In C++, the `try-catch` block is used for handling exceptions, allowing you to gracefully manage errors without crashing the program.
#include <iostream>
#include <stdexcept>
int main() {
try {
throw std::runtime_error("An error occurred!");
} catch (const std::runtime_error& e) {
std::cout << "Caught: " << e.what() << std::endl;
}
return 0;
}
What Are Exceptions?
Exceptions are unexpected events that can occur during program execution, disrupting the normal flow of instructions. They are crucial for error handling in programming, allowing developers to manage errors gracefully rather than allowing the program to crash or behave unpredictably.
Understanding the difference between errors and exceptions is essential. Errors often indicate serious problems that a reasonable application shouldn't try to catch, while exceptions provide a mechanism to handle anomalies elegantly.
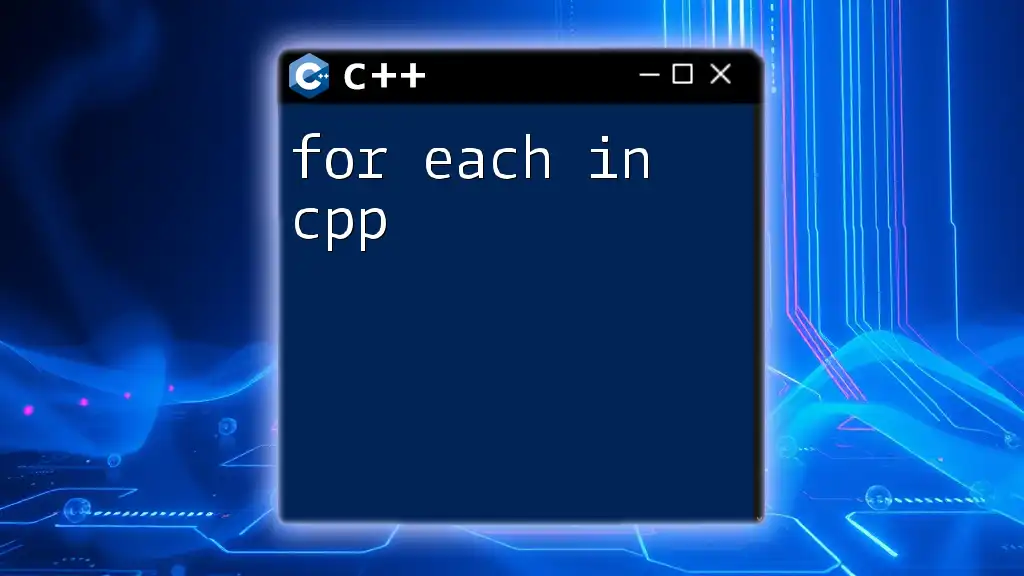
Importance of Exception Handling in C++
Effective exception handling is a vital part of robust programming in C++. By using try-catch blocks, developers can isolate error-prone code segments and maintain control when exceptions arise. This results in more resilient applications capable of functioning correctly even when faced with problems, such as invalid user input or resource unavailability. Furthermore, utilizing exception handling enhances code readability and maintainability by separating error management from standard logic.
