing to acknowledge these limits can lead to unnecessary and complex bugs.
Overflow and Underflow: Risks of Ignoring Max Integers
Ignoring the implications of maximum integers can produce overflow and underflow conditions where the value exceeds the type range. This is a common pitfall in C++ and can lead to unexpected behavior in programs.
Code Snippet: Demonstrating Overflow
#include <iostream>
#include <limits>
int main() {
int a = std::numeric_limits<int>::max();
a++;
std::cout << "Overflowed Value: " << a << std::endl; // Outputs a negative value
return 0;
}
In this scenario, the `int` variable `a` exceeds its maximum limit, resulting in an overflow. The output illustrates how an overflow produces a negative value, leading to potential logical errors.
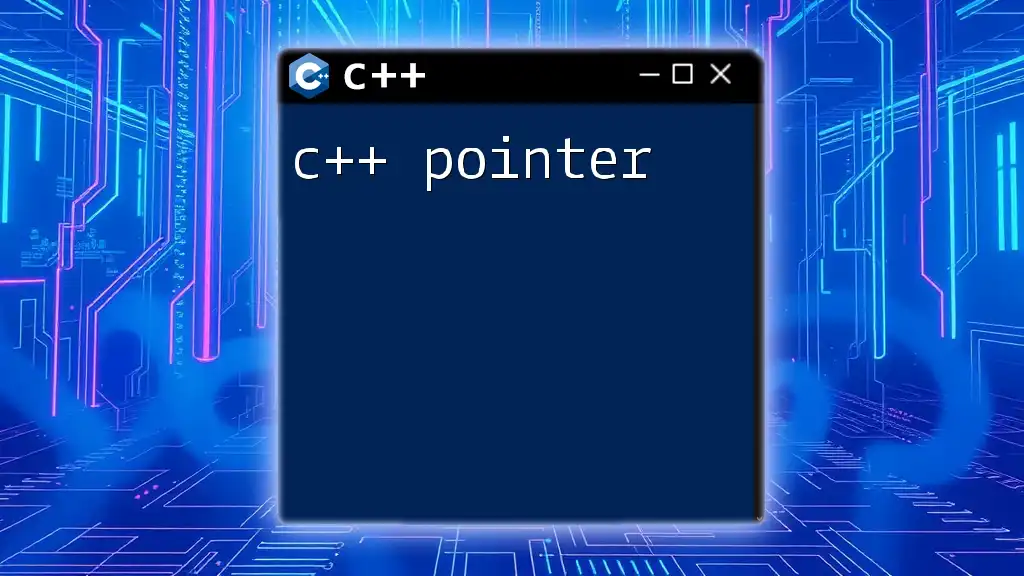
Performance Considerations
Why Choosing the Right Integer Type Matters
Selecting the appropriate integer type is essential for performance optimization. Different integer types consume varying amounts of memory; thus, using smaller types like `short` can save space when large ranges are unnecessary. However, choosing types purely based on size may backfire if they cannot accommodate expected values, leading to performance or logical flaws.
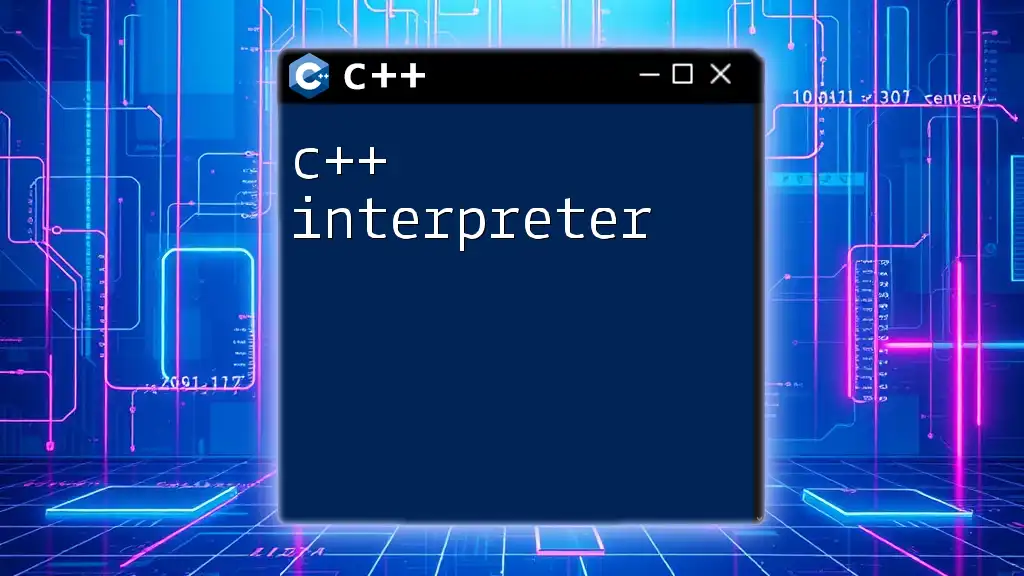
Best Practices
To harness the full potential of maximum integers, consider these best practices:
-
Always Check Limits: Before performing operations that could reach maximum values, incorporate checks to avoid overflow and underflow. This practice is vital in applications like financial calculations.
