In C++, comments are used to provide explanations or annotations within the code and are ignored by the compiler, with single-line comments using `//` and multi-line comments enclosed in `/* ... */`.
Here’s a code snippet demonstrating both types of comments:
#include <iostream>
int main() {
// This is a single-line comment
std::cout << "Hello, World!" << std::endl; // Prints a message
/* This is a multi-line comment
that spans multiple lines */
std::cout << "Learning C++ comments!" << std::endl;
return 0;
}
Types of Comments in C++
Single-line Comments
Single-line comments in C++ are denoted using `//`. This allows you to write short comments that are intended for immediate context or explanation without extending beyond the current line.
Example:
// This is a single-line comment
int x = 5; // Initialize x with 5
Using single-line comments is very effective when you want to clarify the purpose of variables or provide brief notes on specific code operations. They should be utilized for quick notes that aid readability, ensuring that anyone reading the code can easily grasp your intentions.
Multi-line Comments
Multi-line comments enable you to annotate larger sections of code, using the syntax `/* comment */`. This type of comment is useful when you need to write down more extensive explanations or multiple lines of descriptions.
Example:
/* This is a multi-line comment
that spans multiple lines */
int y = 10;
Employing multi-line comments can be beneficial for providing detailed documentation or comprehensive notes on algorithms, functions, or complex logic within your code. It’s an excellent way to enhance the understanding of significant code blocks, especially for intricate processes.
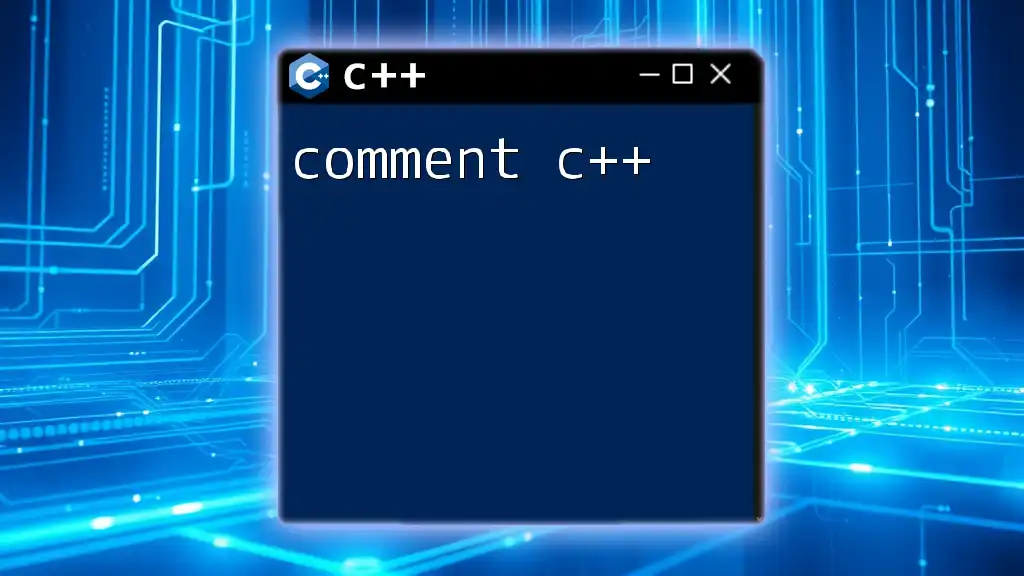
Best Practices for Commenting in C++
Be Clear and Concise
Clarity is key when it comes to comments in C++. A well-written comment can provide direct guidance, while vague or overly complex comments might lead to confusion. Ensure your comments are brief yet informative.
Examples of good vs. bad comments:
- Good: `// Incrementing the count by one`
This comment is clear and indicates precisely what the code does. - Bad: `// Change the variable`
This comment lacks specificity and doesn’t provide meaningful context.
While it’s essential to communicate effectively through comments, strive to present information that adds value to the code without bloating it.
Avoid Obvious Comments
When writing comments, strive to prevent redundancy by avoiding comments that merely restate what the code does. Comments that explain what is apparent should be omitted.
Example:
int z = 0; // Set z to zero (Avoid this)
In this case, the comment adds no real value, as any developer seeing `int z = 0;` would inherently understand its purpose.
