The `RAND_MAX` constant in C++ defines the maximum value that can be returned by the `rand()` function, which generates pseudo-random numbers.
#include <iostream>
#include <cstdlib>
int main() {
std::cout << "Maximum value for rand(): " << RAND_MAX << std::endl;
return 0;
}
Understanding Random Number Generation in C++
What is Random Number Generation?
Random number generation is a fundamental concept in programming that involves producing a sequence of numbers that lacks any predictable pattern. In simpler terms, it allows a program to generate numbers that appear random to the user. Random numbers are widely used in various applications, from games to simulations and cryptography.
The Standard Library's Pseudorandom Number Generator
In C++, random number generation is facilitated by the standard library. Specifically, the `<cstdlib>` header provides convenient functions for generating random numbers, while `<ctime>` can be used to seed the random number generator to ensure a different sequence every time a program runs. Understanding how these libraries work is essential for effectively utilizing random numbers in your applications.
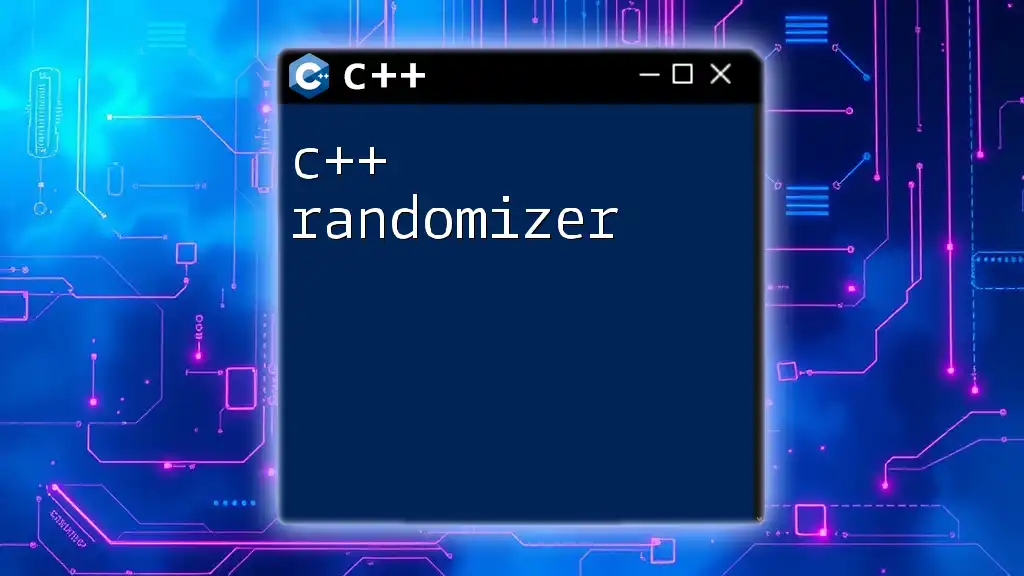
Introduction to `RAND_MAX`
What is `RAND_MAX`?
`RAND_MAX` is a constant defined in the C++ `<cstdlib>` library that represents the maximum value that can be returned by the `rand()` function. This value is often implementation-defined, but it is typically set to 32767 in many systems, meaning that calls to `rand()` will generate numbers from 0 up to `RAND_MAX`.
The Range of Random Values
When you call the `rand()` function, the generated random number is bounded by `RAND_MAX`. This implies that if you were to execute the following code:
#include <iostream>
#include <cstdlib>
int main() {
std::cout << "Random number: " << rand() << std::endl;
return 0;
}
The output will always be between 0 and `RAND_MAX` (inclusive). Understanding this range is crucial for developers who intend to use these random values in practical applications, especially when they need random numbers to fall within specific limits.
