A linear search in C++ is a simple searching algorithm that sequentially checks each element of a list until a match is found or the list ends. Here's a code snippet demonstrating a linear search:
#include <iostream>
using namespace std;
int linearSearch(int arr[], int size, int target) {
for (int i = 0; i < size; i++) {
if (arr[i] == target) {
return i; // return the index of the found element
}
}
return -1; // return -1 if the element is not found
}
int main() {
int arr[] = {5, 3, 8, 4, 2};
int size = sizeof(arr) / sizeof(arr[0]);
int target = 4;
int result = linearSearch(arr, size, target);
(result != -1) ? cout << "Element found at index: " << result
: cout << "Element not found";
return 0;
}
What is Linear Search?
Linear search is a straightforward algorithm used to find a specific element within an array or list. The fundamental operation involves checking each element in the collection sequentially until the target element is found or the entire collection has been traversed. This makes linear search an intuitive and easy-to-implement method, particularly for beginner programmers.
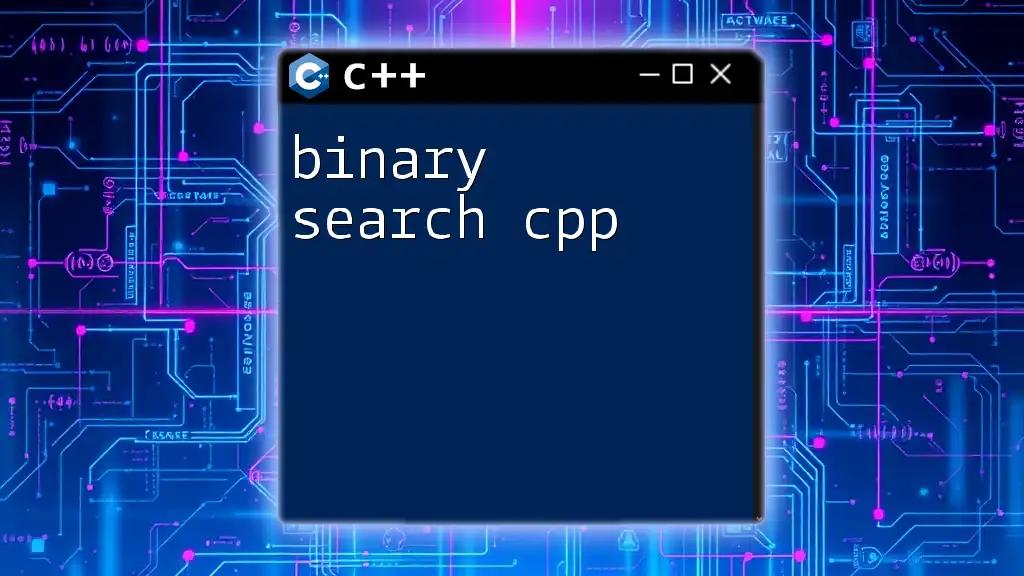
Importance of Learning Linear Search in C++
Understanding linear search in C++ is essential for budding programmers for several reasons. Firstly, it lays the groundwork for more complex algorithms. Grasping the fundamentals of searching operations will enhance your ability to understand and implement more advanced algorithms, such as binary search or search algorithms employed in data structures like trees.
Moreover, compared to other searching algorithms, linear search is particularly advantageous when dealing with smaller datasets or unsorted arrays. It inherently doesn't require preprocessing or pre-sorting, making it easier to apply in real-world scenarios where speed and complexity are less critical.

How Linear Search Works
The linear search algorithm operates by checking each element of the dataset linearly, from the beginning to the end. Here’s the basic process it follows:
- Start at the first element of the array.
- Compare the current element with the target value.
- If they match, return the index of the current element.
- If they don't, move to the next element and repeat the comparison.
- If the end of the array is reached without finding the target, return a value indicating that the target isn’t present (usually -1).
In terms of performance, linear search exhibits different scenarios:
- Best case: O(1) - The target is the first element.
- Average case: O(n) - On average, half of the elements are checked.
- Worst case: O(n) - The target is the last element or not present at all.
Visual Representation of Linear Search
To conceptualize linear search, consider this flow:
- Input an array and a target value.
- If the current element equals the target, return the index.
- If not, continue through the array.
- If the end of the array is hit, return -1.