Use Comments for Documentation
Comments can be utilized effectively for documenting the behavior or design of functions and complex algorithms. This practice ensures that anyone reviewing the code can quickly understand its purpose and usage.
Example:
/*
* Function: calculateFactorial
* ------------------------------
* Calculates the factorial of a given number n.
* n: The number to calculate the factorial for.
*
* returns: The factorial of n.
*/
int calculateFactorial(int n) {
// Function implementation...
}
In this example, the multi-line comment provides crucial information about the function's intent, parameters, and return value. This is particularly advantageous when your code will be reviewed by others or when revisiting older code yourself.
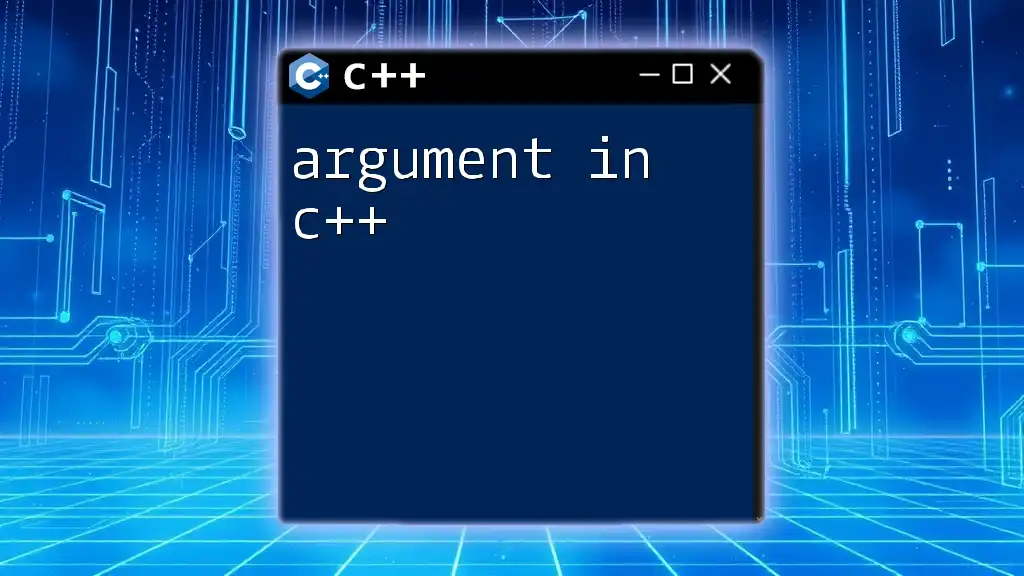
Commenting Styles in C++
Inline Comments
Inline comments are placed on the same line as the code they describe. They offer immediate context without polluting the surrounding code with extra space or lines.
Example:
int main() {
int a = 10; // Initialize variable a
}
Inline comments can enhance readability when used judiciously, but they should be kept short to maintain the flow of the code.
Block Comments
Block comments can be used to annotate multiple lines or a section of code collectively. They are useful for explaining elaborate logic or providing context for large chunks of code.
Example:
/* Initialize variables
before the main calculations */
int b = 5, c = 15;
When using block comments, be careful not to obscure the logic of the code to the reader. Clear delineation of information can enhance understanding significantly.