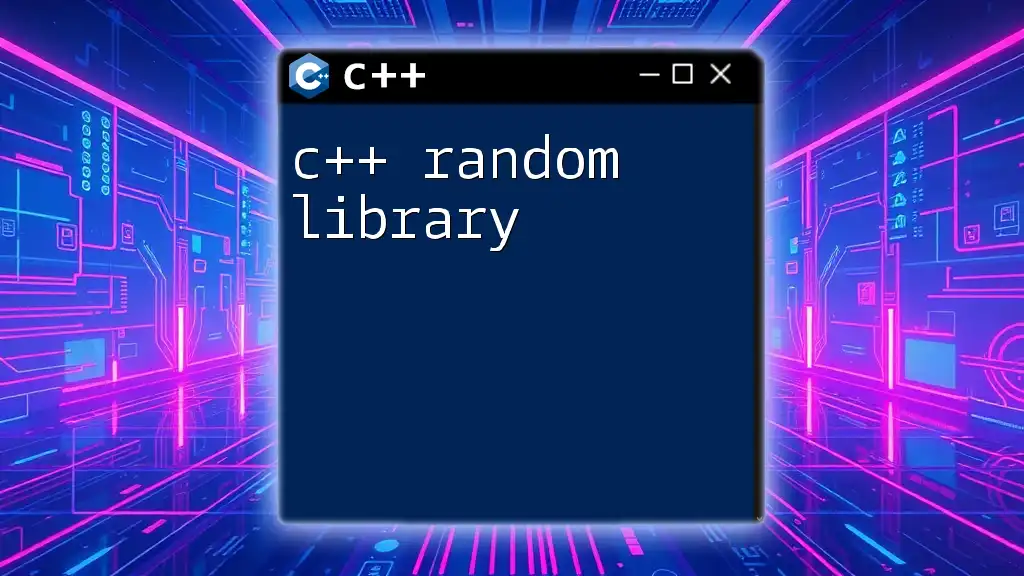
Using `RAND_MAX` with `rand()`
Generating a Random Number
To generate a random number with `rand()`, a fundamental understanding of the procedure is essential. The simplest use of `rand()` will yield a number in the range mentioned earlier. To demonstrate this, the following code snippet generates and prints a random number:
#include <iostream>
#include <cstdlib>
int main() {
std::cout << "Random number: " << rand() << std::endl;
return 0;
}
This piece of code simply invokes `rand()` and prints the generated number.
Normalizing the Random Value
When working with random numbers, you often need to map them to a specific range. This process ensures that the generated random numbers satisfy certain constraints relevant to your application. A common approach is to use the modulo operation combined with an offset.
For example, consider the need to generate a random number between `min` and `max`. Here's how you can achieve this using `RAND_MAX`:
int min = 1;
int max = 100;
int randomValue = min + (rand() % (max - min + 1));
In this code, `rand() % (max - min + 1)` generates a number from 0 to `(max - min)`, and then we add `min` to shift this range suitably. Hence, `randomValue` will always fall within 1 to 100.
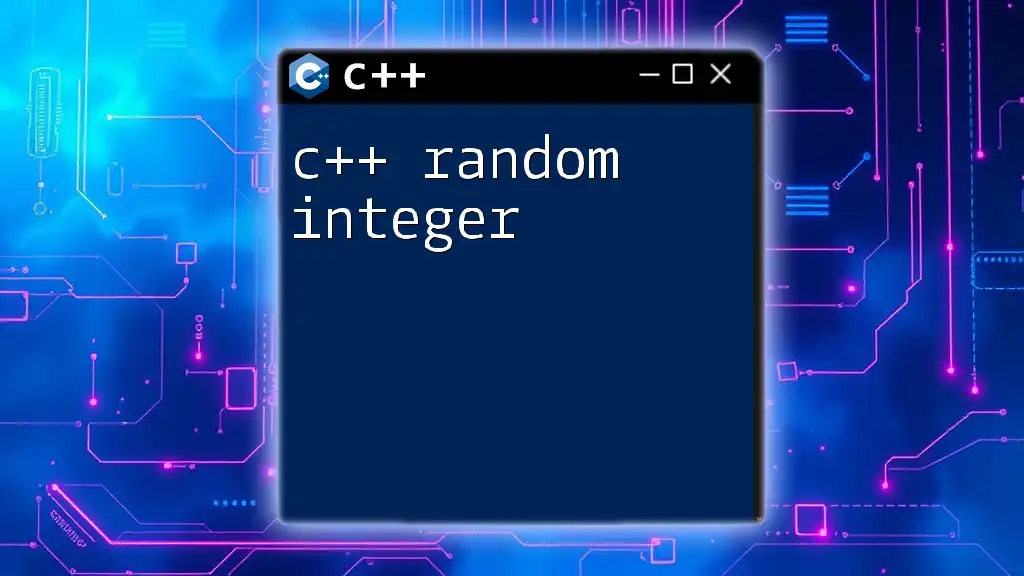
Practical Application of `RAND_MAX`
Simple Game Example
One practical application of generating random numbers is creating a guessing game. In this simple game, the player tries to guess a randomly chosen number within a specified range. Here’s how this can be implemented:
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
srand(static_cast<unsigned int>(time(0))); // Seed the random number generator
int min = 1, max = 100;
int number = min + (rand() % (max - min + 1));
int guess;
std::cout << "Guess the number (between " << min << " and " << max << "): ";
std::cin >> guess;
if (guess == number) {
std::cout << "Congratulations! You guessed it right." << std::endl;
} else {
std::cout << "Sorry! The correct number was " << number << "." << std::endl;
}
return 0;
}
In this example, the program starts by seeding the random number generator with the current time to ensure different sequences of random numbers on each execution. The player guesses a number between 1 and 100, and the program tells them whether they have guessed correctly. This demonstrates a simple yet effective way of utilizing `RAND_MAX` and `rand()` in a meaningful application.