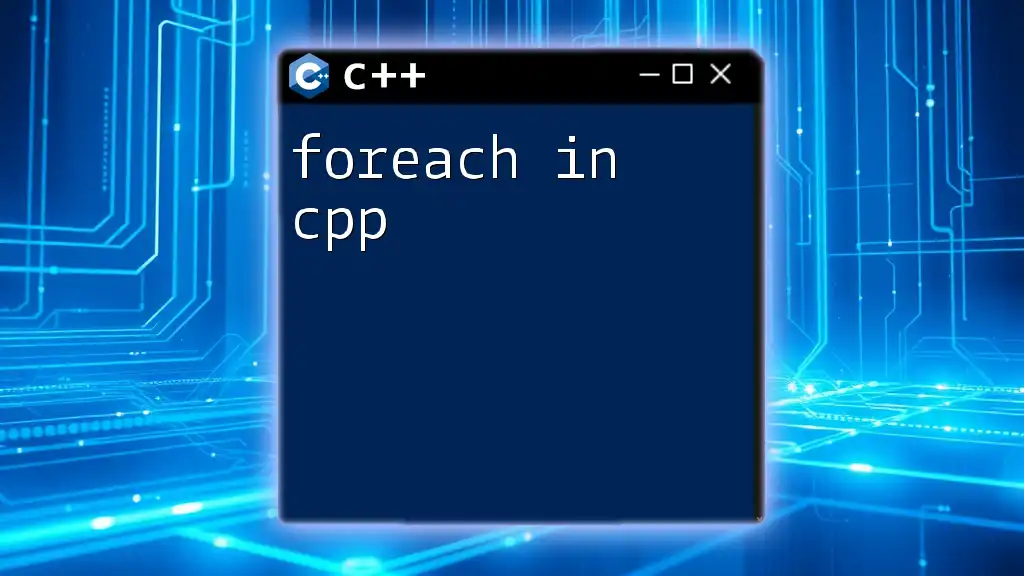
Understanding Try-Catch Blocks
The Structure of Try-Catch
Try Block
A try block contains code that might throw an exception. Placing potentially risky code in this block allows the program to "try" executing it and handle any exceptions should they arise. Here's the basic syntax of a try block:
try {
// Code that may throw an exception
}
Catch Block
Following the try block, a catch block captures and handles the exception. If an exception is thrown in the try block, flow control immediately jumps to the corresponding catch block, where developers can define how to handle the exception. The syntax for a catch block looks like this:
catch (const std::exception& e) {
// Handle the exception
}
Multiple Catch Blocks
When dealing with more than one potential exception, you can utilize multiple catch blocks to handle different types of exceptions separately. This allows for tailored responses depending on the specific issue encountered. Consider the following example:
try {
// Code that may throw exceptions
} catch (const std::runtime_error& e) {
// Handle runtime error
} catch (const std::invalid_argument& e) {
// Handle invalid arguments
}
Here, if a runtime error occurs, the first catch block executes; if an invalid argument is thrown, control transfers to the second catch block.
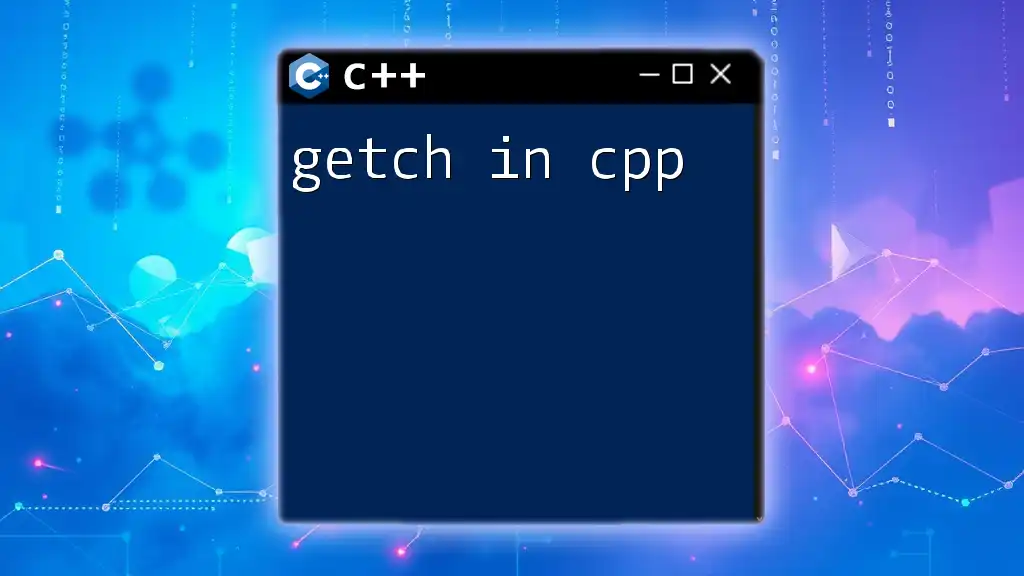
Throwing Exceptions
How to Throw Exceptions in C++
In C++, the `throw` keyword is used to signal that an exception condition has been met. When you throw an exception, you effectively interrupt the normal flow of execution. Here's how to throw exceptions:
throw std::runtime_error("An error occurred");
By throwing an exception, you can communicate to the calling function an unexpected situation that needs to be addressed.
