The primary difference between C and C++ is that C is a procedural programming language that focuses on function and procedure calls, while C++ is an object-oriented programming language that supports encapsulation, inheritance, and polymorphism.
Here’s a simple code snippet demonstrating the difference in handling a basic class in C++:
#include <iostream>
class Greeting {
public:
void sayHello() {
std::cout << "Hello, World!" << std::endl;
}
};
int main() {
Greeting greet;
greet.sayHello();
return 0;
}
What is C?
Definition of C
C is a high-level procedural programming language that was developed in the early 1970s at Bell Labs by Dennis Ritchie. It has a rich set of features that have influenced many other programming languages.
Key Features of C
- Procedural Language: C follows a top-down approach and focuses primarily on structured programming using functions.
- Low-level Manipulation: It allows low-level access to memory and gives programmers greater control through pointers.
- Portability: C code can be compiled on different platforms with minimal changes.
Use Cases of C
C is widely used in various applications, including:
- Embedded Systems: It is commonly used in firmware and for programming microcontrollers due to its efficiency and speed.
- System Programming: Used for operating systems and drivers because of its ability to interact closely with hardware.
- Performance-Critical Applications: In scenarios where speed and resource management are crucial, C remains a popular choice.
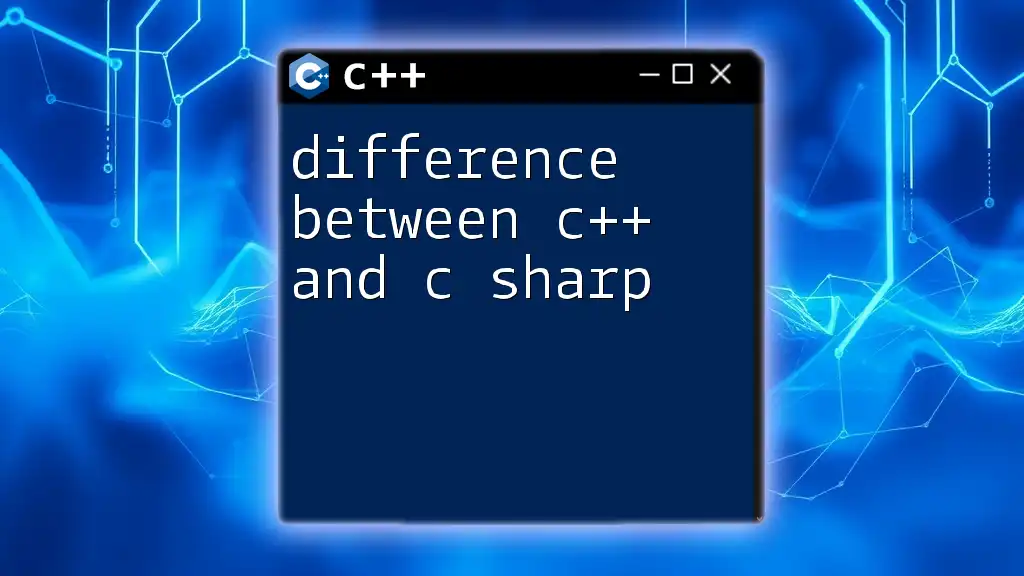
What is C++?
Definition of C++
C++ is an extension of the C programming language developed by Bjarne Stroustrup in the late 1970s. It incorporates object-oriented features alongside the procedural programming concepts from C.
Key Features of C++
- Object-Oriented Programming (OOP): C++ supports OOP principles such as encapsulation, inheritance, and polymorphism, allowing for more modular code.
- Standard Template Library (STL): Provides a rich set of algorithms and data structures to enhance code productivity.
- Function Overloading and Default Arguments: C++ enables functions with the same name but different parameters, improving flexibility and usability.
Use Cases of C++
C++ is preferred for diverse applications, such as:
- Game Development: Its performance and ability to handle complex graphics make it ideal for game engines.
- Application Software: Used in building desktop software where performance and user interface are critical.
- Large Systems with Multiple Modules: C++ supports complex system architecture, making it fit for large-scale applications.
