Multithreading in C++ allows for the concurrent execution of multiple threads to improve program efficiency and responsiveness, typically managed using the C++11 `<thread>` library.
Here's a simple example of creating two threads that print different messages:
#include <iostream>
#include <thread>
void function1() {
std::cout << "Hello from thread 1!" << std::endl;
}
void function2() {
std::cout << "Hello from thread 2!" << std::endl;
}
int main() {
std::thread t1(function1);
std::thread t2(function2);
t1.join();
t2.join();
return 0;
}
Understanding Multithreading Concepts
What is Multithreading?
Multithreading refers to the ability of a CPU, or a single core in a multicore processor, to provide multiple threads of execution simultaneously. In simpler terms, it allows a program to perform multiple tasks at the same time. This is especially useful for optimizing performance and resource utilization in applications that require concurrent processing, such as gaming, server operations, and scientific computing.
The primary difference between multithreading and single-threading lies in the execution of tasks. In a single-threaded environment, tasks are executed sequentially, which can lead to inefficient CPU use, especially during I/O operations. Meanwhile, multithreading enables the overlapping of tasks, which can significantly reduce waiting time and increase throughput.
Threads and Processes
To fully grasp multithreading in C++, it's essential to understand the difference between threads and processes.
Processes are independent executing programs that contain their own memory space. Each process runs separately from the others. In contrast, threads are smaller units of a process that share the same memory space but can execute concurrently. This shared memory model facilitates communication and resource sharing between threads but also necessitates careful management to prevent issues like data races.
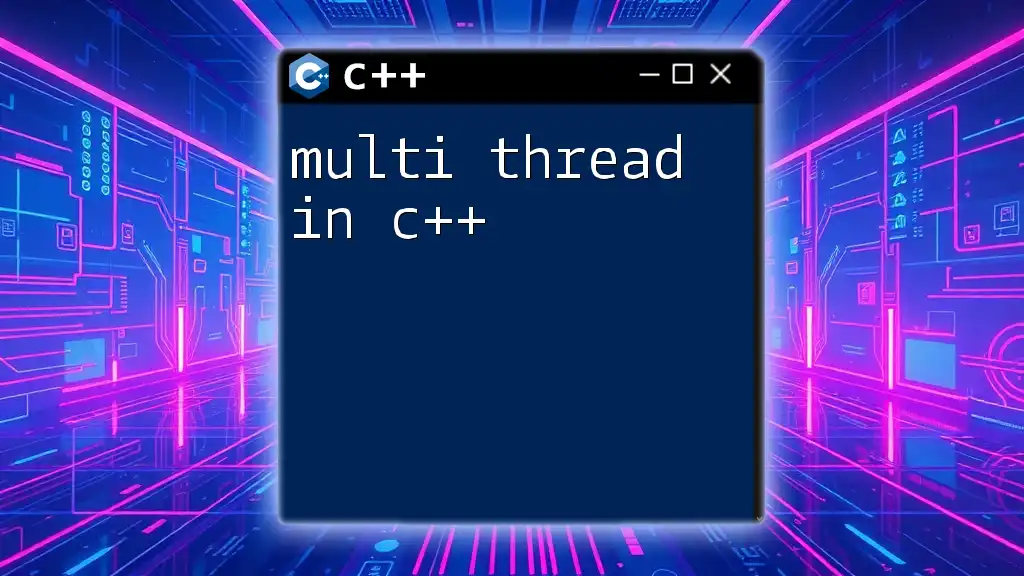
The C++ Standard Library and Multithreading
Overview of C++ Standard Library Support
C++ provides robust support for multithreading starting from the C++11 standard. The `<thread>` header is the primary component for creating and managing threads. This functionality allows developers to write parallel code that can run on multi-core processors efficiently.
Key Components of Multithreading in C++
Here are the major components associated with multithreading in C++:
- Threads: The basic construct that allows the execution of code concurrently.
- Mutexes: Unlike threads, mutexes provide a mechanism for ensuring that only one thread can access shared resources at a time, thereby preventing data corruption.
- Condition Variables: These are used to block a thread until a particular condition is met, allowing for better control over thread execution flow and resource management.