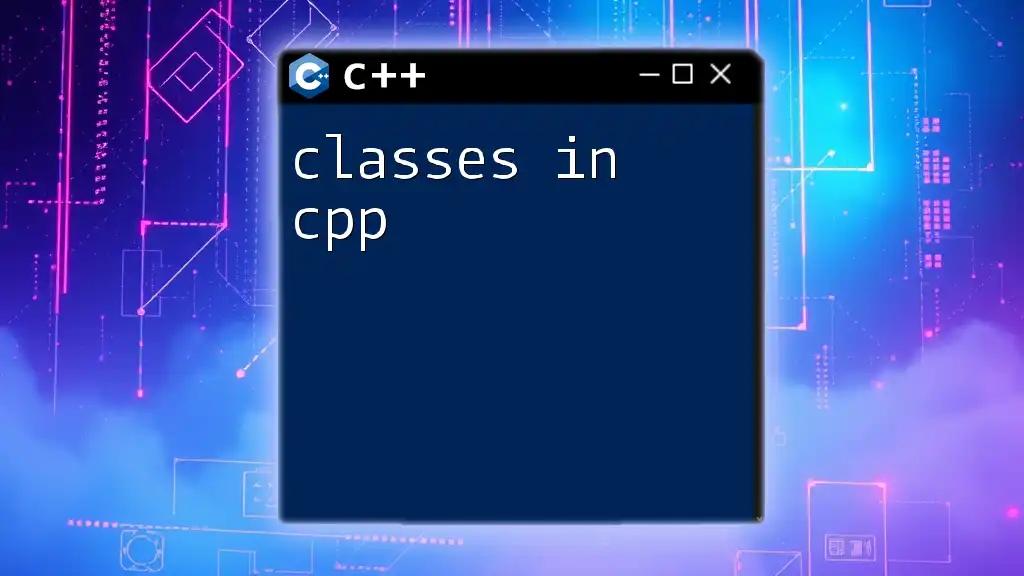
C++ Implementation of Linear Search
Basic Structure of a Linear Search Function
A linear search function in C++ typically consists of three components:
- Parameters: The array to search, its size, and the target value.
- Return type: An integer indicating the index of the target or -1 if not found.
Code Snippet: Basic Linear Search Function
Here’s how to implement a linear search function in C++:
#include <iostream>
using namespace std;
// Function to perform linear search
int linearSearch(int arr[], int size, int target) {
for (int i = 0; i < size; i++) {
if (arr[i] == target) {
return i; // return index if target is found
}
}
return -1; // return -1 if target not found
}
The `linearSearch` function is initiated with parameters for `arr`, `size`, and `target`. The loop iterates through each element of the `arr`, comparing it with the `target`. Upon finding a match, the function returns the index of the matched element; otherwise, it returns -1 if no match is found after checking all elements.
Example Usage of Linear Search in C++
To illustrate how to use the linear search function, consider the following example:
int main() {
int arr[] = {2, 4, 6, 8, 10};
int target = 6;
int size = sizeof(arr)/sizeof(arr[0]);
int result = linearSearch(arr, size, target);
if (result != -1) {
cout << "Element found at index: " << result << endl;
} else {
cout << "Element not found." << endl;
}
return 0;
}
In this code snippet, we defined an integer array, `arr`, and assigned it a target value of 6. The size of the array is computed, and the `linearSearch` function is called. Depending on the function's return value, the appropriate message is printed to indicate whether the target was found.
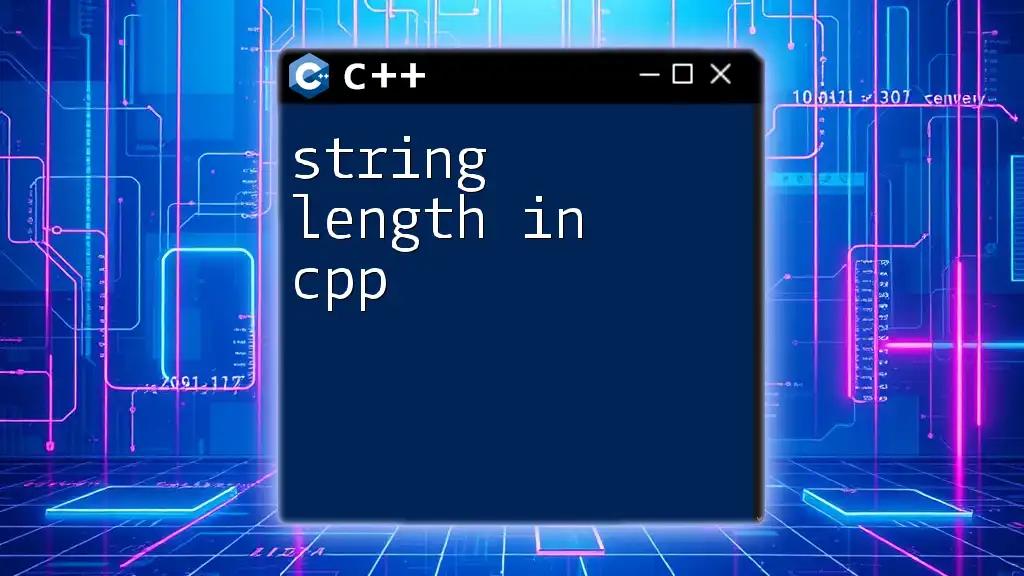
Performance Analysis of Linear Search
Time Complexity
Time complexity is a critical measure of the efficiency of an algorithm. For linear search, the worst-case time complexity is O(n), meaning that in the worst-case scenario, the algorithm must traverse the entire array to find the target element or determine that it isn't present. The best-case time complexity is O(1) when the target is the first element examined.
