Thread local storage in C++ allows you to create variables that are local to a thread, ensuring that each thread has its own separate instance of that variable.
#include <iostream>
#include <thread>
thread_local int threadLocalVar = 0;
void increment() {
threadLocalVar++;
std::cout << "Thread ID: " << std::this_thread::get_id() << ", Value: " << threadLocalVar << std::endl;
}
int main() {
std::thread t1(increment);
std::thread t2(increment);
t1.join();
t2.join();
return 0;
}
What is Thread Local Storage?
Definition of Thread Local Storage
Thread Local Storage (TLS) is a programming feature that allows variable data to be stored in a thread-specific manner. This means that each thread manages its own instance of a variable, making it isolated from other threads. Essentially, each thread maintains a separate copy of the variable, unlike global or static variables which are shared among all threads in a process.
Benefits of Using Thread Local Storage
Using thread local storage in C++ provides several advantages, especially in the context of multithreading:
- Performance Optimizations: Each thread can access its own data without locking mechanisms, reducing the overhead of thread synchronization.
- Avoiding Data Races: Since each thread has a distinct instance of the variable, data races—situations where two or more threads attempt to modify a shared variable simultaneously—are mitigated, significantly improving thread safety.

How to Implement Thread Local Storage in C++
The `thread_local` Keyword
In C++, the `thread_local` storage duration is implemented using the `thread_local` keyword. This modifier tells the compiler to allocate a separate instance of the variable for each thread.
Example of Basic Usage:
#include <iostream>
#include <thread>
thread_local int tls_var = 0;
void threadFunction() {
tls_var++;
std::cout << "Thread local variable: " << tls_var << std::endl;
}
int main() {
std::thread t1(threadFunction);
std::thread t2(threadFunction);
t1.join();
t2.join();
return 0;
}
In this example, we define a thread-local variable `tls_var`, which is incremented by each thread independently. As a result, the threads will not interfere with one another, maintaining their own state.
Custom Types with Thread Local Storage
TLS can also be applied to user-defined types such as structures or classes. Each thread will maintain its own instance of the custom type.
Example of a Thread-Local Object:
#include <iostream>
#include <thread>
struct ThreadLocalCounter {
int count = 0;
};
thread_local ThreadLocalCounter counter;
void incrementCounter() {
counter.count++;
std::cout << "Count: " << counter.count << std::endl;
}
int main() {
std::thread t1(incrementCounter);
std::thread t2(incrementCounter);
t1.join();
t2.join();
return 0;
}
Here, we define a structure `ThreadLocalCounter` with a single member `count`. Each thread increments its own `count`, demonstrating how custom types can leverage thread-local storage.

Understanding the Lifecycle of Thread Local Storage
Initialization and Destruction
Thread-local variables are initialized the first time a thread accesses the variable. This means that in a multithreaded environment, each thread will initialize its own copy independently, promoting encapsulation within the thread's scope.
When a thread terminates, its thread-local variables are destroyed automatically, which helps in managing resource lifecycles seamlessly without additional cleanup needed.
Visibility Across Threads
A crucial aspect of thread local storage is that thread-local variables are not shared between threads at all. This isolation ensures that each thread maintains its own state without concerns about other threads overwriting or corrupting data.

Common Use Cases for Thread Local Storage
Managing Thread-Specific Resources
Thread local storage is particularly useful when managing resources that are inherently thread-specific. Examples include:
- Caching data for performance optimizations that are unique to each thread's execution context.
- Storing thread-specific configurations or user contexts that need to persist across function calls within the same thread.
Implementing Thread-Specific Logging
Another practical use of TLS is in logging systems. This can be especially useful for multithreaded applications where each thread needs to maintain its logging context without interference.
Example of Thread-Local Logging:
#include <iostream>
#include <thread>
#include <string>
thread_local std::string threadName;
void threadFunction() {
threadName = std::to_string(std::this_thread::get_id().hash());
std::cout << "Thread ID: " << threadName << " performing work." << std::endl;
}
int main() {
std::thread t1(threadFunction);
std::thread t2(threadFunction);
t1.join();
t2.join();
return 0;
}
In this example, each thread sets its unique identifier into `threadName`, and logs its own activity independently.

