A C++ max heap is a complete binary tree where each node's value is greater than or equal to the values of its children, ensuring that the maximum value is always at the root.
#include <iostream>
#include <queue>
#include <vector>
int main() {
std::priority_queue<int> maxHeap; // Create a max heap
maxHeap.push(10);
maxHeap.push(20);
maxHeap.push(15);
std::cout << "Max element: " << maxHeap.top() << std::endl; // Output: 20
return 0;
}
What is a Heap?
A heap is a specialized tree-based data structure that satisfies the heap property. In simple terms, this means that it stores elements in a way that allows for efficient access to the largest (in a max heap) or smallest (in a min heap) element. Heaps are commonly implemented using binary trees and are particularly useful in algorithms where priority is essential.
In comparison to other data structures like arrays or binary trees, heaps allow for faster retrieval of extremal values, making them indispensable in various applications such as priority queues.

Types of Heaps
There are primarily two types of heaps: max heaps and min heaps. A max heap is structured such that every parent node is greater than or equal to its child nodes, while a min heap ensures that every parent node is less than or equal to its children. The max heap is typically used in scenarios where we need quick access to the maximum element.
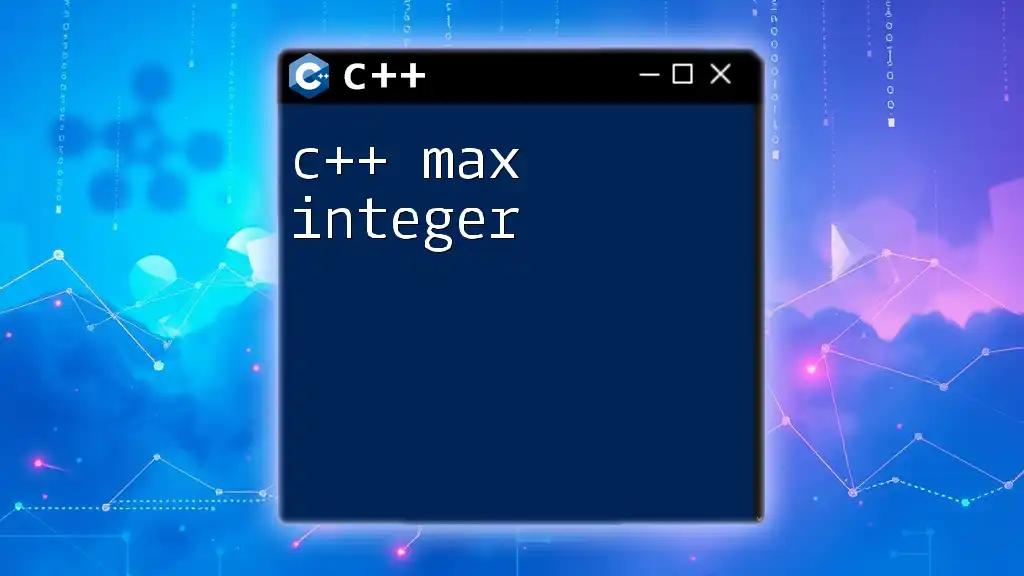
Understanding Max Heaps
What is a Max Heap?
A max heap is a complete binary tree where each node's value is greater than or equal to the values of its children. This organizational structure allows the largest element to be at the root of the tree.
For example, in the following max heap:
10
/ \
8 9
/ \ / \
4 7 3 2
The root node holds the highest value (10), and both of its children (8 and 9) hold values that are less than or equal to it.
Applications of Max Heaps
Max heaps are widely used in algorithms, particularly in:
- Heap Sort: An efficient sorting algorithm that utilizes the properties of heaps.
- Priority Queues: Which allow efficient retrieval of the highest priority element.
Real-world applications include scheduling systems and resource management, where prioritization is crucial.