Avoiding Bias in Random Number Generation
While `rand()` serves many purposes, it’s essential to be aware of its limitations. It is a pseudorandom number generator, which can lead to patterns if not used properly. It’s crucial to use `srand()` to seed the generator with varying values (like the current time) before calling `rand()`. Otherwise, your program may produce the same sequence of numbers upon every run.
Moreover, the randomness quality of `rand()` can be insufficient for high-stakes applications like cryptography. Best practices involve ensuring adequate seeding and considering the distribution of outcomes.
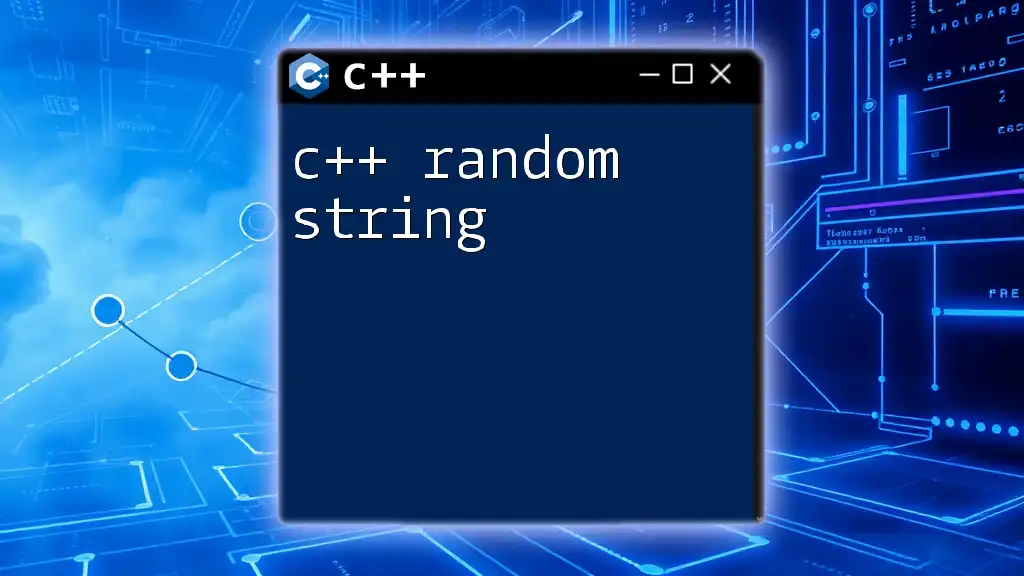
Advanced Random Number Generation Techniques
C++11 and Beyond: Introducing `<random>`
Starting with C++11, the language introduced a more robust library for random number generation: `<random>`. This library provides more options for generating truly random numbers, including various distributions and engines. Using `<random>` not only yields a better quality of randomness but also gives developers finer control over the generation process.
Using `<random>` to Improve on `rand()`
The following code snippet demonstrates how to use the modern `<random>` library to create random numbers:
#include <iostream>
#include <random>
int main() {
std::random_device rd;
std::mt19937 gen(rd());
std::uniform_int_distribution<> dis(1, 100);
std::cout << "Random number: " << dis(gen) << std::endl;
return 0;
}
Here, `std::random_device` provides a non-deterministic random seed, while `std::mt19937` is a Mersenne Twister engine. The `std::uniform_int_distribution` ensures that numbers generated lie uniformly within the specified range of 1 to 100.
This approach not only enhances randomness but also avoids the potential biases associated with `rand()`.
