The `thread_local` storage class specifier in C++ allows you to define variables that are unique to each thread, enabling safe access to data without the need for locks.
#include <iostream>
#include <thread>
thread_local int counter = 0; // Each thread has its own instance of counter
void increment() {
for (int i = 0; i < 5; ++i) {
++counter;
std::cout << "Thread ID: " << std::this_thread::get_id() << ", Counter: " << counter << std::endl;
}
}
int main() {
std::thread t1(increment);
std::thread t2(increment);
t1.join();
t2.join();
return 0;
}
What is `thread_local`?
`thread_local` is a storage class specifier in C++ that allows you to declare variables that are local to each thread. Each thread gets its own separate instance of this variable, which means modifications made by one thread do not affect the value of the variable in another thread. This functionality is essential for developing efficient multithreaded applications where data consistency is vital.
The primary difference between `thread_local` and standard variable storage is that with `thread_local`, you have isolated data, ensuring thread safety. This is crucial in high-performance environments where you want to maximize parallel execution while avoiding data races.

Why Use `thread_local`?
Improved Performance
In multithreaded applications, traditional global or local variables can lead to undue contention among threads. With `thread_local`, each thread maintains its own copy of the variable, which significantly reduces contention and enhances performance.
Consider the following example that demonstrates speed advantages when using `thread_local` storage compared to regular variables:
#include <iostream>
#include <thread>
#include <vector>
thread_local int threadLocalCounter = 0;
void incrementCounter() {
for (int i = 0; i < 1000; ++i) {
++threadLocalCounter;
}
std::cout << "Thread-local counter: " << threadLocalCounter << "\n";
}
int main() {
std::vector<std::thread> threads;
for (int i = 0; i < 10; ++i) {
threads.emplace_back(incrementCounter);
}
for (auto& t : threads) {
t.join();
}
return 0;
}
Using `thread_local` ensures that each thread has its own `threadLocalCounter`, enabling non-blocking increments and thus better performance across concurrent operations.
Avoiding Data Races
When multiple threads access shared data without proper synchronization, it can lead to data races, which introduce undefined behaviors in your program. By using `thread_local`, each thread's instance is completely separate, eliminating the risk of these data races.
Here’s an illustration of how data races can manifest when using non-thread-local variables:
#include <iostream>
#include <thread>
#include <vector>
int counter = 0;
void increment() {
for (int i = 0; i < 1000; ++i) {
++counter; // Data race
}
}
int main() {
std::vector<std::thread> threads;
for (int i = 0; i < 10; ++i) {
threads.emplace_back(increment);
}
for (auto& t : threads) {
t.join();
}
std::cout << "Final counter value (expected 10000): " << counter << "\n"; // May be incorrect
return 0;
}
In this example, `counter` is accessed by multiple threads concurrently, which can lead to unpredictable and incorrect results.

How to Declare a `thread_local` Variable
Syntax of `thread_local`
To declare a variable as `thread_local`, you can simply prefix the variable declaration with the `thread_local` keyword:
thread_local int myThreadLocalVar = 0;
This variable will be initialized for each thread that accesses it, ensuring each thread works with its own instance.
Allowed Data Types
You can use `thread_local` with various data types. From built-in types to complex objects, `thread_local` can be applied broadly:
thread_local std::string myString = "Hello, Thread Local!";
thread_local std::vector<int> myVector;
This means you can leverage `thread_local` not only for primitive types but also for objects and containers, making it versatile for many scenarios.

Accessing `thread_local` Variables
Accessing and modifying `thread_local` variables is performed just like accessing global or local variables, but within the context of a function. Here's an example:
void modifyThreadLocal() {
thread_local int count = 0;
++count;
std::cout << "Count for this thread: " << count << "\n";
}
When this function is called from different threads, each will maintain its own copy of `count`, providing insight into how often the function has been executed per thread.