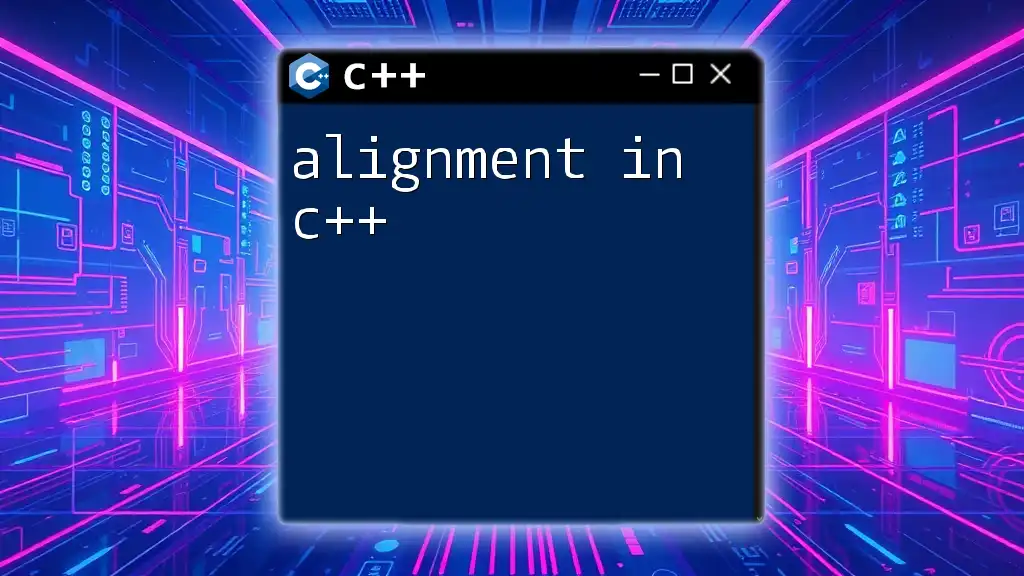
Common Mistakes to Avoid When Commenting in C++
Over-commenting vs. Under-commenting
Finding the right balance in your commenting practice can be tricky. Over-commenting can clutter your code and detract from clarity, while under-commenting can leave readers confused about your logic. Aim for clarity without unnecessary verbosity.
Outdated Comments
Outdated or incorrect comments can mislead readers and create confusion. It’s essential to maintain your comments as your code evolves. When you change functionality, ensure your comments reflect those updates.
Example of outdated comments:
// The variable now stores the user's age (but it doesn't)
In this case, the comment can lead to misunderstandings and should be revised or removed to avoid confusion.
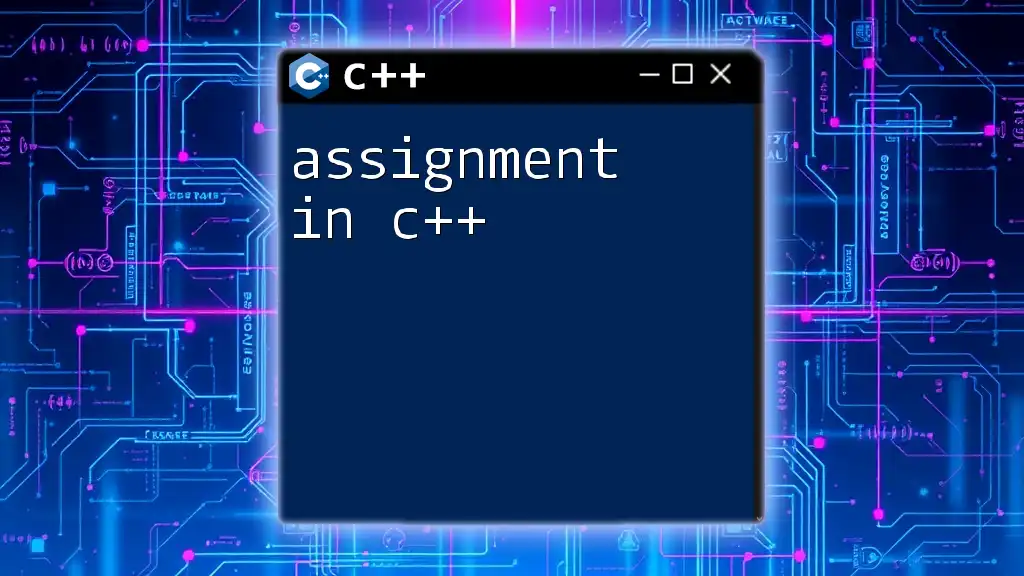
Tools and Practices to Enhance Commenting in C++
Using IDE Features
Modern Integrated Development Environments (IDEs) often come equipped with features that assist in commenting effectively. For example, many allow auto-generating documentation from comments, making it easier to maintain consistent documentation practices.
Code Review and Commenting
Comments play a significant role in code reviews, facilitating constructive feedback and discussions. Leveraging comments for providing or requesting feedback improves collaborative efforts and enhances code quality. Embrace the opportunity to make observations and inquiries within code comments for a smoother review process.
