Merge sorting in C++ is a divide-and-conquer algorithm that recursively splits an array into two halves, sorts each half, and then merges them back together in order.
Here’s a simple implementation of merge sort in C++:
#include <iostream>
#include <vector>
void merge(std::vector<int>& arr, int left, int mid, int right) {
int n1 = mid - left + 1, n2 = right - mid;
std::vector<int> L(n1), R(n2);
for (int i = 0; i < n1; i++) L[i] = arr[left + i];
for (int j = 0; j < n2; j++) R[j] = arr[mid + 1 + j];
int i = 0, j = 0, k = left;
while (i < n1 && j < n2) {
if (L[i] <= R[j]) arr[k++] = L[i++];
else arr[k++] = R[j++];
}
while (i < n1) arr[k++] = L[i++];
while (j < n2) arr[k++] = R[j++];
}
void mergeSort(std::vector<int>& arr, int left, int right) {
if (left < right) {
int mid = left + (right - left) / 2;
mergeSort(arr, left, mid);
mergeSort(arr, mid + 1, right);
merge(arr, left, mid, right);
}
}
int main() {
std::vector<int> arr = {38, 27, 43, 3, 9, 82, 10};
mergeSort(arr, 0, arr.size() - 1);
for (int i : arr) std::cout << i << " ";
return 0;
}
Merge Sorting Overview
What is Merge Sort?
Merge sort is a classic divide and conquer algorithm that efficiently sorts data by recursively dividing the array into two halves, sorting them, and finally merging the sorted halves. It was invented by John von Neumann in 1945 and remains a fundamental algorithm in computer science due to its predictable performance and stability.
Understanding merge sort is essential, especially when dealing with large datasets or when stability during sorting is a crucial requirement—where elements of the same value maintain their original relative order.
How Merge Sort Works
The merge sort algorithm follows a straightforward process:
- Divide: Split the unsorted array into two halves.
- Conquer: Recursively sort both halves.
- Combine: Merge the sorted halves back together.
This recursive strategy efficiently narrows down the problem size and ensures that each element is compared and positioned correctly.
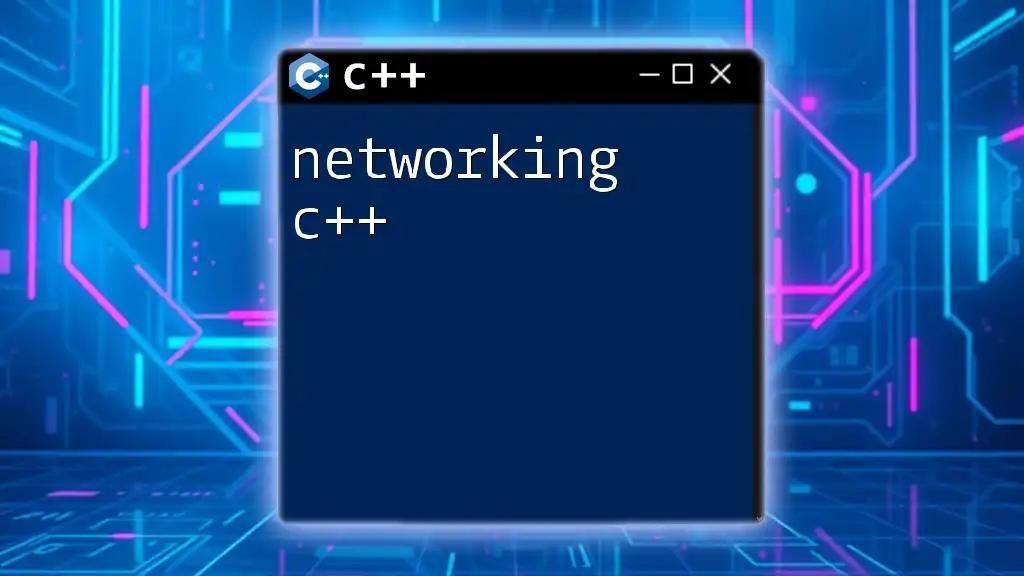
Characteristics of Merge Sort
Time Complexity
One of the standout features of merge sort is its time complexity, which is O(n log n), making it significantly faster than simpler algorithms like bubble sort or insertion sort, especially for large datasets. The O(n log n) arises from the fact that the array is repeatedly divided in half (creating a logarithmic factor) and each element must be compared (linear factor).
Space Complexity
Merge sort requires additional space, leading to a space complexity of O(n). This space is necessary to hold the temporary subarrays during the merging process. This characteristic may be a consideration when implementing merge sort in memory-constrained environments.

Implementing Merge Sort in C++
Setting Up the Environment
Before delving into the implementation, you need a suitable development environment. Popular IDEs like Visual Studio, Code::Blocks, or even GCC on the command line will serve well. Ensure that your C++ compiler supports features like the Standard Template Library (STL) to simplify implementations.
