In C++, "managed" typically refers to using the C++/CLI language extensions that allow for the integration of C++ with the .NET framework, enabling the use of garbage collection and other managed features.
Here's a simple code snippet demonstrating how to define a managed class in C++/CLI:
using namespace System;
public ref class ManagedExample
{
public:
void DisplayMessage()
{
Console::WriteLine("Hello from Managed C++!");
}
};
What is Managed C++?
Managed C++ is an extension of the C++ programming language that enables developers to incorporate the features of the .NET framework alongside traditional C++. It facilitates the seamless interaction between unmanaged C++ code and managed code within the CLR (Common Language Runtime).
Originally introduced in Visual C++ .NET, Managed C++ allows for the creation of applications that can leverage both the performance of C++ and the robustness of .NET functionalities. The core purpose of Managed C++ is to facilitate the migration of existing C++ codebases into a managed environment while offering a path for developing new applications using both paradigms.
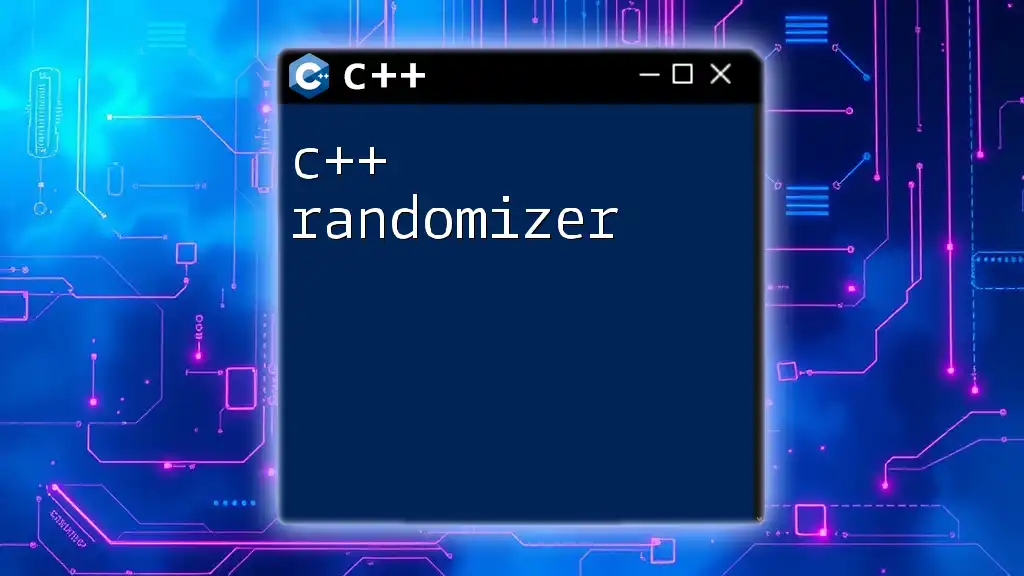
The Purpose of Managed C++
One of the primary advantages of using managed code is automatic memory management through garbage collection, which significantly reduces the risk of memory leaks and pointer errors that are often prevalent in unmanaged C++. Managed C++ is also beneficial in scenarios where developers wish to take advantage of the extensive libraries and tools available within the .NET ecosystem, such as Windows Forms, WPF, and ASP.NET.
