Embedding Python in C++ allows developers to utilize Python scripts within C++ applications by integrating the Python C API for seamless interaction between the two languages. Here's a simple example:
#include <Python.h>
int main() {
Py_Initialize(); // Initialize the Python Interpreter
PyRun_SimpleString("print('Hello from Python!')"); // Run a simple Python command
Py_Finalize(); // Clean up and close the Python Interpreter
return 0;
}
Understanding Python's C API
What is the Python C API?
The Python C API serves as a bridge that allows C and C++ programs to interact with Python code. It provides the necessary functions to manipulate Python objects and call Python functions directly from C++. By leveraging this API, developers can harness the power of Python libraries for scripting and high-level programming while maintaining the performance benefits of C++.
Key components of the C API include:
- PyObject: The fundamental building block for all Python objects. Every object in Python is represented as a PyObject in C.
- Reference Counting: A method used for memory management, whereby the number of references to an object is tracked. When the reference count reaches zero, the object is deallocated.
- Initialization: Requires initializing the Python interpreter in your C++ application to manage Python-related functionalities properly.
Installing the Python Development Kit
To begin embedding Python in C++, you must have the Python development headers installed. This is essential for compiling C++ code that interacts with Python.
The installation varies based on your operating system:
- For Linux, install the package using:
sudo apt-get install python3-dev
- For macOS, you would typically install Python through Homebrew:
brew install python
Once installed, you can check the installed version using:
python3 --version
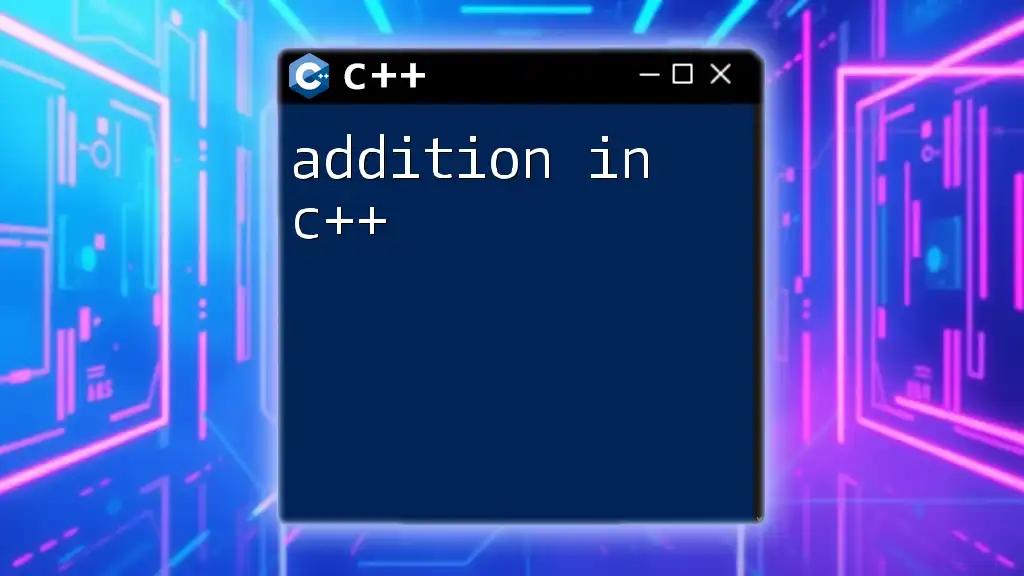
Setting Up Your C++ Project
Environment Configuration
Proper configuration of your development environment is crucial for successful integration. You need to set the include directories for the Python headers and link against the Python libraries.
Sample Makefile Configuration
A simple Makefile example to illustrate how to compile a C++ file that embeds Python:
CXX = g++
CXXFLAGS = -I/usr/include/python3.x
LDFLAGS = -lpython3.x
all: your_program
your_program: your_program.cpp
$(CXX) -o your_program your_program.cpp $(LDFLAGS)
This configuration specifies the C++ compiler, includes the necessary headers, and links the relevant Python library, allowing seamless usage of the Python API.

Basic Example: Running a Python Script from C++
Sample Python Script
Before embedding Python in C++, prepare a simple Python script. Save the following code as `hello.py`:
# hello.py
def greet(name):
return f"Hello, {name}!"