Space Complexity
In terms of space complexity, linear search is very efficient, boasting O(1) space complexity. This indicates that the algorithm does not require any additional storage proportional to the input size — it only uses a constant amount of space.
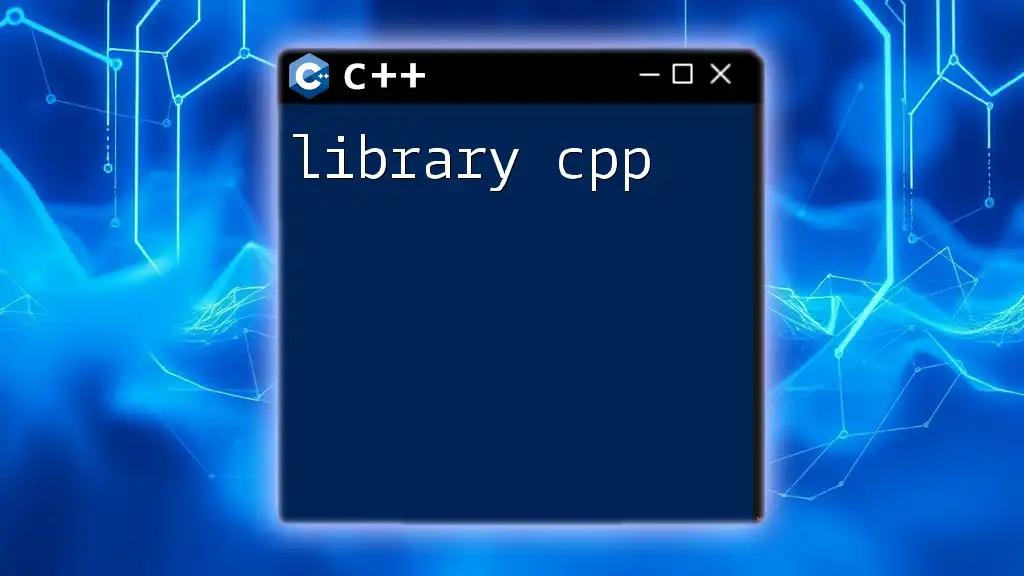
Pros and Cons of Linear Search
Advantages of Linear Search
- Simplicity of Implementation: The linear search algorithm is easy to code and understand, making it ideal for novice programmers.
- No Preprocessing Required: There’s no need to sort the data beforehand, allowing for immediate implementation on unsorted arrays.
Limitations of Linear Search
- Efficiency: Linear search can become inefficient with larger datasets since it checks each element individually.
- Comparison with Other Algorithms: In scenarios with sorted data, algorithms like binary search provide significantly better performance (O(log n) time complexity).

Practical Applications of Linear Search
Real-World Scenarios Where Linear Search Is Useful
Linear search shines in situations involving small amounts of data, such as:
- Validating user inputs.
- Searching in small lists or datasets where the overhead of more complex algorithms may not be justifiable.
When to Use Linear Search Over Other Algorithms
Linear search is often more suitable for unsorted data or small datasets. In contrast, when working with larger or sorted datasets, consider using more advanced algorithms like binary search for improved efficiency.