Common use cases for Managed C++ include:
- Migrating legacy C++ applications to .NET
- Creating GUI applications that require rapid development and deployment
- Integrating C++ code with .NET libraries for enhanced functionality
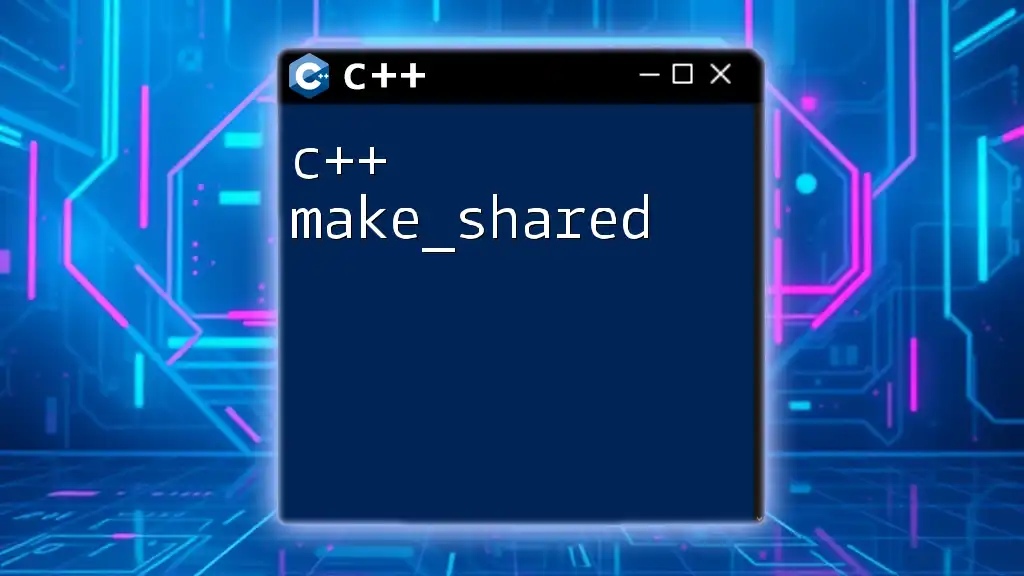
Understanding Managed Code in C++
What is Managed Code?
Managed code refers to the code that runs under the control of the CLR, which provides several core services such as memory management, exception handling, and type safety. In contrast, unmanaged code is executed directly by the operating system without such provisions, making it more prone to memory-related errors.
Characteristics of managed code include:
- Automatic garbage collection: The CLR takes care of freeing up unused memory and managing object lifetimes.
- Type safety: CLR checks the types of all objects at runtime, minimizing the risk of type-related errors.
- Intermediate Language (IL): Managed code is typically compiled into IL, which the runtime environment translates to machine code at execution.
The Role of Common Language Runtime (CLR)
The Common Language Runtime (CLR) is fundamental to managed code and serves as the execution environment for all .NET applications. It plays a pivotal role in memory management, type safety, and interoperability.
The CLR affects how resources are allocated and freed. Instead of manual memory management, the CLR uses a Garbage Collector (GC) to automatically reclaim memory that is no longer in use, significantly simplifying resource management for developers.
An example of how CLR operates can be illustrated with managed object creation:
// Example of creating a managed object in Managed C++
String^ greeting = gcnew String("Hello, CLR!");
In this example, `gcnew` is a unique keyword that indicates the compiler to create a managed instance, thus ensuring that memory allocation is handled by the CLR.
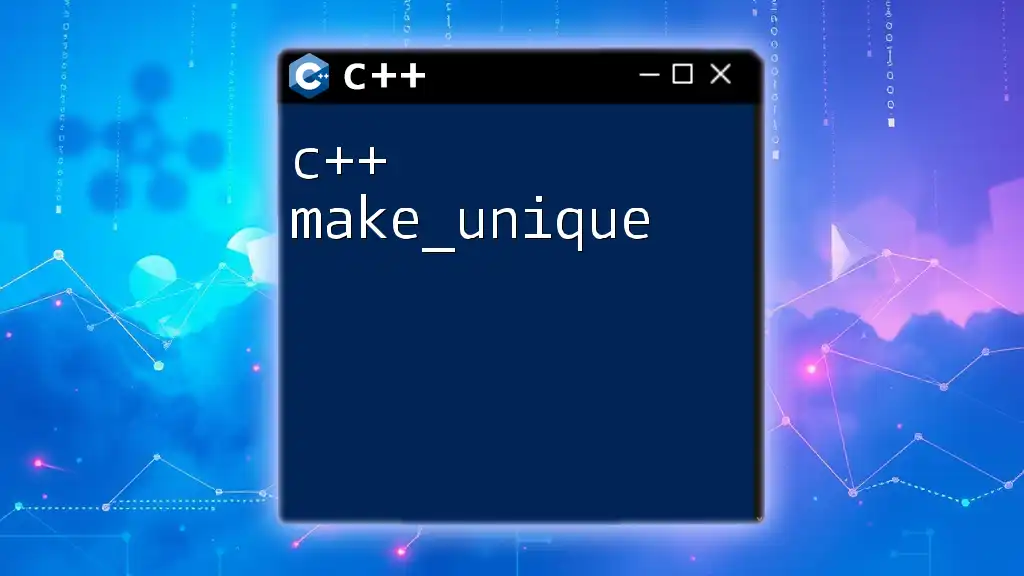
Managed C++ Syntax and Structure
Basic Syntax of Managed C++
Managed C++ syntax resembles traditional C++ but incorporates new elements that bridge the gap with the .NET environment. Below is an overview of some syntactic features unique to Managed C++:
// Example of managed C++ syntax overview
using namespace System;
void main() {
String^ myString = gcnew String("Managed C++ Example");
Console::WriteLine(myString);
}
Notice the use of `^` when declaring `myString`, which denotes that it is a handle to a managed object. This handle-type distinction is essential in Managed C++.