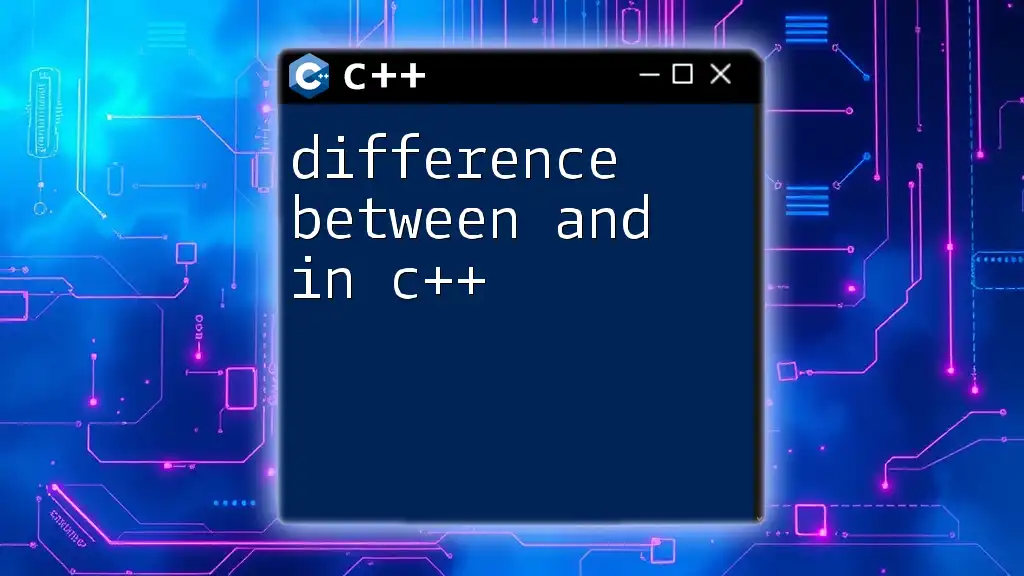
Major Differences Between C and C++
Paradigm Shift
Procedural vs. Object-Oriented Programming
C is fundamentally procedural, promoting structured programming, while C++ introduces object-oriented concepts. In C, functions operate independently of the data they manipulate, whereas C++ allows for bundling data and functions together into objects.
For example, in C:
#include <stdio.h>
void display(int num) {
printf("Number: %d\n", num);
}
int main() {
display(5);
return 0;
}
In C++, you can encapsulate data and behavior:
#include <iostream>
using namespace std;
class Number {
public:
void display(int num) {
cout << "Number: " << num << endl;
}
};
int main() {
Number n;
n.display(5);
return 0;
}
Syntax and Features
Data Types and Structures
C has a limited set of built-in data types, while C++ expands this with classes and structures. For instance, C structures are primarily used to group data, whereas classes in C++ can encapsulate both data and functions, enhancing code organization.
Functions and Overloading
C does not support function overloading, meaning the same function name can only be used once. C++ allows multiple functions with the same name as long as they accept different parameters, making the code cleaner and easier to read.
Example in C++ using function overloading:
#include <iostream>
using namespace std;
class Overload {
public:
void show(int num) {
cout << "Integer: " << num << endl;
}
void show(double num) {
cout << "Double: " << num << endl;
}
};
int main() {
Overload obj;
obj.show(5); // Calls show(int)
obj.show(5.5); // Calls show(double)
return 0;
}
Memory Management
Dynamic Memory Allocation
C uses `malloc` and `free` for memory management, which can lead to memory leaks if not handled correctly. In C++, you have `new` and `delete`, which not only allocate memory but also construct and destruct objects.
Example in C:
#include <stdlib.h>
int main() {
int *arr = (int *)malloc(5 * sizeof(int));
// Use the array
free(arr);
return 0;
}
Example in C++:
#include <iostream>
using namespace std;
int main() {
int *arr = new int[5];
// Use the array
delete[] arr; // Automatically calls destructor for object
return 0;
}
Standard Libraries
Library Differences
The C standard library provides basic functionality, while C++ offers the Standard Template Library (STL) that comes with advanced data structures like vectors, lists, and algorithms.
