C++ is a statically typed, compiled language that emphasizes performance and low-level memory manipulation, while Python is a dynamically typed, interpreted language that prioritizes readability and ease of use.
Here's a code snippet demonstrating a simple "Hello, World!" program in both languages:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
print("Hello, World!")
Understanding Python and C++
Definition of Python
Python is a high-level, interpreted programming language known for its simplicity and readability. It supports multiple programming paradigms, including procedural, object-oriented, and functional programming. Key features that contribute to its popularity include dynamic typing, extensive libraries, and an active community.
Definition of C++
C++ is a powerful, compiled programming language that builds on the foundation of the C language, adding features such as object-oriented programming. Known for its performance and efficiency, C++ allows developers to manage system resources closely, making it suitable for system-level programming. Essential features include static typing, a rich standard library, and low-level memory manipulation.
Why Compare Python and C++?
Both Python and C++ are widely used in various domains but cater to different needs. While Python is favored for rapid development, prototyping, and data science, C++ is the go-to choice in performance-critical applications such as game development, operating systems, and high-frequency trading.

Difference Between C++ and Python: Syntax
General Syntax
When considering the syntax, C++ can be seen as more complex compared to Python. In C++, every program must define a `main` function, and it requires semicolons at the end of each statement.
Here is how a simple Hello World program looks in C++:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!";
return 0;
}
In contrast, Python prioritizes simplicity and clarity. A Python program does not require a specific structure and eliminates unnecessary punctuation, leading to more straightforward syntax.
The equivalent Hello World program in Python is:
print("Hello, World!")
Code Readability
C++ code can become verbose, which might deter beginners. The need for curly braces, semicolons, and type declarations often complicates otherwise simple tasks. On the other hand, Python's syntax is designed to be intuitive, which enhances code readability. Python encourages best practices such as code indentation, which makes it visually clean and easier to debug.
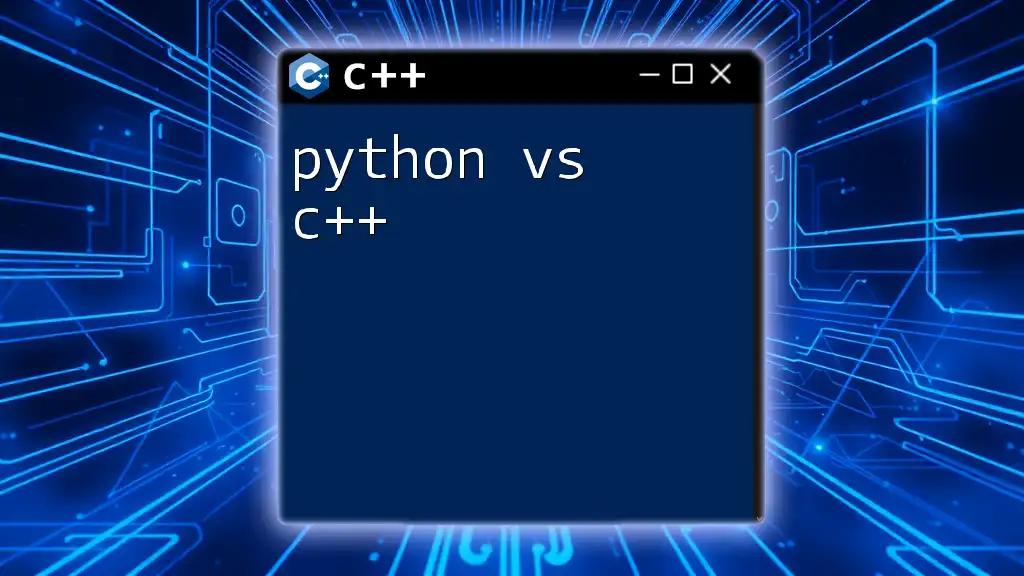
Programming Paradigms
C++ Paradigms
C++ is fundamentally object-oriented, which emphasizes code reuse through classes and objects. It supports procedural programming as well, allowing developers to write both structured and object-oriented code.
For instance, consider this simple example of a class in C++:
class Animal {
public:
void sound() {
cout << "Animal sound" << endl;
}
};
In this case, we define a class named `Animal` with a method `sound` that outputs a string.
Python Paradigms
Python is also an object-oriented language but adds dynamic and multiparadigm features. It supports procedural and functional programming, allowing developers to choose the best paradigm suited for their tasks.
Here’s how you might define a similar class in Python:
class Animal:
def sound(self):
print("Animal sound")
In Python, methods and data members are typically simpler to manage due to its dynamic nature.

Performance and Speed
Execution Speed
One of the crucial differences when discussing the diff between Python and C++ is execution speed. C++ is a compiled language, meaning that the code you write is transformed into machine code ahead of execution, which significantly improves performance. This compilation step allows C++ programs to run faster than those written in Python, which is interpreted line-by-line at runtime.
Use Cases Where Performance Matters
C++ excels in domains that require high performance such as game development, real-time systems, and applications that demand low-level manipulation of hardware resources. Conversely, Python shines in scenarios where development speed and ease of use are prioritized, such as in scripting, data analysis, and web development.

Memory Management
C++ Memory Management
C++ utilizes manual memory management, allowing developers to create and destroy objects as needed, which gives them fine-grained control over resources. However, this comes with the responsibility of handling memory leaks, where memory allocated for variables is not freed properly, leading to wasted resources. Here’s an example using pointers in C++:
int* p = new int(5);
delete p;
In this instance, an integer pointer `p` is created and assigned a value of 5, which is then manually deallocated.
Python Memory Management
In contrast, Python employs automatic garbage collection, where the Python interpreter takes care of memory management. This feature simplifies development, as programmers do not need to actively manage memory allocation and deallocation, reducing the risk of memory-related bugs.

Libraries and Frameworks
Availability and Usefulness
Both languages offer comprehensive libraries that enhance their capabilities. In C++, developers typically rely on the Standard Template Library (STL) for essential data structures and algorithms, while the Boost library adds significantly to the language's functionality.
On the other hand, Python boasts a plethora of libraries that make it particularly suitable for data manipulation and analysis. Libraries such as NumPy, Pandas, and Matplotlib have become industry standards, making it easy for developers to perform complex tasks with minimal code.

Community and Support
C++ Community
The C++ community is robust and consists of numerous forums, user groups, and vast documentation that facilitate learning. This support system is invaluable for developers seeking to navigate the complexities of the language effectively.
Python Community
Python enjoys a rapidly growing community which has been crucial to its rise in popularity. With open-source contributions and a wide range of tutorials and resources available, new learners can easily find support and opportunities to collaborate on projects.

Conclusion
In summary, while there are significant differences between C++ and Python—ranging from syntax and memory management to performance and paradigms—each language has its unique strengths and applications. C++ is ideal for situations requiring high performance and low-level control, while Python is well-suited for rapid development and ease of use.
Choosing the Right Language for Your Needs
Understanding the strengths of both C++ and Python will empower you to choose the right language for your specific projects. Embrace both languages for a well-rounded skill set and unlock your potential as a programmer.

Call to Action
Explore our classes to learn about C++ commands in a quick, concise manner! Share your thoughts and experiences with Python and C++ in the comments section below and join the community of learners.