Max Heapify in C++
What is Max Heapify?
Max Heapify is a crucial operation that ensures that the subtree rooted at a given index satisfies the max heap property. It is often used during situations where the structure of the heap gets disrupted, such as after inserting or removing an element.
Max Heapify Algorithm
The max heapify algorithm works by repeatedly checking the node's children and swapping values to maintain the max heap property. If a child node violates this property, the algorithm swaps the values and recursively enforces the property down the tree.
Sample Code Snippet for Max Heapify
void maxHeapify(int arr[], int n, int i) {
int largest = i;
int left = 2 * i + 1;
int right = 2 * i + 2;
if (left < n && arr[left] > arr[largest])
largest = left;
if (right < n && arr[right] > arr[largest])
largest = right;
if (largest != i) {
swap(arr[i], arr[largest]);
maxHeapify(arr, n, largest);
}
}
Explanation of the Code
- The function `maxHeapify` takes an array `arr`, the number of elements `n`, and the current index `i`.
- It initializes the largest variable to the index being checked.
- The left and right children indices are calculated, and comparisons are made to determine the largest value.
- If the largest value is not at the current index, a swap occurs, and max heapify is called recursively on the affected subtree.

Building a Max Heap in C++
Prerequisites for Building a Max Heap
To build a max heap, the underlying data must be structured in an array format. You should ensure that the array can represent a complete binary tree.
Building a Max Heap using Max Heapify
The max heap can be built efficiently by applying the max heapify operation to each non-leaf node in the tree. By starting from the last non-leaf node and working upwards, we can ensure that the entire tree satisfies the max heap property.
Sample Code Snippet to Build a Max Heap
void buildMaxHeap(int arr[], int n) {
for (int i = n / 2 - 1; i >= 0; i--) {
maxHeapify(arr, n, i);
}
}
Explanation of the Code
- The `buildMaxHeap` function iterates from the last non-leaf node down to the root.
- It calls the `maxHeapify` function on each node, recursively ensuring that each subtree satisfies the max heap property.

Inserting an Element into Max Heap
Insertion Process Overview
When inserting an element into a max heap, it is crucial to maintain the max heap property. The new element is initially added at the end of the heap and is then "bubbled up" to its appropriate position.
Sample Code Snippet for Insertion
void insertNode(int arr[], int& n, int key) {
n++;
arr[n - 1] = key;
int i = n - 1;
while (i != 0 && arr[(i - 1) / 2] < arr[i]) {
swap(arr[i], arr[(i - 1) / 2]);
i = (i - 1) / 2;
}
}
Explanation of the Code
- The function `insertNode` takes the array `arr`, increments the size `n`, and assigns the new key to the last position.
- It then compares the inserted element with its parent and swaps them if the inserted element is larger, continuing this process until the correct position is found.
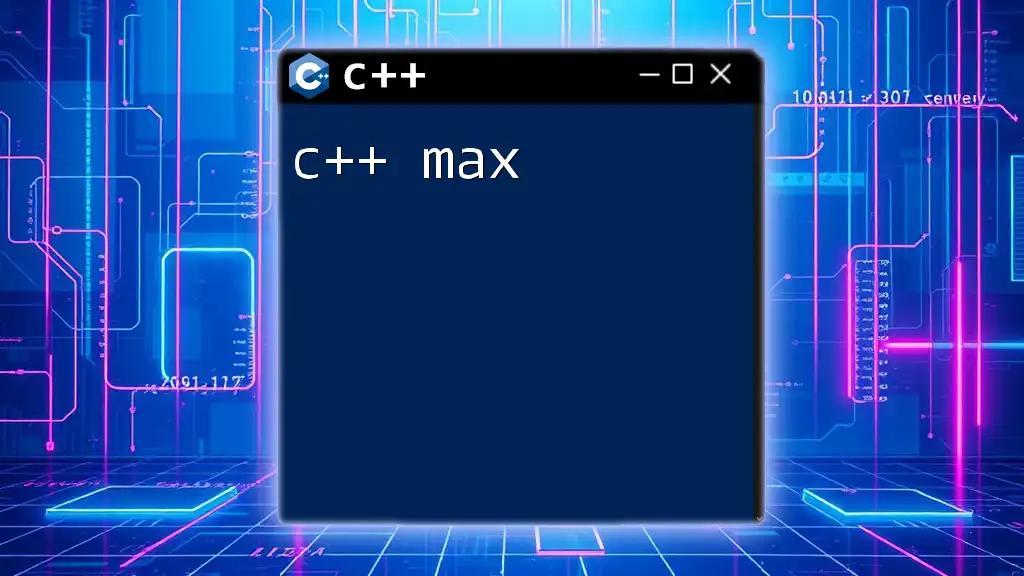
Deleting the Max Element from Max Heap
Deletion Process Overview
Deleting the maximum element (the root of the max heap) is straightforward. The root is replaced with the last element in the heap, and max heapify is called to restore the max heap property.
Sample Code Snippet for Deletion
void deleteMax(int arr[], int& n) {
if (n <= 0) return;
if (n == 1) {
n--;
return;
}
arr[0] = arr[n - 1];
n--;
maxHeapify(arr, n, 0);
}
Explanation of the Code
- The `deleteMax` function checks if the heap is empty or has only one element. If the heap has more than one element, it replaces the root with the last element in the array.
- After decreasing the size of the heap, `maxHeapify` is invoked to restore the max heap property starting from the root.