Important Keywords and Constructs
Some critical keywords and constructs unique to managed C++ include:
- `^`: This symbol indicates a handle to a managed object.
- `gcnew`: This operator is utilized for allocating managed objects.
- `::`: This scope resolution operator is used to access members of .NET namespaces or classes.
These constructs allow for more intuitive manipulation of managed objects within a C++ environment while ensuring compatibility with the .NET framework.
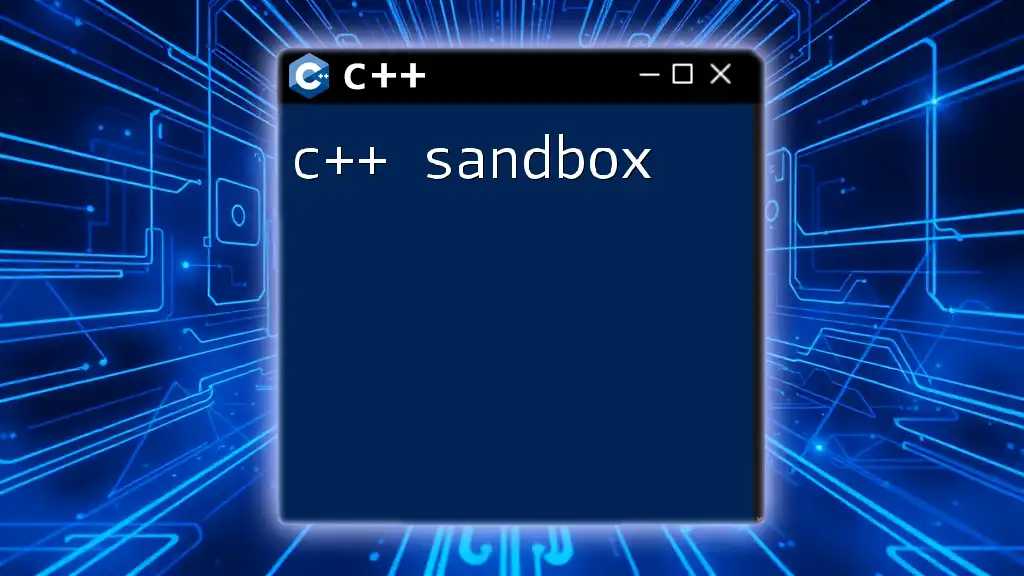
Memory Management in Managed C++
Garbage Collection in Managed C++
One of the most significant advantages of managed code is garbage collection. In Managed C++, developers do not need to manually allocate and free memory, as the garbage collector automatically tracks object lifetimes. This mechanism reduces common issues in unmanaged C++, such as dangling pointers and memory leaks.
When an object is no longer referenced, the garbage collector considers it for cleanup, allowing developers to write more robust and reliable code.
Creating and Using Managed Objects
Creating managed objects in Managed C++ is straightforward, thanks to the `gcnew` keyword:
// Creating a managed object
MyClass^ obj = gcnew MyClass();
In this case, `obj` is a handle to an instance of `MyClass`, and the garbage collector will automatically manage its lifecycle. This separation of managed and unmanaged code allows for cleaner interaction with various frameworks and libraries.