-
Leverage Unsigned Types Wisely: Use unsigned integers when you are certain that negative values do not apply. This increases the maximum possible value.
-
Consistent Type Usage: Stick to one integer type within specific operations or data structures to avoid implicit conversions that can introduce errors.
Example Scenarios
If processing user input that only requires non-negative values, opt for `unsigned int` instead of `int` to maximize the range for valid inputs, thus preventing an overflow issue in scenarios with large values.
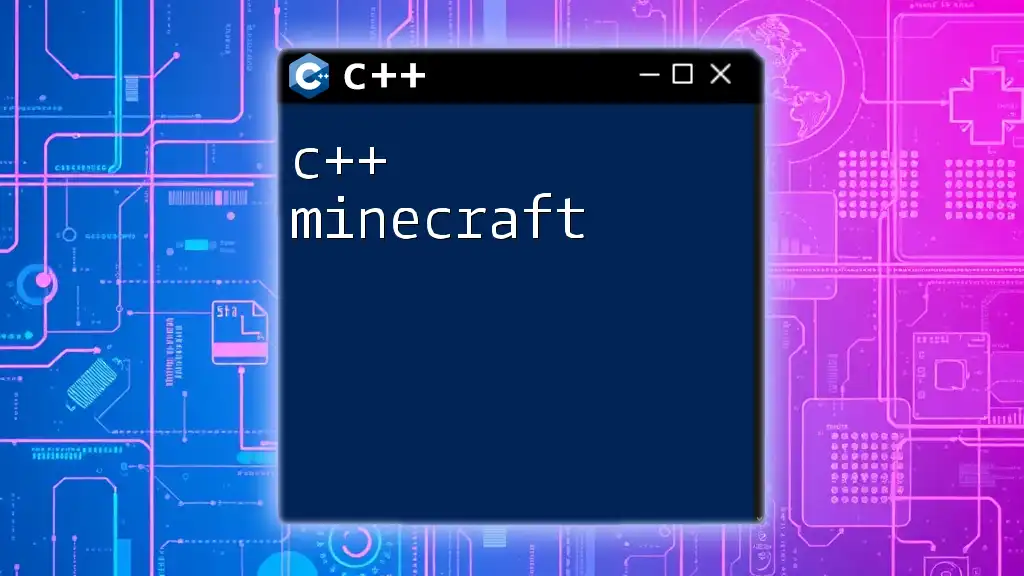
Conclusion
Understanding the C++ max integer and its applications is key to optimizing code and preventing overflow errors. By utilizing the resources available in the `<limits>` header and adhering to best practices, developers can write safer and more efficient C++ programs. Incorporating these principles into your coding routine will lead to better programming outcomes and reduce the potential for bugs related to integer limits.
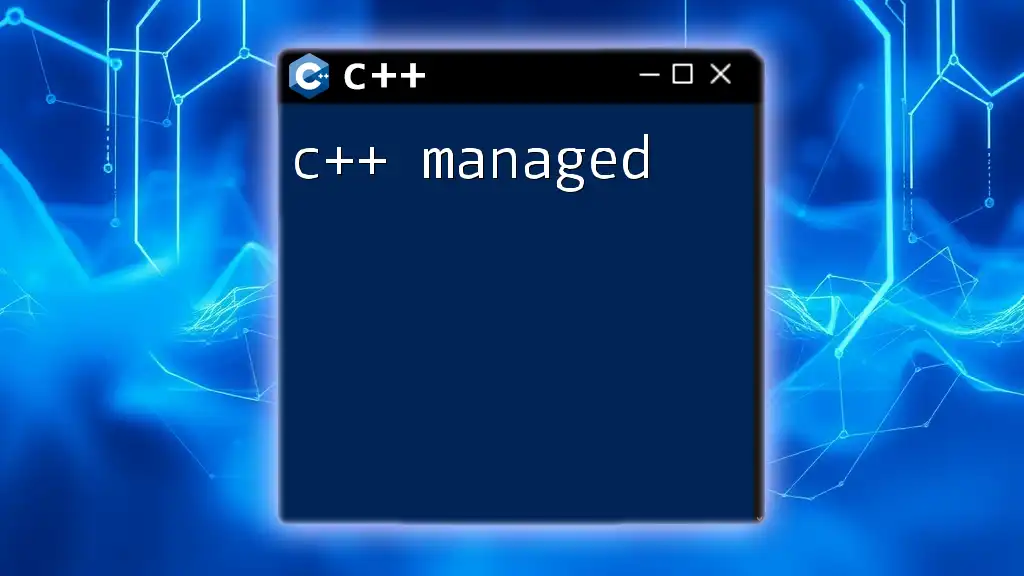
Additional Resources
For those seeking to deepen their understanding of integers in C++, consider exploring reputable online resources, community forums, and comprehensive programming books that cover integer types and best practices in greater detail. Engaging with fellow developers can also provide valuable insights and coding techniques.