String slicing in C++ can be achieved using the `substr` method, which allows you to extract a substring from a given string based on a starting position and length.
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
std::string sliced = str.substr(7, 5); // Extracts "World"
std::cout << sliced << std::endl; // Output: World
return 0;
}
Understanding C++ Strings
C++ String Types
In C++, there are predominantly two types of strings: C-style strings and std::string.
-
C-style strings are arrays of characters that end with a null terminator (`\0`). They require manual management of memory and can lead to issues if not handled correctly.
-
std::string, included in the C++ Standard Library, offers a richer set of functionalities. It automatically manages memory, offers member functions for manipulation, and is generally easier and safer to work with for most applications.
The key advantage of using std::string is its ability to handle various operations like concatenation, comparison, and dynamic resizing without the programmer needing to manage the underlying memory manually.
Basic String Operations in C++
Before diving deeper into string slicing in C++, it's essential to understand some basic string operations:
-
Concatenation: Joining two or more strings together using the `+` operator.
-
Comparison: Comparing strings lexicographically using comparison operators.
-
Length and Capacity: Using the `.length()` or `.size()` methods to obtain the number of characters in a string.
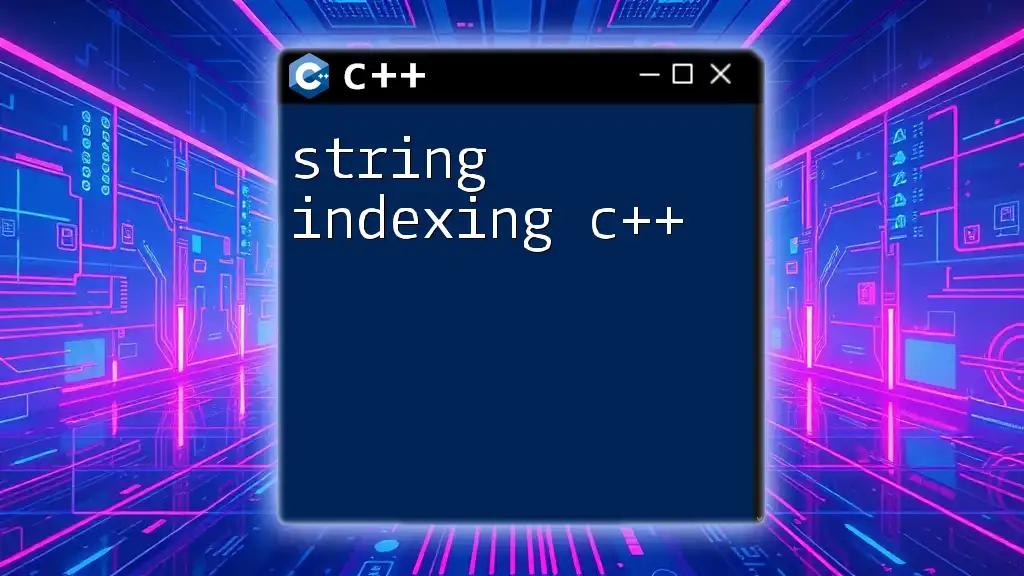
What is String Slicing?
String slicing is the technique of extracting a subset of characters from a string. This operation is especially crucial in data manipulation and text processing where specific substrings are required for analysis or modification.
Think of string slicing as a way to "cut" a string into smaller parts. It can be visualized as selecting a segment from a line of text, much like using scissors to cut a piece of paper.
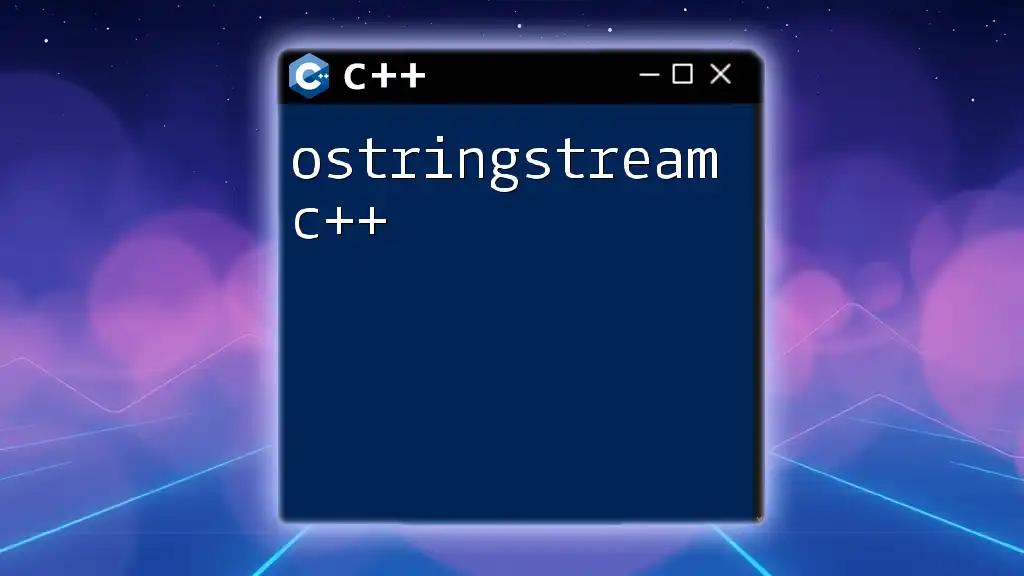
How to Slice Strings in C++
Using Substr Method
A fundamental method for slicing strings in C++ is `std::string::substr`.