Basic Structure of Merge Sort
Pseudocode for Merge Sort
The pseudocode for merge sort encapsulates the core elements of the algorithm:
mergeSort(arr, left, right):
if left < right:
mid = (left + right) / 2
mergeSort(arr, left, mid)
mergeSort(arr, mid + 1, right)
merge(arr, left, mid, right)
C++ Code Implementation
The following code demonstrates how to implement merge sort in C++ using the standard library for dynamic array management.
#include <iostream>
#include <vector>
using namespace std;
// Function to merge two halves
void merge(vector<int>& arr, int left, int mid, int right) {
// Implementation of the merge function
}
// Merge Sort function
void mergeSort(vector<int>& arr, int left, int right) {
// Implementation of the merge sort function
}
This structure provides a clear foundation for developing the algorithm, and each code section can be expanded later.
Step-by-Step Implementation
The Merge Function
The merge function is vital as it combines two sorted halves into a sorted whole. Here’s a simple implementation:
void merge(vector<int>& arr, int left, int mid, int right) {
int n1 = mid - left + 1;
int n2 = right - mid;
vector<int> L(n1), R(n2);
for (int i = 0; i < n1; i++)
L[i] = arr[left + i];
for (int j = 0; j < n2; j++)
R[j] = arr[mid + 1 + j];
int i = 0, j = 0, k = left;
while (i < n1 && j < n2) {
if (L[i] <= R[j]) {
arr[k] = L[i];
i++;
} else {
arr[k] = R[j];
j++;
}
k++;
}
while (i < n1) {
arr[k] = L[i];
i++;
k++;
}
while (j < n2) {
arr[k] = R[j];
j++;
k++;
}
}
This code snippet efficiently merges two halves of the array by first copying the elements into temporary arrays and then merging them back.
The Merge Sort Function
The merge sort function orchestrates the overall sorting process through recursion:
void mergeSort(vector<int>& arr, int left, int right) {
if (left < right) {
int mid = left + (right - left) / 2;
mergeSort(arr, left, mid);
mergeSort(arr, mid + 1, right);
merge(arr, left, mid, right);
}
}
This function checks if the left index is less than the right. If so, it calculates the mid-point and recursively sorts the two halves.
Putting It All Together
Complete Code Example
To tie everything together, here’s the full implementation of merge sort in C++:
#include <iostream>
#include <vector>
using namespace std;
void merge(vector<int>& arr, int left, int mid, int right) {
int n1 = mid - left + 1;
int n2 = right - mid;
vector<int> L(n1), R(n2);
for (int i = 0; i < n1; i++)
L[i] = arr[left + i];
for (int j = 0; j < n2; j++)
R[j] = arr[mid + 1 + j];
int i = 0, j = 0, k = left;
while (i < n1 && j < n2) {
if (L[i] <= R[j]) {
arr[k] = L[i];
i++;
} else {
arr[k] = R[j];
j++;
}
k++;
}
while (i < n1) {
arr[k] = L[i];
i++;
k++;
}
while (j < n2) {
arr[k] = R[j];
j++;
k++;
}
}
void mergeSort(vector<int>& arr, int left, int right) {
if (left < right) {
int mid = left + (right - left) / 2;
mergeSort(arr, left, mid);
mergeSort(arr, mid + 1, right);
merge(arr, left, mid, right);
}
}
int main() {
vector<int> arr = {38, 27, 43, 3, 9, 82, 10}; // sample data
mergeSort(arr, 0, arr.size() - 1);
cout << "Sorted array: ";
for (int i : arr)
cout << i << " ";
cout << endl;
return 0;
}
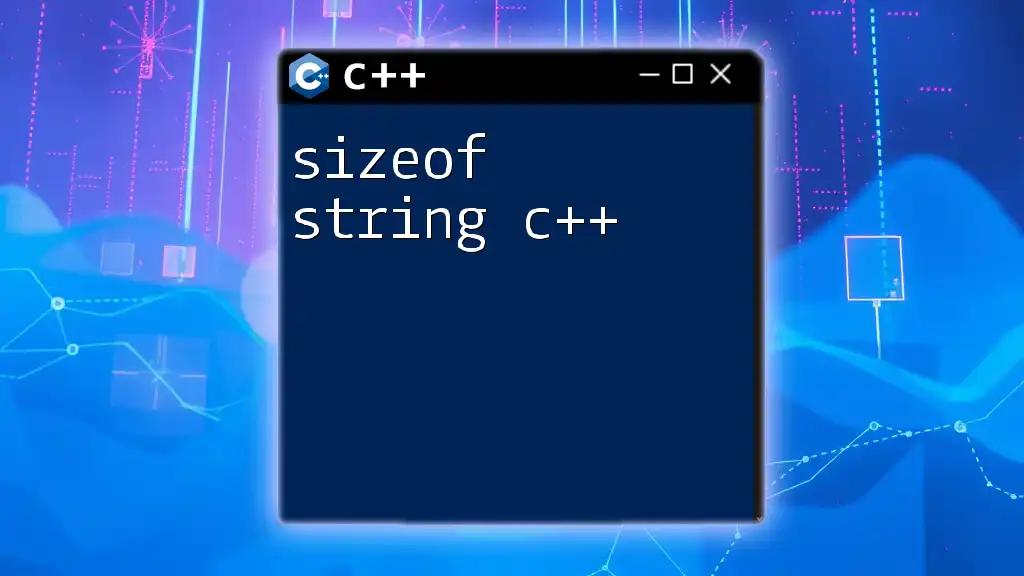
Advanced Techniques
Optimizing Merge Sort
While merge sort is efficient, there are ways to enhance its performance:
- Early Exit: Before proceeding with sorting, you can check if the array is already sorted, which can dramatically speed up the sorting process for nearly sorted data.