For instance, using STL in C++ to manage a collection of integers:
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> vec = {1, 2, 3, 4, 5};
for (int num : vec) {
cout << num << " ";
}
return 0;
}
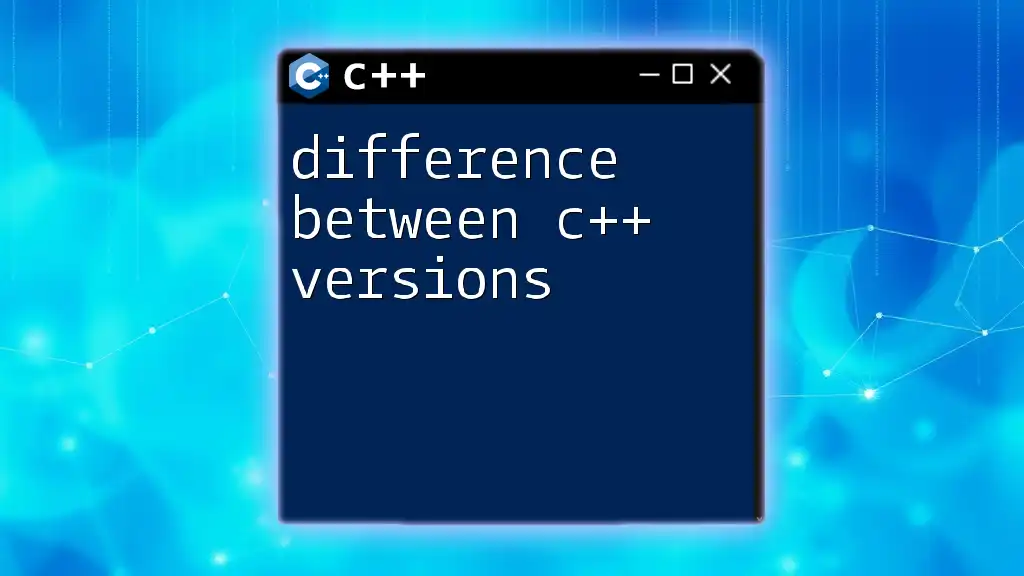
Performance Considerations
C vs C++ in Execution Speed
In general, C programs are faster due to their low-level control and minimal abstraction. However, C++ can achieve similar performance levels because of optimizations in compilers. The choice between them often comes down to the specific needs of the application, such as whether the overhead of OOP in C++ is justifiable in a performance-critical project.
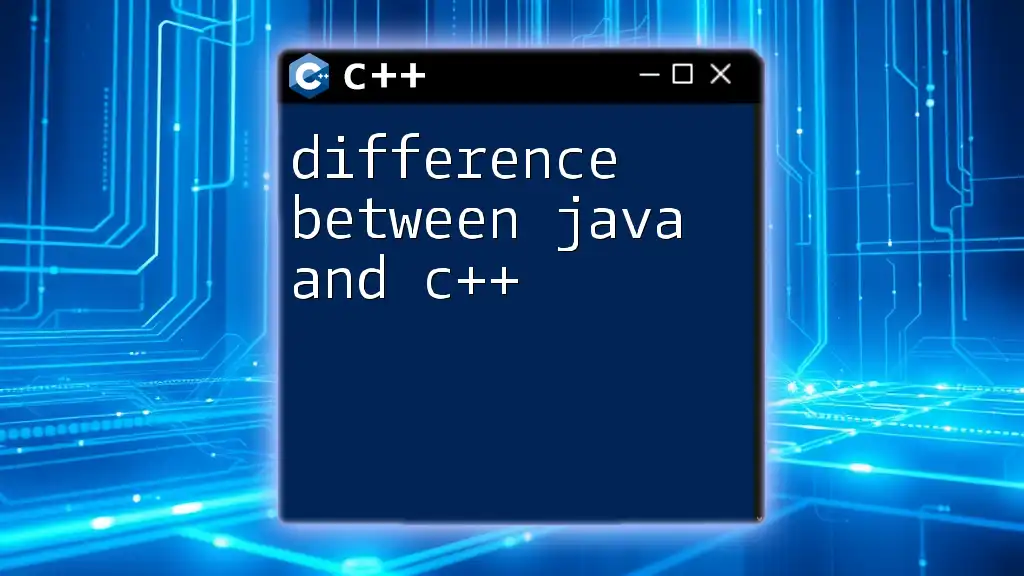
Error Handling
Error Handling Techniques
C employs traditional error handling through return codes, which can lead to code that's difficult to maintain. In contrast, C++ utilizes exception handling, enabling cleaner and more manageable error management.
