In C++, the `throw` statement is used to signal the occurrence of an exceptional condition (an error), which can then be handled by a corresponding `catch` block.
#include <iostream>
#include <stdexcept>
void testFunction() {
throw std::runtime_error("An error occurred!");
}
int main() {
try {
testFunction();
} catch (const std::runtime_error& e) {
std::cout << "Caught: " << e.what() << std::endl;
}
return 0;
}
Understanding Exception Handling in C++
In C++, exceptions serve as a powerful mechanism for handling errors and unexpected situations in a robust manner. An exception is an event that occurs during the execution of a program that disrupts the normal flow of control, typically due to issues like invalid user input or resource unavailability. Exception handling allows developers to manage these situations gracefully without crashing the program.
Utilizing exception handling not only makes your programs more resilient but also improves code clarity by separating error-handling logic from regular code flow. This leads to better maintainability and readability.
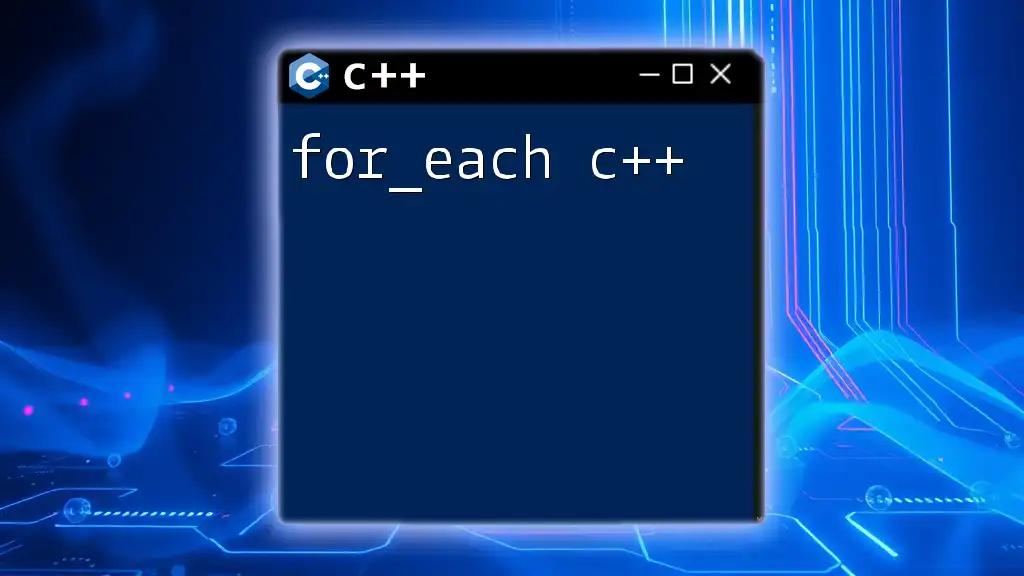
The Basics of Try-Catch in C++
What is a Try-Catch Block?
A try-catch block is a fundamental construct in C++ for managing exceptions. The code that may potentially throw an exception is wrapped in a `try` block, and `catch` blocks follow to handle those exceptions. The program execution gets diverted to the `catch` block when an exception is thrown.
How Exception Handling Works
When an exception is thrown using the `throw` keyword, the runtime checks the stack to find a matching catch block that can handle that type of exception. If matched, control transfers to the appropriate catch block, effectively unwinding the stack until the exception is handled.

Syntax of Throw, Try, and Catch in C++
Basic Syntax
The syntax for using `throw`, `try`, and `catch` can be summarized as follows:
try {
// Code that may throw an exception
} catch (const ExceptionType& e) {
// Code to handle the exception
}
This structure allows you to define a block of code (the `try` block) that is evaluated, and if an exception occurs, control is transferred to the corresponding `catch` block.
Detailed Syntax Breakdown
- throw: This keyword is used to trigger an exception, often followed by an instance of an exception type.
- try: The block of code that is safeguarded from exceptions. If any exception is thrown in this block, the control moves to the matching catch block.
- catch: This block contains the code that executes if the specific exception is thrown, allowing for proper error handling.
