To generate a random number between 1 and 100 in C++, you can use the `<cstdlib>` library along with the `<ctime>` library to seed the random number generator. Here's a simple code snippet:
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
std::srand(std::time(0)); // Seed the random number generator
int randomNumber = std::rand() % 100 + 1; // Generate random number between 1 and 100
std::cout << "Random number: " << randomNumber << std::endl;
return 0;
}
Understanding Random Number Generation in C++
Random number generation is a fundamental concept in programming, used in various applications such as simulations, gaming, cryptography, and more. In C++, generating a random number entails utilizing built-in functions and libraries that facilitate this process. Understanding how random number generation works will help you implement it correctly and efficiently in your projects.

Libraries Required
For generating random numbers in C++, you will typically use two libraries: `<cstdlib>` and `<ctime>`.
- `<cstdlib>` provides functions to generate random numbers using the `rand()` function.
- `<ctime>` allows us to obtain the current time, which we can use as a seed for our random number generator.
To get started, include these libraries in your program:
#include <iostream>
#include <cstdlib>
#include <ctime>

How to Generate a Random Number
Initializing the Random Seed
Before you generate random numbers, you must initialize the random seed. The seed is crucial because it influences the sequence of random numbers generated by `rand()`. If you do not change the seed, `rand()` will produce the same number each time you run the program.
By seeding the random number generator with the current time, you ensure that the sequence changes on each run:
std::srand(std::time(0)); // Seed with current time
Generating a Random Number Between 1 and 100
Now that we have seeded the random number generator, we can easily generate a random number between 1 and 100. The key to achieving this range is to use the `rand()` function in conjunction with the modulus operator:
- `rand()` generates a random integer between 0 and `RAND_MAX`.
- To restrict this to a specific range, use the modulus operator combined with an offset.
Here’s the formula:
int randomNumber = std::rand() % 100 + 1; // Range from 1 to 100
This expression will yield a random number from 0 to 99, and adding 1 shifts the range to 1 to 100.

Practical Example
Full Code Example
Combining what we have discussed, here is a complete program that generates and displays a random number between 1 and 100:
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
std::srand(std::time(0)); // Seed the random number generator
int randomNumber = std::rand() % 100 + 1; // Generate random number between 1 and 100
std::cout << "Random Number: " << randomNumber << std::endl; // Display the random number
return 0;
}
In this code:
- We include the necessary libraries.
- We seed the random number generator to ensure randomness each time the program runs.
- We generate a random number within the desired range and print it to the console.
Running the Code
To compile and run the C++ code, you can use any C++ compiler. For example, if you're using `g++`, the command would look like this:
g++ random_number.cpp -o random_number
./random_number
Expected output would be something like: `Random Number: 42`. However, the number will change each time you run it.

Common Mistakes to Avoid
Not Seeding the Random Number Generator
One of the most common mistakes is neglecting to seed the random number generator. When omitted, the sequence remains constant across program executions, producing the same random number repeatedly. Always remember to seed to achieve the desired randomness.
Misunderstanding the Range
Another common pitfall is miscalculating the range. Ensure you understand how the modulus operator works with `rand()` to set your desired limits correctly. Remember, the expression `rand() % 100` gives numbers between 0 to 99, so always add 1 for a range of 1 to 100.

Enhancing Randomness in C++
Using `<random>` Library
C++11 introduced a more robust way to generate random numbers through the `<random>` library. It offers better randomness and more sophisticated features, making it the preferred choice for many scenarios.
Here’s how to generate random numbers using the `<random>` library:
#include <iostream>
#include <random>
int main() {
std::default_random_engine generator(std::time(0)); // Initialize the generator with a seed
std::uniform_int_distribution<int> distribution(1, 100); // Define the distribution range
int randomNumber = distribution(generator); // Generate the random number
std::cout << "Random Number: " << randomNumber << std::endl; // Display the random number
return 0;
}
In this code:
- We create a default random number generator.
- We define an integer distribution that specifies our desired range.
- Finally, we generate and display a random number from that range.
Using the `<random>` library not only offers better randomness but also allows easier customization to suit different requirements.

Conclusion
In this guide, we've explored how to generate a C++ random number between 1 and 100 using various approaches, including the basic methods utilizing `<cstdlib>` and the advanced techniques employing `<random>`. Experimenting with these methods will equip you with essential skills in C++ programming and enhance your applications. Don’t hesitate to dive deeper or reach out if you have any questions or need assistance!
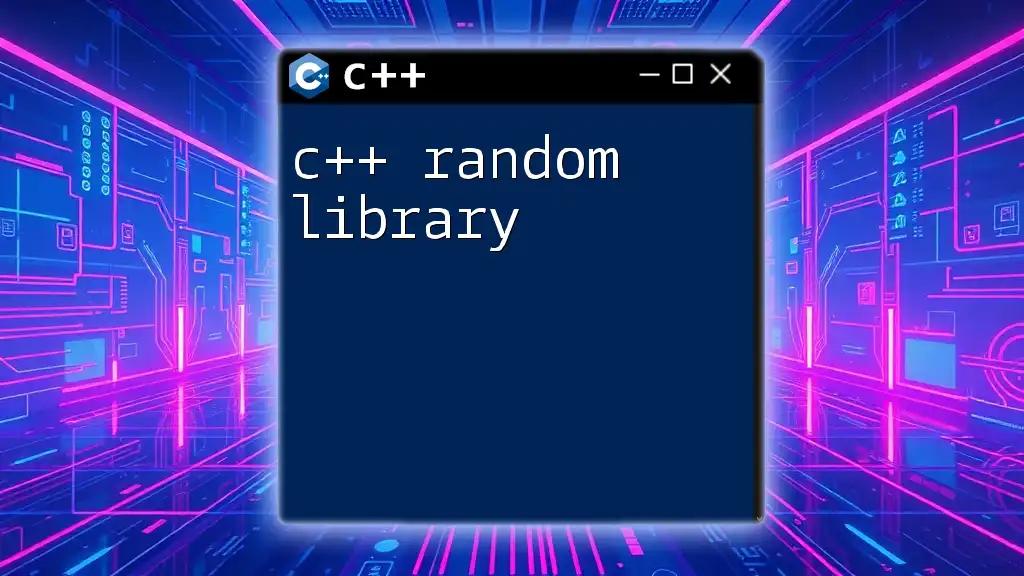
Additional Resources
For more detailed documentation and further reading on random number generation in C++, you can check out:
- [C++ Reference - cstdlib](https://en.cppreference.com/w/cpp/numeric/random/rand)
- [C++ Reference - random](https://en.cppreference.com/w/cpp/numeric/random)
As you grow your knowledge in C++, consider joining our community for more tutorials and workshops on mastering programming concepts!