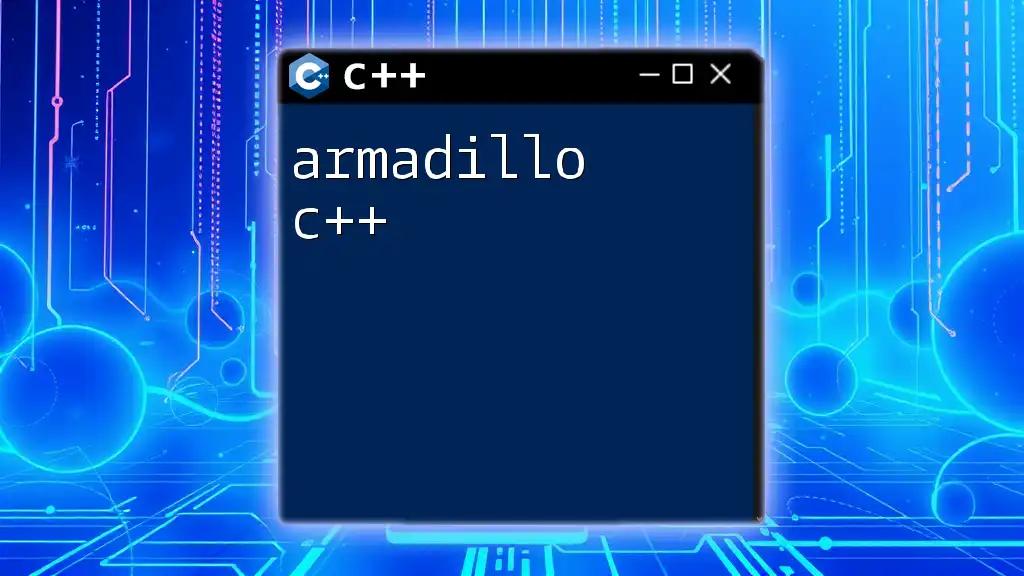
Scope of `thread_local`
Lifetime of `thread_local` Variables
The lifetime of `thread_local` variables extends for the duration of the thread. When the thread ends, the `thread_local` variable is destroyed. This is comparable to how local variables’ lifetimes are tied to the function in which they are defined.
Visibility in Multithreading
One of the most crucial aspects of `thread_local` is visibility. Each thread has its separate instance of the `thread_local` variable, meaning changes made in one thread are invisible to other threads. Here’s an illustrative example:
#include <iostream>
#include <thread>
thread_local int localVariable = 0;
void threadFunction(int id) {
localVariable = id;
std::cout << "Thread " << id << " sees localVariable: " << localVariable << "\n";
}
int main() {
std::thread t1(threadFunction, 1);
std::thread t2(threadFunction, 2);
t1.join();
t2.join();
return 0;
}
In this code, Thread 1 and Thread 2 each set `localVariable`, but their changes remain isolated within their threads.
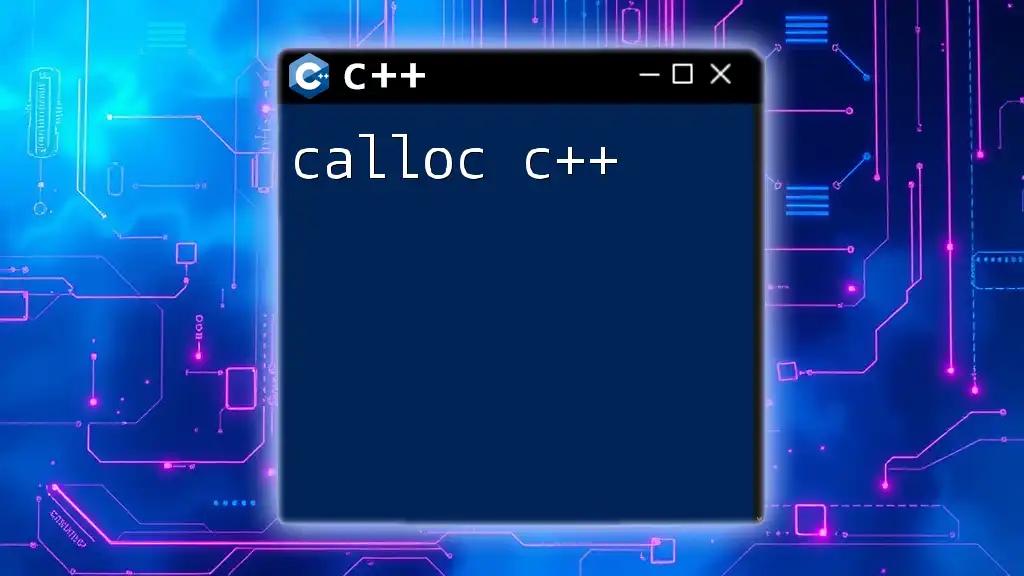
Potential Pitfalls and Best Practices
Misunderstandings about `thread_local`
A common misunderstanding is that `thread_local` provides automatic synchronization or makes variables thread-safe. While `thread_local` eliminates data races concerning the variable itself, you still need to manage synchronization if multiple threads read/write to shared data concurrently.