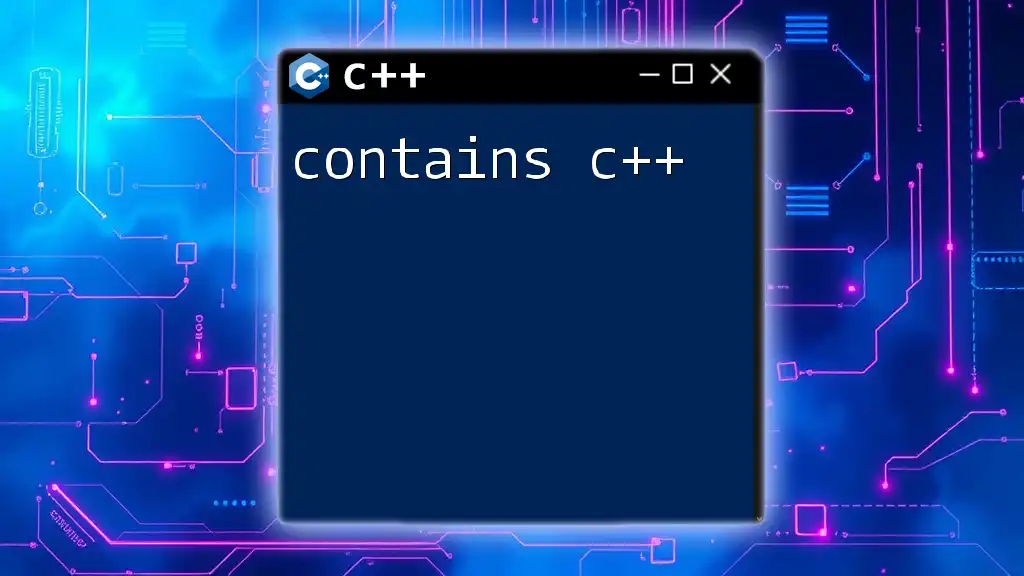
Conclusion on Commenting in C++
Effective commenting in C++ significantly improves code clarity, maintainability, and collaboration. By adhering to best practices and avoiding common pitfalls, you can foster an environment where code is not only written but understood. Embrace the art of commenting as a fundamental skill in your programming toolkit.
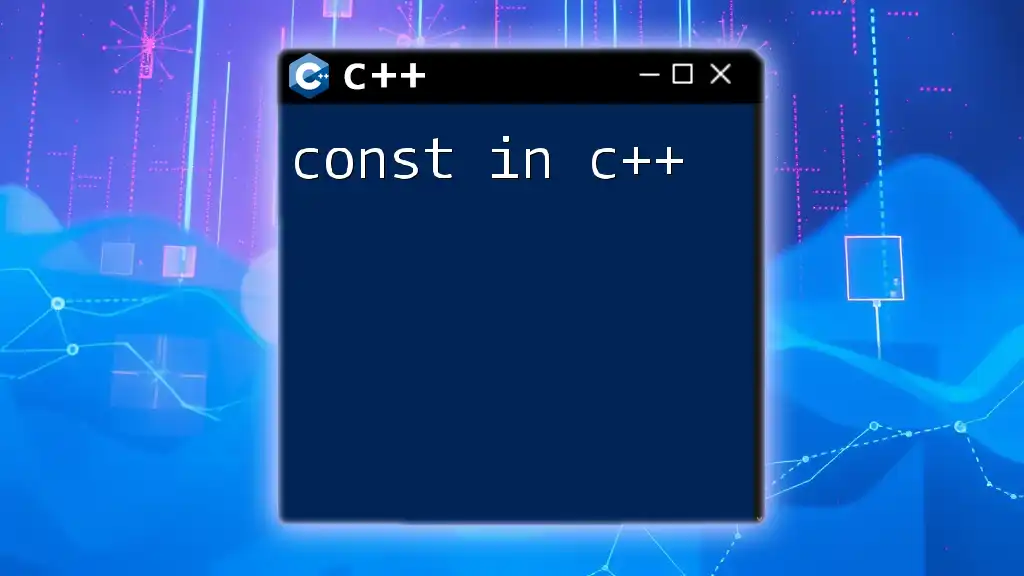
Additional Resources
- Explore the official C++ documentation for detailed explanations of syntax and functions.
- Check out recommended books on C++ programming to deepen your understanding of coding practices and methodologies related to comments and beyond.