- In-Place Merge Sort: Implementing a merge sort that minimizes the use of extra space can be complex but beneficial in high-memory scenarios.
Iterative Merge Sort
An alternative to the recursive implementation is an iterative merge sort, which utilizes a bottom-up approach. This avoids the overhead of multiple function calls and can be advantageous in certain performance contexts:
void iterativeMergeSort(vector<int>& arr) {
int n = arr.size();
for (int curr_size = 1; curr_size <= n - 1; curr_size = 2 * curr_size) {
for (int left_start = 0; left_start < n - 1; left_start += 2 * curr_size) {
int mid = min(left_start + curr_size - 1, n - 1);
int right_end = min(left_start + 2 * curr_size - 1, n - 1);
merge(arr, left_start, mid, right_end);
}
}
}
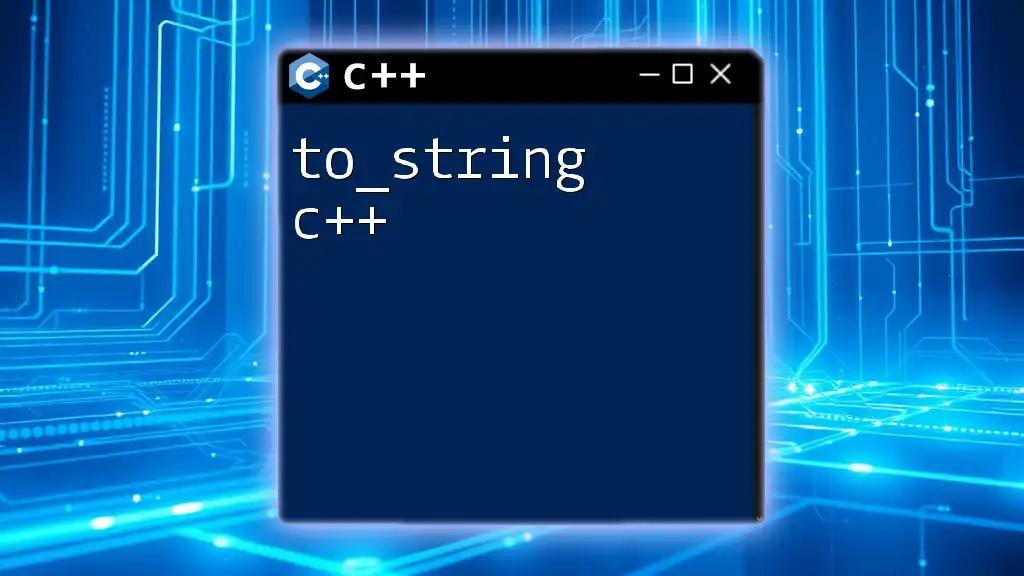
Applications of Merge Sort
Real-World Use Cases
Merge sort is commonly employed in applications where large amounts of data need to be sorted efficiently. Due to its stable sort properties, it finds use in sorting linked lists and files where maintaining order is crucial.
In particular, merge sort shines in scenarios involving external sorting, where data doesn’t fit into memory. The algorithm can handle chunks sorted in-memory and merged later, making it indispensable for database management systems.
Merge Sort in Algorithmic Competitions
In competitive programming, knowing when to use merge sort is vital. Its stability and predictable performance can lead to solving problems efficiently, especially when required to sort lists or handle large inputs quickly.
When participating in contests, focus on writing clean, optimized merge sort implementations that you can adapt in various problems involving sorting.