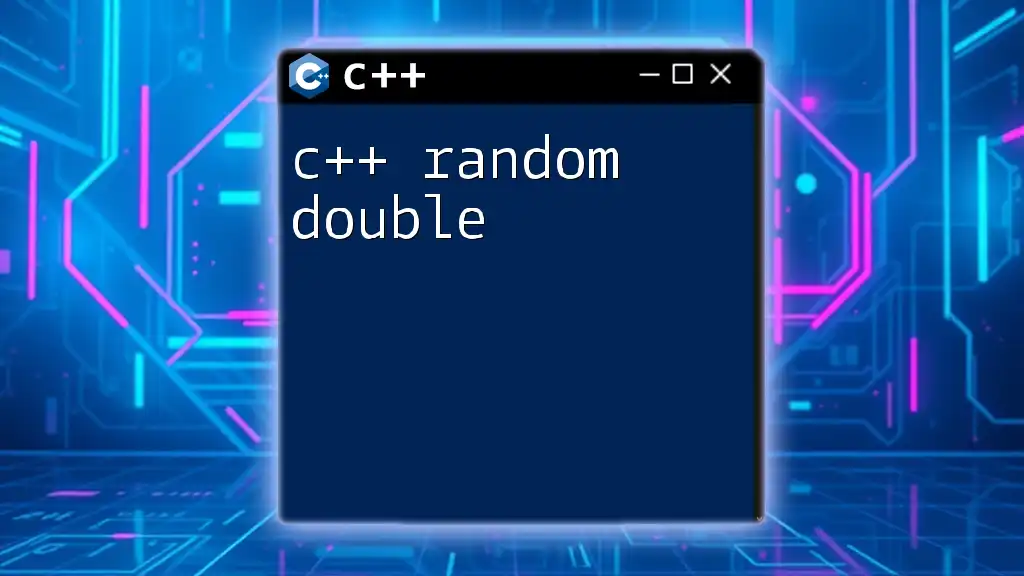
Conclusion
In conclusion, `RAND_MAX` plays a pivotal role in random number generation in C++. Understanding its value and how to utilize it effectively with the `rand()` function is essential for generating random numbers that serve various applications.
However, as C++ evolves, the introduction of `<random>` provides more advanced capabilities, allowing developers to achieve better randomness with more control. As the landscape of C++ continues to change, embracing these improvements will significantly enhance the quality of your random number generation techniques.
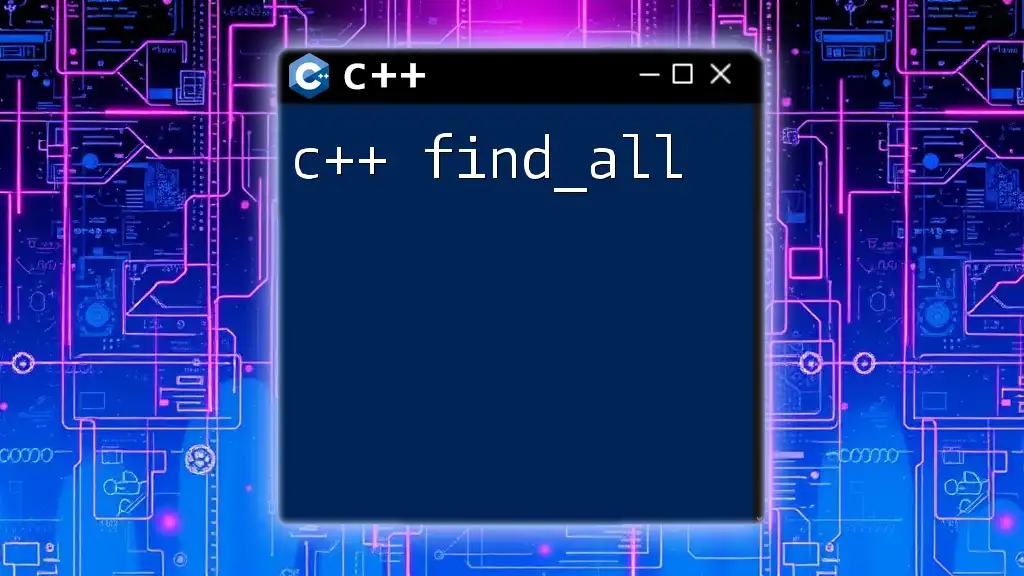
Additional Resources
For those eager to delve deeper into random number generation in C++, several resources are available, including official documentation, online tutorials, and comprehensive guides. Exploring these materials will cement your understanding and help improve your expertise in this vital programming area.