C++ Code to Embed Python
With the Python script ready, you can create a C++ program to execute it. Here’s a straightforward implementation:
#include <Python.h>
#include <iostream>
int main() {
Py_Initialize();
PyObject *pName = PyUnicode_DecodeFSDefault("hello");
PyObject *pModule = PyImport_Import(pName);
Py_DECREF(pName);
if (pModule != nullptr) {
PyObject *pFunc = PyObject_GetAttrString(pModule, "greet");
if (pFunc && PyCallable_Check(pFunc)) {
PyObject *pArgs = PyTuple_Pack(1, PyUnicode_FromString("World"));
PyObject *pValue = PyObject_CallObject(pFunc, pArgs);
Py_DECREF(pArgs);
if (pValue != nullptr) {
std::cout << PyUnicode_AsUTF8(pValue) << std::endl;
Py_DECREF(pValue);
} else {
PyErr_Print();
std::cerr << "Call failed" << std::endl;
}
Py_DECREF(pFunc);
}
Py_DECREF(pModule);
} else {
PyErr_Print();
std::cerr << "Failed to load \"hello\"" << std::endl;
}
Py_Finalize();
return 0;
}
In this code, we initialize the Python interpreter and import the `hello` module. We then retrieve the `greet` function, call it with an argument, and print the result. Finally, we clean up our references and finalize the Python interpreter.

Managing Data Between C++ and Python
Converting Data Types
One essential aspect of embedding Python in C++ is understanding how to convert data between the two languages. Here are some common conversions:
- Integer: Use `PyLong_FromLong()` to convert C++ integers to Python integers.
- Float: Use `PyFloat_FromDouble()` for float-to-Python conversions.
- String: You can convert C++ strings using `PyUnicode_FromString()`.
Understanding how to manage these data types will significantly improve the interoperation between your Python and C++ code.
Example: Passing a List from C++ to Python
Here’s how to create a Python list from C++:
PyObject *pList = PyList_New(3);
PyList_SetItem(pList, 0, PyLong_FromLong(10));
PyList_SetItem(pList, 1, PyLong_FromLong(20));
PyList_SetItem(pList, 2, PyLong_FromLong(30));
In this example, we create a new Python list with three elements. After invoking `PyList_New()`, we use `PyList_SetItem()` to add integer values to the list. Remember that `PyList_SetItem` takes ownership of the reference, so you don’t need to decrease the reference count for the added items.
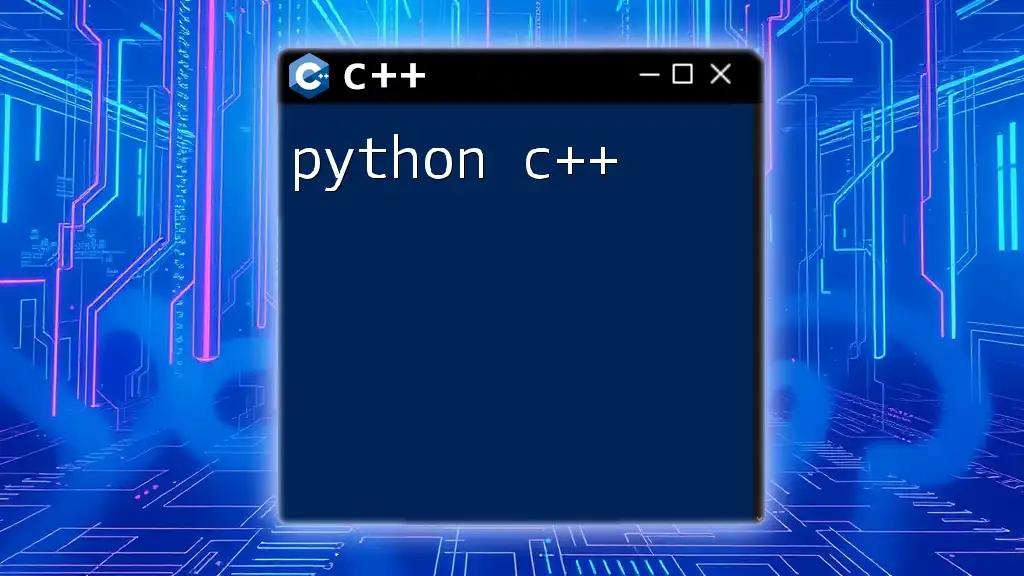
Handling Exceptions and Errors
Python Error Handling in C++
Error handling is critical when embedding Python in C++. Use `PyErr_Occurred()` to check if an error occurred during Python function calls.