Throwing Custom Exceptions
Creating custom exceptions can be highly beneficial for providing new, specific error types that can carry additional information pertinent to your application. This requires defining a new exception class. For example:
class MyException : public std::exception {
public:
const char* what() const noexcept override {
return "My custom exception occurred";
}
};
throw MyException();
By throwing a custom exception, you're equipping your code with unique error-handling capabilities tailored to the application's needs.
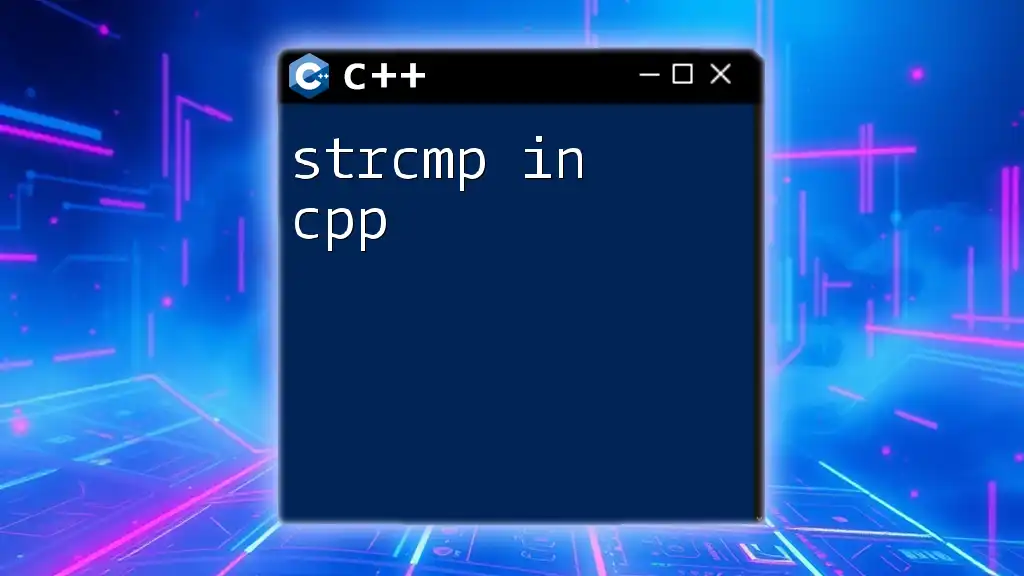
Best Practices for Using Try-Catch
When to Use Try-Catch
While exceptions are powerful, it's essential to use them judiciously. Try-catch blocks are most appropriate for handling unforeseen situations, such as resource access errors, parsing issues, or invalid state conditions. Misusing try-catch for scenarios where you can predict errors (like validation) can complicate debugging and obscure code logic.
Performance Considerations
It's worth noting that while utilizing exception handling provides substantial benefits in terms of reliability, it can have performance implications. Exception handling should not be used for common control flow; it is best reserved for truly exceptional circumstances. In cases where performance is critical, consider using alternative methods, like error codes, to manage issues.
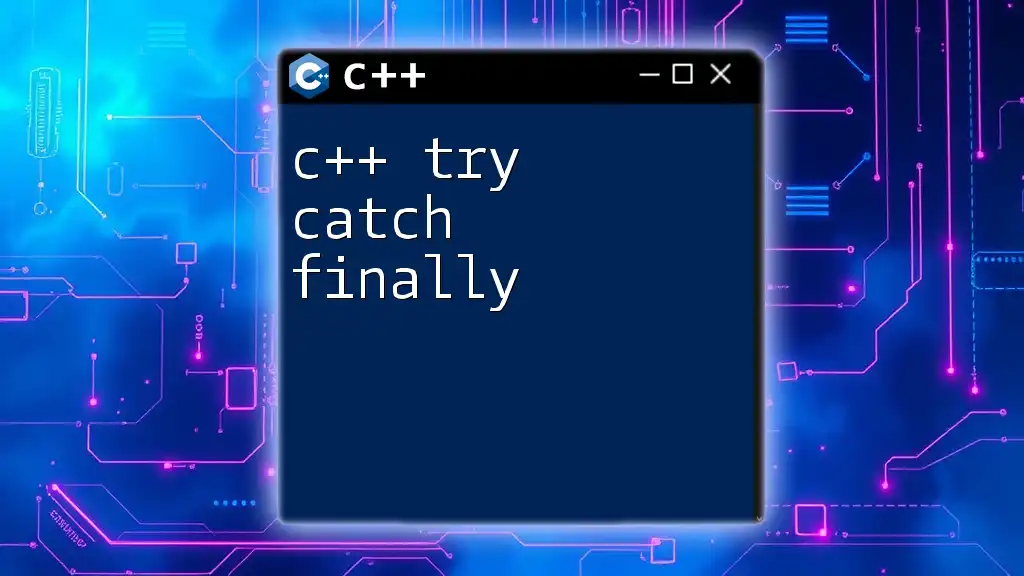
Exception Safety Levels
Understanding Exception Safety
Exception safety is a concept that concerns what happens to program state when exceptions are thrown. It is categorized into several levels of safety:
- No-throw guarantee: The operation cannot throw exceptions.
