Formatting C++ refers to the practice of organizing and structuring your code for better readability and maintainability, which includes consistent indentation, spacing, and the use of meaningful naming conventions.
Here's a simple code snippet that demonstrates proper formatting in C++:
#include <iostream>
int main() {
int number = 10;
if (number % 2 == 0) {
std::cout << "The number is even." << std::endl;
} else {
std::cout << "The number is odd." << std::endl;
}
return 0;
}
Understanding C++ Formatting
What is C++ Formatting?
C++ formatting refers to the conventions and methods used to structure code in a clear and consistent way. Proper formatting is crucial for any programming language, but it is particularly significant in C++, given its complexity and the flexibility it offers developers. Well-formatted code enhances readability, maintainability, and collaboration among programmers.
Why Is Formatting Important?
The importance of formatting cannot be overstated. Here are a few reasons why effective formatting is vital:
- Enhances maintainability: Well-structured code is easier to read and update. This is especially useful when multiple developers are involved in a project or when returning to code after a long time.
- Makes collaboration easier: When all team members adhere to a consistent formatting style, it reduces the friction and confusion that can arise from varying coding conventions.
- Reduces errors in code: Properly formatted code often helps in spotting logical errors and syntactic issues, making debugging a more straightforward process.
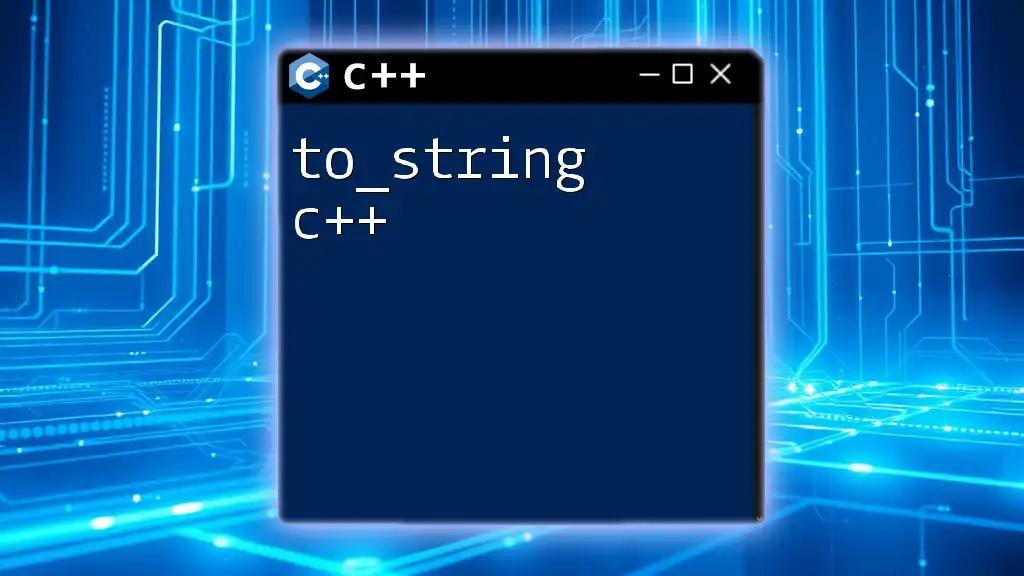
Basic Principles of C++ Formatting
Consistent Indentation
Indentation in C++ serves as a visual cue that indicates the structure and flow of the code. Inconsistent indentations can mislead a programmer regarding the scope of code blocks. Here are examples of good and poor indentation:
// Good Indentation
if (x > 0) {
cout << "Positive";
} else {
cout << "Non-Positive";
}
// Poor Indentation
if (x > 0){
cout << "Positive";
}else{cout << "Non-Positive";}
In the first example, it's easy to identify the scope of the if-else statement, while in the second example, the lack of indentation obscures the control flow.
Whitespace Usage
Strategic use of whitespace can greatly enhance the readability of your code.
- Leading and trailing spaces: Be mindful about unnecessary spaces, as they can lead to ignored errors or confusing output.
- New lines: Use new lines generously to separate logical blocks of code; this gives the reader a moment to digest what has just been read.
Line Length
While coding in C++, it is widely recommended to limit line length to 80-120 characters. This practice is supported by the majority of C++ coding standards, as it prevents horizontal scrolling and encourages better organization of code.