Best Practices
-
Limit Usage: Use `thread_local` variables only when necessary. Excessive use may lead to increased memory usage and complexity.
-
Initialization: Ensure that objects declared as `thread_local` can be initialized correctly for each thread context, especially for types with non-trivial constructors.
-
Thread Management: Always be conscious of the total number of threads. Creating too many threads may lead to unexpected overhead.
-
Debugging: Use debugging tools to trace variable values across threads. Mistakes involving `thread_local` can sometimes be elusive.
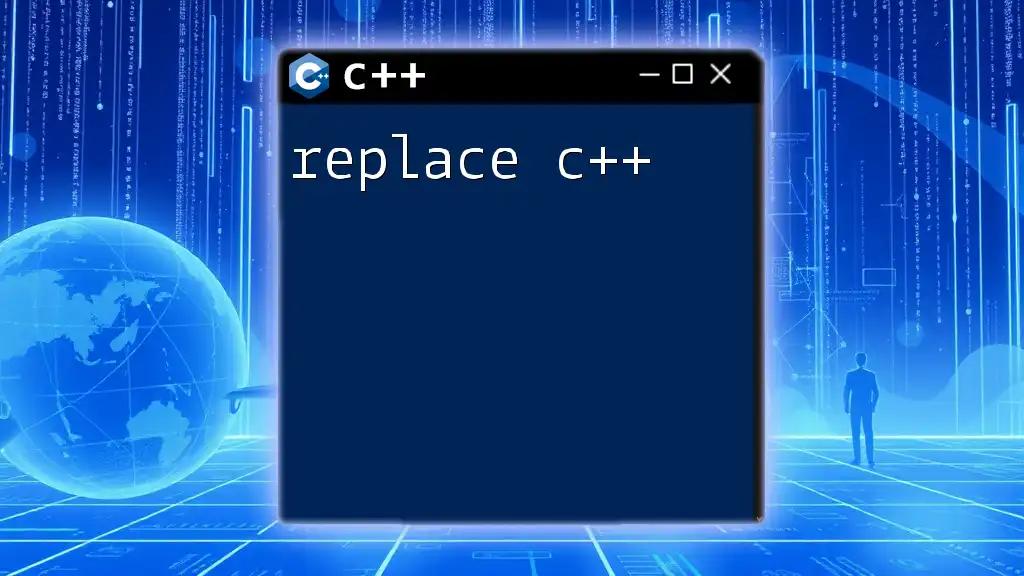
Conclusion
By understanding and leveraging `thread_local` in C++, you unlock the power to write efficient and thread-safe code. This feature not only boosts performance by minimizing contention but also contributes significantly to the safe management of thread data. As you explore multithreading in C++, keep in mind the best practices discussed and experiment with `thread_local` to discover its full potential in your applications.

Further Reading
To expand your knowledge on this topic, consider checking out the C++ documentation on `thread_local`, as well as exploring additional resources on concurrency and multithreading in C++. Books and articles focused on these subjects can provide deeper insights and advanced techniques.