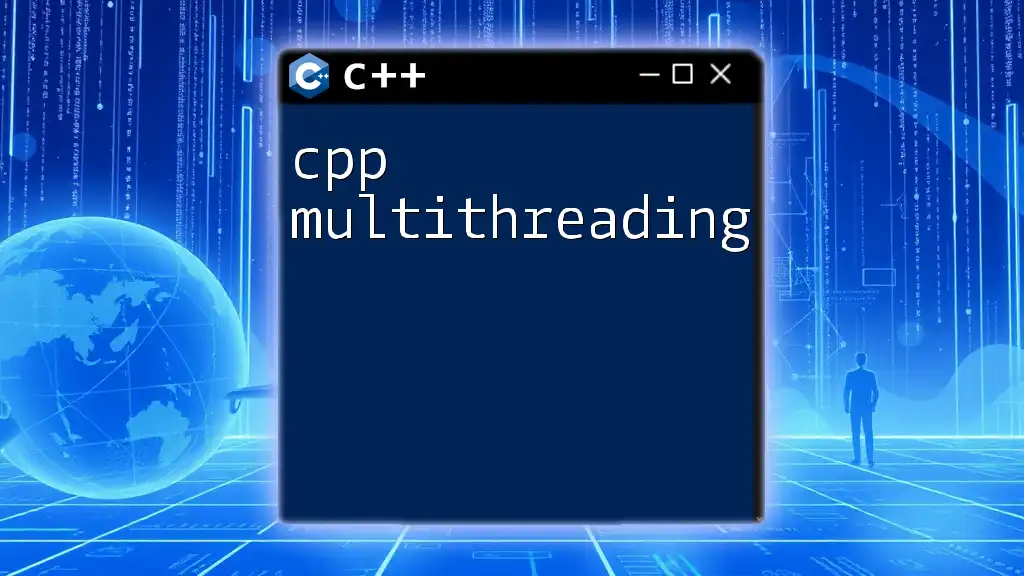
Creating and Managing Threads in C++
Starting a Thread
Creating a thread in C++ is straightforward. You can use the `std::thread` class for this purpose. Here’s how you can start a thread with a simple example:
#include <iostream>
#include <thread>
void hello() {
std::cout << "Hello from thread!" << std::endl;
}
int main() {
std::thread t(hello); // Create a new thread that executes the hello function
t.join(); // Wait for the thread to finish
return 0;
}
In this example, we define a function `hello` that prints a message. We then create a thread `t` that runs the `hello` function. The `join()` method waits for the thread to complete its execution before the program moves on, ensuring proper synchronization.
Joining and Detaching Threads
In multithreading, managing threads effectively is vital. Joining and detaching threads are two essential operations.
- The `join()` method blocks the calling thread until the thread associated with `t` has finished executing.
- The `detach()` method allows the thread to continue executing independently, freeing the thread object. Here's a sample code snippet demonstrating both:
std::thread t(hello);
if (some_condition) {
t.join(); // Wait for the thread to finish
} else {
t.detach(); // Let the thread run independently
}
Using `detach()` can be advantageous for fire-and-forget tasks, but caution should be exercised since you lose control over the thread.