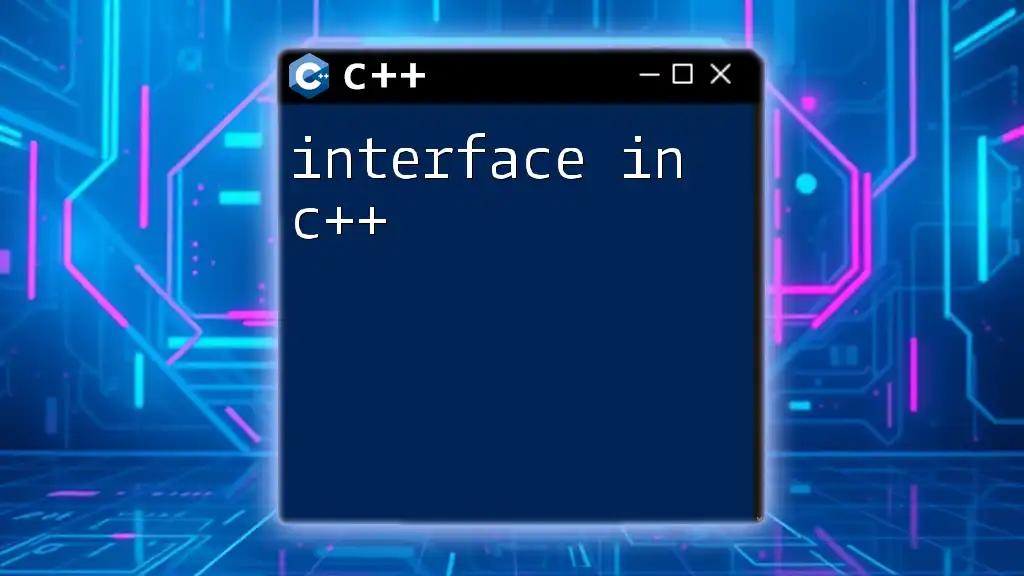
Conclusion
To wrap up, linear search in C++ is a foundational algorithm that serves multiple purposes, particularly for beginners. Its straightforward approach provides clarity on how searching algorithms function at a fundamental level. Through appropriate understanding and application, linear search can be a powerful tool in your programming arsenal.
For further exploration of C++ and other programming topics, resources such as dedicated literature, online courses, and coding forums can provide greater depth in knowledge and skill enhancement.
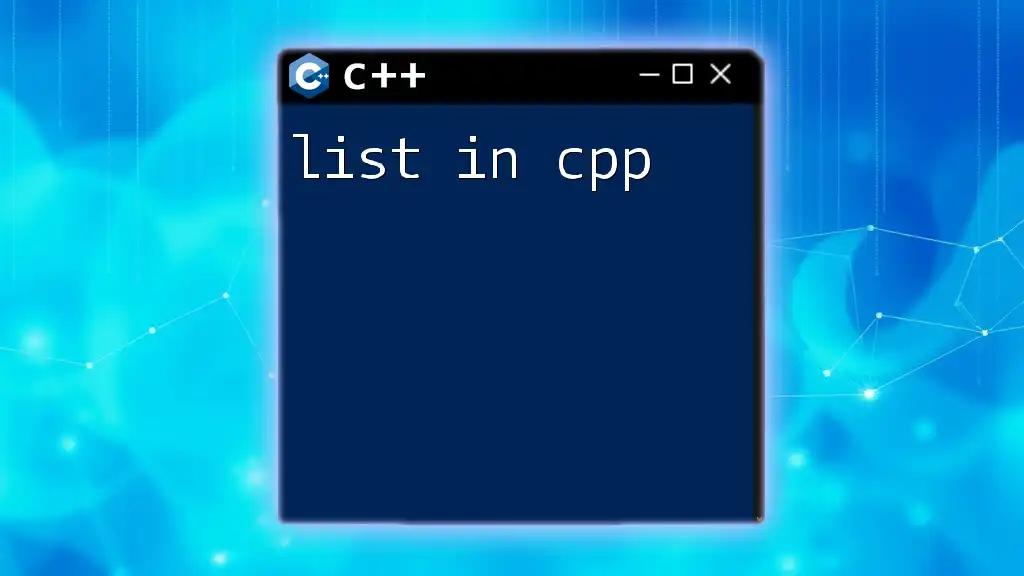
Call to Action
Now that you have a comprehensive understanding of linear search in C++, why not implement it in your next project? Experimenting and applying this algorithm can significantly boost your proficiency in coding. Don’t forget to subscribe for more insightful tips and tutorials on mastering C++ commands and coding practices!