If line length becomes problematic, consider the following method to handle long statements:
cout << "This is a very long statement that should be broken up for better readability"
<< " instead of putting everything on one line.";
Braces and Block Formatting
C++ allows for different styles of brace placement, leading to various opinions on the most effective practices.
- Egyptian style places the opening brace on the same line as the control structure, while K&R style has the opening brace on the same line for if-else and loops but is placed on the next line in function definitions.
- K&R Style Example
if (condition) { // Do something }
- Egyptian Style Example
if (condition) { // Do something }
Understanding both styles can help you adapt to team conventions and maintain a unified codebase.
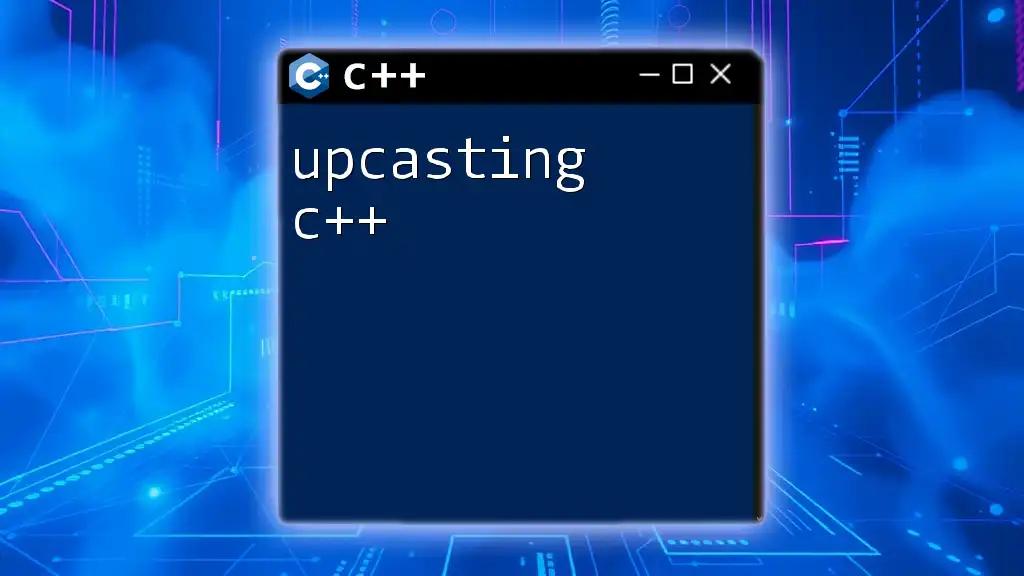
Advanced Formatting Techniques
Variable Naming Conventions
Choosing appropriate variable names is essential for clarity in your code. Different naming conventions can be applied, including CamelCase and snake_case.
For example:
- Using `int totalSum;` is CamelCase.
- Using `float total_sum;` is snake_case.
Clear variable names help communicate the variable's purpose, eliminating ambiguity and enhancing readability.
Commenting Code Effectively
Effective commenting is a cornerstone of good programming practices. Comments should not clutter the code but should provide clarity where necessary. There are two types of comments in C++:
-
Single-line comments
// This is a single-line comment
-
Multi-line comments
/* This is a multi-line comment that explains the following code block */
Properly placed comments can guide readers through complex logic, making it easier to understand quickly what you intend to achieve.
Code Organization
Proper organization of your C++ files and folders is essential, especially in larger projects. By separating functionality into header files and source files, your code becomes more modular and easier to manage. Header files contain declarations, while source files contain definitions and implementations.