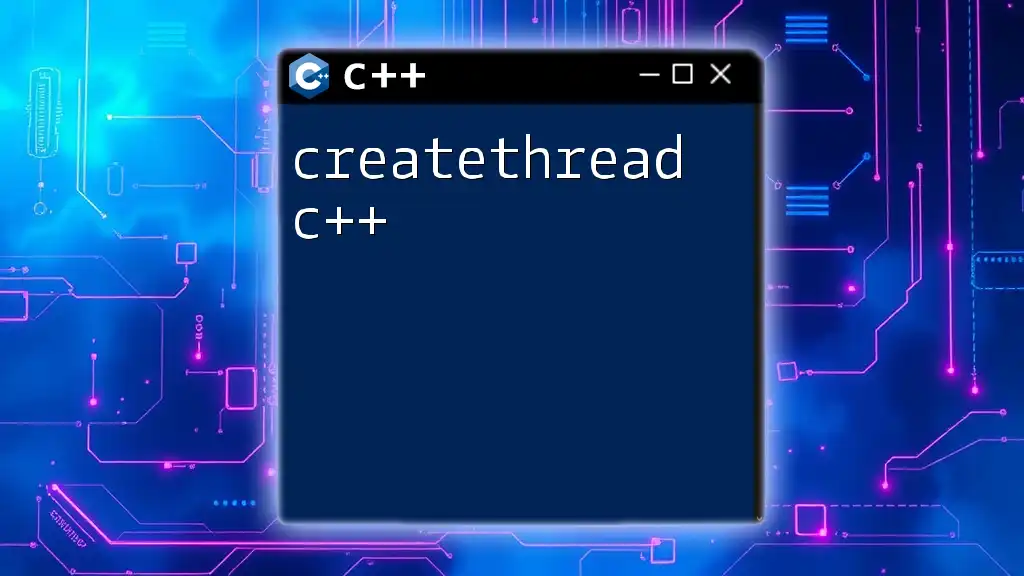
Synchronization Mechanisms in C++
Introduction to Synchronization
As threads run concurrently, those that access shared data can lead to race conditions. Synchronization is crucial in multithreading as it ensures that only one thread can access shared resources at a time, preventing data inconsistencies and unexpected behavior.
Mutexes
A mutex (mutual exclusion) is a synchronization primitive that protects shared resources by allowing only one thread to access the resource at a time. Here’s a simple example of using a mutex in C++:
std::mutex mtx;
void safePrint() {
mtx.lock(); // Lock the mutex before accessing shared resources
std::cout << "Thread-safe output!" << std::endl;
mtx.unlock(); // Unlock the mutex afterwards
}
In the `safePrint` function, we use `mtx.lock()` to ensure that no other thread can enter this section of code until the lock is released via `mtx.unlock()`.
Condition Variables
Condition variables facilitate communication between threads. They allow threads to wait for certain conditions within a concurrent program. If the condition is not met, using the `wait()` method will block the thread until another thread signals it.
Consider the following example with `std::condition_variable`:
#include <condition_variable>
#include <mutex>
std::condition_variable cv;
std::mutex cv_m;
void worker() {
std::unique_lock<std::mutex> lk(cv_m);
cv.wait(lk); // Wait until notified
// Execute event after waking up...
}
In this code, the worker thread will block on `cv.wait(lk)` until it's notified. This ensures that threads can coordinate their actions effectively.
![Understanding Literals in C++ [A Quick Guide]](/images/posts/l/literals-in-cpp.webp)
Advanced Multithreading Techniques
Thread Pools
A thread pool is an architectural pattern that involves creating a fixed number of threads (worker threads) that are reused to execute a large number of tasks. This approach minimizes the overhead associated with thread creation and destruction. Here’s how you might implement a simple thread pool:
class ThreadPool {
// Implementation for managing a pool of worker threads
};
This class would handle adding tasks to the pool and managing the lifecycle of worker threads efficiently.
Handling Exceptions in Threads
Handling exceptions in a multithreaded environment requires careful consideration. If a thread throws an exception, it may terminate unexpectedly, affecting other running threads. Here's how to manage exceptions within a thread:
void threadFunction() {
try {
throw std::runtime_error("Error in thread!");
} catch (const std::exception& e) {
std::cerr << "Caught exception: " << e.what() << std::endl;
}
}
Using try-catch blocks ensures that exceptions are captured and handled gracefully, allowing the program to continue running or shutdown cleanly.
Atomic Operations
To facilitate safe operations on shared variables, atomic operations come into play. These operations are performed entirely or not at all, eliminating race conditions. C++ provides the `std::atomic` template to work with atomic types conveniently:
std::atomic<int> counter(0);
Using atomic types guarantees that operations on them are thread-safe without needing explicit locks, thus improving performance.