Example of Error Handling
Here’s an example of how to handle errors gracefully:
if (PyErr_Occurred()) {
PyErr_Print();
std::cerr << "Error occurred while calling Python function" << std::endl;
}
In this snippet, if an error is detected, it is printed, and you can examine the error message outputted to help debug any issues that arise in the Python code.

Integrating Python Libraries with C++
Using Popular Libraries (NumPy, Pandas)
Integrating libraries such as NumPy and Pandas can enhance your application greatly. These libraries offer extensive functionality for mathematical operations, data manipulation, and analysis, all of which can be crucial in high-performance applications.
The process typically involves importing the library within your Python code and managing the interactions from C++.
Code Snippet: Using NumPy in C++
To use NumPy, first, ensure you have NumPy installed in Python:
pip install numpy
Then, you can call Python functions and manipulate NumPy arrays from C++ just like you did with plain Python functions.
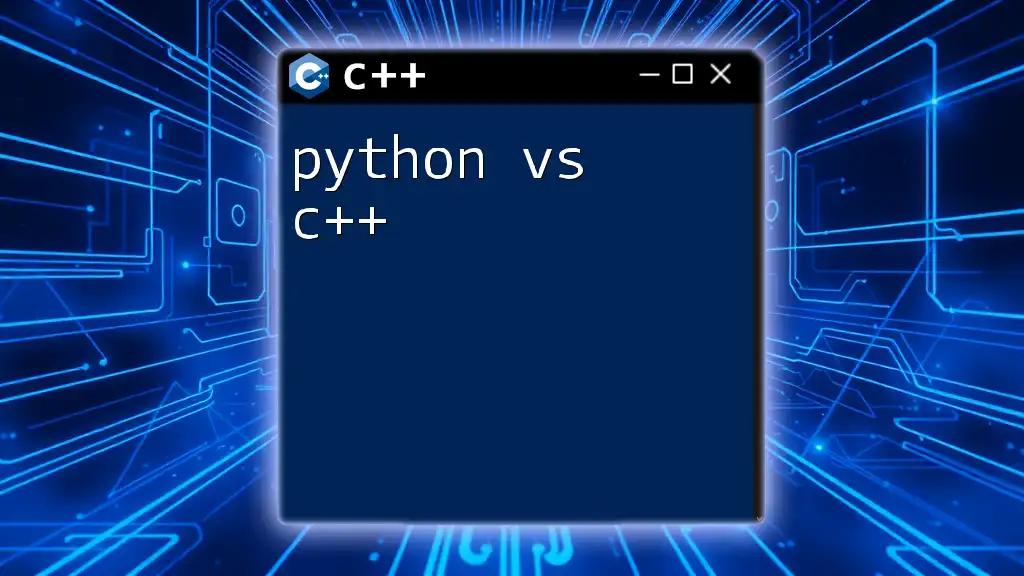
Best Practices for Embedding Python in C++
Performance Considerations
Managing the Global Interpreter Lock (GIL) is important when embedding Python in multi-threaded applications. The GIL allows only one thread to execute Python bytecode at a time, which can be a bottleneck for CPU-bound tasks. Use `PyGILState_Ensure()` and `PyGILState_Release()` to manage the GIL correctly.
Code Maintenance Tips
Separation of concerns is essential for maintaining clarity in your codebase. Keep your C++ and Python code organized by creating separate files for each language, which will help in regulating the functionality of each part of your program effectively.