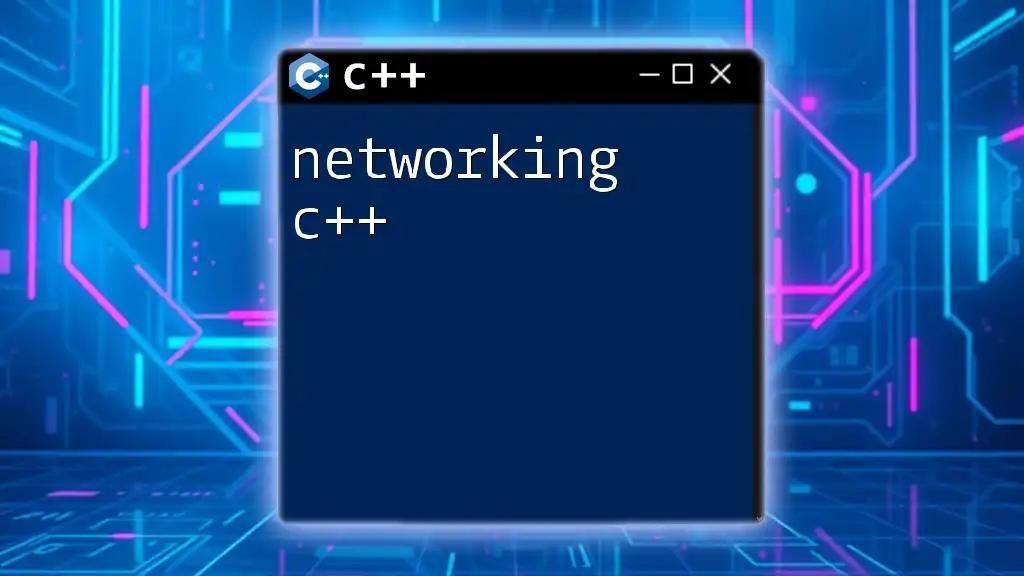
C++ Code Formatting Tools
Integrated Development Environments (IDEs)
Modern IDEs like Visual Studio and CLion come equipped with features that aid in formatting C++ code automatically. Many offer customizable settings to enforce specific coding standards, saving time and ensuring consistency across projects.
Code Formatters
Tools such as `clang-format` and `Astyle` are excellent for ensuring that your code adheres to predetermined formatting styles. These tools can transform your code into your intended format instantly. Consider the following command to format a file with `clang-format`:
# Example of using clang-format
clang-format -i my_code.cpp
Linters and Static Analysis Tools
Linters are invaluable in maintaining code quality by spotting issues related to formatting, style, and potential errors. For instance, `cpplint` is a popular tool for checking formatting issues against the Google C++ Style Guide. Utilizing these tools can automate the enforcement of your code formatting standards.
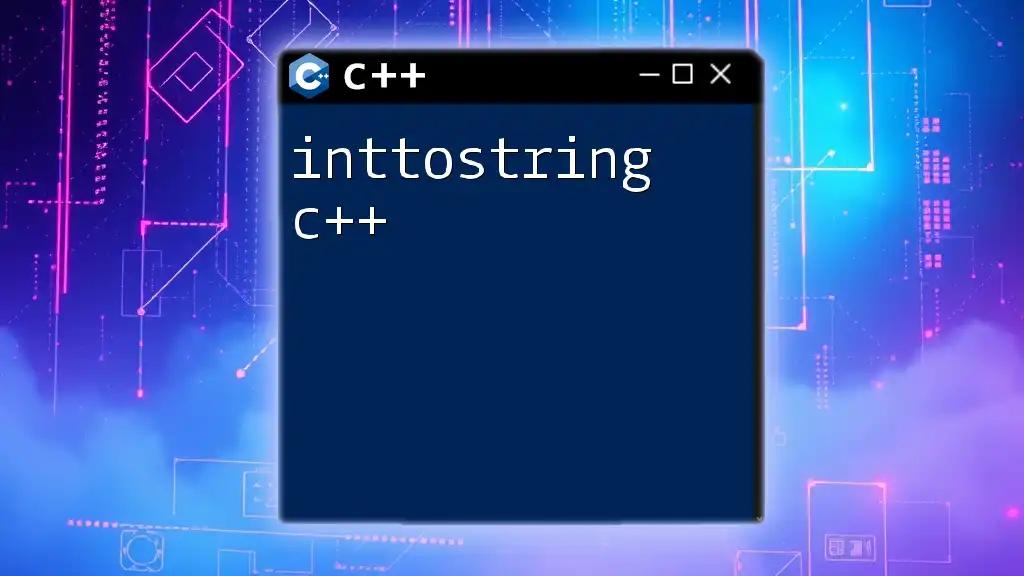
Best Practices for C++ Formatting
Seasoned Developer Tips
Learning from the experiences of seasoned developers can be instrumental. Some common advice includes using consistent formatting across all projects, regularly reviewing and refactoring code, and creating a shared style guide for team projects.
Continuous Learning and Adaptation
C++ is an evolving language with frequent updates and changes in best practices. Staying current on these trends is essential. Engaging in the C++ community through forums, attending programming conferences, and utilizing educational resources will promote a culture of continuous learning.