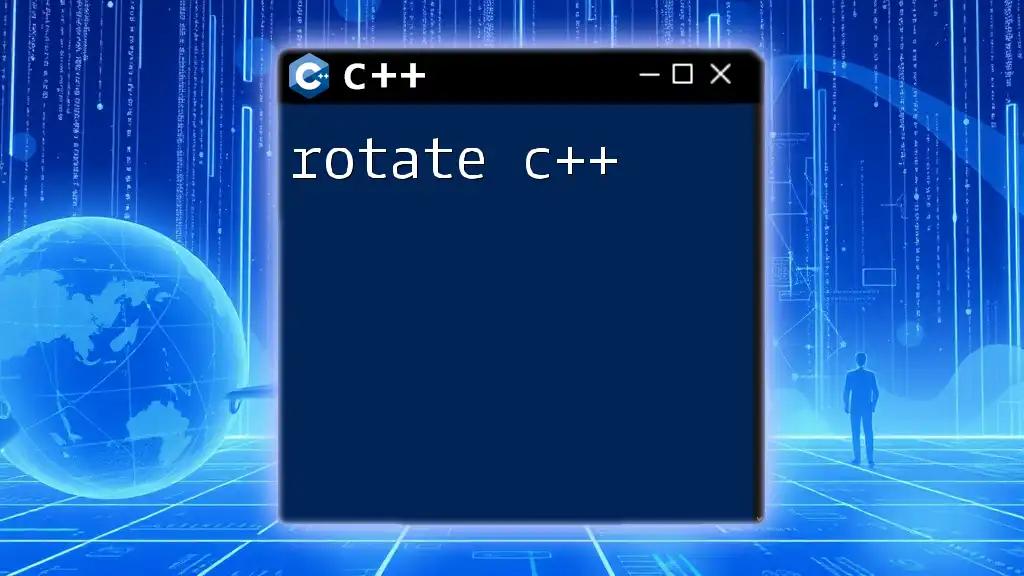
Try Catch in C++ Example
Simple Example of Try-Catch
A basic example of a try-catch block is illustrated below. In this case, we are throwing a runtime error:
#include <iostream>
#include <stdexcept>
int main() {
try {
throw std::runtime_error("An error occurred");
} catch (const std::exception& e) {
std::cout << "Caught: " << e.what() << std::endl;
}
return 0;
}
In this example, the code attempts to throw a runtime error. The `catch` block captures the exception and prints an informative message to the console. The output will be:
Caught: An error occurred
More Complex Try Catch Example in C++
Here’s a more practical example where we handle user input and perform division. If the user attempts to divide by zero, an exception will be thrown:
#include <iostream>
#include <stdexcept>
int divide(int numerator, int denominator) {
if (denominator == 0) {
throw std::invalid_argument("Denominator cannot be zero");
}
return numerator / denominator;
}
int main() {
try {
std::cout << "Result: " << divide(10, 0) << std::endl;
} catch (const std::invalid_argument& e) {
std::cout << "Caught: " << e.what() << std::endl;
}
return 0;
}
In this example, the `divide` function checks if the `denominator` is zero, triggering an exception when it is. The `catch` block captures this specific exception and prints an error message. The output will be:
Caught: Denominator cannot be zero
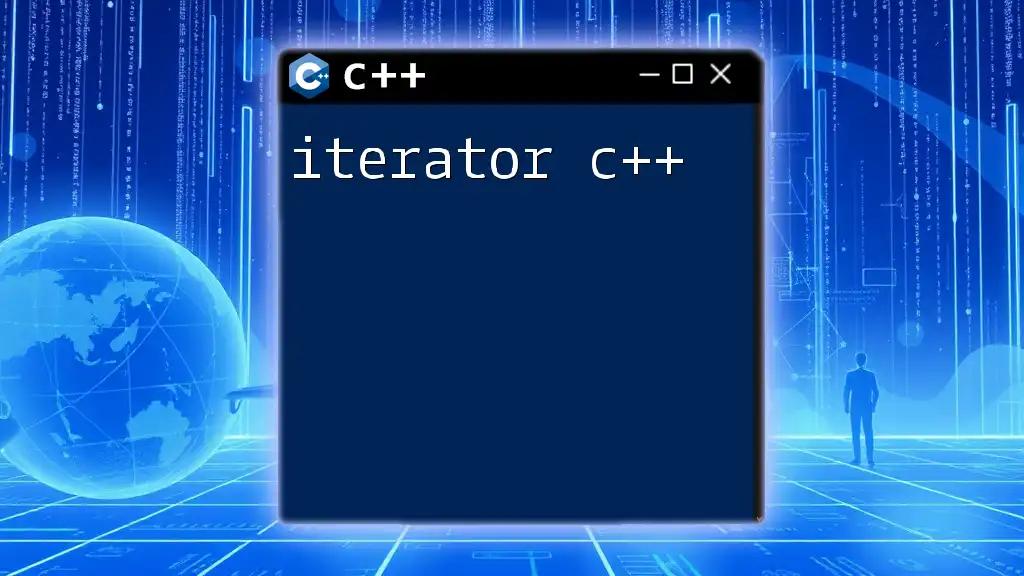
Throwing Custom Exceptions in C++
Why Create Custom Exceptions?