An error handling example in C:
#include <stdio.h>
int divide(int a, int b) {
if (b == 0) {
return -1; // Indicate an error
}
return a / b;
}
int main() {
int result = divide(10, 0);
if (result == -1) {
printf("Error: Division by zero\n");
}
return 0;
}
An error handling example in C++ using exceptions:
#include <iostream>
using namespace std;
double divide(int a, int b) {
if (b == 0) {
throw runtime_error("Division by zero");
}
return static_cast<double>(a) / b;
}
int main() {
try {
cout << divide(10, 0) << endl;
} catch (const runtime_error &e) {
cout << "Error: " << e.what() << endl;
}
return 0;
}
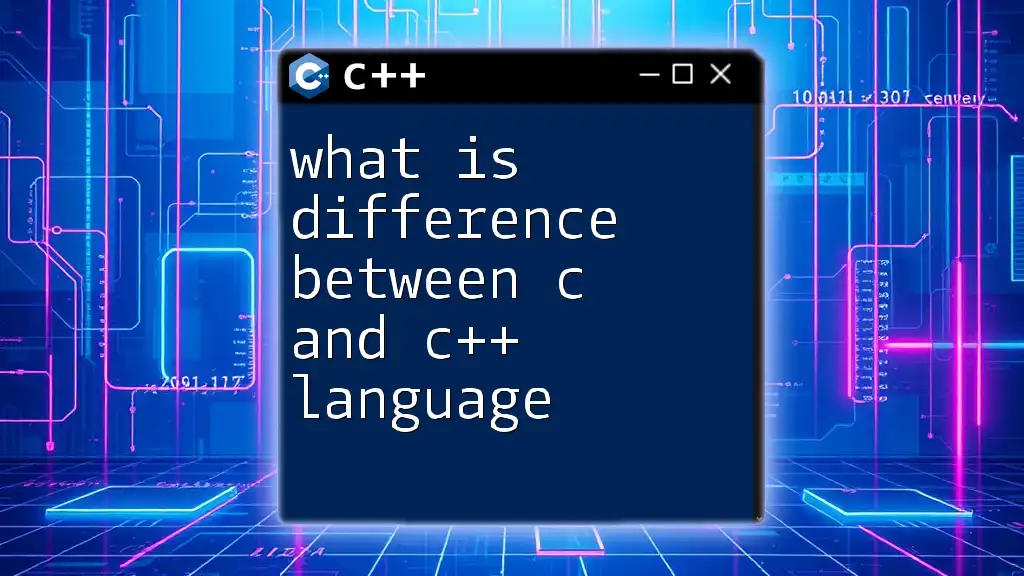
Code File Extensions and Compiling
.c vs .cpp
File naming conventions are crucial in distinguishing C from C++. C source code files typically use the `.c` extension, while C++ files use `.cpp`. Most compilers treat these extensions differently, applying appropriate language standards during compilation.
For example, to compile a C file, you might use:
gcc -o output_file file.c
To compile a C++ file, you would use:
g++ -o output_file file.cpp
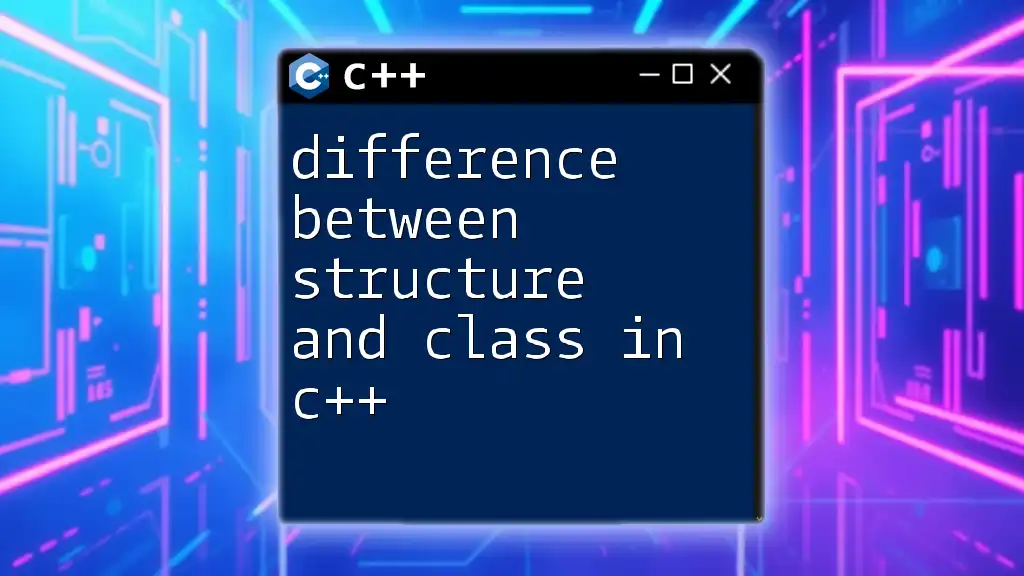
Conclusion
Understanding the difference between C and C++ is essential for any programmer. While C is ideal for low-level programming and system design, C++ offers powerful features that facilitate complex software development through object-oriented programming. Ultimately, selecting the right language depends on the project requirements, performance needs, and personal preferences.