- Strong guarantee: If an exception occurs, the program state remains unchanged.
- Basic guarantee: The program continues to function correctly, though it may not retain the original state.
Implementing Exception Safety in C++
To implement exception safety effectively, one common strategy involves using resources that automatically manage their cleanup, such as smart pointers. Adopting this practice ensures that memory is correctly freed even when an exception is thrown.
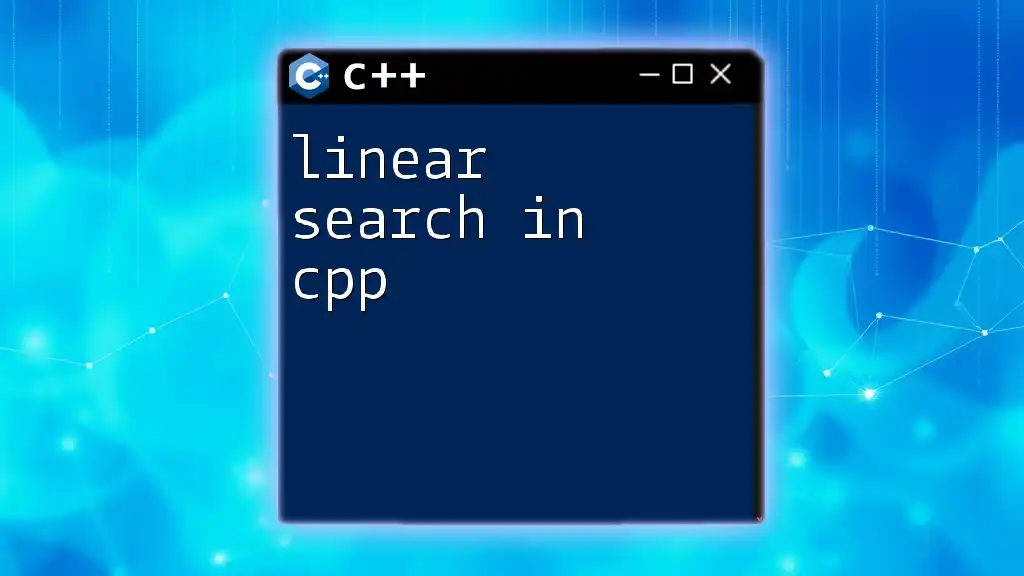
Real-World Examples
Example of File Operations with Try-Catch
When dealing with file operations, exceptions can occur if files aren’t found or accessible. The following code demonstrates how to handle potential exceptions while reading a file:
#include <fstream>
#include <iostream>
void readFile(const std::string& filename) {
try {
std::ifstream file(filename);
if (!file) {
throw std::runtime_error("File cannot be opened");
}
// Process file data
} catch (const std::runtime_error& e) {
std::cerr << e.what() << std::endl;
}
}
In this example, if the file opening fails, a runtime error is thrown, which is then caught and handled within the catch block.
Example of Memory Allocation with Try-Catch
Memory allocation can also fail, particularly in systems with limited resources. Below is an example of how to handle exceptions when dynamically allocating memory using C++ standard containers:
#include <iostream>
#include <vector>
void allocateMemory() {
try {
std::vector<int> vec(1000000000); // This may throw std::bad_alloc
} catch (const std::bad_alloc& e) {
std::cerr << "Memory allocation failed: " << e.what() << std::endl;
}
}
If the allocation exceeds available memory, a `bad_alloc` exception is thrown and caught, allowing the program to respond appropriately.