Potential Pitfalls of Thread Local Storage
Overhead Considerations
While TLS provides benefits, it comes with performance overhead. Allocating separate instances for each thread might increase memory usage, especially if the number of threads is significant or if the thread-local variables are bulky. Careful consideration should be given to the scale and context in which TLS is used.
Compatibility Issues
Thread local storage is supported in most modern compilers and platforms; however, older C++ standards (before C++11) do not support `thread_local`. As such, developers should ensure compatibility based on the target environment.
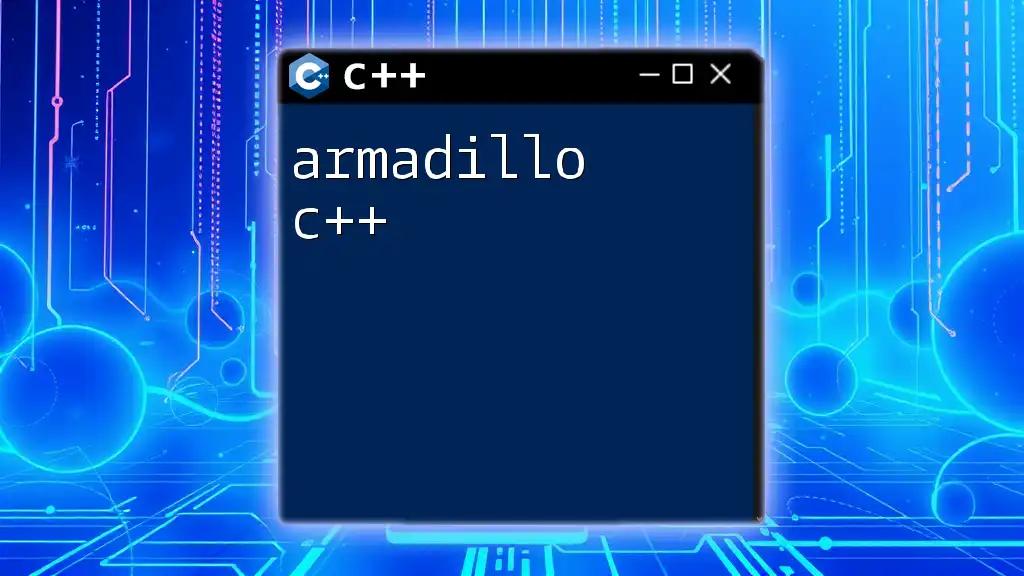
Alternatives to Thread Local Storage
Other Synchronization Mechanisms
While TLS is a great option, other synchronization mechanisms like mutexes, condition variables, and atomic types also play vital roles in managing thread safety. These tools are useful for cases where data needs to be shared across threads safely.
Comparing TLS with Immutable Objects
Immutable objects provide an alternative approach for safely sharing data across threads. When data cannot be altered post-creation, race conditions are avoided, promoting safety without relying on thread local storage.
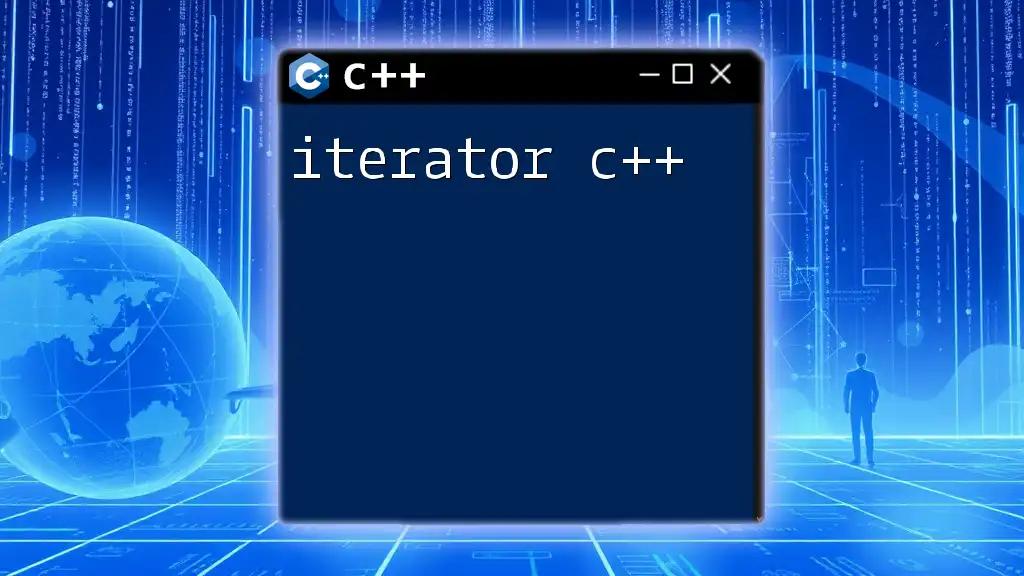
Best Practices for Using Thread Local Storage
Keep It Simple
When utilizing thread local storage, simplicity is key. Overusing TLS can lead to complex code that is harder to maintain. Using TLS judiciously only where it provides substantial benefits helps keep the codebase clean.
Documenting Thread Local Variables
Proper documentation is essential when using thread-local variables, especially in large codebases. Clearly indicating where TLS is employed ensures that team members understand data isolation and the threading model, enhancing maintainability and readability.

Conclusion
Thread local storage in C++ is an invaluable tool for enhancing multithreading safety and performance. By understanding how TLS operates, its lifecycle, and potential pitfalls, developers can harness its benefits effectively. Whether managing thread-specific resources or developing thread-safe logging systems, leveraging thread local storage leads to cleaner, safer, and more efficient multithreaded applications.

Additional Resources
For those looking to deepen their understanding of thread local storage and multithreading in C++, various books, articles, and online resources are available. The official C++ documentation is also an excellent starting point for exploring the capabilities of `thread_local` and related features.