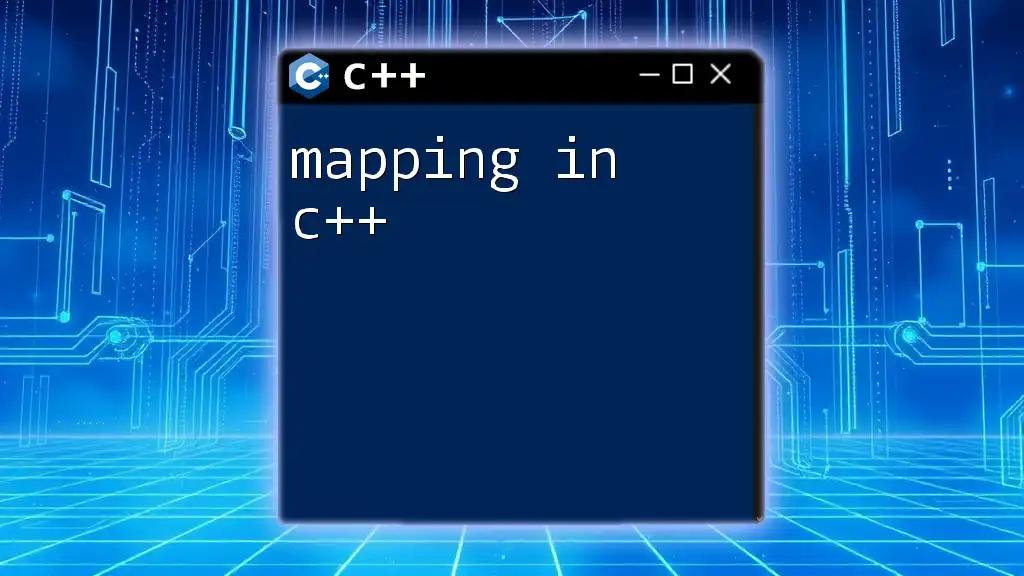
Conclusion
By embedding Python in C++, you unlock the opportunity to combine high-level scripting with performance-critical applications. This integration allows developers to write more maintainable and flexible code while benefiting from Python's vast ecosystem of libraries.
As you delve deeper into this integration, remember to consult the official Python documentation for updates and best practices. With proper understanding and utilization of the Python C API, you can create powerful applications that leverage the strengths of both programming languages.
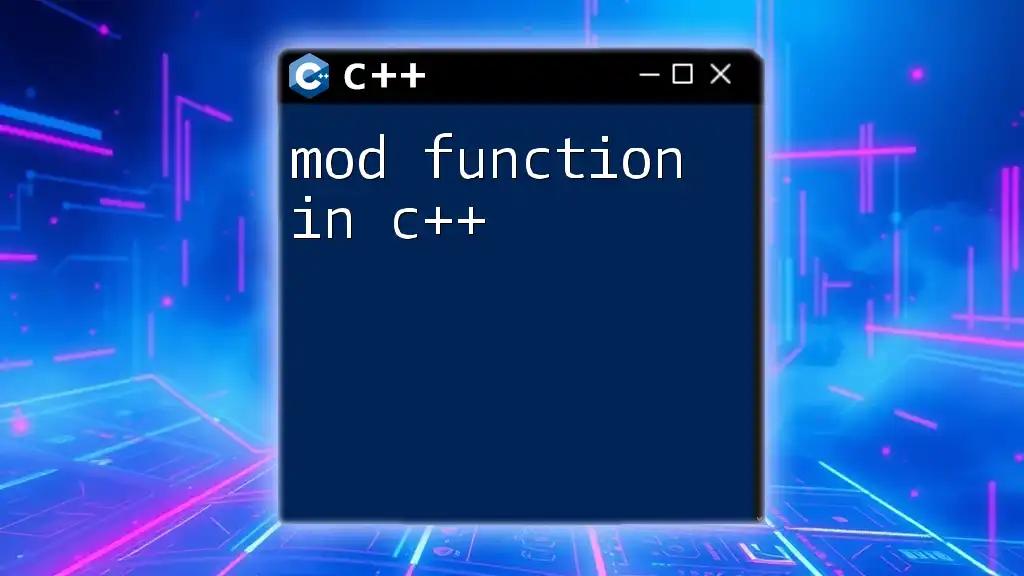
FAQs
Common Questions About Python and C++ Integration
What are the main benefits of embedding Python into C++?
Embedding Python allows for using high-level features and libraries from Python while keeping the performance advantages of C++.
Can I run Python scripts without installing Python?
No, Python must be installed on the system to embed it within a C++ application, as the Python interpreter is required for execution.
By integrating Python within C++, you greatly enhance your programming capabilities and leverage the strengths of both languages effectively. As you embark on this journey, continue to explore the intricacies of the Python C API to maximize your development potential.