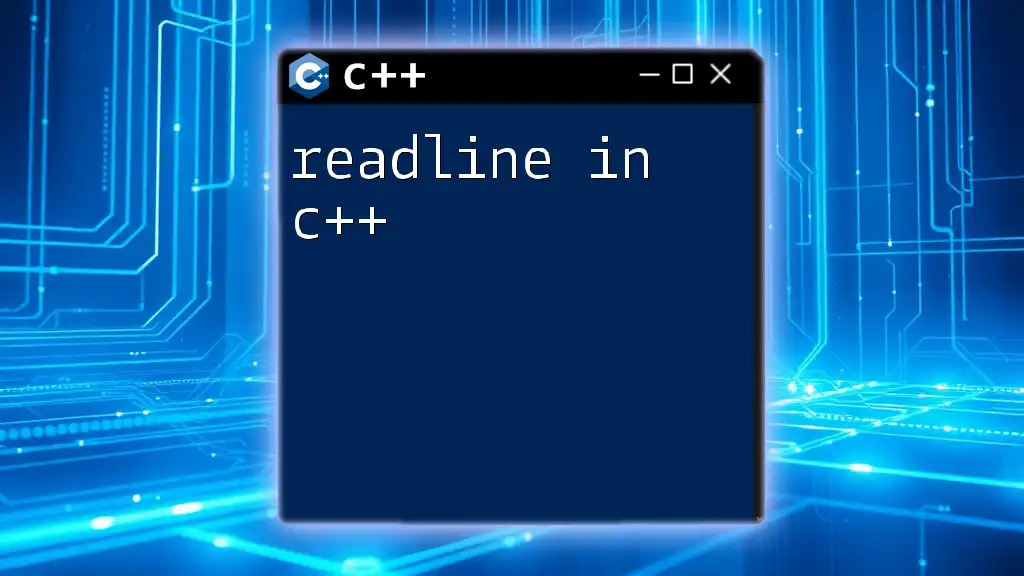
Best Practices for Multithreading in C++
When implementing multithreading in C++, adhere to these best practices:
- Avoid Shared State: Whenever possible, minimize the use of shared data between threads. Prefer passing data by value.
- Use Mutexes Wisely: Always lock and unlock mutexes carefully to avoid deadlocks, and consider using RAII with `std::lock_guard`.
- Prefer Thread Pools: Use thread pools for managing tasks instead of creating new threads for each task to reduce overhead.
- Profile Performance: Measure and analyze the performance of your multithreaded code to identify bottlenecks.
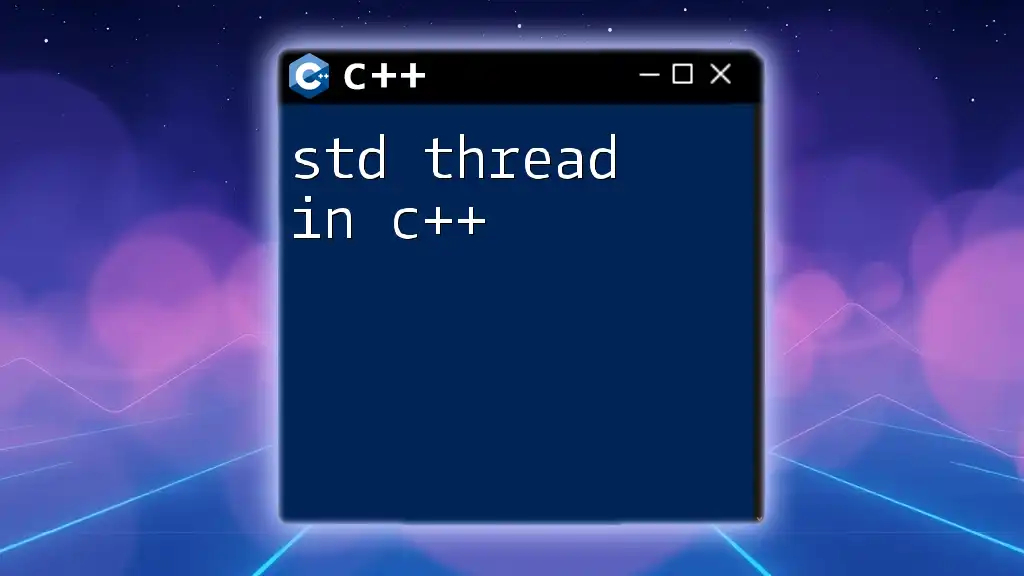
Conclusion
Multithreading is an essential skill in C++ programming that enhances performance, efficiency, and responsiveness in applications. By understanding the mechanisms, best practices, and advanced techniques associated with multithreading, developers can leverage C++ to create high-performing applications.