-
Definition: The `substr` method creates a substring starting from a specified index and extends for a given length.
-
Syntax: The typical syntax is `string.substr(start, length)`.
-
Example:
#include <iostream>
#include <string>
int main() {
std::string text = "Hello, World!";
std::string slice = text.substr(0, 5);
std::cout << slice << std::endl; // Output: Hello
return 0;
}
In this example, we extract the substring starting at index 0 with a length of 5, resulting in "Hello".
Handling Edge Cases
When using `substr`, it’s crucial to consider edge cases to prevent runtime errors. If the starting index exceeds the string's length, it may lead to undefined behavior.
-
To guard against this:
- Use `std::string::npos` to denote "not found" for lengths that aren’t predetermined.
-
Example:
std::string text = "Hello, World!";
std::string slice = text.substr(7); // from index 7 to the end
std::cout << slice << std::endl; // Output: World!
In this instance, we safely slice from index 7 to the end of the string, yielding "World!".
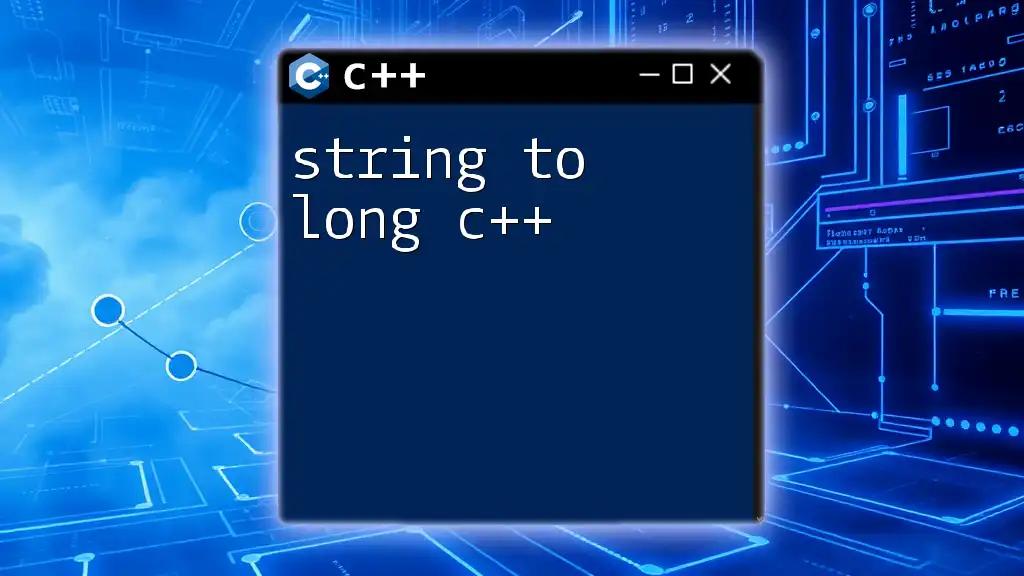
Advanced String Slicing Techniques
Slicing with Iterators
Another way to slice strings is using iterators, which provides a more flexible approach.
-
Definition: Iterators are similar to pointers and can traverse through the characters of a string.
-
You can slice a string using iterators, effectively defining start and end points for the substring.
-
Example:
std::string text = "Programming";
std::string slice(text.begin() + 3, text.begin() + 6);
std::cout << slice << std::endl; // Output: gra
Here, we select characters from the 3rd to the 6th position, yielding "gra".
Custom Functions for Slicing
Creating reusable functions to encapsulate slicing behavior can enhance code readability and maintainability.
-
Benefits: This modular approach allows for easy updates and reusability throughout your code.
-
Example: A custom slice function might look like this:
std::string customSlice(const std::string& str, size_t start, size_t length) {
if (start >= str.size()) return "";
return str.substr(start, length);
}
This function checks the starting index and safely returns a substring, handling cases where the start may be out of bounds.