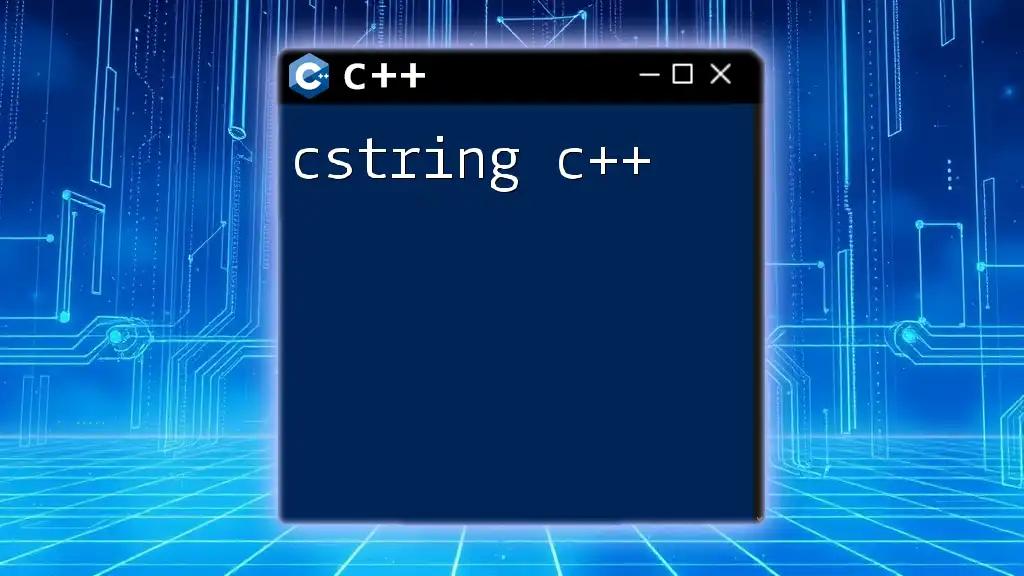
Conclusion
Summary of Key Points
In this comprehensive guide, we explored merge sorting in C++, covering its definition, operation, the code implementation, and its advantages and drawbacks. Merge sort's O(n log n) time complexity makes it highly efficient, while its stability is a considerable advantage for various sorting tasks.
Further Resources
For those looking to deepen their understanding, consider exploring additional resources such as textbooks on algorithms, online coding platforms for practice, and knowledgeable communities where you can discuss and refine your coding skills.
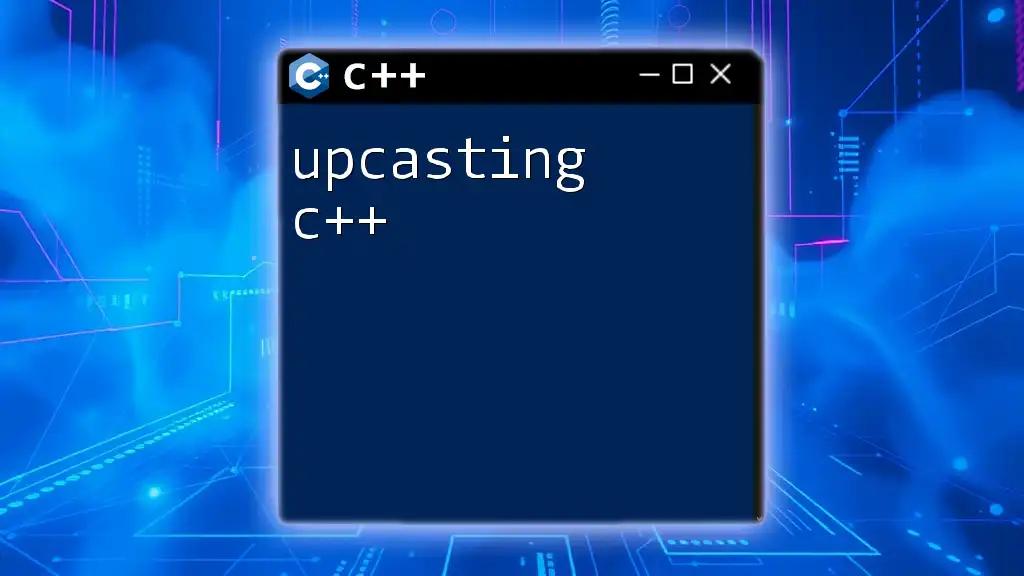
FAQs about Merge Sort in C++
Common Questions and Answers
-
What are the advantages of using merge sort?
Merge sort provides predictable performance, stability, and is well-suited for large datasets and scenarios with a requirement for stable sorting. -
Can merge sort be used for linked lists?
Yes, merge sort is particularly effective for linked lists as it requires minimal pointer changes and is efficient for datasets that grow dynamically. -
How does merge sort handle large datasets?
Merge sort excels with large datasets through its external sorting capabilities, allowing it to sort data that doesn't fit into memory by processing chunks sequentially and merging results.