Creating custom exceptions allows developers to provide more context and specificity in error handling. They are particularly useful for distinguishing between different types of errors that may occur within the application. A custom exception can carry additional information or a unique message that identifies the exact issue.
Example of Custom Exception in C++
Here’s how to define and use a custom exception in C++:
#include <iostream>
#include <stdexcept>
class MyException : public std::exception {
public:
const char* what() const noexcept override {
return "Custom Exception Caught";
}
};
int main() {
try {
throw MyException();
} catch (const MyException& e) {
std::cout << "Caught: " << e.what() << std::endl;
}
return 0;
}
In this code, `MyException` derives from `std::exception` and overrides the `what()` method to provide a descriptive message. When the exception is thrown, the custom message is displayed when caught. The output will be:
Caught: Custom Exception Caught
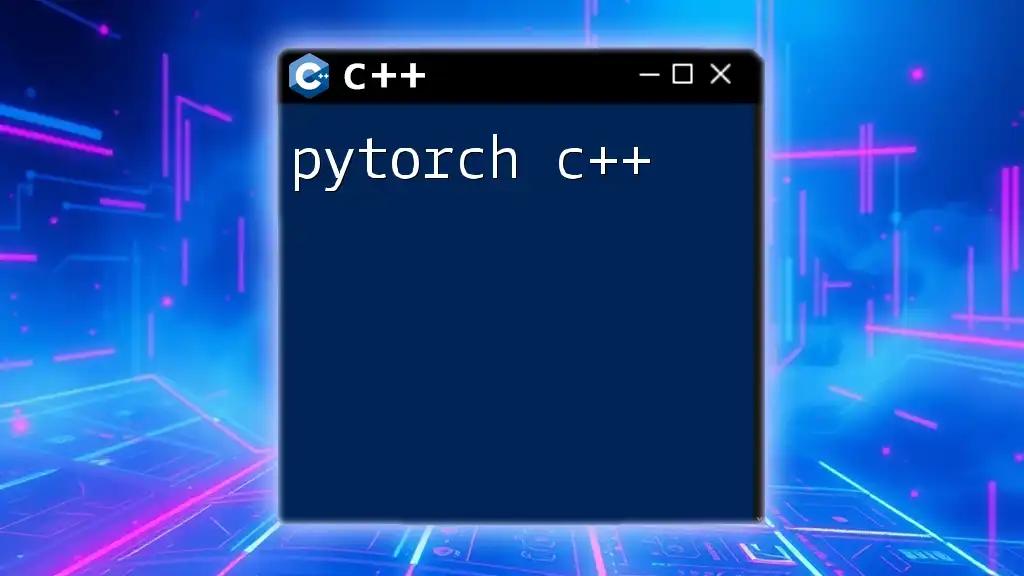
Best Practices for Exception Handling in C++
When to Use Exceptions
Exceptions should primarily be used for exceptional circumstances—situations that are not trivial or expected. Scenarios such as resource allocation failures, file read errors, or network interruptions are typical cases where throwing exceptions may be appropriate. Generally, it’s advisable to avoid using exceptions for regular control flow as it can lead to confusing code.
Catching Exceptions Effectively
When catching exceptions, specific catch blocks should be prioritized over general ones. This means catching exceptions by type rather than using `catch(...)`, which will capture all exceptions but may obscure which particular error occurred. Additionally, be cautious about rethrowing exceptions using the `throw;` without specifying an exception to propagate the original context.
Performance Considerations
While exceptions are powerful, they do have performance overhead compared to regular function calls. To minimize slowdowns, avoid throwing exceptions in performance-critical paths and instead validate input before executing operations that might fail.