Implementing Heap Sort with Max Heap
Understanding Heap Sort Algorithm
Heap sort is a sorting algorithm that utilizes the properties of a max heap to sort an array. By building a max heap and repeatedly removing the maximum element, we can produce a sorted array.
Implementation of Heap Sort
void heapSort(int arr[], int n) {
buildMaxHeap(arr, n);
for (int i = n - 1; i > 0; i--) {
swap(arr[0], arr[i]);
maxHeapify(arr, i, 0);
}
}
Step-by-Step Breakdown
- The `heapSort` function first builds a max heap from the input array.
- It then iterates from the end of the array to the beginning, swapping the root of the heap with the last element.
- After each swap, it calls `maxHeapify` to ensure that the remaining elements still fulfill the max heap property.
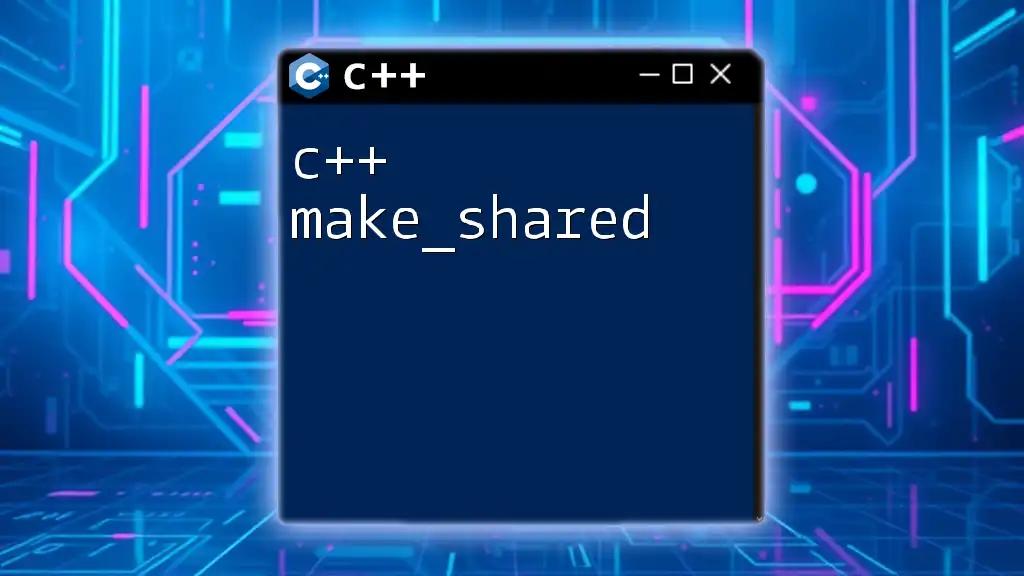
Conclusion
In summary, C++ max heaps are powerful data structures that allow for efficient retrieval and management of the largest elements. Understanding how to implement the max heap, along with operations like `maxHeapify`, insertion, and deletion processes, equips you with the tools needed for efficient sorting and prioritization in programming.
For those eager to master max heaps further, practicing through problem-solving and utilizing online resources can enhance your understanding and application of this essential concept in computer science.