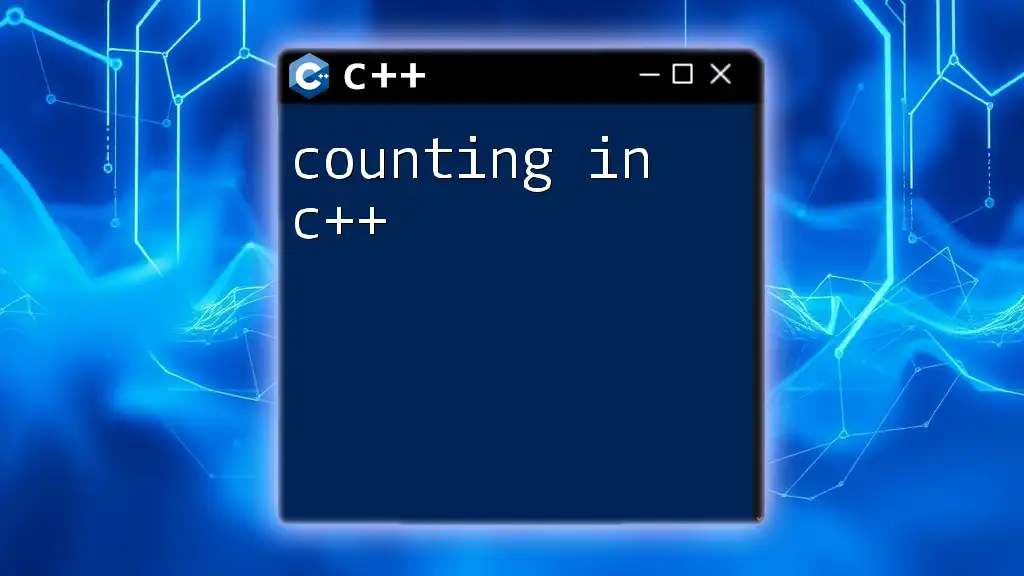
References
Further reading on multithreading in C++ can deepen your understanding and competence in this crucial area of programming.
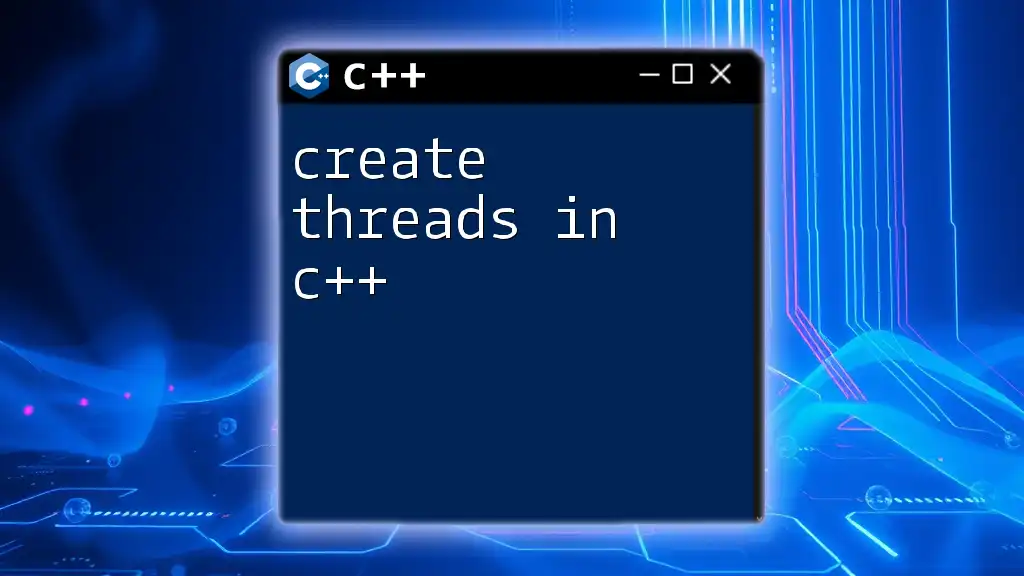
Call to Action
Follow our company for more concise tutorials and insights on C++ programming, including specialized content on multithreading and optimization techniques.