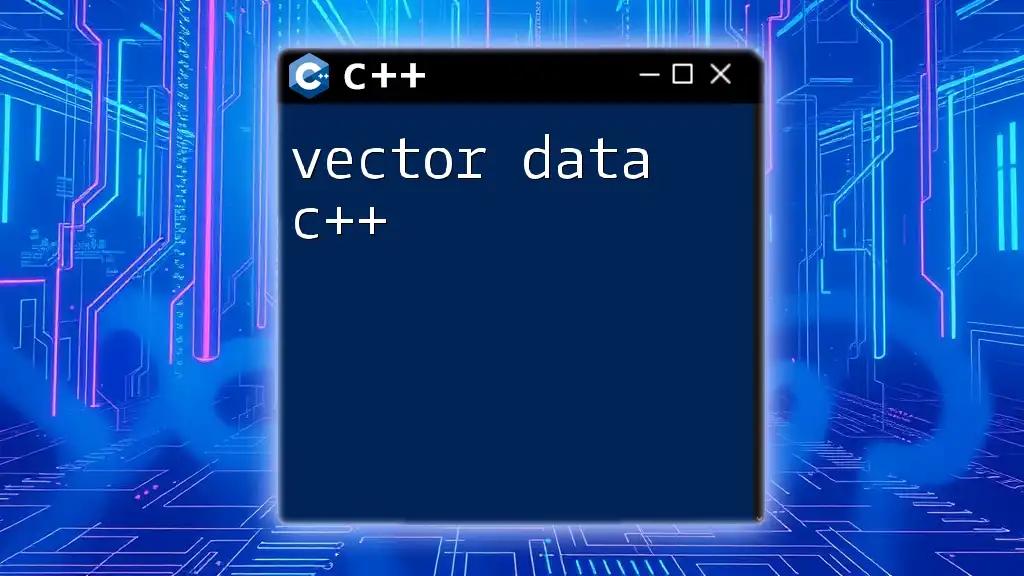
Conclusion
Understanding and mastering the concepts of `throw` and `catch` in C++ enhances your programming capabilities and allows you to write more robust applications. By leveraging exception handling effectively, you can deal with errors gracefully while maintaining clean and readable code. Embrace these techniques to elevate your C++ skills and improve the quality of your programs.
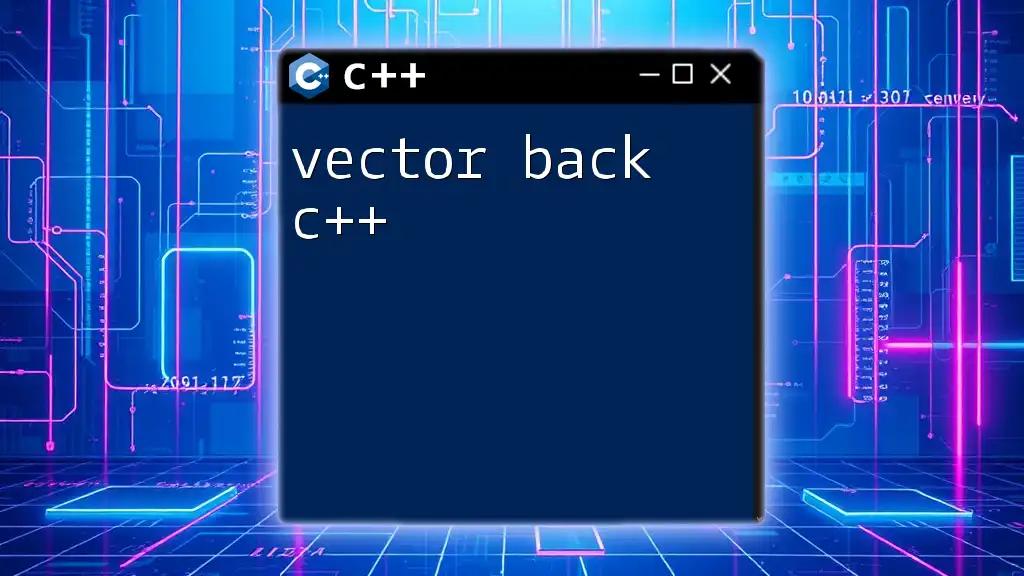
Call to Action
Now it's your turn! Experiment with your own try-catch blocks, explore custom exceptions, and share your experiences or questions in the comments section below. Your feedback and insights are valuable as we all strive to become better C++ developers.