In C++, the maximum value of an integer can be retrieved using the `std::numeric_limits` class from the `<limits>` header.
Here's a code snippet demonstrating how to find the maximum integer value:
#include <iostream>
#include <limits>
int main() {
std::cout << "The maximum value of an int is: " << std::numeric_limits<int>::max() << std::endl;
return 0;
}
What is an Integer in C++?
Definition of Integer
In programming, an integer is a data type that represents whole numbers without any fractional component. C++ provides several integer types that allow developers to choose the most appropriate one based on their needs. The primary integer types in C++ include:
- `int`: Typically the standard choice for whole numbers.
- `short`: Used for smaller integers, often taking less memory than `int`.
- `long`: Suitable for larger integers.
- `long long`: Designed to handle very large integers.
Each type has its specific use cases, but understanding the maximum and minimum limits is crucial to avoid unexpected behaviors, such as overflow.
Integer Sizes and Limits
Different integer types in C++ have varying sizes, which determines their range of values. Typically:
- `int`: Usually occupies 4 bytes, allowing for a maximum value of `2,147,483,647`.
- `short`: Generally 2 bytes, with a limit of `32,767`.
- `long`: Often 4 bytes but can be 8 bytes on 64-bit systems, with limits upwards of `2,147,483,647`.
- `long long`: Usually 8 bytes with a limit of `9,223,372,036,854,775,807`.
Knowing the limits helps in making informed decisions when working with large datasets or performing arithmetic operations.
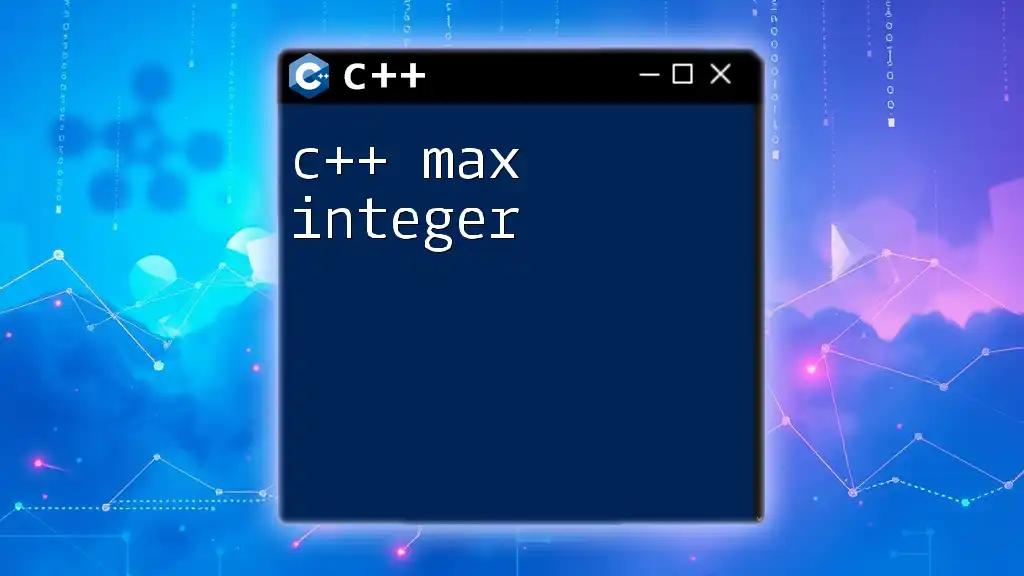
Understanding `INT_MAX`
What is `INT_MAX`?
`INT_MAX` is a macro defined in the `<limits.h>` header file that represents the maximum value an integer can hold in C++. It plays a significant role in preventing errors, especially in arithmetic operations and algorithms that could exceed or stack up past this limit.
Location of INT_MAX
To find the definition of `INT_MAX`, developers should refer to the `<limits>` header file, which provides various constant values related to data types, including maximum and minimum values.
Example of INT_MAX
To demonstrate how `INT_MAX` works, consider the following code snippet:
#include <iostream>
#include <limits>
int main() {
std::cout << "The maximum value of int is: " << std::numeric_limits<int>::max() << std::endl;
return 0;
}
This code outputs the maximum value an integer can have. This information is essential for developers when designing systems that rely on numerical calculations, ensuring that they account for the limits imposed by data types.
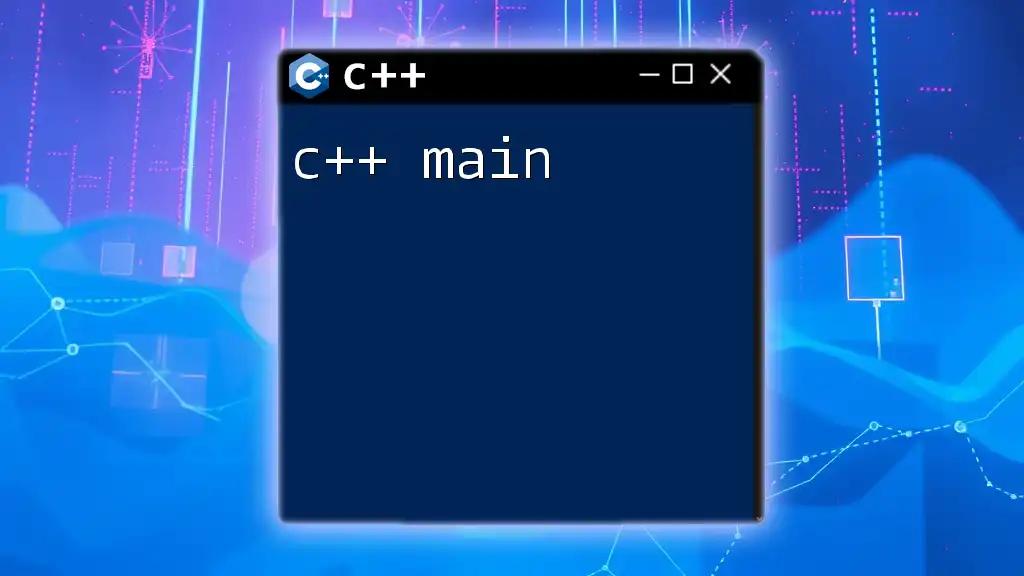
Practical Applications of INT_MAX
Using INT_MAX in Code
Checking against `INT_MAX` is crucial in various programming situations. For example, consider the scenario of adding two integers:
#include <iostream>
#include <limits>
int main() {
int a = INT_MAX;
int b = 1;
if (a + b > INT_MAX) {
std::cout << "Overflow detected!" << std::endl;
} else {
std::cout << "No overflow." << std::endl;
}
return 0;
}
In this example, when `a` (set to `INT_MAX`) is incremented by `1`, the condition checks for overflow. Recognizing overflow prevents potential errors in applications.
Handling Overflows
Understanding how to handle overflows is essential in C++. Developers should implement checks around arithmetic operations involving integers, especially where unexpected numerical growth can occur.
- Use conditions to check values against `INT_MAX` for any mathematical operations.
- Consider alternative data types or structures if you anticipate encountering large integers frequently.