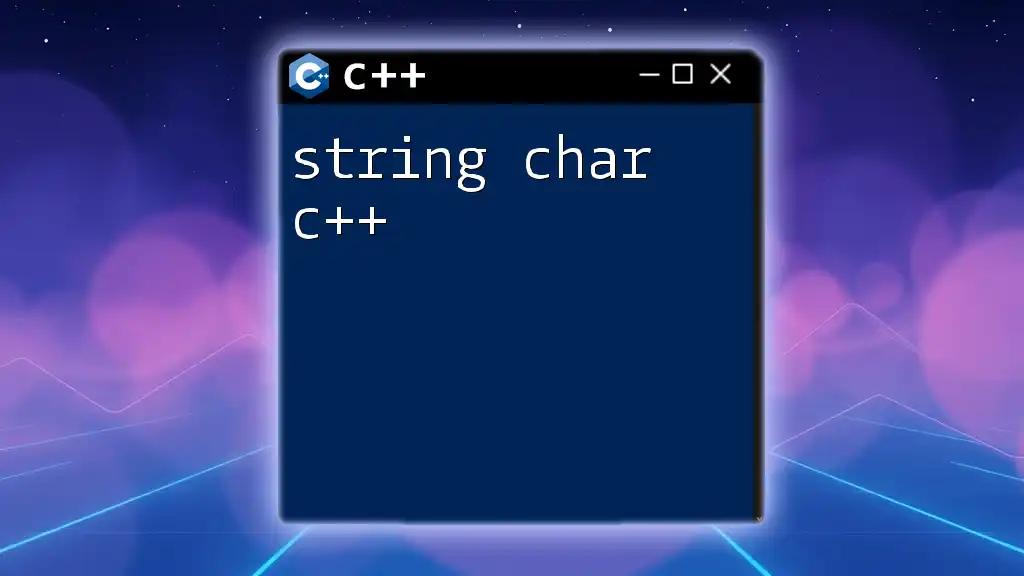
Performance Considerations
While string slicing is straightforward, it requires careful consideration regarding performance.
-
Memory Allocation: Each substring operation creates a new string, leading to potential overhead in high-performance applications.
-
Impact of Copying: Frequent slicing operations can lead to excessive copying of string data. Understanding the use of references and move semantics introduced in C++11 can mitigate this issue.
For optimal performance, consider the scope and frequency of your string slicing needs.

Practical Applications of String Slicing
Data Parsing
String slicing proves invaluable in data parsing. For example, when processing CSV data, slicing allows for easy extraction of values.
- Example: Extracting values from a comma-separated string.
std::string data = "name,age,city";
std::string name = data.substr(0, data.find(','));
std::cout << name << std::endl; // Output: name
In this snippet, we extract the name before the first comma, highlighting its applicability in parsing structured formats.
Text Processing
In fields such as natural language processing, string slicing is widely applied to segment text into useful components, whether that be extracting sentences, words, or phrases.
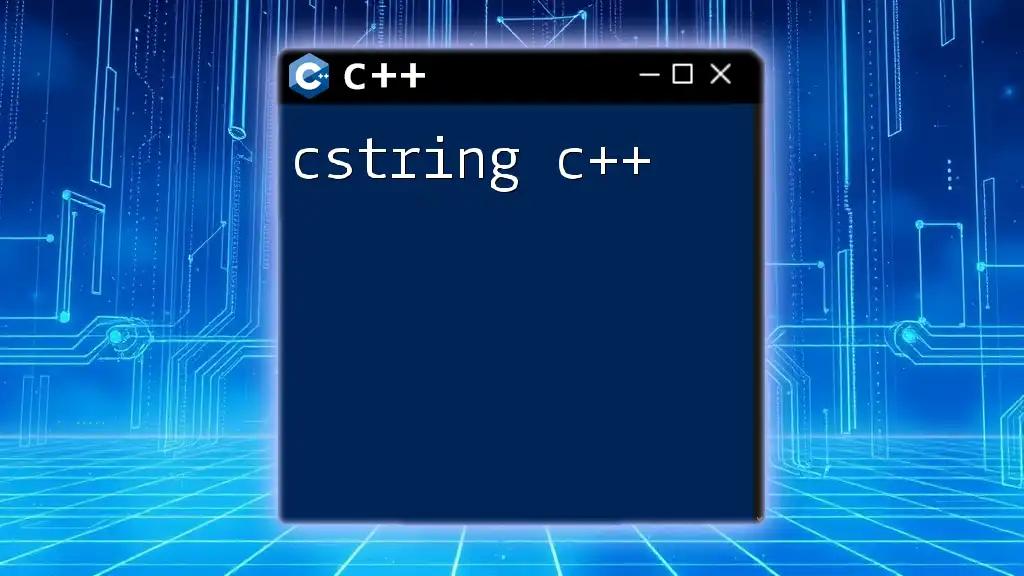
Conclusion
Mastering string slicing in C++ empowers programmers to manipulate text effectively and efficiently. By utilizing the `substr` method, iterators, and custom functions, developers can tackle a myriad of tasks from data parsing to text processing.
We encourage you to practice these techniques and explore their applications further. String manipulation is a critical skill in any programmer's toolbox, and proficiency in it can significantly advance your coding ability.