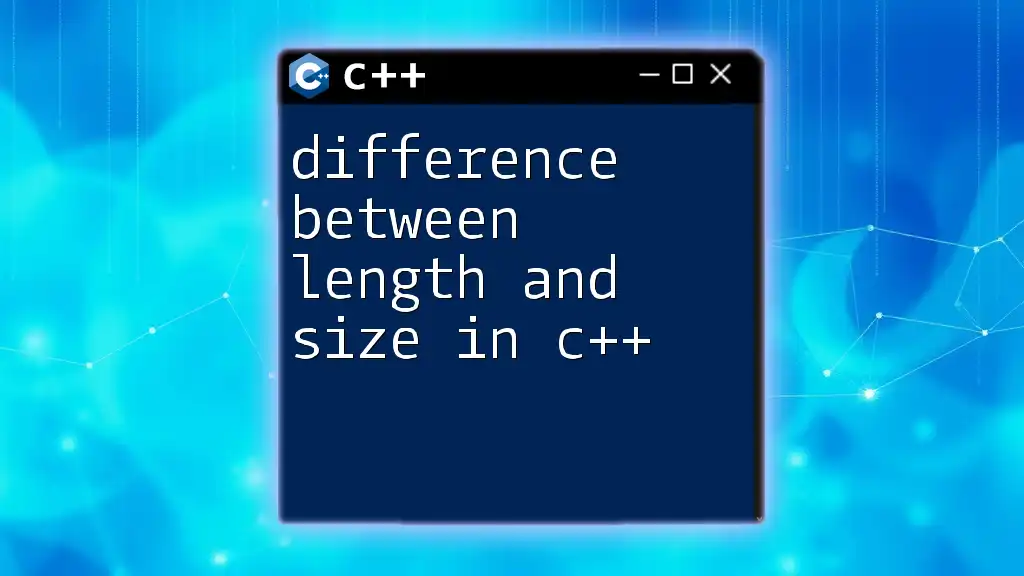
Frequently Asked Questions (FAQs)
What is the difference between C and C++ programming language?
The primary difference lies in C being a procedural language while C++ supports object-oriented programming, thus offering a richer set of features and libraries.
Why choose C over C++?
C is often preferred in situations where low-level hardware manipulation and system resource efficiency are necessary, such as in embedded systems.
Is C++ backward compatible with C?
C++ maintains a level of compatibility with C, allowing most C programs to compile in a C++ environment, but some discrepancies do exist due to language evolution.
Can you mix C and C++ code?
It's possible to mix C and C++ code; however, doing so requires careful management of compilation and linking processes to resolve any compatibility issues. Best practices involve isolating the C code into separate files and calling it from C++ when necessary.
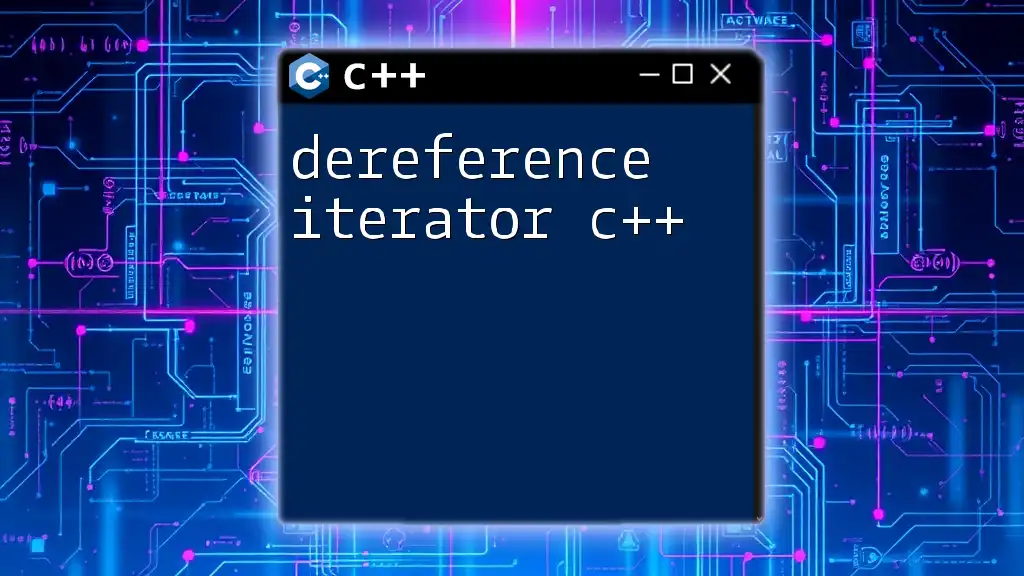
Additional Resources
For those looking to deepen their knowledge of the difference between C and C++, consider exploring the following resources:
- Recommended books like "The C Programming Language" by Brian W. Kernighan and Dennis M. Ritchie for C
- "The C++ Programming Language" by Bjarne Stroustrup for C++
- Online platforms such as Codecademy and Coursera that offer courses on C and C++ programming