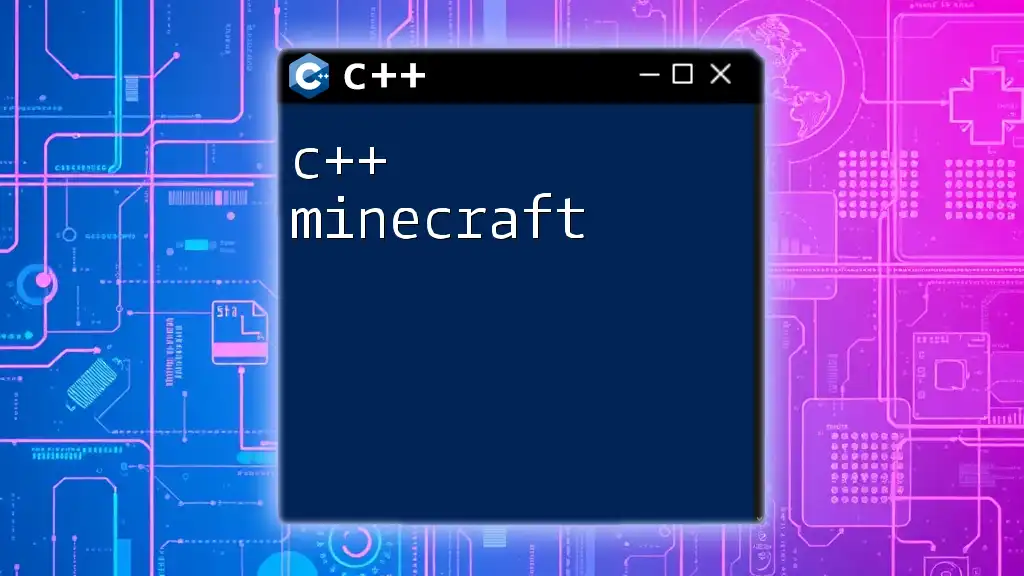
Exception Handling in Managed C++
Mechanisms of Exception Handling
Managed C++ simplifies exception handling with constructs like `try`, `catch`, and `finally`, allowing developers to write robust error handling logic. This structured approach to error management contrasts with the traditional error-handling mechanisms in unmanaged C++.
Here’s an example:
try {
// Some code that may throw an exception
int result = 10 / 0; // Forcing a divide by zero error
}
catch (Exception^ ex) {
Console::WriteLine("An error occurred: {0}", ex->Message);
}
In this example, if an exception is thrown, the execution jumps to the catch block, where the error message can be handled gracefully.
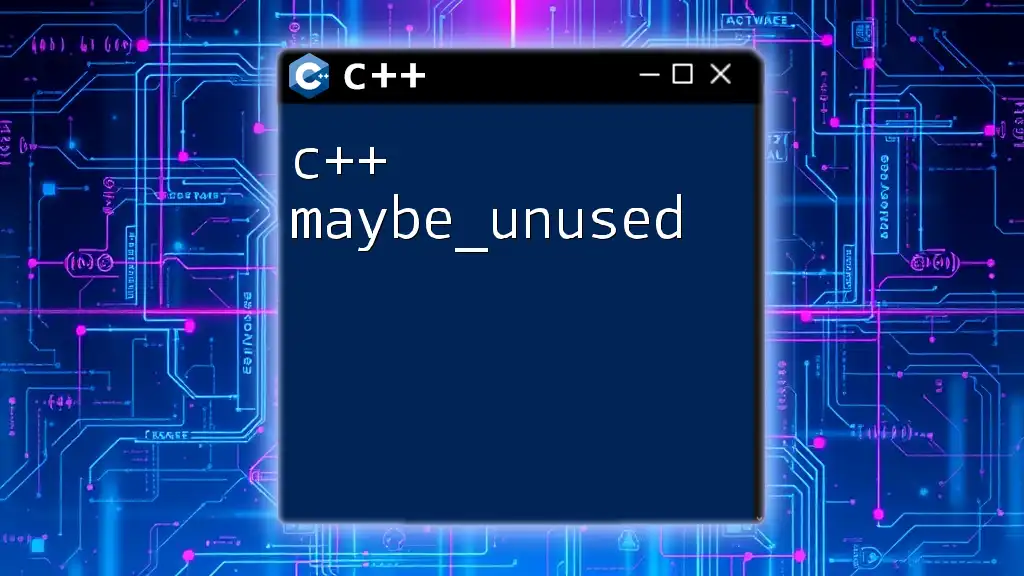
Application Scenarios for Managed C++
Integration with .NET Framework
Managed C++ shines in scenarios where integration with the .NET framework is essential. For instance, it allows you to create Windows Forms applications that utilize both C++ performance and .NET capabilities seamlessly:
// Managed C++ integration example
using namespace System;
using namespace System::Windows::Forms;
void main() {
Application::EnableVisualStyles();
Application::Run(gcnew Form());
}
This simple example demonstrates how easy it is to create graphical applications using managed C++.