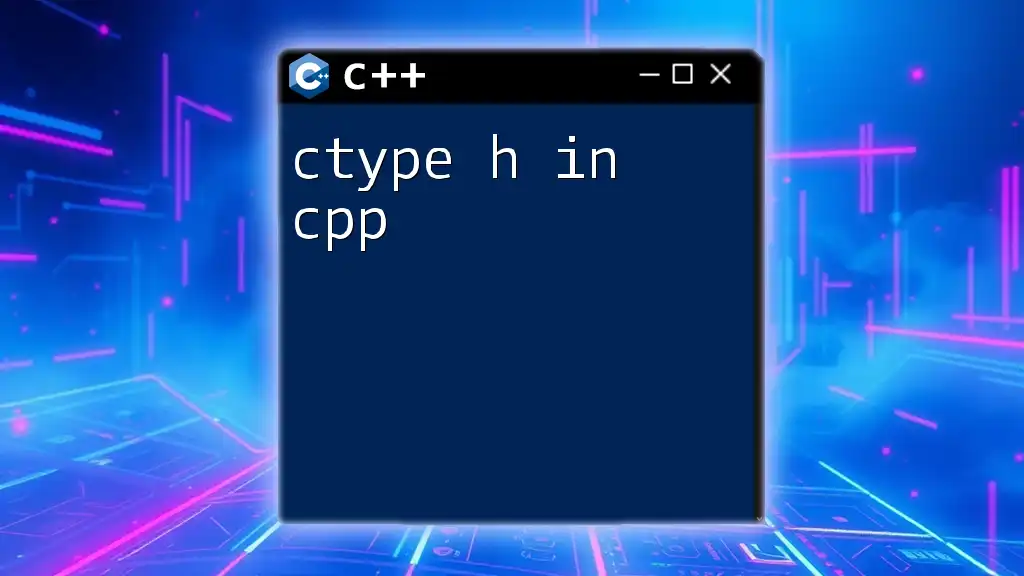
Conclusion
In summary, try catch in cpp is a powerful feature that enhances the robustness of your applications. By wrapping potentially error-prone code in try blocks and employing catch blocks to manage exceptions, you can maintain control of your program's flow even in the face of unexpected conditions. As you explore exception handling in depth, keep in mind best practices that prioritize performance and ensure clarity in your control structures.
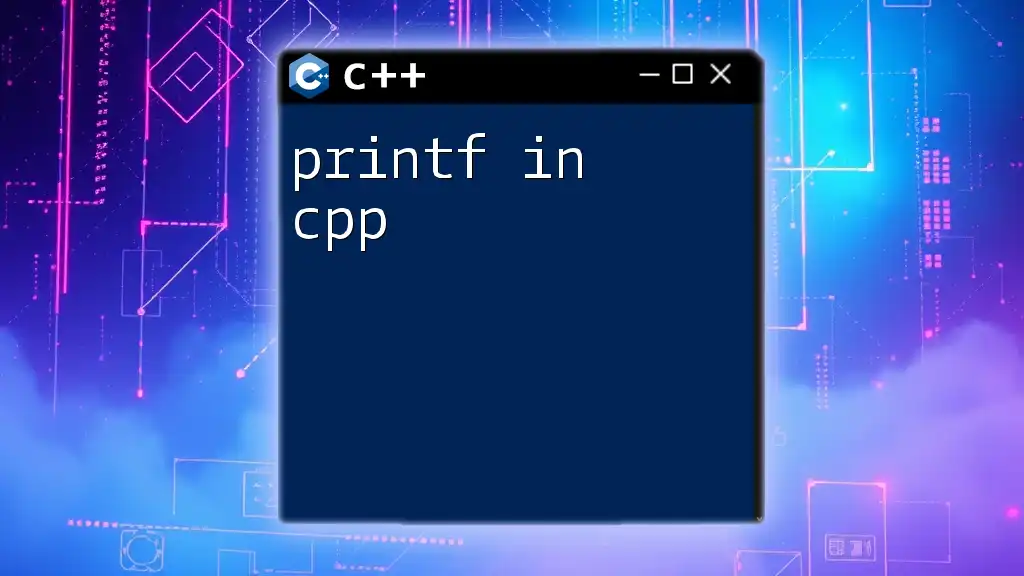
Additional Resources
For further learning, consider exploring books and online courses focused on advanced C++ topics, and engage with online communities or forums dedicated to C++ programming to stay updated on best practices and new techniques in exception handling.