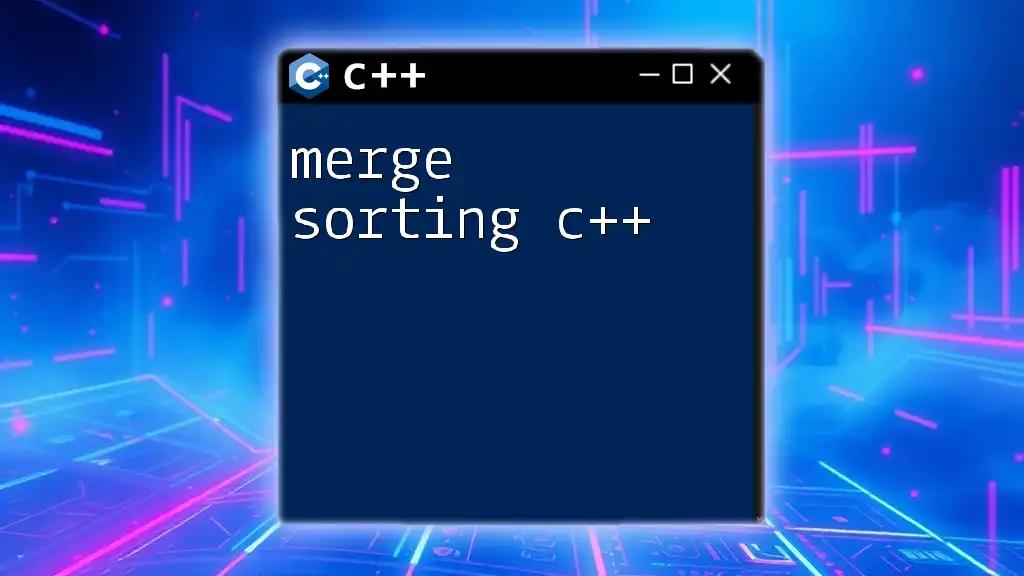
Conclusion
Practicing effective C++ formatting leads to improved code readability, maintainability, and overall programming experience. Incorporating the discussed guidelines not only enhances your own coding style but also fosters collaboration among your peers.
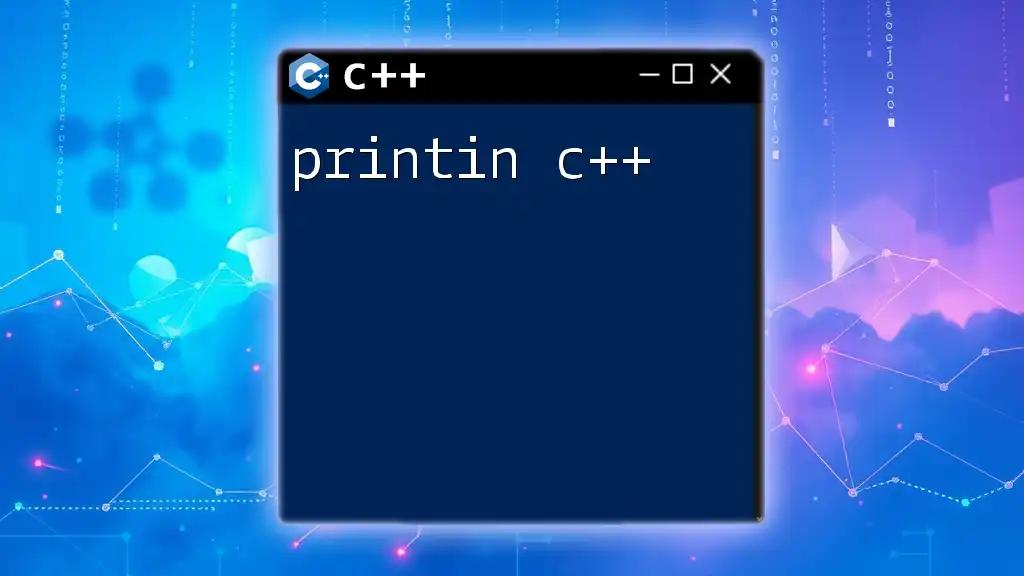
Call to Action
Don’t hesitate to implement these formatting techniques into your projects and subscribe for more tips on mastering C++. Each step toward better formatting is a step toward better programming.