When to Use Managed C++
Managed C++ is particularly useful in the following scenarios:
- When needing to leverage existing C++ libraries while also utilizing .NET features.
- When focusing on rapid application development with GUI frameworks.
- In environments where safety and stability are a primary concern, reducing the risk of memory-related bugs.
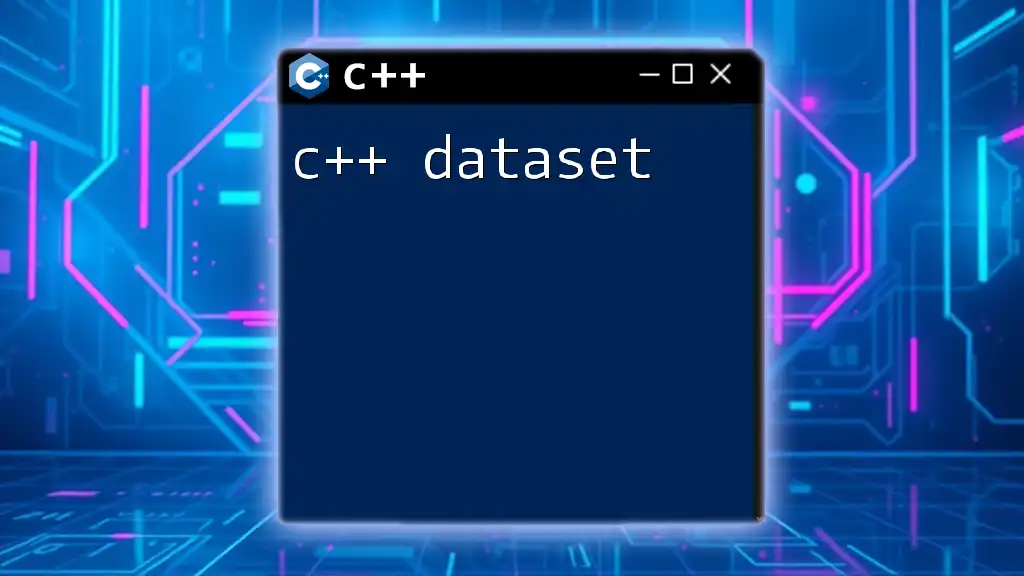
Best Practices for Working with Managed C++
Code Optimization Techniques
To write efficient managed code, consider the following best practices:
- Minimize the use of `gcnew` in tight loops to reduce the load on the garbage collector.
- Be cautious about creating excessive short-lived objects, as they can lead to frequent garbage collection cycles.
- Use value types judiciously; they are allocated on the stack and can improve performance when used correctly.
Navigating Dependencies and Libraries
Utilizing existing libraries within Managed C++ can often lead to significant productivity gains. By importing .NET libraries, you can extend the functionality of your code base without reinventing the wheel.
To use a .NET library, include it in your project references, then use the following syntax:
// Importing and using a .NET library
using namespace MyDotNetLibrary;
MyLibraryClass^ obj = gcnew MyLibraryClass();
obj->SomeMethod();
This approach encapsulates the power of C++ with the rich feature set of .NET.