Comparison with Other Integer Types
MAX Limits of Other Integer Types
When discussing `c++ max int`, it’s helpful to compare `INT_MAX` against the maximum values for other integer types:
#include <iostream>
#include <limits>
int main() {
std::cout << "Max short: " << std::numeric_limits<short>::max() << std::endl;
std::cout << "Max int: " << std::numeric_limits<int>::max() << std::endl;
std::cout << "Max long: " << std::numeric_limits<long>::max() << std::endl;
std::cout << "Max long long: " << std::numeric_limits<long long>::max() << std::endl;
return 0;
}
This code snippet reveals the various limits associated with different integer types. Understanding these maximum values allows developers to optimize storage and processing.

Performance Considerations
Why INT_MAX Matters
Knowing about `INT_MAX` is not just theoretical; it holds practical significance. For example, in sorting algorithms or combinatorial calculations, exceeding integer limits can cause incorrect results or crashes. Therefore, frameworks built around algorithms must consider these factors.
Real-world Scenarios
In real-world computational scenarios, such as handling monetary values, tracking large-scale datasets, or using mathematical computations extensively, the implications of `INT_MAX` can lead to significant impacts. Here, performing arithmetic tests using `INT_MAX` can ensure stability and correctness in your applications.
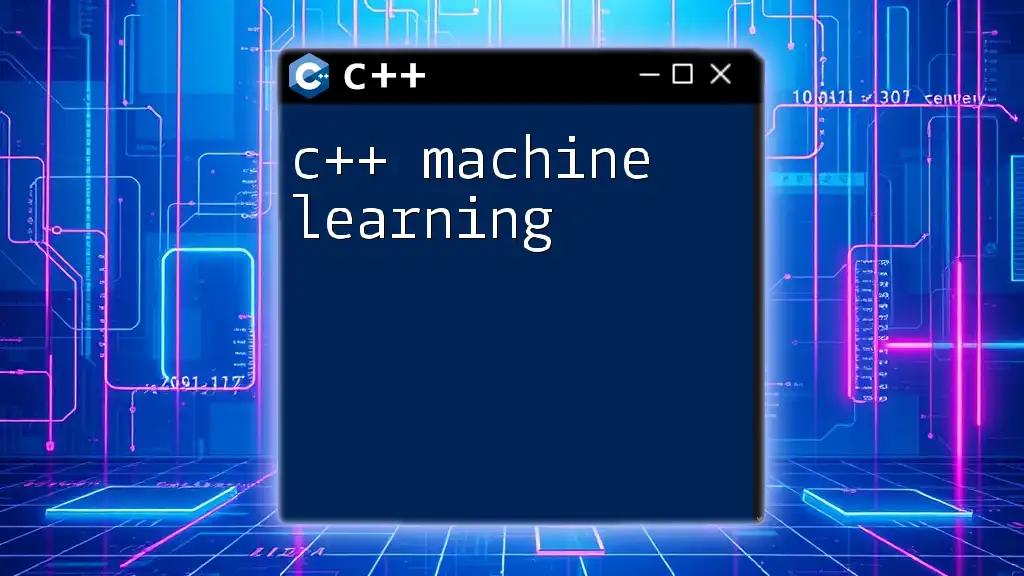
Conclusion
In summary, understanding `c++ max int` and `INT_MAX` is paramount for effective C++ programming. Developers must remain vigilant about data type limits and implement checks to ensure their applications function correctly without encountering undefined behavior. Educating yourself about these concepts not only improves your coding skills but also leads to more robust software design.

Additional Resources
For those wanting to delve deeper into C++ integer types and memory handling, consider exploring a range of books, articles, or online tutorials. Engaging with community forums can also provide valuable insights and assistance as you continue your programming journey.