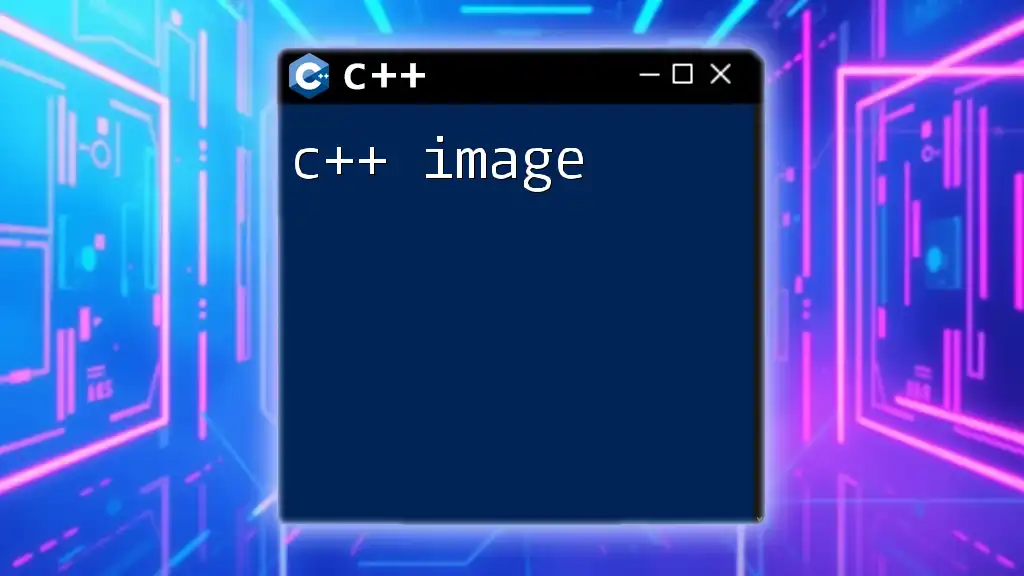
Conclusion: Embracing Managed C++
Future of C++ and Managed Code
As development continues to evolve, Managed C++ remains a critical tool for developers who wish to leverage the robustness of .NET while enjoying the performance benefits of C++. While the programming landscape has many alternatives, the specific needs of certain applications make Managed C++ an enduring option.
Key Takeaways
In summary, Managed C++ offers a unique combination of performance and safety, allowing developers to write code that is both powerful and less prone to errors. By understanding its core principles, syntax, and frameworks, programmers can effectively navigate the intricacies of Managed C++ and build reliable applications that interact seamlessly with .NET environments.
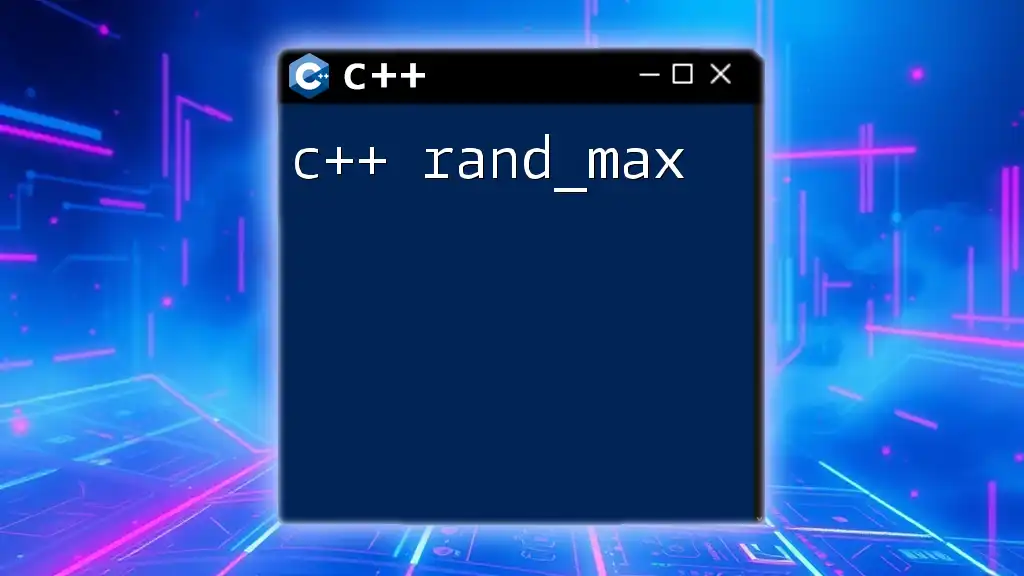
Additional Resources
Books and Online Courses
For those interested in deepening their knowledge of Managed C++, several resources are available, including in-depth books on C++ and .NET frameworks, along with online tutorials and courses tailored to different learning levels.
Community and Support
Engaging with developer communities on forums, social media, or online groups can provide invaluable insights and support for those working with Managed C++. Collaborating with peers often leads to unexpected discoveries and optimizations in coding practices.